FUNDAMENTALS A Complete Guide for Beginners
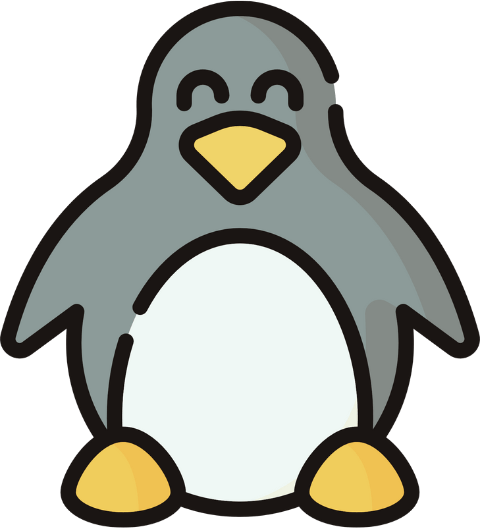
A string is a collection of characters including numbers and special characters. In Bash scripting, string manipulation is one of the important tasks to accommodate necessary updates to information and data. To remove the last character from a string in Bash, follow the below method:
- Using the cut command:
echo "$original_string" | cut -c -n
(n=total number of characters excluding the last character) - Using the sed command:
echo "$original_string" | sed 's/.\{1\}$//'
- Using parameter expansion:
echo ${original_string::-1}
- Using the awk command:
echo "$original_string" | awk '{ print substr( $0, 1, length($0)-1 ) }'
- Using the ? symbol:
echo ${original_string%?}
- Utilizing parameter expansion with string length:
echo ${original_string:0: string_length -1}
1. Using the “cut” Command
The cut command extracts a range of a substring from a big string. With the echo command, it can remove characters from a string. Here is how:
#!/bin/bash
string="Welcome to Linuxsimply"
echo "$string" | cut -c -21
The output of the echo command goes as input to the cut command. Here, cut -c -21
specifies extracting the first 21 characters from the original string and printing it on the terminal.
2. Using the “sed” Command
The sed command can edit a string by removing or adding characters to that string. Piping the output of the echo command to the sed command, the last character can easily be removed. Here, I will show a bash script that will remove the last character from a string:
#!/bin/bash
string="Welcome to Linuxsimply"
echo "$string" | sed 's/.\{1\}$//'
The output of the echo command goes as input to sed 's/.\{1\}$/’
. Here, sed 's/.\{1\}$//'
is a command substitution for ‘sed’. The ‘s’ indicates that sed should perform a substitution. And .\{1\}$
matches the last character of the string. Finally, //
replaces the last character with nothing.
3. Using Parameter Expansion
The parameter expansion is a method that can modify a string. The ${string_name::-1} removes the last character. Here is a Bash script on this:
#!/bin/bash
string="Welcome to Linuxsimply"
echo ${string::-1}
The echo ${string::-1}
is a parameter expansion that specifies a substring up to the second last character.
4. Using “awk” Command
The awk command is a string processing tool that looks for a specific pattern inside a string and manipulates it. Use the substr( $0, start_character_position, original_string_length($0)-1 ) }'
function inside the awk command with the print command to extract a substring excluding the last character from the original_string($0). Here, is a complete bash script to do this:
#!/bin/bash
string="Welcome to Linuxsimply"
echo "$string" | awk '{ print substr( $0, 1, length($0)-1 ) }'
The output of the echo command goes as input to the awk command. Here, substr( $0, 1, length($0)-1 )
extracts a substring starting from the first character and the length of the substring is of the original string length minus one.
5. Using “?” to Remove the Last Characters of a String
The “?” symbol removes the shortest matches from a string. ${original_string%?}
fetches a substring from the original string excluding the last character from the string. Here is the Bash script how:
#!/bin/bash
string="Welcome to Linuxsimply"
echo ${string%?}
Here, ${string%?}
removes the shortest possible match of the ‘?’ pattern from the ending of the string which is a single character.
6. Using Bash Parameter to Remove the Last Character from a String
The last characters of a string can be removed by using the string length inside the echo command with a curly bracket. The syntax to remove the last character from a string is: echo ${original_string:start_index: length -(number of characters to remove from the end)}
. However, it is a parameter expansion approach. Here is a complete Bash script for doing so:
#!/bin/bash
string="Welcome to Linuxsimply"
length=${#string}
echo ${string:0: length -1}
length=${#string}
calculates the length of the string. After that, the ${string:0: length -1}
represents a substring that starts from the first character of the original string and the length will be the original string length minus 1.
Practice Problem
- Write a bash script to remove the first and last characters of a string.
- Write a bash script to remove the last 5 characters of a string.
Conclusion
This article explores different approaches to removing the last character from a string including cut command, sed command, awk command, and parameter expansion. This feature will equip a bash programmer to manipulate a string whenever necessary for efficient, accurate, and updated data management.
People Also Ask
How do I remove the last character of a string in Bash?
To remove the last character of a string in Bash, execute: echo ${original_string%?}
command. It will remove the last character of a string in Bash and print the remaining part of the string in the terminal.
How do I remove the last space in Bash?
To remove the last space in a string in bash, execute nospace_string=${original_string% }
. If the original string is ”Hello, how are you? ” then the nospace_string will be ”Hello, how are you? ”
How do you check if a string is empty in Bash?
To check if a string is empty in Bash, use the ‘-z’ option inside an if statement. If the string is empty, the output of the ‘-z’ option will be zero.
How to find substring from string in Bash?
To find a substring from a string in Bash, use the syntax: substring=${string:start_position:substring_length}
This will extract a substring from the original string starting from the start_position of the original string, and the substring_length is the number of characters you want to extract.
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial