FUNDAMENTALS A Complete Guide for Beginners
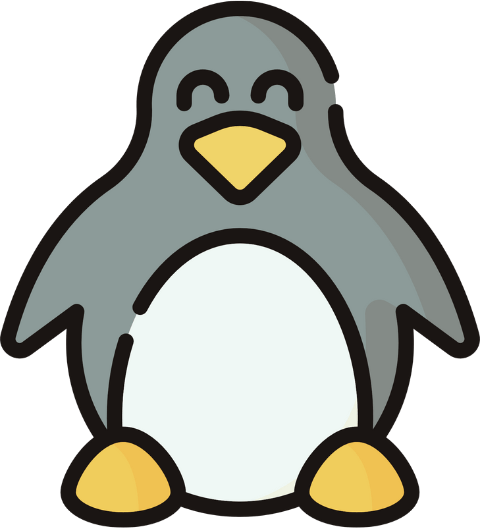
A bash string is a sequence of characters that includes letters, symbols, numbers and spaces, and special characters. To manipulate and store the textual data, knowing about bash string and how to handle it is important. Let’s take a look at how to create, manipulate, and text a bash string in this article.
What is Bash String in Linux?
Bash string in Linux is a sequence of characters that is enclosed with single or double quotes and even with no quotes. Using a string with space will show an error without any quotes. Single quotes preserve the literal values of each character. Like@
and*
are treated as literal values in single quotes. But double quotes allow variable expansion and command substitution,@
and *
have a special meaning in double quotes which are parameter expansion at certain constructs and as a wildcard.
So, the bash string is not only a distinct data type like integers and booleans but is also represented using the general concept of variables. Generally, text is represented with a bash string.
How to Create a Bash String?
Creating a bash string refers to assigning a character or a sequence of characters into a variable. This variable can be read, searched, and manipulated as textual data. Using a single quote(''
), and double quotes (""
) one can easily create a bash string. By combining two strings, a new string can also be created. You can create the multiline string using a new line character and here document.
Single-line String
Using single quotes and double quotes you can create a single line string. Here is how it looks:
#!/bin/bash
string1='Hello World!'
string2='It’s a new string.'
string3= "This is another string"
echo $string1
echo $string2
echo $string3
Three different strings are assigned into three different variables string1,string2, and string3. The$
is used to make the variable accessible. The echo command is used to print the strings in the terminal.
In the output, a single-line string has been printed using single and double quotes.
Multiline String
Using here doc and new line character, you can create a multiline string. Here is how it looks:
#!/bin/bash
echo "Using new line character"
multiline="Hello World!\nIt’s a new string.\nThis is another string."
echo -e "$multiline"
echo "Using here document"
Multilinestring=$(cat << EOF
Hello World!
It’s a new string.
This is another string.
EOF
)
echo "$Multilinestring"
The \n
is the newline character which is used to separate lines. To interpret the backslash escape the-e
option is used with theecho
command.
Note: Here with the “echo” command in the command line, you can read the string. If you want to print the string into a file then use the “>” operator followed by the filename in which you want to print the string.
Basic String Operation
The basic string operation refers to the process and actions that can be performed on the string. The operation of a string is important for text processing, data manipulation, configuration, and automation. Let’s see some basic string operations:
Concatenation
To concatenate two strings, you can list them in order within another variable, as shown below:
#!/bin/bash
echo "Using Simple Concatenation"
string1="Hello"
string2="World"
concatenated_string="$string1 $string2"
echo "Concatenated String:$concatenated_string"
echo "Using Listing"
string1="The first string."
string2="This is new string."
concatenated_string=${string1}${string2}
echo "Concatenated String:$concatenated_string"
The"$string1 $string2"
is concatenated using a space. And the ${string1}${string2}
concatenates two strings without any special character or space; it is only concatenated using the string side by side.
String Length
Generally, the#
symbol is used for commenting, but it is also used as a variable substitution and special parameter in bash. Using this parameter with a variable and curly brackets you can get the length of the given variable’s value.
To know the length of a string you can use the following code:
#!/bin/bash
String="Hello, World!"
length=${#String}
echo "The length of the string is: $length"
The${#string}
will calculate the length of the string and store it in the length variable.
Here the string length is 13.
Extraction of Substring
Extraction of substring refers to the process of finding a sequence of characters from a large bash string. To isolate and manipulate specific portions of text data and string manipulation, extraction of substring is an important task. Let’s see 3 methods of extracting substring:
Method 1: Using Parameter Expansion
Parameter expansion is a way to perform various operations on the values of variables. To extract a value from a substring, ${variable:offset:length}
form of expansion is used.
To extract a specific substring use the following code:
#!/bin/bash
original_string="The extracted substring."
substring=${original_string:4:9}
echo "Original String: $original_string"
echo "Substring: $substring"
The${original_string:4:9}
extracted a substring from the original string using the parameter expansion. the substring length is 9 and it starts after the $4th character of the original string or from the 5th character of the original string.
From the output, you can see the extracted substring from the input.
Method 2: Using “cut” Command
The cut command is a command line utility that is used to cut a specific section from a line of the input. It can be also used for substring extraction. The basic syntax of thecut
command is cut [option] [file]
.
To extract the substring use thecut -c
command. Here is how:
#!/bin/bash
original_string="Today is $(date)"
substring=$(echo "$original_string" | cut -c 9-40)
echo "Original String: $original_string"
echo "Substring: $substring"
Theecho
command prints the values of the original string inecho "$original_string"
which will be the standard input for the cut command. The cut command extracts the substring of the output from the pipe and cuts each line from characters from 9 to 40 from the string.
From the output, you can see the date in the string has been extracted.
Note: In the input, a single line has been used. For that reason, it cuts only from that line. Otherwise, it cuts from each line from the character 9 to 40. If you want to cut a specific substring it is better not to use thecut
command.
Method 3: Using “awk” Command
The awk command is a versatile command line utility that can be used for column extraction, pattern matching, arithmetic, string operation, and formatting the output.
To extract a specific substring, use the awk command following the code:
#!/bin/bash
original_string="Hello, World!"
substring=$(echo "$original_string" | awk '{print substr($0, 8, 5)}')
echo "Original String: $original_string"
echo "Substring: $substring"
The awk command takes the output from the echo command through pipe (|). Thesubstr()
function takes three arguments: the string, the start position, and the length of the substring. It extracts a substring from the 8th character of the original string and the substring’s length will be 5. The$0
indicates the entire line of the input.
The extracted word from the string in World.
Split Bash String
Splitting bash string is the process of dividing a string into smaller pieces of substring based on a delimiter. The delimiter is a character or sequence of characters on which it is decided where the string will be divided. Splitting is important for data extraction from a large string, text processing, and the creation of an array. Let’s see 3 methods to split a bash string:
1. Split Bash String Using IFS
The IFS is a variable that stands for Internal Field Separator which is used to determine how to split fields or words in a string.
To split a bash string using IFS, follow the code shown below:
#!/bin/bash
myString="Is the string splitted?"
oldIFS=$IFS
IFS=' '
# Create an array by reading the string into variables
read -a myArray <<< "$myString"
# Restore the original IFS value
IFS=$oldIFS
# Print the elements of the array
for element in "${myArray[@]}"; do
echo "$element"
done
The IFS is set to the space. The read command will words from the string into the array using the-a
option. The<<<
is a here-string that provides the string to the read command. The for loop iterates over the line and prints the word using the space.
From the output, you can see the input has been split based on space.
2. Split Bash String Without IFS
Based on the delimiter, one can split a string without using IFS. Here is how the code looks:
#!/bin/bash
#Read the main string
echo "Enter the string with colon(:) to split"
read mainstr
#Split the string based on the delimiter, ':'
readarray -d : -t arr <<< "$mainstr"
# Print each value of the array by using the loop
for (( i=0; i < ${#arr[*]}; i++))
do
echo "${arr[i]}"
done
Theread
command will read the main string from the command line. The readarray command with -d will split the main string based on the colon(:). The -t option removes the trailing newline character. Thefor
loop iterates over the array and prints each word split by the colon.
The input string has been split based on the : symbol.
3. Split Bash String Using Another String
To split a string into substring you can use another string as a delimiter. Here is a script to do so:
#!/bin/bash
text="ThisThe FirstThisword This."
delimiter="This"
string=$text$delimiter
myarray=()
while [[ $string ]]; do
myarray+=( "${string%%"$delimiter"*}" )
string=${string#*"$delimiter"}
done
#Print the words after the split
for value in ${myarray[@]}
do
echo -n "$value "
done
printf "\n"
The $text$delimiter
concatenates the text and delimiter. The"${string%%"$delimiter"*}"
remove from the end the longest matched pattern with the delimiter. And the ${string#*"$delimiter"}
removes the shortest-matched pattern from the beginning. Thefor
loop iterates over the elements and prints the output. The -n option is used to prevent the new line.
In the output, the input string is split using this string as a delimiter.
String Manipulation
String manipulation refers to the process of modifying the sequence of characters. In bash scripting, string manipulation mainly includes substring extraction, searching for a specific pattern, replacing a pattern, and removing a substring. Here, some of the string manipulation process has been shown below:
A. Case Conversion
Case conversion refers to converting the case of the character in the string from upper case to lower case and lower case to upper case, and reversing a string.
To make the conversion from lower case to upper case of bash string, use the following code:
#!/bin/bash
original_string="Hello world"
uppercase_string="${original_string^^}"
echo "Original String: $original_string"
echo "Uppercase String: $uppercase_string"
The${original_string^^}
is used for parameter expansion. Inside the bracket{}
the^^
operator is used to convert the characters into uppercase.
From the output, you can see I have renamed the script to uppercase.sh you can rename according to your preference.
Note: For older versions of bash, the^^
operator is not supported. So in that case you can use the tr command for the conversion from the lower case to the upper and upper case to the lower case.
To convert the characters of the string from upper case to lower case copy the following code:
#!/bin/bash
original_string="Hello World"
lowercase_string="${original_string,,}"
echo "Original String: $original_string"
echo "Lowercase String: $lowercase_string"
Using the parameter expansion in${original_string,,}
, the,,
operator is used to convert the characters of the string into lowercase and store the converted value in lowercase_string.
To convert the first character of each word from lower case to upper case you can copy the following code:
#!/bin/bash
# Check if the number of arguments is correct
if [ "$#" -ne 1 ]; then
echo "Usage: $0 <string>"
exit 1
fi
inputString="$1"
capitalizedString=$(echo "$inputString" | sed 's/\b\(.\)/\u\1/g')
echo "Original String: $inputString"
echo "Capitalized String: $capitalizedString"
The[ "$#" -ne 1 ]
checks the number of correct arguments. The pipe (|) takes the standard output of the commandecho “$inputstring”
and the standard input for the right of the pipe. The sed command performs a substitution operation on the string. The's
indicates substitution. The\b
indicates the word boundary. The\(.\)
indicates the first character of each word and\u\1
changes the first character of each word into uppercase. The g is the global replacement which changes all the occurences.
From the output, you can see the first character of each word is converted into uppercase.
B. Search Pattern
To search for a specific pattern of string from a large string use the following code:
#!/bin/bash
# Check if the script is called with an argument
if [ $# -eq 0 ]; then
echo "Usage: $0 <string>"
exit 1
fi
# Retrieve the string from the command-line argument
string=$1
if [[ $string == a* ]]; then
echo "String starts with 'a'"
else
echo "String does not start with 'a'"
fi
if [[ $string == *123 ]]; then
echo "String ends with '123'"
else
echo " String does not end with '123'"
fi
if [[ $string == *ll* ]]; then
echo "String contains 'll'"
else
echo "String does not contain 'll'"
fi
The[ $# -eq 0 ]
checks the script is called with one command line argument. It takes a string as the first command line which is denoted by $1. The$string == a*
checks if the string starts with a. The$string == *123
is used to check if the string ends with 123. The$string == *ll*
checks if the string contains double l.
In the output, a specific character or pattern has been checked.
C. Replace String
To replace a specific character in a string with your desired character you can use the sed command. Here’s how:
#!/bin/bash
Main_string="Hello World"
New_string=$(echo "$Main_string" | sed -e 's/H/h/g' -e 's/W/w/g')
echo "Main_string:" $Main_string
echo "Modified_string:" $New_string
The's/H/h/g'
is used to replace the H with h and the's/W/w/g'
is used to replace the W with the w. The-e
option is used with the sed command to execute multiple expressions.
To replace a substring with another in a large string, follow the code:
#!/bin/bash
# Check if the number of arguments is correct
if [ "$#" -ne 3 ]; then
echo "Usage: $0 <original_string> <substring_to_replace> <replacement_string>"
exit 1
fi
original_string="$1"
substring_to_replace="$2"
replacement_string="$3"
new_string=$(echo "$original_string" | sed "s/$substring_to_replace/$replacment_string/g")
echo "The original_string:$original_string"
echo "The substring to replace:$substring_to_replace"
echo "The replaced_string:$replacement_string"
echo "The new string:$new_string"
Three command line arguments have been used as$1
,$2
, and$3
. The sed command replaces the substring with another in the original string.
From the output, you can see the new substring has been replaced by another substring replaced in the original string.
D. Substring Removal
To remove a substring from a string you can also use the sed command. Use the following code:
#!/bin/bash
original_string="Today is $(date)"
substring_to_remove="Today is "
# Remove substring
new_string=$(echo "$original_string" | sed "s/$substring_to_remove//g")
echo "Original string: $original_string"
echo "Substring to remove: $substring_to_remove"
echo "Updated string: $new_string"
The sed command removes a specific substring from the original string. It performs on the input globally(g).
In the output, you can notice a specific substring has been removed using the script sedremove.sh from the original string.
Note: If the variable contains special characters then it may change the behavior of the sed command.
Check Bash String
Checking a bash string refers to the process of examining the string which includes the existence, equality, and search for a specific pattern. Checking is important for input data processing, conditional operation, and data validation. In the following section, I’ll depict some of the checking processes of a string.
Check Whether a String is Empty or Not
To check whether a string is empty or not follow the code which has been shown below:
#!/bin/bash
string=""
if [ -z "$string" ]; then
echo "String is empty."
else
echo "String is not empty."
fi
The-z
option is used to check if a string is empty or not. If it is empty then it returns the true value and if the string is not empty it will return false.
To check a nonempty string you can use the following code:
#!/bin/bash
my_string="Is the string empty?"
if [ -n "$my_string" ]; then
echo "String is not empty."
else
echo "String is empty."
fi
The-n
option checks a nonempty string. If it is true then it returns true values and prints it is not empty and if it is false it will print the other output.
From the output, you can see with the script one can test the string whether it is empty or not. In this case, the string is empty.
Pattern Matching
To check if a specific pattern is available or not in a string, you can copy the following code:
#!/bin/bash
my_string="Matching string with @"
substring="@"
if [[ $my_string == *"$substring"* ]]; then
echo "String contains the substring."
else
echo "String does not contain the substring."
fi
In the if statement it is checked if the specific substring is present in the main string. The wildcard *
is used before and after the substring which means it checks in the main string whether it can be anywhere.
From the output, you can see the script specific.sh has been used to check whether a string contains a specific substring.
Check If Two Strings are Equal
To check whether two strings are equal or not, use the following code:
#!/bin/bash
string1="The string is"
string2="Not same."
if [ "$string1" == "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
In the if statement, the values of two string has been compared. If it is true then it shows the result of the if statement otherwise it shows the result of the else statement.
From the image, you can see the two strings are not equal.
Conclusion
In the article, the basic concept of bash string has been shown briefly. For the beginner, it is important to know what bash string is, and how to create and manipulate it. Hope it will be helpful to use and handle string efficiently.
People Also Ask
What does ${} mean bash?
The ${}
syntax is used for parameter expansion in bash. The $ in the ${} means the beginning of the parameter expansion and the {}
is used to delimit the variable name and all the operations are performed in it. It is used for access variables, string manipulation, arithmetic expansion, and array access.
How to store a string in bash?
To store a string create a variable followed by the =
operator and the string which will be enclosed with the single quote or double quote. Print the value using the echo command and $
operator.
How to show one is greater than another string?
Using the conditional statement you can compare two strings dictonarily. It only uses the string’s first character to compare its ASCII value and declare which is greater than the other. Use the following code:
#!/bin/bash
string1="apple"
string2="banana"
if [[ "$string1" > "$string2" ]]; then
echo "$string1 is greater than $string2"
else
echo "$string1 is not greater than $string2"
fi
It will show that string1 is not greater than string2 because the ASCII value of a is less than b. This is case-sensitive. If you use Apple as string1 and banana as string2 then it will also show string1 is not greater than string2 because the value of A is less than b.
How can I reverse a string?
To reverse a string use the following code:
#!/bin/bash
original_string="Hello, World!"
reversed_string=$(echo "$original_string" | rev)
echo "Original String: $original_string"
echo "Reversed String: $reversed_string"
The rev command will take the output from the echo command and reverse the string.
How to read string in shell?
To read a string in a shell one can use the echo command and read command by the following process:
echo "Enter the first word: " read firstword echo "The first word is $firstword"
read -p "Enter First Name: " firstname echo "First Name is $firstname"
read -s -p "Print name: " name echo "The name is $name"
The read command with the -p option will combine the prompt and input to read a single line in the shell. The -s option is used to suppress the echo of the user input to the terminal.
Related Articles
- Bash String Basics
- Bash String Operations
- String Manipulation in Bash
- 8 Methods to Split String in Bash [With Examples]
- How to Extract Bash Substring? [5 methods]
- Check String in Bash
- A Complete Guide to Bash Regex
- Bash Multiline String
<< Go Back to Bash Scripting Tutorial