FUNDAMENTALS A Complete Guide for Beginners
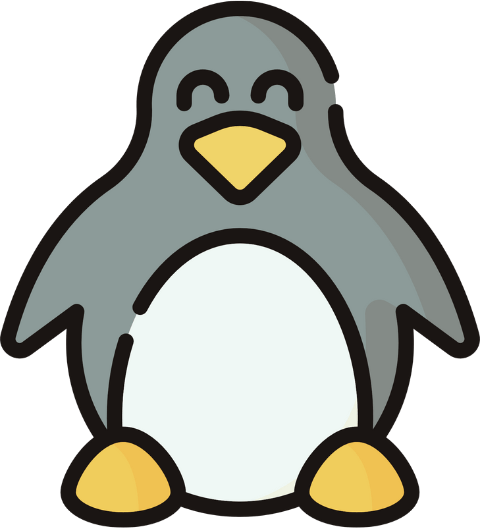
A Bash loop is a control structure that allows you to execute a block of commands or statements repeatedly in a Bash script. Loops are used to automate repetitive tasks, process data, and perform various operations as long as a specified condition is met. However, a loop should have an exit condition to avoid infinite loops.In this tutorial, I’ll discuss the basics of different kinds of bash loops with examples.
Types of Bash Loops in Linux
There are 4 types of loops in Bash that allow you to iterate over a set of commands multiple times. They are:
- For Loop: The for loop is suitable to iterate over a range of values or a list of items.
Syntax >for variable in list do # Commands to be executed for each value in the list done
OR,
for ((initialize; condition; increment)) do # Commands to be executed during condition true done
- While Loop: The while loop is used to execute a block of commands as long as a specified condition is true.
Syntax >while [ condition ] do # Commands to be executed as long as the condition is true done
- Until Loop: The until loop executes a block of commands as long as a specified condition is false.
Syntax >until [ condition ] do # Commands to be executed as long as the condition is false done
- Select Loop: This loop is used for creating simple text-based menus with all the values in a list.
Syntax >select option in "Option 1" "Option 2" "Quit"; do case $option in "Option 1") # commands for Option 1 "Option 2") # commands for Option 2 "Quit") break *) # handle invalid options esac done
You can see the Comparison of Bash Loops to decide which one is suitable for which scenario.
Now in the following sections, I’ll dive into the details of every loop and demonstrate their aspects with example scripts.
Bash For Loop
A Bash for loop is a control structure that iterates over a list of items or elements. It typically consists of three parts: initialization, condition, and increment or decrement. Additionally, it allows you to execute a set of commands for each item in the list.
Bash For Loop on a List
The basic syntax of a for loop on a list in Bash is as follows:
for variable in list
do
# Commands to be executed for each item in the list
done
The for keyword indicates the beginning of a for loop, variable denotes a user-defined variable, and list denotes a list of values. So, the whole line means, for every value stored in the list, follow some commands. Here, do and done mark the beginning and end of the block of commands respectively. Between do and done, commands to be executed in each iteration are written.
Let’s see an example of how the for loop
works. So write the following script inside the Bash file:
#/bin/bash
fruits="apple banana cherry mango"
for item in $fruits
do
echo "I like $item"
done
Here, in #! /bin/bash, #! is called shebang or hashbang. It indicates the interpreter to be used for executing the script. The next line declares and initializes a list named fruits with 4 values (name of fruits). Then for every value in the fruits list, the value is stored in the item variable. Then, the echo command echoes the value of item variable after a quoted message.
The output indicates that the for loop iterates over the 4 values in the fruits list (separated by spaces) and prints “I like” followed by the name of each fruit.
Bash For Loop on a Range
In Bash, you can create a for loop to iterate over a range of numbers using a sequence. To loop through a range, here’s the basic syntax:
for variable in {start..end}
do
# Commands to be executed for each value in the range
done
The for keyword indicates the beginning of a for loop, variable is a user-defined variable that will take on the values in the specified range during each iteration of the loop. {start..end}
denotes the for loop will iterate from start to end, including both values. Here, do and done mark the beginning and end of the block of commands respectively. Between do and done, commands to be executed in each iteration are written.
Let’s look at an example of a for loop
that iterates through a range of numbers. Here’s how:
#! /bin/bash
for num in {1..5}
do
echo "Number: $num"
done
{1..5}
denotes the range of values from 1 to 5. Then for every integer value in the {1..5} range, the value is stored in the num variable. Then, the echo
command echoes the value of the num variable after the quoted message “Number:”.
The output indicates that the for loop iterates over the range {1..5} and prints “Number:” followed by the number in the range.
Note: You can customize the range by specifying different starting and ending values within the curly braces. Additionally, you can use variables to represent the start and end of the range, making it more dynamic.
Bash For Loop in C Style
In Bash, you can create a for loop with initialization, condition, and increment (or decrement) using a C-style loop construct. This allows you to have more control over the loop’s behavior such as increments in a complex manner and improvising multiple conditions. The basic syntax for a for loop with initialization, condition, and increment is as follows:
for ((initialize; condition; increment))
do
# Commands to be executed during condition true
done
- initialize: This is where you set the initial value of a counter variable.
- condition: The condition written here is performed before each iteration of the loop. If the condition is true, the loop continues. If it’s false, the loop exits.
- increment: This is the action to be taken after each iteration. This section typically increments or decrements the counter variable.
- done: Mark the beginning and end of the block of commands respectively. Between ‘do’ and ‘done’, commands to be executed in each iteration are written.
Let’s see an example of a for loop
with initialization, condition, and increment. Here’s the bash script for this:
#! /bin/bash
for ((i = 1; i <= 5; i++))
do
echo "Count: $i"
done
echo “Exit with value: $i”
In the script, the for loop initializes i to 1, checks if i is less than or equal to 5 in each iteration, increments i by 1 after each iteration, and prints the value of i after the message “Count: “. When the value of i becomes 6, the condition (i<=5) is false, and it prints the value of i outside the loop.
The output indicates that the for loop incremented the value of i from the initial 1 to 5 in each iteration and printed its value after the “Count: “ message.
This type of loop is particularly useful when you need fine control over the loop’s behavior, such as when iterating over a range of numbers with specific increments.
Bash While Loop
A while loop is used for executing a block of commands repeatedly as long as a specified condition remains true. In other words, the loop continues to execute until the condition evaluates to false. It is one of the easiest loops to work with. Here, the condition is typically tested before each iteration.
Let’s see an example script of how a bash while loop
works:
#! /bin/bash
count=0
while [ $count -le 5 ]
do
echo "Count: $count"
((count++))
done
echo “Loop exit with count value $count”
The first line initializes the value of count to 0. while keyword indicates the beginning of a while loop. Here, the condition is the value of the variable count is less than or equal to 5. It prints the value of the count variable after the text “Count: “ and increments the count by 1 in every iteration. When count becomes 6, control comes outside of the loop as condition (count <=5 ) false.
The output shows that while loop executed the echo command inside do..done block as long as the count value was less than or equal to 5. Then condition became false and it echoed the count value from outside the loop.
Bash Until Loop
In Bash, the until loop is a control structure that allows you to execute a block of commands repeatedly as long as a specified condition remains false. In other words, the loop continues to execute until the condition becomes true. So, it’s opposite to the while loop. Here’s an example of an until loop
:
#! /bin/bash
count=5
until [ $count -lt 1 ]
do
echo "Count: $count"
((count–))
done
echo “Exit with count value $count”
The first line initializes the value of count to 5. until keyword indicates the beginning of a while loop. Here, the condition is the value of the variable count is less than 1. It prints the value of the count variable after the text “Count: “ and decrements the count by 1 in every iteration. When count becomes 0, control comes outside of the loop as condition (count < 1 ) true.
The output shows that the until loop executed the echo command inside the do..done block as long as the count value was not less than 1. Then condition became true (count < 1) it echoed the count value from outside the loop.
Bash Select Loop
The select loop in Bash is a specialized loop used for creating simple text-based menus. It allows you to present a list of choices to the user and take their selection as input. However, it is particularly useful for building interactive command-line interfaces (CLI). Here’s the basic syntax of the select loop
:
select variable in list
do
# Commands to be executed based on the user's selection
done
The select keyword indicates the beginning of a select loop, variable denotes a user-defined variable, and list denotes a list of values. So, the whole line means, for every value stored in the list, follow some commands. Here, do and done mark the beginning and end of the block of commands respectively. Between do and done, commands to be executed in each iteration are written.
Here’s an example of a select loop
that presents a menu for the user to choose a color:
#! /bin/bash
colors=("Red" "Blue" "Green" "Yellow") # List of color options
select choice in "${colors[@]}"
do
case $choice in
"Red")
echo "You chose Red. It's a warm color."
;;
"Blue")
echo "You chose Blue. It's a cool color."
;;
"Green")
echo "You chose Green. It's associated with nature."
;;
"Yellow")
echo "You chose Yellow. Exiting the menu."
break
;;
*)
echo "Invalid choice. Please select a valid option."
;;
esac
done
In this example, an array called colors contains the options for the user to choose from. The select loop displays the menu, and the user can make a selection. Depending on the choice, a corresponding message is displayed. The loop continues until the user selects “Yellow”.
The output shows that the script performs different echo commands according to the selection.
Loop Control in Bash
In Bash scripting, loop control refers to mechanisms that allow you to modify the behavior of loops. In this regard, you can use the break
and continue
statements to control the loop flow (such as for, while, and until loops) and exit or skip iterations based on certain conditions.
Loop Control in Bash Using Break Statement
The break statement is used to exit a loop prematurely. When it’s encountered within a loop, it immediately terminates the loop and continues with the next command after the loop. So, you will typically use it when you want to exit a loop based on a specific condition, breaking out of the loop early.
Here’s an example of using a break
statement in a for loop:
#! /bin/bash
for i in {1..10}
do
if [ $i -eq 5 ]; then
break
fi
echo $i
done
echo “Exit loop”
{1..10}
denotes the range of values from 1 to 10. The variable i iterates over every value of the range and the echo
command echoes the value of the i variable. But in the above example, when the value of i is equal to 5, the loop breaks. So, although the range specified to run from 1 to 10, control goes outside the loop block when i=5.
The output prints the iterated values by the loop and starts executing commands written after the loop block as soon as the condition to break is met.
Loop Control in Bash Using Continue Statement
The continue statement is used to skip the current iteration of a loop and proceed to the next iteration. So, it is useful when you want to skip a specific iteration of a loop based on a condition without exiting the entire loop.
Here’s an example of using the continue
statement in a for loop:
#! /bin/bash
for i in {1..5}
do
if [ $i -eq 3 ]; then
continue
fi
echo $i
done
echo “Loop complete”
{1..5}
denotes the range of values from 1 to 5. The variable i iterates over every value of the range and the echo command echoes the value of the i variable. But in the above example, when i=3, it skips the later commands and control goes to the new iteration. So, it will print 1 to 5 except 3.
The output prints the iterated values except 3 and starts executing commands written after the loop block when all iteration is complete.
Comparison of Bash Loops
So far I’ve discussed 4 types of Bash loops and their usage in script. You may feel confused when choosing among them. Here is a general comparison among loops in Bash:
Loop | Characteristic | Usage |
---|---|---|
For Loop | Has a clear structure with an initialization, condition, and an increment/decrement statement. They also can be used with ranges and lists. | It is suitable to use when you know in advance how many times you need to iterate. |
While Loop | Has a condition that’s evaluated at the beginning of each iteration. | It is suitable to use when you want to continue executing a block of commands as long as a condition is true. |
Until Loop | It is a control structure that allows you to execute a block of commands repeatedly as long as a specified condition remains false. | Use the loop when you have to execute until the condition becomes true. |
Select Loop | Presents a list of items as options to the user, and the user selects one option from the list. | It is suitable for creating text-based menus in interactive scripts. |
Keep in mind that, there is no bound to use a specific loop in a specific scenario. A loop can be replaced by another one by modifying the script logic a little. So, keep developing your logic by practicing it.
Practice Tasks on Bash Loop
Practicing with Bash loops is a great way to improve your scripting skills. Here are some basic practice tasks to get you started. Try to write solutions and share your answers in the comment section.
- Print the sum of the first N numbers using the for and while loops.
- Print the even numbers between 1 to 10 using continue.
- Choose a number between 1 to 10 using select and print whether it is prime or not.
- Print 10 to 1 reversely except 5 using the until loop.
- Create a script that generates random passwords of a specified length.
Conclusion
Loops are fundamental in Bash scripting and are used for tasks like iterating through files, processing data, and creating scripts that automate various actions. Properly constructed loops help you streamline tasks and efficiently manage repetitive operations. However, it’s important to ensure that your loop has an exit condition to avoid infinite loops, which can lead to high CPU usage and unresponsive scripts.
People Also Ask
What is the use of continue statement in Bash while loop?
The continue
statement in Bash while loop
is used to skip the current iteration of a loop and proceed to the next iteration. So, it is useful when you want to skip a specific iteration of a loop based on a condition without exiting the entire loop.
How many loops are in Bash?
There are 4 kinds of loops in Bash. They are: for loop, while loop, until loop, and select loop. These four loop types provide different mechanisms for controlling program flow and iteration in Bash scripts. The choice of loop type depends on the specific requirements of your script and the conditions you need to check.
What is exit condition in a loop?
An exit condition in a loop is a condition that, when met, causes the loop to terminate or exit prematurely. It allows you to control the number of iterations and the circumstances under which a loop should terminate. In Bash scripting, exit conditions are typically used with while
and until
loops to control when the loop should stop executing its block of commands.
Can I run loops from the command line?
Yes, you can run loops directly from the command line in Bash. This is often useful for quick, one-time tasks or for testing purposes. You can use inline loops to perform specific actions without creating a separate Bash script file. A basic example of running a for loop from the command line: for i in {1..5}; do echo $i; done
.
How to replace while loop by until loop?
You can replace the while loop with an until loop by altering the condition used in it. Generally, the while loop runs as long as the condition is true, and the until loop runs as long as the condition remains false. For example, printing 1 to 5 with a while loop is:
count=1
while [ $count -le 5 ]
do
echo "$count"
((count++))
done
You can replace it with:
count=1
until [ $count -gt 5 ]
do
echo “$count”
((count++))
done
Is select a loop?
Yes, select is sometimes referred to as a type of loop in Bash although it’s not a loop in the traditional sense like for, while, or until. Basically, it is a control structure that creates a simple menu system for interactive scripts until a specific choice is made. In this iterative sense, select is considered a type of loop.
Related Articles
- For Loop in Bash
- While Loop in Bash
- Until Loop in Bash
- Select Loop in Bash [5 Practical Examples]
- How to Use Loop Control in Bash [Break & Continue Statement]
<< Go Back to Bash Scripting Tutorial
answer to print the even numbers
#!/bin/bash
for i in {1..10}
do
if [[ $i -eq 1 ]];then
continue
elif [[ $i -eq 3 ]];then
continue
elif [[ $i -eq 5 ]];then
continue
elif [[ $i -eq 7 ]];then
continue
elif [[ $i -eq 9 ]];then
continue
fi
echo $i
done
echo “You win the even number’s game :)”
Thanks for your response. You’re correct and really win the even number’s game! But what do you think about this code below?
#!/bin/bash
for ((i=1; i<=10; i++))
do
#Check if the number is not even by using modulo
if ((i % 2 != 0))
then
continue
fi
echo $i
done
This is short and saves your time.