FUNDAMENTALS A Complete Guide for Beginners
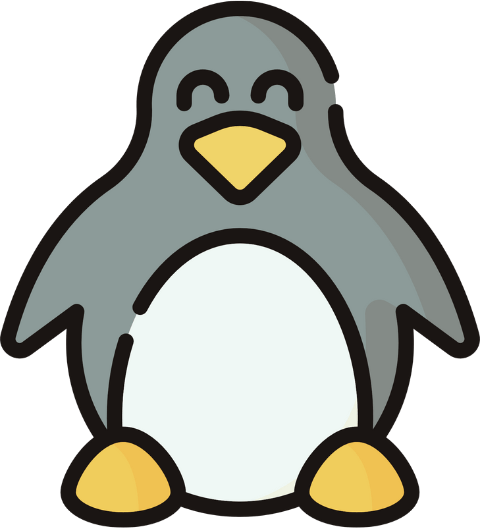
Bash variable stores essential data such as strings, numbers, and arrays of a Bash script. Bash programmer uses variables to manipulate data and do necessary arithmetic operations. In this article, I will explore various aspects of the Bash variable. Let’s start and unleash the full potential of the Bash variable.
What is Bash Variable?
A Bash variable in Linux is a symbolic name or identifier that stores a value or text string. It is a way to store and manipulate data within a Bash script or the Bash environment. Variables in Bash scripts are typically used to hold temporary or user-defined data, making it easier to reference and manipulate values as needed.
Applications of Variables in Bash Scripts
The variable can be used in the following situations:
- You can use Bash variables to store data.
- Arithmetic operations can be done using the Bash variable.
- Taking valuable input from users and preserving it.
- Bash variable can be used to create dynamic output.
- The bash variable lets you access the environment variables.
Fundamental Characteristics of Bash Variables
Bash variables maintain some unique characteristics. These are discussed below:
- Declaring a Variable: Programmers do not need to declare Variables in Bash explicitly. They can assign a value to a variable directly, and Bash will automatically create it.
- Naming Convention of a Variable: Bash variables are case-sensitive. Var and var are treated as two different variables. You can use underscore(_) between the words of a variable name.
- Value Assigning in a Variable: Variables are assigned values using the ‘=’ operator. No spaces should be present around the ‘=’ sign. To assign value in variable_name variable, you have to execute the command:
variable_name=value
- Accessing Values of Variable: To access the value stored in a variable, you can use the variable name preceded by a dollar sign(‘$’). To print the value of variable_name, you have to execute the command:
echo $variable_name
- Types of Variables: Bash does not have explicit variable types. All variables are treated as strings by default. However, you can perform numeric operations on variables containing numeric values.
- Usage of Variables: Variables can be used in various ways, such as arithmetic calculations, string manipulation, command substitution, and passing values between scripts or functions.
Types of Variables in Linux
There are two types of variables. These are system variables and user-defined variables.
- System variables are variables defined by the system. These variables have been existing since the installation of the operating system. Some of the system variables with their function are given below:
Variable | Example | Function |
---|---|---|
SHELL | /bin/bash | Defines the name of the shell used. |
PWD | /root | Shows the current Working directory. |
LOGNAME | root | Defines the message of the dayfor the system. |
MOTD_SHOWN | pam | Defines the message of the day for the system. |
HOME | root | Home directory of the user. |
LS_COLORS | rs=0:di=01;34:ln=01;36:mh=00:pi=40; | Used to set the colors the filenames will be displayed for the user. |
LESSCLOSE | /usr/bin/lesspipe %s %s | Used to invoke input postprocessor. |
USER | root | Name of the current user. |
SHLVL | 1 | Displays the number of shell levels the current shell is running on top of. |
SSH_CLIENT | 185.185.185.185 54321 12 | SSH client information [user IP] [user port] [Linux machine port]. |
PATH | /usr/local/sbin | Defines the directories to be searched for bash to find a command. |
SSH_TTY | /dev/pts/0_=/usr/bin/printenv | Displays the path to the device associated with the current shell or command. |
- User-defined variables are defined by the users to accomplish the task of the script. User-defined variables are whatever you want as variables in your script.
Some Special Variables in Linux
There are some special variables that stand for some special task. A list of special variables with their operation is given below:
Variables | Description |
---|---|
$# | Number of parameters passed to the script. |
$@ | All the command line parameters are passed to the script. |
$? | The exit status of the last process to run. |
$$ | The Process ID (PID) of the current script. |
$USER | The username of the user executing the script. |
$HOSTNAME | The hostname of the computer running the script. |
$SECONDS | The number of seconds the script has been running for. |
#RANDOM | Returns a random number. |
$LINENO | Returns the current line number of the script. |
Variable Scope in Bash
The scope of a variable in a program or script is a region where the variables have their existence. There are two types of variable scope. These are listed below:
- Local Variable: If a variable is declared inside a function then it is generally a local variable.
- Global Variable: If it is declared outside then it is a global variable.
But in the case of a bash script, whether it is written inside a function or outside a function by default is a global variable. To make a variable local, use the ‘local’ keyword.
Input and Export of Bash Variables
The simplest way to take any variable input from the user is to use the read
command. Follow the below syntax to take variable input from the user:
read -p variable_name
The simplest way to export any variable and print it on the terminal is to use the echo command. Follow the following syntax to do this:
echo $variable_name
7 Practical Examples of Working With Bash Variables
Here I have listed 7 examples related to the Bash variable. I believe you will be able to improve your basic knowledge about bash variables by going through these.
1. Declaration and Assignment of Variables in Bash Scripts
Bash variables must be defined with proper name format. Then the programmer might assign a value to that variable or keep the variable empty. Here I will show you a script where I will declare a variable by assigning a value to it. Then print the value of a variable on the terminal. To achieve so, follow the bash script:
#!/bin/bash
#setting a variable
declare a=10
#printing the variable
echo $a
The #!/bin/bash interprets that it is a Bash script. Then, the value of the variable a is assigned. Afterward, the echo
command printed the value of “a” on the terminal.
The above image shows that the Bash script has printed the value of the variable on the terminal that I have declared.
2. Performing Arithmetic Operations of Variables in Bash Scripting
You can easily use variables in the Bash script. For using variables, you must mention the variable’s name correctly. The programmer can easily manipulate the value of a variable by an arithmetic operation.
Here I will show you a script where the variable is taken from the user as input and then some arithmetic operations will be done on these variables. To know how to perform arithmetic operations of bash variables, follow the below script:
#!/bin/bash
#taking number as input from the user
read -p "Enter First Number: " a
read -p "Enter Second Number: " b
#calculation summation
var=$((a*b))
#printing the summation value
echo "Multiplication of The Two Number:$var"
At first, the Bash script has taken numbers a and b as input. Then I did the arithmetic multiplication. And finally, print the multiplication on the terminal.
The above image illustrates that the Bash script has taken the value of two numbers as input, calculated the multiplication, and printed the result on the terminal.
3. Use of Local and Global Variables in Bash Script
Programmers use a variable as local or global whatever they want. In this example, I will show you how to work with local and global variables. To use local and global variables in bash, follow the below script:
#!/bin/bash
#setting value to number variable
number=10
#defining addition function
function addition()
{
#defining number variable as local variable
local number=1
#defining m variable as local variable
local m=10
#adding number and m variable
(( number=number+m ))
#printing the value of number variable which is local
echo $number
}
#calling addition function
addition
#printing the value of number variable which is global
echo $number
At first number=10 sets a global variable assigning a value 10 to it. Then the addition function is defined and the “number” variable is set as a local variable assigning a value of 10 to it and the same goes for the m variable. After that value of the number variable is added to the value of the m variable and the result is kept to the number variable. Afterward, the value of the number variable is printed on the terminal. Finally, after the end of the addition function, the value of the number variable is printed again. Where the printed value is the value of the global number variable.
The image shows that the Bash script has worked with local and global variables.
4. Using Array Variables in Bash Script
As a Bash programmer, you might need to use an array as a variable in Bash to preserve string data. Here I have a script where I will show the value of all elements of that script along with a key. To learn more about array variables, follow the below script:
#!/bin/bash
my_array=(Cook Graeem Lee Wade Mathew Jumpa)
#count the total number of elements in an array
total=${#my_array[*]}
echo "Total array elements are: $total"
#display value of each element of an array
echo "Array Elements values:"
for val in ${my_array[*]}
do
printf " %s\n" $val
done
#display each array’s element value with a key
echo "Array Elements values with key:"
for key in ${!my_array[*]}
do
printf "%4d: %s\n" $key ${my_array[$key]}
done
The my_array=(Cook Graeem Lee Wade Mathew Jumpa)
command sets the value of the my_array variable. After that the total=${#my_array[*]}
command calculates the length of the my_array variable and prints a line mentioning the total element number on that array. Afterward the for loop prints the value of all values inside of the my_array variable. Then the next for loop finds the key for all elements and prints all of the elements’ values with the key on the terminal.
The image illustrates that the script printed the total number of the elements, all elements of that script along with a key for every element.
5. Command Substitution With Bash Variables
Here I have developed a Bash script that will substitute a command. So, to learn how to substitute commands with bash variables, check the below script:
#!/bin/bash
#command assignment to a variable
variable=$( ls /usr | wc -l )
#printing value on the terminal
echo Total $variable entries on usr directory
The variable=$( ls /usr | wc -l )
command has assigned a command to variable. Then the echo Total $variable entries on usr directory
command has printed a text on the terminal.
The image shows that a variable has substituted a command and printed the output of the command on the terminal.
6. Working With Environment Variables
Here, I will develop a Bash script that will print the SHELL, PWD, HOME and USER variable values which are environment variables. To know how to work with environment variables, check the below script:
#!/bin/bash
#priting SHELL, PWD, HOME, and USER variable value
echo "This user is using $SHELL shell"
echo "Their working directory is: $PWD"
echo "While their home directory is: $HOME"
echo "And the user name is: $USER"
The echo "This user is using $SHELL shell"
, echo "Their working directory is: $PWD"
,echo "While their home directory is: $HOME"
, and echo "And the user name is: $USER"
commands have printed the SHELL, PWD, HOME, and USER variable values respectively which are environment variables.
The image shows that the Bash script has printed the SHELL, PWD, HOME, and USER variable values on the terminal which are environment variables.
7. Quoting String in a Bash Variable
Here I will show you a Bash script that will print the string from the variable along with another string utilizing the quotes. To know how to quote string in a bash variable, check out the below script:
#!/bin/bash
#assigning string to quotes
quotes="Command-line arguments"
#printing the value of quotes on the terminal
echo "Quotes work like this: $quotes"
The quotes="Command-line arguments"
command has assigned a string to the quotes variable. Then the echo "Quotes work like this: $quotes"
command has printed the string from the variable along with another string.
The image shows that the Bash script has printed the string from the variable along with another string utilizing the quotes.
Conclusion
In conclusion, I would like to say that the Bash variable is one of the most important parts of the Bash script. It makes the Bash script more effective for solving problems and automating tasks. After going through this article, I believe you will be productive enough to use the Bash variable on the script.
People Also Ask
What is the use of a variable in bash?
Bash variable stores string or number temporarily. The bash variable is an integral part of Bash scripting. It enables users to write Bash scripts to solve complex problems.
What are the rules for naming variables in bash?
Variable names can contain alphanumeric characters and underscores. It can start with nothing but numbers.
Are bash variables all stored as strings?
Bash variable stores all types of data in a variable as a string. By giving proper instructions, programmers can do arithmetic operations and comparisons on variables.
Where is the shell variable set?
The shell contains the shell variables. They are often used to keep track of ephemeral data, like the current working directory.
Related Articles
- What is Variable in Programming? [The Complete Guide]
- Introduction to Variables in Bash Scripting [An Ultimate Guide]
- Variable Declaration and Assignment
- Types of Variables in Bash
- Variable Scopes in Bash
- Using Variables in Bash Scripting
<< Go Back to Bash Scripting Tutorial