FUNDAMENTALS A Complete Guide for Beginners
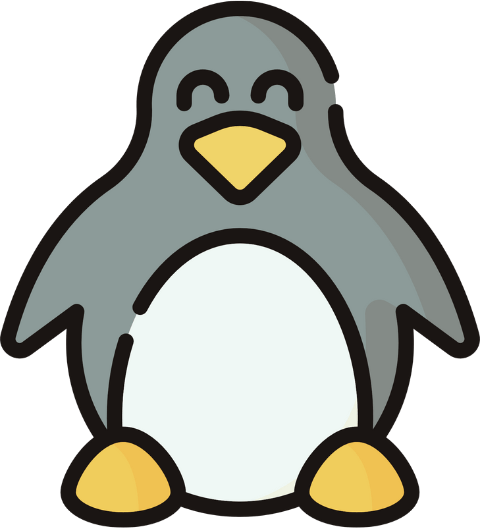
In bash scripting, string formatting is important for increasing readability and enhancing the presentation. There are different types of formatting such as aligning text, inserting placeholders, and modifying the appearance of strings. Let’s dive into the article to understand what string formatting is and how to format a bash string.
What is Bash String Formatting?
Bash string formatting means changing the look of the input text. It helps to make the text easier to read and better suited for certain tasks by using multiple methods. For example, you want to return a text that is the combined form of two strings and want the string to be right aligned. String formatting mainly includes string concatenation, padding, case modification, and string substitution.
A. Format String Using “printf” Command in Bash
The printf command in Linux is a tool to print the string, number, and special characters in the terminal. This command provides more formatted and flexible output than the echo command. The basic syntax of the printf command is:
printf [-v var] format [arguments]
Here the [-v var]
is optional. In the var variable, you can store the formatted output in it. The format is a string that specifies how the output will be formatted. The format string can contain a placeholder %s
for str and %d
for integer. Then [arguments]
replace the placeholder in the output string.
Format Specifier of “printf” Command
A format specifier is a placeholder for defining the datatype and formatting. The specifier starts with % followed by data type and format. Take a look at the following section:
- %s: Insert a string
- %d: Insert an integer
- %f: Insert floating-point number
- %c: Insert a character
- %u: Insert an unsigned character
- %%: Print the % symbol
Some other format specifiers are %x to insert numbers in hexadecimal format and here the hexadecimal letters are in lowercase whereas %X is for uppercase hexadecimal letters.
Flags and Modifiers to Format String in Bash
Using flags and modifiers in the printf command, you can format a string in various ways. There are different types of flags which include the minus sign(-) left justifies the string within the field width, the plus sign(+) prefixes the output with a signed value, zero padding(0), and hash(#) format the output like representing into hexadecimal value. To format the output for understanding better, you can use modifiers which include width modifiers for example %10s to specify the width 10, and precision modifier (%.pf) to specify the maximum number of characters to print from a string. Check the following script:
#!/bin/bash
# Define a string
string="Hello"
# Left-align the output within a field width of 10 characters
printf "Left align:%-10s\n" "$string"
# Right-align the output within a field width of 10 characters
printf "Right align:%10s\n" "$string"
# Always include a sign (+ or -) in the output
number=10
printf "Including a sign: %+d\n" "$number"
# Pad the output with zeros instead of spaces
number=7
printf "Zero padding: %04d\n" "$number"
# If the first character isn't a sign, prepend a space
number=10
printf "Prepending a space: % d\n" "$number"
# Used with certain format specifiers to modify the output format (Example: hex output)
number=15
printf "Hexadecimal representation: %#x\n" "$number"
Firstly, the script declares the input string in a variable named string
. The %-10s\n
format specifier indicates the left alignment with. After that, the script uses 10
which indicates a field width of 10. To denote string, the script uses %s
. And prints the modified output. Using the 10
with no character, you can align the string to the right. To format the string with a +
or -
sign. Once the script inputs the sign then it follows the data type specifier. With %04d\n
, the script pads 0
with minimum field width 4
instead of space. Then the % d\n
, prepend a space before the input number. At last, the %#x\n
, modifies the output format by adding the “0x” prefix.
The script has adjusted the alignment of the string, included signs, padded with zeros, and modified the output format of strings and numbers.
2 Practical Examples of Format String Using “printf” Command
To learn effective formatting and a better understanding, practical examples are important in bash scripting. Here take a look at the two practical examples for a better understanding of formatting:
1. Format Table in Bash
To format a table in bash using the printf
command, check the following script:
#!/bin/bash
# Define table data
printf "%-10s %-15s %-10s\n" "Name" "Age" "Gender"
printf "%-10s %-15s %-10s\n" "Alice" "30" "Female"
printf "%-10s %-15s %-10s\n" "Bob" "25" "Male"
printf "%-10s %-15s %-10s\n" "Charlie" "35" "Male"
Firstly, the script declares the header using the printf
command. To specify the column width, the script uses the %10
,%15
, and %10
widths ensuring the left alignment with “-”. Similarly, the script creates all three rows and columns with specific elements. The printf command prints all the elements for each individual.
The output shows the script has created a formatted table that contains 3 columns and 4 rows including the header.
2. Format Dates in Bash
To format the current date to year, month, and time, you can use the printf
command. Check out the following script:
#!/bin/bash
# Get the current date
current_date=$(date +"%Y-%m-%d %H:%M:%S")
echo "Current date and time: $current_date"
In the current_date
variable, the script uses the date command to obtain the current date and time. After that, the %Y-%m-%d %H:%M:%S
option specifies the year (%Y), month(%m), day (%d), hour (%H), minute( %M) and second (%S). At last, the echo command prints the formatted output to the terminal.
The image shows the script has formatted the output according to year, month, date, and time.
B. Format String Using Parameter Expansion
Using the parameter expansion, you can easily format and manipulate strings. Parameter expansion provides extensive formatting which includes substring extraction and matching, case modification, and adding two strings. To format a string using parameter expansion, take a look at the following approaches:
1. Format String to Convert Case
When you want to format the output in lowercase or uppercase, you can use the parameter expansion to do this. To change the case of a string using parameter expansion, copy the following script:
#!/bin/bash
string="Hello World"
# Convert the string to uppercase
uppercase_string=${string^^}
# Convert the string to lowercase
lowercase_string=${string,,}
echo "Uppercase string: $uppercase_string"
echo "Lowercase string: $lowercase_string"
Firstly, the operator ^^
, inside the parameter expansion converts all the characters to uppercase. On the other hand, the ,,
operator converts all the uppercase characters to lowercase.
The output shows the script formatted all the characters of the input string to both uppercase and lowercase.
2. Format String by Extracting Substring
To format and manipulate the text of a string, you can extract a specific length of string using parameter expansion. Take a look at the following script below:
#!/bin/bash
string="Hello World"
# Extract the first 5 characters of the string
substring=${string:0:5}
echo "Substring: $substring"
With the ${string:0:5}
, the script extracts a substring starting from index 1
of the input string and ending with index 4
, the fifth character. The script stores the output in a variable named substring
.
From the input string “Hello World”, the script extracts a substring “Hello”.
3. Format String by Replacing String
Replacing a string from the input string is the command task in bash scripting. To format string you can replace a string according to your need. Check the following script:
#!/bin/bash
string="Hello World"
# Replace "Hello" with "Hi" in the string
new_string=${string/Hello/Hi}
echo "New string: $new_string"
Using ${string/Hello/Hi}
, the script matches the first occurrences of the substring and replaces the string hello
with hi
. The script stores the modified output in a string named new_string
.
The script replaces the string “Hello” with another string “Hi”.
4. Format String by Concatenating Two Strings
Concatenation generally refers to appending the new string to the right of the existing string. To format a string by concatenating two strings using parameter expansion, check out the following script below:
#!/bin/bash
echo "Using Listing"
string1="The first string."
string2="This is a new string."
concatenated_string=${string1}${string2}
echo "Concatenated String:$concatenated_string"
At first, the script declares two input strings in two variables named string1
and string2
. The ${string1}${string2}
concatenates two strings by placing the string side by side. The script stores the output in a variable named concatenated_string
.
The script appends the string2 “This is the new string” to the right of the existing string “The first string”.
How to Generate and Print Sequential Strings?
To generate sequential strings, you need to use the seq
, a command-line utility. For generating ranges of values, the bash script uses the seq inside the looping construct. Check the following code to create a string sequence:
#!/bin/bash
foo=$(seq 1 1 100)
for i in $foo; do
echo "input${i}_now"
done
At first, the script declares the seq
command to generate a sequence of numbers from 1
to 100
with an increment of 1
. The loop variable iterates over each element in the variable foo. Inside the loop, the echo
command prints each string at each iteration.
The image shows that the script generates and prints a sequence of strings from 1
to 100
.
Error Handling While String Formatting in Bash
While handling the special characters in formatting string, some important points should be kept in mind. Take a look at the overview of error handling in the following section:
Incorrect Format Specifier
Using an incorrect format specifier with the printf command, you will find unexpected results. For example, if you want to print a string but use an integer format specifier then it is unable to interpret “James” as an integer and treats the non-integer value as 0
. Look at the code below:
#!/bin/bash
printf "%d\n" James
The script has returned 0 instead of the string “James”.
So, to avoid these types of unexpected results, use the correct format specifier. For example, to print a non-integer value you have to use %s
, and to print an integer value, use the format specifier %d
.
Missing Arguments
Another common error happens when you use the format specifier but do not provide enough input arguments. For example, if you want to print the name and age of a person but provide one argument, then it will replace the second specifier with 0
. Take a look at the following code:
#!/bin/bash
printf "Name: %s, Age: %d\n" James
The image shows the script has returned zero for the missing argument.
To fix the missing argument issue, you need to provide all the input arguments properly. Try this to fix the above error:
printf "Name: %s, Age: %d\n" James 30
So the script has returned the value of the input argument properly.
While using the printf command, ensure that you have provided all the input arguments to match all the format specifiers.
Handling Special Characters
While printing special characters like backslash, and single and double quotes, you need to escape the characters using backslash(\). Check out the following script to handle special characters:
#!/bin/bash
printf "Message: %s\n" "Hello, \"World\"!"
Here, the script escapes the double quotes using the backslash(\).
Considerations for Formatting Strings
Here are some suggestions for formatting strings in bash:
- While using the printf command, ensure that your input arguments match the expected format specifier.
- When formatting string using parameter expansion and other external commands, ensure safety by creating a file backup.
Conclusion
The article shows an overview of formatting bash string in detail. It reflects two methods of formatting string which are using the printf command and another is parameter expansion. The printf command shows extensive results in formatting strings such as formatting alignment, padding number, and include sign. On the other hand, parameter expansion helps in replacing strings, converting cases, and extracting substrings. You can also use other external commands such as sed, awk, and perl instead of the parameter expansion.
People Also Ask
How to Format a String in Bash?
To format a string in bash, you can use the printf
command by specifying the output using the format specifier and placeholder. For formatting tasks such as replacing the string, extracting substring, and concatenating strings, use the parameter expansion as well as other external commands such as sed
, awk
, perl
.
How to print formatted strings in Bash?
To print a formatted string in bash, you can use the printf
command. The printf command takes input arguments and substitutes the placeholders in the formatted string. Take a look at the following script:
#!/bin/bash
name="Alice"
age=30
printf "Name: %s\nAge: %d\n" "$name" "$age"
#output:Name: Alice
#Age: 30
How do I edit a string in Bash?
To edit a string in bash, you can use various manipulation techniques like parameter expansion and external commands such as sed
, awk
, and Perl
. By extracting and removing substring, you can easily edit an input string. For example, if you want to replace a specific substring with another, you can use the following script:
#!/bin/bash
string="Hello, World!"
replaced="${string/World/Universe}"
#Output: Hello, Universe
How to convert formatted string to date?
To convert a formatted string to date you can use the date command with the -d
option. The -d option specifies the date string. Then you can specify the format for the desired output. Check out the following script below:
#!/bin/bash
formatted_date="2024-02-22"
date_obj=$(date -d "$formatted_date" +"%a %b %d %T %Z %Y")
echo "Date string: $formatted_date"
echo "Date object: $date_obj"
#output: Date string: 2024-02-22
#Date object: Thu Feb 22 00:00:00 +06 2024
How do you convert timestamps to minutes?
To convert the timestamps to minutes, first find the difference between the two timestamps. The output will be in seconds. So to convert the output to minutes, divide the difference by 60 which will convert the time into minutes. Check out the following code:
#!/bin/bash
# Example timestamps in seconds
timestamp1=1645484400 # February 22, 2022, 08:00:00 UTC
timestamp2=$(date +%s) # Current timestamp
# Calculate the difference in seconds
difference=$((timestamp2 - timestamp1))
# Convert seconds to minutes
minutes=$((difference / 60))
echo "Timestamp 1: $timestamp1"
echo "Timestamp 2: $timestamp2"
echo "Difference in minutes: $minutes"
#Output:Timestamp 1: 1645484400
#Timestamp 2: 1708579462
#Difference in minutes: 1051584
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial