FUNDAMENTALS A Complete Guide for Beginners
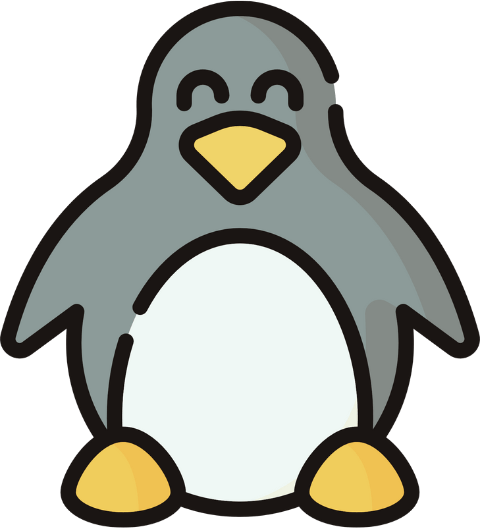
In Bash scripting, case conversion is important for case-sensitive comparison, pattern matching, and file and directory operations. So for an effective and efficient conversion of Bash string to lowercase, a clear concept of case conversion is essential.
To convert a Bash string to lowercase, check the following methods:
- Using Parameter Expansion:
${String,,}
- Using “tr” command:
tr [Option] [set1] [set2]
, here the tr command translates or deletes set1 with set2. - Using declare command:
declare -l variable_name
- Using awk command:
echo "input_str" | awk 'pattern { action }'
- Using sed command:
sed 's/pattern/replacement/' filename
- Using perl command:
echo "$input_string" | perl [Option] 'action'
- Using ASCII value with a case statement and a for loop.
Dive into the article to learn these methods of how to convert a bash string to lowercase in detail.
7 Methods to Convert a String to Lowercase
The basic and simple way to convert a string’s characters to lowercase is by using the tr
command. There are some other commands like awk
, sed
which gives flexibility while converting multibyte characters. In this section, 7 methods will be discussed which have been mentioned earlier on how to convert a string to lowercase.
1. Using Parameter Expansion With “,,” Operator
To convert a string to lowercase, you can use parameter expansion. Parameter expansion refers to retrieving and manipulating the value that is stored in the parameter or variable. By using parameter expansion with,
,,,
, you can convert the first character of a string to lowercase, as well as convert all the characters in the string to lowercase.
A. Convert All Characters to Lowercase
To convert all characters of a string to lowercase using,,
, follow the below script:
#!/bin/bash
original_string="HELLO WORLD"
lowercase_string="${original_string,,}"
echo "Original String: $original_string"
echo "Lowercase String: $lowercase_string"
Firstly the script declares a string into a variable namedoriginal_string
. Then the script uses a bash parameter expansion syntax${original_string,,}
which converts all the characters of the original_string to lowercase. Later, the echo command prints the converted output in the terminal.
From the output, you can see the script converted all the characters of the input string to lowercase.
B. Convert the First Character to Lowercase
To convert only the first character to lowercase, replace the,,
with ,
in the code that you have used earlier to convert all characters to lowercase. Check the code:
#!/bin/bash
original_string="HELLO WORLD"
lowercase_string="${original_string,}"
echo "Original String: $original_string"
echo "Lowercase String: $lowercase_string"
The "${original_string,}"
converts the first character of the original_string to lowercase.
Here the script only converts the first character of the string to lowercase.
C. Convert a Specific Character Using Pattern
To convert a specific character using a pattern, check the following code:
#!/bin/bash
# Declare a string
string="HERE, ALL THE STRING IN UPPERCASE"
# Converts the first letter to lowercase if it’s ‘H’
echo "${string,H}"
# Convert all occurrences of 'L' to lowercase
echo "${string,,L}"
# Convert all occurrences of any of the characters in 'STRING' to lowercase
echo "${string,,[STRING]}"
Firstly the bash script declares a string named “string”. Here the ${string,H}
checks if the first character of the string is H
, then it will convert the found H
to lowercase. After that, the ${string,,L}
converts all the occurrences of the characterL
to lowercase. Lastly, the ${string,,[STRING]}
changes all the occurrences of any character in STRING to lowercase.
The script successfully converts the first character, a specific character, and a group of characters to lowercase.
Note: The use of ~
in parameter expansion allows you to switch the case of the first character, while ~~
switches the case of all characters. This results in a modification of the current character, alternating between lowercase and uppercase.
2. Using “tr” Command
To convert the upper case character to a lower case character, you can use the tr command. In bash scripting, the tr command is used to translate and delete characters. Take a look at the following script to convert a string from upper case to lower case:
#!/bin/bash
Original_string="ALL THE CHARACTERS ARE IN UPPERCASE"
lowercase_string=$(echo "$Original_string" | tr '[:upper:]' '[:lower:]')
echo "Original String: $Original_string"
echo "Lowercase String: $lowercase_string"
At first, the bash script declares a string in a variable namedOriginal_string
. In the lowercase_string=$(echo "$Original_string" | tr '[:upper:]' '[:lower:]')
, the | (pipe) symbol pipes the output of theecho
command to thetr
command. Then the tr command matches the upper-case characters and replaces them with lowercase characters.
From the output you can see, that the script converts the characters from upper case to lower case.
3. Using “declare” Command
While declaring a variable, if you use the -l
attribute with declare command then it will convert any value of the declared variable to lowercase. To convert a string to lowercase using the declare command, check the following script:
#!/bin/bash
# Declare a string and convert it to lowercase
declare -l str="CONVERTING THE STRING INTO LOWERCASE"
# Print the string
echo $str
At first, the bash script declares a string into a variable named str. Moreover, the script uses the -l option with the declare command which converts all the characters to lowercase. At last, the echo command prints the output of the str variable in the terminal.
The output shows that the declare command has converted the characters to lowercase.
4. Using “awk’ Command
To convert the case of a string to lowercase, you can use the awk command. The awk is a versatile command tool used for text processing, handling multi-byte characters, and especially for pattern matching and manipulation. The awk command uses a tolower function to change the case of the characters of a given string.
Here’s the script to convert the character of a string to lowercase using the awk command:
#!/bin/bash
# Define the input string
input_string="BASH LOWERCASE"
# Use echo to print the original string and then pipe it to awk for lowercase conversion
echo "$input_string" | awk '{print tolower($0)}'
The script uses |
(pipe) operator to take the output of theecho
command to theawk
command. In the awk '{print tolower($0)}'
, the awk command converts the echo command output to lowercase using its function tolower. In tolower($0)
, the ($0)
indicates the entire output from the pipe operator.
The script converts the characters to lowercase using the awk command with the tolower function.
5. Using “sed” Command
The sed command is a simple yet very useful command for stream editors in bash scripting. The sed command uses a special sequence that includes backslashes and one of the following letters L, l, U, u, E. These sequences have a special meaning, like:
- \L: Continue the replacement to lowercase until you find \U or \E.
- \ l: Turn the next character to lowercase.
- \U: Continue the replacement to uppercase until you find \U or \E.
- \u: Turn the next character to uppercase.
- \E: Stoped case conversion started by \U and \L.
The sed command mainly uses these sequences for case conversion.
To convert a string to lowercase using the sed command, follow the script below:
#!/bin/bash
# Method 1
med1=$(echo 'Bash Lowercase with SED' | sed -e 's/\(.*\)/\L\1/')
echo "Method1: $med1"
# Method 2
med2=$(echo 'Bash Lowercase with SED' | sed 's/.*/\L&/g')
echo "Method2: $med2"
Firstly in method 1, thesed
command takes the output through the pipe operator. In the sed command \(.*\)
captures the string and the\L
converts the strings’ characters to lowercase. Later in method 2, the script uses's/.*/\L&/g'
which also converts the characters to lowercase. It uses-g
flag which globally replaces all the characters in the output.
From the output, you can see the inputs’ uppercase characters have converted to lowercase.
Convert a Specific Character
To convert a specific character to lowercase using the sed command, take a look at the following script:
#!/bin/bash
Main_string="Hello World"
New_string=$(echo "$Main_string" | sed -e 's/H/h/g' -e 's/W/w/g')
echo "Main_string:" $Main_string
echo "Modified_string:" $New_string
In the $(echo "$Main_string" | sed -e 's/H/h/g' -e 's/W/w/g')
, the pipe operator takes the output of theecho
command and pipes it as input to the sed command. Thesed
command replaces the characterH
withh
andW
withw
globally. At last, the bash script stores the modified string in the New_string variable.
The output shows that the script replaces the first character of the words from upper case to lower case.
6. Using “Perl” Command
In bash scripting, the Perl command provides a simple way to convert strings to lowercase. The Perl command reads each line of the input then uses the lc function to convert the input to lowercase and later it displays the modified output. To convert a string to lowercase using the Perl command, check the following script:
#!/bin/bash
input_string="Bash Lowercase with Perl"
echo "$input_string" | perl -ne 'print lc'
In theperl -ne
command, the-n
option makes the perl command process the input line by line and the -
option executes the perl command in the terminal. Later, the 'print lc'
syntax, prints the converted lowercase of the input characters using the lc
function.
The output shows the script converts the uppercase characters B
, L
, and P
to lowercase b
, l
, and p
respectively.
7. Using the ASCII Value
To convert the case of a string to lowercase, you can also use the ASCII value of the characters and arithmetic operations. Here’s the script:
#!/bin/bash
lc(){
case "$1" in [A-Z])
n=$(printf "%d" "'$1")
n=$((n+32))
printf \\$(printf "%o" "$n") ;; *)
printf "%s" "$1"
;;
esac
}
word="THIS IS a ASCII value."
for((i=0;i<${#word};i++)) do
ch="${word:$i:1}"
lc "$ch"
done
printf "\n"
At first, the script declares a bash function named lc(). This function takes one input argument using the $1. In the function, the script uses a case statement that checks the uppercase character [A-Z] and converts them into lowercase by adding 32 to the ASCII value of uppercase characters. After converting to lowercase, the printf \\$(printf “%o” “$n”) prints the output using the octal form. If the character is not in uppercase, the *) syntax executes the later print command to print it as it is. In the for loop, the ${#word} syntax counts the length of the input string, and ch=”${word:$i:1}” syntax extracts a single character at each iteration and converts it to lowercase using the lc function.
From the image you can see the script converts uppercase characters of the string “THIS IS a ASCII value.” to lowercase characters, “this is a ascii value.”
Conclusion
To sum up, the article shows multiple methods to convert a string to lowercase. While dealing with multibyte characters, the tr command will not show the expected result. On the other hand, the awk and sed command shows flexibility while handling multibyte characters. In the conversion of case, parameter expansion also shows versatile results. Hope this guide will give you a clear concept of converting a bash string to lowercase.
People Also Ask
How to convert a bash string to lowercase?
To convert a Bash string to lowercase, you can use the tr command with the syntax: tr '[:upper:]' '[:lower:]'
. Simply use this syntax within a pipeline to convert uppercase input into lowercase. For instance, echo 'LINUX' | tr '[:upper:]' '[:lower:]'
, here sample output is: linux. Or, you can also feed the stdin file containing uppercase strings to the tr command by using input redirection. Like this, tr '[:upper:]' '[:lower:]' < file.txt
.
How to convert a string to uppercase?
To convert a string from lowercase to uppercase, you can use the tr command. See the following code for better understanding:
#!/bin/bash
Original_string="all the characters are in uppercase"
uppercase_string=$(echo "$Original_string" | tr '[:lower:]' '[:upper:]')
echo "Original String: $Original_string"
echo "Uppercase String: $uppercase_string"
#output:Original String: all the characters are in uppercase
#Uppercase String: ALL THE CHARACTERS ARE IN UPPERCASE
Which function converts a string to lowercase?
To convert a string to lowercase you can use {string,,}
and tr command. There is no direct function to convert a string to lowercase. You can use tolower
and lc
functions but for this, you need to use external commands like awk and perl.
How to convert a specific character between the same characters to lowercase in bash?
To convert a specific character to lowercase you can use the awk text processing command. Inside the awk command use the gsub function which stands for global substitution. With (/W/, "w", $2)
, the script converts the W
to w
of the word “WORLD”. Check the script below:
#!/bin/bash
# Declare a string
string="HELLO WORLD WITH MULTIPLE Ws"
# Replace only the second occurrence of 'W' with 'w'
echo "$string" | awk '{gsub(/W/, "w", $2)}1'
#output:HELLO wORLD WITH MULTIPLE Ws
How to convert a multibyte character to lowercase?
To convert a multibyte character to lowercase, you can use the Perl
command. Here with the Perl command use the -CS option to enable the UTF-8 mode. The lc($_)
function converts each character to lowercase. Here’s the script:
#!/bin/bash
#Converting the multibyte characters to lowercase
input_string="HELLO ÆØÅ"
lowercase_string=$(echo "$input_string" | perl -CS -pe '$_ = lc($_)')
echo "$lowercase_string"
#output: hello æøå
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial