FUNDAMENTALS A Complete Guide for Beginners
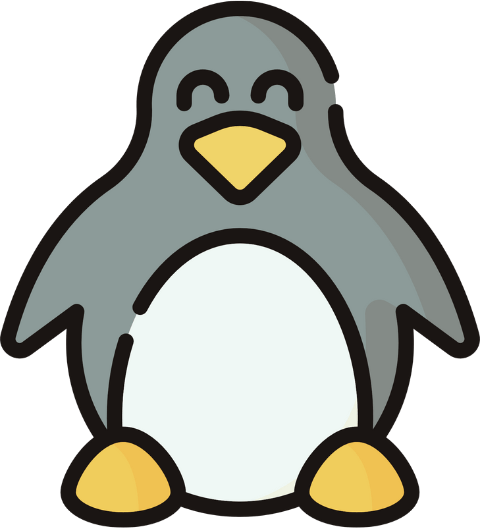
To encode and decode text files using base64 in Bash, use the following syntaxes:
- Encode text incorporating pipe command:
echo -n 'Sample string' | base64
- Encode with text file:
base64 file1.txt
- Encode and save encoded text to a file:
base64 file2.txt > encoded_file.txt
- Encode with line wrapping:
echo 'Long string.' | base64 -w 0
- Decode text file:
base64 -d encodedfile.txt
- Encode text ignoring non-alphabet character:
echo 'String with non-alphabet characters' | base64 -i
Base64 encoding and decoding secure data transfer by translating binary data to ASCII code. This article will demonstrate practical examples of encoding and decoding text by implementing each of these syntaxes. Additionally, it will cover 3 different modules of “base64” for encoding and decoding data using OpenSSL, Perl, and Python. So, let’s get started!
What is “base64”?
The base64 is an encoding and decoding system that represents various binary data file content types, including standard input and output in ASCII string format. It encodes and decodes data to simplify the data transmission and storage process. Programmers can quickly reveal the encoded data through the decoding process by using this command.
Syntax of base64:
base64 [OPTION] [FILE]
Here, OPTION is task-dependent flag and FILE is the file name that is intended to be encoded.
Now, here is a list of some command line arguments or OPTIONs that can modify the output of the base64 command:
- -d, – -decode: Decodes the data.
- -i, – -ignore-garbage: Ignores non-alphabet characters when decodes.
- -w, – -wrap=COLS: Wraps the encoded text after 76 characters, but usages of 0 disables line wrapping.
- – -help: Displays the help section and exit.
- – -version: Displays version information and exit.
Why and When to Use Base64?
Base64 is primarily used for transmitting binary data over text-based protocols such as email, HTTP, or XML. Here’s why and when you might want to use Base64 encoding and decoding:
- E-mail: Base64 can convert binary data (such as an image or file attachment) into ASCII characters for secure transmission in email systems.
- URLs: Use Base64 in URL encoding to convert binary data.
- Data Storage: Use Base64 to encode sensitive binary data into a text format before storing it in a database.
- Web development: Use base64 image encodings in CSS and inline images in HTML.
Base64 is only an encoding tool. It is not an encryption method. The encoded data can be securely stored in a database.
Encoding with “base64” in Bash
In Bash, the base64 command can encode the string data. Use one of the syntaxes from below to encode a string:
echo -n 'Sample string' | base64
The output of the echo command is piped to the base64 command. Here -n option prevents “echo” from adding a trailing newline character to the string.
base64 <<< “Hello, World!”
Here, the “Hello, World” string is passed to the base64 command using here-string.
Decoding with “base64” in Bash
To decode an encoded string or a file containing an encoded string, use one of the below syntaxes:
base64 -d encodedfile.txt
OR,
base64 -d <<< “encoded string”
Here, the -d
option enables the base64
command to decode the given string.
6 Examples to Decode & Encode Using “base64” in Bash
Here, I have demonstrated some examples of encoding and decoding using the base64 command. These are encoding & decoding text files, checking the validity of users, encoding and decoding large files, encoding with line wrapping, and ignoring non-alphabetical characters.
1. Encode Text File Using “base64” in Bash
To encode a text file using Base64 in Bash, you can simply use the base64 command followed by the name of the file. For example:
base64 file1.txt
Here, the base64
command encodes the content of the file1.txt
file and prints it on the terminal.
2. Decode Text File Using “base64” in Bash
Decoding means returning to the original string from the coded text. To decode a text file containing coded strings, put the file name after the base64 command. Here is a complete command to decode a text file:
base64 -d encodedfile.txt
Here, the base64
command decodes the content of the encodedfile.txt
and prints it on the terminal.
3. Check User Validity by Decoding Text
To check user validity, you can ask for a secret code from the user. Then, compare the secret code with the original secret code to validate. Here is a complete bash script to check user validity:
#!/bin/bash
echo "Enter your secret code"
read secret
original=$(echo “Nzc3Nzk5Cg==” | base64 --decode)
if [ $secret == $original ]; then
echo "You are authenticated"
else
echo "You are not authenticated"
fi
The read secret
command takes input from the user and keeps it on the ‘secret’ variable. After that, original=$(echo 'Nzc3Nzk5Cg==' | base64 --decode)
decodes the base64 encoded string and stores it to the ‘original’ variable. Afterward, $secret == $original
checks the equality of the ‘secret’ with ‘original’ variable value inside the if condition and prints a message accordingly.
4. Encode and Decode Large Files
The base64 command can efficiently encode and decode the large files. To encode the content of a file and keep the encoded text on another file, use the syntax: base64 file_name.txt > encoded_file.txt
. For decoding the encoded text inside a file and keeping the decoded text inside a file, use the syntax: base64 -d encoded_file.txt > decoded_file.txt
. Here is a complete bash script:
#!/bin/bash
#ecode the file
base64 file2.txt > encoded_file.txt
echo “encoded text”
cat encoded_file.txt
#decode the file
base64 -d encoded_file.txt > decoded_file.txt
echo “coded text”
cat decoded_file.txt
The script’s part, base64 file2.txt > encoded_file.txt
, encodes the content of the file2.txt file using Base64 encoding and saves the encoded output to the ‘encoded_file.txt’ file. After that, the cat command prints the content of the ‘encoded_file.txt’ file on the terminal. Afterwards, base64 -d encoded_file.txt > decoded_file.txt
decodes the encoded contents inside the ‘encoded_file.txt’ file.
5. Encode with Line Wrapping
In general, the base64 command wraps the encoded text after 76 characters. For encoding text containing more than 76 characters, use the -w
flag with 0 argument. It will encode a long single string without any line break. Follow the complete bash script given below:
#!/bin/bash
echo 'Linux is an open-source operating system renowned for its versatility, stability, and security.' | base64 -w 0
The echo command pipes a string with more than 76 characters to the base64 -w 0
command that encodes the whole string using Base64 encoding. Here, the -w 0
option specifies to encode the large string without line wrapping.
6. Ignore Non-Alphabet Characters
The base64 command can ignore unexpected and unwanted non-alphabetical characters using the -i
flag. It instructs the encoder to continue encoding even if it encounters invalid characters during the encoding process. Here is a complete bash script to ignore non-alphabet characters:
#!/bin/bash
echo “Encoded text:”
echo 'Here are some #@$% non alphabet characters' | base64 -i
The echo command pipes a string containing non-alphabet characters to the base64 -i
command. The base64 command ignores the non-alphabet characters for the -i option and encodes the string.
3 Different Modules of “base64” for Encoding and Decoding
There are some other modules where you can use bash64 to encode and decode data, such as openssl, python, and perl. Here I have explored them below with practical examples.
1. Using “openssl” for Encoding and Decoding
OpenSSL is a cryptographic software library or a toolkit that improves the security of communication over computer networks. To encode a string or text using openssl follow the syntax: echo 'Sample string' | openssl base64
. To decode a coded string or text, follow the syntax: echo 'coded string' | openssl base64 -d
. Here is the complete bash script to encode and decode a string using openssl:
#!/bin/bash
echo “Encoded Text:”
echo 'This is a string' | openssl base64
echo “Decoded Text:”
echo 'VGhpcyBpcyBhIHN0cmluZwo=' | openssl base64 -d
At first, echo command pipes a string to the openssl base64
command that encodes the string. Afterward, the echo command pipes the encoded text to the openssl base64 -d
command. Here, the -d
option specifies the instructions for decoding the piped text.
2. Using “perl” for Encoding and Decoding
MMIME::Base64 is the core module of Perl that encodes or decodes data in the Base64 format. Here is a complete bash script to encode a text using the perl command:
#!/bin/bash
echo “Encoded text:”
perl -MMIME::Base64 -ne 'printf "%s",encode_base64($_)' <<< "This is a string."
The perl command encodes the string using the Base64 encoding. Here -MMIME::Base64
option loads the “MMIME::Base64’ perl module that provides function for Base64 encoding. After that, the -ne
option enables to read input line by line and execute the encoding process. Finally, 'printf "%s",encode_base64($_)'
formats and prints the output using the printf function. And encode_base64
function from the ‘MMIME::Base64’ module encode the input string ‘$-’.
3. Using “python” for Encoding and Decoding
Python also provides the base64 module for encoding and decoding. Here is a simple Python code to encode and decode text:
import base64
# Encoding
encoded_text = base64.b64encode(b'This is a string')
print(encoded_text)
# Decoding
decoded_text = base64.b64decode(encoded_text)
print(decoded_text.decode('utf-8'))
The part of Python code, import base64
, imports the base64 module. After that, base64.b64encode()
function encodes the “This is a string” string. Then the base64.b64decode()
function decodes the encoded text for the previous string.
Warning: One common issue while working with the base64 command is an “Invalid Input” error. This error occurs when you try to decode coded text that is not properly encoded. To overcome this issue, make sure your text is correctly encoded.
Conclusion
In this article, I have explored various perspectives of encoding and decoding text with the base64 command in Bash. I hope this will help young learners grasp encoding and decoding using base64 in Bash. Don’t forget to comment with your valuable thoughts if you have suggestions for improving this.
People Also Ask
How Do I Use the Base64 Command in Linux?
To encode text/string in bash, use the syntax: base64 filename
, and to decode the coded text, use the syntax: base64 -d encoded_file
What does Base64 do in Bash?
The base64 encodes binary data. It allows programmers to transport binary over protocols or mediums that can not handle binary data formats and require simple text.
What is the Base64 file type?
Base64 is an ASCII string format. It is a group of binary-to-text encoding schemes that represent binary data in ASCII code.
How to convert password to Base64?
To convert a password to base64, type the password you want to encode. Then, you need to pipe the password to the base64 command. For this, follow the syntax: echo “password string” | base64
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial