FUNDAMENTALS A Complete Guide for Beginners
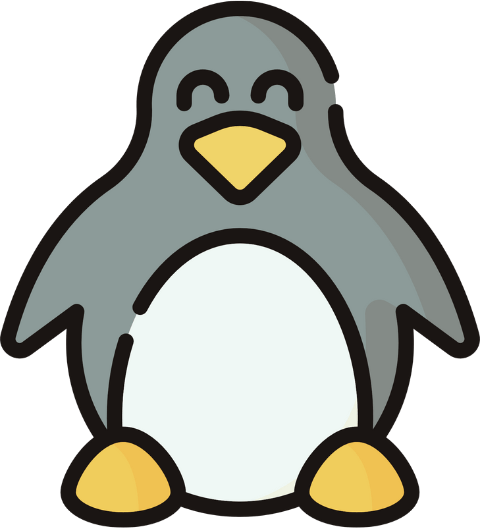
Truncate refers to making something shorter. In bash scripting, it means cutting the length or size of an input string or an input file to a specific size.
To truncate a bash string to a specific length, the available methods are:
- parameter expansion:
${parameter:offset:length}
- head command: a. With file:
head -c N filename.txt
b. With pipe:echo "input" | head -c N
- tail command: a. With file:
tail -c N filename.txt
b. With pipe:echo "input" | tail -c N
- sed command:
sed 's/^\(.\{N\}\).*$/\1/'
- cut command:
cut [options] [action] filename
Truncation of a string is important for formatting output, saving memory space, and managing data. So, dive into the article to learn how to truncate a bash string to a specific length in detail.
1. Using Parameter Expansion
You can truncate the Bash string by utilizing parameter expansion without any external command. Parameter expansion refers to retrieving and manipulating the value stored in the parameter or variable. Using the substring expansion${parameter:offset:length}
, you can easily extract the substring by setting the starting at a specified offset that truncates the rest of the string.
To truncate a string to a specific length in bash, check the following script:
#!/bin/bash
# Function to truncate string using parameter expansion
truncate_string() {
local string="$1"
local length="$2"
# Truncate string using parameter expansion
echo "${string:0:length}"
}
# Usage example
input_string="The string will be truncated to a specific length."
max_length=20
truncated=$(truncate_string "$input_string" "$max_length")
echo "Truncated string: $truncated"
Firstly, the script declares a function that takes two input arguments- the input string and the desired truncated string length. Using the{string:0:length}
, the script accesses the string, extracts a substring from the starting position of the first character, and ends with the character that is at themax_length
. At last, the script calls the truncate function with the input argument input_string
andmax_length
.
From the image, you can see, using the parameter expansion the script has truncated the input string into “The string will be t”.
Truncate a String of Multibyte Characters
The characters that need more than one byte of memory are called multibyte of characters. For example: Latin characters with diacritics, emoji, Asian characters, etc. To truncate a multibyte of characters, check the following script below:
#!/bin/bash
# Function to truncate string to a specific byte length
truncate_string() {
local string="$1"
local max_bytes="$2"
# Convert string to bytes and truncate if necessary
local truncated=""
local total_bytes=0
local char
for (( i = 0; i < ${#string}; i++ )); do
char="${string:i:1}"
char_bytes=$(printf '%s' "$char" | LC_ALL=C wc -c)
(( total_bytes += char_bytes ))
if (( total_bytes <= max_bytes )); then
truncated+="$char"
else
break
fi
done
echo "$truncated"
}
# Usage example
input_string="Multibyte áçñ😊.Others 😊ñçá."
max_bytes=20
truncated=$(truncate_string "$input_string" "$max_bytes")
echo "Truncated string: $truncated"
Firstly the script declares a function namedtruncate_string
. In the function, the for loop iterates over each character of the input string and calculates the number of bytes of each character usingLC_ALL=C wc -c
. The function appends the characters until themax_byte
is reached. At last, the script calls the truncate_string function with input argument input_string
and max_length
.
The output shows that the script has successfully truncated the input string up to emoji which is not 20 characters rather than 20 byte.
2. Using “head” Command
The head command can show the first 10 lines of the input by default. When you use the -c option with the command followed by a number it outputs the specific byte or number of characters from the input string.
To truncate the input string with the head
command, take a look at the following script below:
#!/bin/bash
# Define the maximum byte length you want to truncate the string to
max_bytes=20
# Example string to truncate
string="This is a long string that needs to be truncated"
# Truncate the string to the specified maximum byte length
truncated_string=$(echo -n "$string" | head -c "$max_bytes")
# Output the truncated string
echo "$truncated_string"
Firstly, the script declares the input string in a variable named string
. Then, the echo command with the-n
option prints the input without the trailing newline characters. After that, the pipe(|) operator redirects the output of theecho
command to the head command. With head-c "$max_bytes"
, the script outputs only the first max_bytes bytes and here the max_bytes is set at 20
. At last, the echo
command prints the output to the terminal.
The output shows the script has truncated the input string to 20 bytes in size.
echo "$truncated_string" > truncated_string.txt
This command will create a new file named truncated_string.txt and store the modified string in it.
Truncate Text Files to a Specific Number of Lines
To truncate text lines to specific lines you can use thehead
command which will show the first specified line of the input file. Take a look at the following script:
#!/bin/bash
# Function to truncate lines to N lines
truncate_lines() {
local file="$1"
local max_lines="$2"
# Use head to extract the first N lines
head -n "$max_lines" "$file"
}
# Usage example
file="colors.txt" # Change this to your input file
max_lines=3 # Change this to the maximum number of lines you want
truncate_lines "$file" "$max_lines"
At first, the cat command shows all the contents of the input file. In the truncate function, the script takes two input arguments and then utilizes the head
command to extract the max_lines
from the input file. The head command will print the first 3
lines from the input file. At last, the script calls the function with two arguments file and max_lines.
The image shows that the script truncated the content into 3 lines of the input file.
3. Using “tail” Command
The tail command usually displays the last part of the file or the end of the input string. Like the head command, if you use the -c
option with the tail command followed by a number it outputs the specific byte or number of characters from the end of the input string. To truncate a string from the beginning of the input string, check the following script:
#!/bin/bash
# Define the maximum byte length you want to truncate the string to
max_bytes=20
# Example string to truncate
string="The input string needs to be truncated from the beginning."
# Use tail command to truncate the string to the specified maximum byte length
truncated_string=$(echo -n "$string" | tail -c "$max_bytes")
# Output the truncated string
echo "$truncated_string"
The pipe operator redirects the output of theecho
command to the tail
command. With tail -c "$max_bytes"
, the script outputs only the last max_bytes bytes and here the max_bytes is set at 20
.
The output shows that the tail command outputs the last 20 bytes of characters of the input string.
4. Using “sed” Command
The sed command is a simple yet handy command to remove characters, replace strings, match patterns, and manipulate files and texts. Using thesed
command, you can easily truncate a string to a specific length of characters or bytes. Take a look at the following script:
#!/bin/bash
# Define the maximum length you want to truncate the string to
max_length=10
# Example string to truncate
string="This is a long string that needs to be truncated"
# Use sed to truncate the string to the specified maximum length
truncated_string=$(echo "$string" | sed "s/^\(.\{$max_length\}\).*$/\1/")
# Output the truncated string
echo "$truncated_string"
With the$(echo "$string" | sed "s/^\(.\{$max_length\}\).*$/\1/")
, the pipe operator takes the output of the echo command to thesed
command. In the sed command,the ^
matches the pattern of the beginning of the line. Then the\{$max_length\}\
selects max_length from the beginning of the string. After that,.*$
matches the rest of the string after the selected substring. At last, with\1
the script replaces the entire string with the output.
The image shows that the sed command has truncated the input to a specific length of 10 from the beginning.
5. Using “cut” Command
To truncate the input string to a specific length or byte of characters, you can use the cut command. The cut command simply extracts the substring and removes the specific portion of the input string. Take a look at the script below:
#!/bin/bash
# Define the maximum length you want to truncate the string to
max_length=20
# Example string to truncate
string="This is another string that also needs to be truncated"
# Use cut command to truncate the string to the specified maximum length
truncated_string=$(echo "$string" | cut -c1-"$max_length")
# Output the truncated string
echo "$truncated_string"
At first, theecho
command redirects the string to thecut
command using the pipe operator. Then the cut command extracts a specific length of string using the following options and the script sets the max_length of the substring to 20. Here, the-c
option specifies the characters, and the1-
indicates the extraction starts at the first character of the input string and goes up to the max_length without including the character at the max_length position.
The output shows that the script has truncated the input string to a length of 20.
How to Truncate String to Specific Length and Number of Lines in Bash?
While condensing a text file, let’s say you need to truncate the length of the line and also the number of lines. If you specify the length of the line and number then you can efficiently truncate the text file according to your preferences.
To truncate a text file to a specific line length and number of lines, check the following script:
#!/bin/bash
truncate_text() {
local max_chars_per_line="$1"
local max_lines="$2"
local input_file="$3"
# Read the content of input file
local input_text=$(< "$input_file")
# Split input text into lines
IFS=$'\n' read -r -d '' -a lines <<< "$input_text"
# Truncate each line to max_chars_per_line and store in truncated_lines array
local truncated_lines=()
for line in "${lines[@]}"; do
truncated_lines+=("${line:0:max_chars_per_line}")
done
# Join the first max_lines lines with newline separator
local result=$(printf "%s\n" "${truncated_lines[@]:0:max_lines}")
echo "$result"
}
# Example usage
max_chars_per_line=15
max_lines=7
input_text="song.txt"
truncated_text=$(truncate_text "$max_chars_per_line" "$max_lines" "$input_text")
echo "$truncated_text"
At first, the script declares a function named truncate which takes three input arguments. In the function, with local input_text=$(< "$input_file")
, the script reads the content of the input file and stores it in the input_text
. After that, with IFS=$'\n' read -r -d '' -a lines <<< "$input_text"
, it splits the input into an array of lines and stores the line array. Then it declares an empty variable in which the script will store the truncated value of the string. Using the parameter expansion, the function extracts a specific length of string from each line. The printf "%s\n" "${truncated_lines[@]:0:max_lines}")
joins each line to the existing one with a newline separator. At last, the script calls the function with the arguments max_chars_per_line
, max_lines
and input_text
.
To display the content of the input file, use the cat command following with the filename just like below:
cat song.txt
From the image you can see, that the text file has around 12 lines including empty lines.
The script has truncated the first 7 lines without empty lines and each line contains 15 characters.
Error Handling in String Truncation
Sometimes while truncating a string to a specific length, the length you choose may exceed the original string size. In this case, without showing any error the script returns the original string. If you want to know whether the string length exceeds or not by a message and then truncate it, check the following script:
#!/bin/bash
str="This is a string"
desired_length=20
if [[ ${#str} -gt $desired_length ]]; then
truncated_string=${str:0:desired_length}
echo "String truncated to the desired length: $truncated_string"
else
truncated_string=$str
echo "Desired length is greater than original length: No truncation needed"
fi
echo "Truncated string: $truncated_string"
With if [[ ${#str} -gt $desired_length ]]
, the script checks whether the string length is greater than the desired length. If the condition is true, the script executes the if block and returns the truncated string. Otherwise, it executes the else block which displays the input string and a feedback message.
The output shows a message that provides feedback as the input string is shorter than the desired length.
Conclusion
In conclusion, this article illustrates 5 methods to truncate strings in Bash, truncate strings from a text file, and error handling of string truncation. It reflects mainly two approaches one is pure bash scripting and another is using external commands. The external commands like head, sed, cut, and tail can not handle multi-byte characters. So if you want to handle the single and multi-byte characters you have to look at the customize function that handles both characters as shown in the parameter expansion section.
People Also Ask
What is the truncation of a string in Bash?
In bash scripting, string truncation means trimming the length or size of an input string or an input file to make it fit within a certain size limit. It is essential while displaying information and saving memory.
How do I truncate a string in Bash?
To truncate a string to a specified length, you can use the ${string:0:length}
syntax. Additionally, you can also use external commands like head
, sed
, and cut
commands to do the task. This syntax will truncate the input string from the beginning till the specified length.
Can I truncate text without using external commands in Bash?
Yes, you can truncate a string using the parameter expansion which is pure bash scripting. By using the ${string:0:max_chars}
syntax, you can easily truncate a substring that starts from index 0
up to max_chars
.
Can I truncate strings containing multi-byte characters in Bash?
Yes, you can truncate strings containing multi-byte characters by converting all the characters into bytes. Firstly you need to create a custom function that iterates over each character of the input string. It calculates the byte length of each character and truncates the string.
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial