FUNDAMENTALS A Complete Guide for Beginners
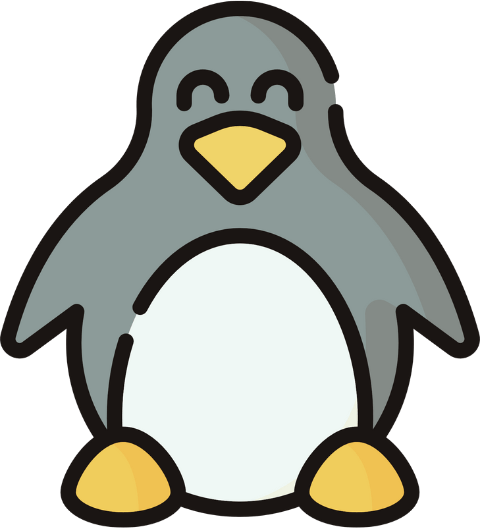
In bash scripting, string manipulation is a process of performing several operations to use and modify the value of a given string. For creating dynamic scripts, parsing information, and task automation, string manipulation is an important concept. Dive into the article to understand bash string manipulation and its multiple processes.
What is String Manipulation in Bash?
In bash scripting, a string is a sequence of characters that is enclosed by either a single quote, double quote, or even no quotations. String manipulation refers to shaping and modifying the textual data according to your will. A bash user needs to know about string manipulation to convert the string case and type, search a specific part of a substring from a large string, or even replace and remove a specific part of a string.
9 Practical Cases of String Manipulation in Bash
There are two ways to manipulate a string one is fundamental bash techniques such as parameter expansion, length of string, and string extraction and another is using powerful external commands like awk, sed, cut, and tr. In this section, 9 practical cases of string manipulation will be discussed. Explore the section to learn string manipulation.
1. Convert Bash String to Integer Value
Attempting to transform a string into an integer, successful conversion is only achievable for strings that exclusively consist of numerical characters. If you try to convert a string that contains non-numeric elements or pure string into an integer, the conversion will not result in numerical values. To convert a string to an integer, follow the script below:
#!/bin/bash
string_number="123"
integer_number=$(expr "$string_number" + 0)
echo "Integer: $integer_number"
Firstly the script declares a number as a string in a variable string_number
. Then in integer_number=$(expr "$string_number" + 0)
theexpr
command converts the string into a number. Adding0
with the string forces bash to interpret the string as an integer and perform the arithmetic expression. Later, the echo command prints the string as a number into the terminal.
2. String Case Conversion in Bash
Case conversion refers to converting the case of the character in the string from upper case to lower case and lower case to upper case. When you need to change the case of a character, you can follow the methods below:
i. Convert String to Lowercase
To convert the upper case character with a lower case character, you can use the tr command. In bash scripting, the tr command is used to translate and delete characters. Take a look at the following code to convert a string from upper case to lower case:
#!/bin/bash
Original_string="HELLO WORLD"
lowercase_string=$(echo "$Original_string" | tr '[:upper:]' '[:lower:]')
echo "Original String: $Original_string"
echo "Lowercase String: $lowercase_string"
At first, the bash script declares a string in a variable namedOriginal_string
. In the lowercase_string=$(echo "$Original_string" | tr '[:upper:]' '[:lower:]')
, the | (pipe) symbol pipes the output of theecho
command to thetr
command. Then the tr command matches the upper-case characters and replaces them with lowercase characters.
From the output, you can see the script converts the characters from upper case to lower case.
Note: The eval is a powerful tool and a part of the POSIX. It acts as an interface either as a shell built-in or external command. Here I have shown an example of converting the case of a string using eval:
#!/bin/bash
Original_string="HELLO WORLD"
conversion_command='tr "[:upper:]" "[:lower:]"'
eval "lowercase_string=\$(echo \"$Original_string\" | $conversion_command)"
echo "Original String: $Original_string"
echo "Lowercase String: $lowercase_string"
#Output: Original String: HELLO WORLD
#Lowercase String: hello world
The eval will dynamically execute a string as a shell command. Here the string is lowercase_string=\$(echo \"$Original_string\" | $conversion_command)
. So the script returns the output of the string that is declared after the eval and prints it using the echo
command.
ii. Convert String to Uppercase
To convert the characters of a string from lowercase to uppercase, use the following code:
#!/bin/bash
lowercase="hello world"
uppercase=$(echo "$lowercase" | tr '[:lower:]' '[:upper:]')
echo "Uppercase: $uppercase"
The|
(pipe) operator takes the echo
command output as input for thetr
command. In the tr '[:lower:]' '[:upper:]'
, the tr command translates the lower-case characters into uppercase characters.
The output shows the script successfully converts the case from lower case to upper case.
3. Replace Specific Characters of Bash String
To replace a specific character in a string with your desired character you can use the sed command. Check the following bash script:
#!/bin/bash
Main_string="Hello World"
New_string=$(echo "$Main_string" | sed -e 's/H/h/g' -e 's/W/w/g')
echo "Main_string:" $Main_string
echo "Modified_string:" $New_string
Firstly the bash script declares a string in a variable namedMain_string
. Then in the $(echo "$Main_string" | sed -e 's/H/h/g' -e 's/W/w/g')
, the pipe operator takes the output of the echo
command and pipes it as input to the sed command. Thesed
command replaces the H
withh
andW
withw
globally. Later, the bash script stores the modified string in the New_string variable.
The output shows that the script replaces the first character of the words from upper case to lower case.
Replace a Substring with Another String:
To replace a set of characters or substrings from a string, you can copy the following script:
#!/bin/bash
if [ "$#" -ne 3 ]; then
echo "Usage: $0 <original_string> <substring_to_replace> <replacement_string>
exit 1
fi
original_string="$1"
substring_to_replace="$2"
replacement_string="$3"
new_string=$(echo "$original_string" | sed "s/$substring_to_replace/$replacment_string/g"
echo "The original_string:$original_string"
echo "The substring to replace:$substring_to_replace"
echo "The replaced_string:$replacement_string"
echo "The new string:$new_string"
At first the if block checks whether the input has three arguments or not. If not then it shows a usage message and exits. Otherwise, the script assigns the first, second, and third arguments to the variables named original_string,substring_to_replace, and replacement_string respectively. Later, thesed
command replaces the substring with the replacement_string.
From the image you can see, that the script takes the input of the original_string, then the substring_to_replace, and later the replacement_string. And returns the modified output of the original_string.
4. Cut a Substring from a String in Bash
To cut or extract a specific substring, you can use the cut command. In bash, the script uses the cut command to extract a specific section from a line of the input. The basic syntax of the cut command is cut [option] [file]
.
Follow the script below to cut a Bash string:
#!/bin/bash
original_string="Today is $(date)"
substring=$(echo "$original_string" | cut -c 9-40)
echo "Original String: $original_string"
echo "Substring: $substring"
At first, the $(echo "$original_string" | cut -c 9-40)
, extracts a string from the original_string. Then the pipe
operator takes the output of the echo
command to the cut command. After moving to the cut
command, the bash script uses the -c
option that specifies substring extraction based on position. Finally, the cut command extracts a substring from the 9
th to the 40
th position.
The script returns the current date which is a part of the original string.
Truncate Bash String
Truncating refers to shorting or cutting a string to a desired length or size. As you can cut a string to extract a substring, you can also extract a specific size from a string. To truncate a bash string into a specific size, check the following script:
#!/bin/bash
original_string="This is a long string with some special characters: áéíóú"
# Set the desired byte length
max_bytes=20
# Truncate the string into the specified byte length
truncated_string=$(echo -n "$original_string" | cut -c1-"$max_bytes")
echo "Original String: $original_string"
echo "Truncated String: $truncated_string"
Initially, the bash script declares a string namedoriginal_string
. Then the script sets the byte length into a variable named max_bytes which is set at20
bytes. Finally, the cut command extracts characters from the beginning to the max_byte.
From the output, you can see the script returns a truncated string size 20 bytes.
5. Trim White-Spaces from Bash String
In Bash scripting, trimming is the process of removing the whitespaces from the beginning and end of a string. If you have a string that contains unexpected white spaces, then you can follow the script to trim the string:
#!/bin/bash
original_string=" Hello, World! "
trimmed_string="${original_string#"${original_string%%[![:space:]]*}"}"
trimmed_string="${trimmed_string%"${trimmed_string##*[![:space:]]}"}"
echo "Original String: '$original_string'"
echo "Trimmed String: '$trimmed_string'"
The bash script declares a string named original_string which contains leading and trailing spaces. Here, the${original_string%%[![:space:]]*}
will remove the leading space. Moving to the next syntax, the${trimmed_string##*[![:space:]]}
will delete the trailing spaces.
The output of the script shows the string does not contain any leading and trailing spaces anymore.
6. Remove the First Character of a String
To remove a specific character from a string you can use thesed
command. Use the following script for a practical approach:
#!/bin/bash
new_string="Removing the first character."
echo "Original String: $new_string"
echo "$new_string" | sed 's/^.//'
In the "$new_string" | sed 's/^.//'
syntax, the sed
command removes the first character of the echo command’s output. Here, the ^.
finds the first character and then removes it using //
.
The output shows the returned string from the script does not contain the first character.
Remove the Last Character of a String
To remove the last character from a string, you can use the same script as before, which you used for removing the first character. Only you have to replace the^.
with$.
to remove the last character. Check the following script below:
#!/bin/bash
new_string="Is it the last character?"
echo "Original_string: $new_string"
echo "$new_string" | sed 's/.$//'
The "$new_string" | sed 's/.$//'
syntax removes the last character of the input string. In the sed command, .$
finds the last character and removes it.
The script successfully removes the last character from the input string.
7. Generate a Random String in Bash
The random string refers to the sequence of random characters which is important for uniqueness, security, and cryptographic operations. To generate a string, follow the script below:
#!/bin/bash
random_string=$(echo "$RANDOM" | md5sum | cut -c 1-10)
echo "$random_string"
At first, the$RANDOM
creates a random number between 0 to 32767. Then the script prints the random number using theecho
command. The|
operator pipes the output to the md5sum
command which will calculate a 128-bit MD5 sum check. Later the output of the md5sum is piped to the cut
command that will extract characters from 1 to 10 position.
From the output, you can see the script generates a random string.
As you learned to generate a random string, next let’s see how you can write and save a string to a file. Take a look at the following process of how to write a string to a file:
#!/bin/bash
# Writing a string to a file, overwriting its content if the file exists
echo "Hello, World!" > output.txt
# Appending a string to a file
echo "This is a new line." >> output.txt
Firstly the redirection operator >
redirects the output to a new file named output.txt. If there is no existing file named output.txt then the script will create a new txt file otherwise it will overwrite the existing file. Then the>>
operator appends a new string to the existing file.
To see the content of the new file, use the following code:
cat output.txt
The output shows the script writes a new line “Hello, World!” to a file. Then it appends another line to that existing file.
8. Manipulate Bash String Arrays
A string array is a collection of multiple string values that are in a single variable entity. It is important for data storage, file handling, and data validation and verification. Here I will show how to declare, access, print, and iterate the elements of a string array. Check the script below:
#!/bin/bash
# Declare an array
my_array=("apple" "banana" "orange" "grape")
# Access elements by index
echo "First element: ${my_array[0]}"
echo "Second element: ${my_array[1]}"
# Print all elements
echo "All elements: ${my_array[@]}"
# Iterate over elements
echo "Iterating over elements:"
for fruit in "${my_array[@]}"; do
echo "$fruit"
done
# Get the length of the array
array_length=${#my_array[@]}
echo "Array length: $array_length"
At first, the bash script declares an array with four elements in a variable named my_array
. After that with the${my_array[0]}
syntax, the script prints the first elements of the array. Also with the${my_array[@]}
syntax, it prints all the elements of the array. To iterate over elements, the script uses the for loop. With each iteration, the loop will print one element. Lastly, with the {#my_array[@]}
syntax, the script finds the length of the string.
The image shows all the outputs of the script which includes the accessed elements, iterating output values, and the length of the array.
9. Extract the First Character of a Bash String
To extract the first character of a string, you can use parameter expansion. Inside the curly bracket use the variable from which you want to extract the character and specify the extraction position and length of the extracted characters. Follow the script that has been shown below:
#!/bin/bash
str="The username."
char=${str:0:1}
echo $char
The${str:0:1}
syntax, will print the first character of the input string. It shows the extraction starts at the0
position and it extracts one character as the length is set at 1.
Conclusion
The article shows a detailed overview of string manipulation that will help you understand the process of handling and modifying a string efficiently. Mastering string manipulation unlocks the collection of text data processing and analysis. Hope this article clears your concept of string manipulation and empowers your work with textual data.
People Also Ask
What is string manipulation in shell scripting?
In bash shell scripting, string manipulation refers to performing various operations using fundamental bash techniques and external commands to modify the string according to your preference.
How do you manipulate variables in Bash?
To manipulate variables in bash you can use parameter expansion to access, match patterns, and extract a specific part from the variable. To compare two variables you can also be able to use the conditional operator. In bash scripting external commands like awk, sed, and tr also enrich your ability to manipulate variables.
How to find the length of a string?
To find the length of a string you can use the # operator with parameter expansion. The syntax of the expansion is {#String}
where the String is a variable that stores the input string. Check the script to find the length of a string:
#!/bin/bash
String="This is the new string. The length of the string has been calculated."
length="${#String}"
echo "The length of the string is: $length"
#Output: The length of the string is: 69
How to concatenate two strings in bash?
To concatenate two strings in bash you can use the +=
operator. Without changing the existing string, the +=
operator mainly appends the new string to the right of the existing string. Follow the script to concatenate strings:
#!/bin/bash
echo "Using Simple Concatenation"
string="Hello"
string+="1234"
concatenated_string="$string"
echo "Concatenated String:$concatenated_string"
#Output:Using Simple Concatenation
#Concatenated String:Hello1234
How can I reverse a string?
To reverse a string use the rev
command. The rev command reverses each character of a file or the output of the left-hand side command of the pipe operator. Follow the script to reverse a string:
#!/bin/bash
original_string="Hello, World!"
reversed_string=$(echo "$original_string" | rev)
echo "Original String: $original_string"
echo "Reversed String: $reversed_string"
#Output:Original String: Hello, World!
#Reversed String: !dlroW ,olleH
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to Bash String | Bash Scripting Tutorial