FUNDAMENTALS A Complete Guide for Beginners
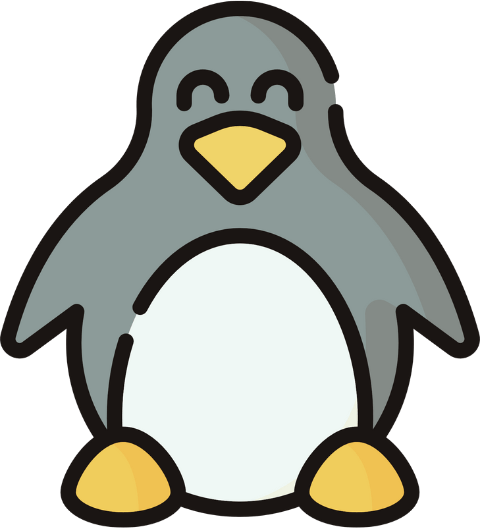
Bash presents different types of loops and one of them is the while loop. A while loop in bash is a set of commands that repeat as long as a certain condition is met. To automate repetitive tasks, it is crucial to learn about the while loop. In this article, I will provide an overview of the while loop with its behavior under various conditions, as well as some of the key commands associated with a while loop, such as break, continue, etc. Additionally, 7 practical cases will be presented to illustrate the concept.
What is “while” Loop in Bash?
In Bash, a while loop is a repeating sequence of statements, provided that a specified condition is true. The loop evaluates the condition before executing any commands. It is used to iterate through a series of operations that enable you to perform repetitive tasks. Once the operation is completed, the loop is terminated with the done keyword. Let’s see an illustration for a clearer overview of how the while loop works:
Now, let’s see the basic syntax of the while loop:
while [ condition ];
do
#Statements to be executed as long as the condition is true
done
- while [ condition ]: Starts the while loop, which will run as long as the condition is true. You can replace this condition to check your desired one.
- do: Indicates the start of the statements that execute in every iteration of the loop.
- #Statements to be executed as long as the condition is true: These statements will be executed over and over again as long as the condition is true.
- done: Marks the end of the while loop.
7 Cases of “while” Loop in Bash
In this section, I will explain 7 different cases of the while loop. How the while loop works with single or multiple conditions will be described here. Then the infinite while loop, break and continue statements, nested while loops, etc. will be covered. Finally, I’ll demonstrate the while loop in one line over multiline codes.
1. “while” Loop With Single Condition in Bash
The while loop can check a single condition and execute the output based on the condition. Here, the condition is $number -le 5
which checks whether the number is less than or equal to 5. The ((number++))
syntax increases the number in each iteration by 1 until the condition is met. Follow the script below to see the entire process:
#!/bin/bash
number=1
while [ $number -le 5 ]; do
echo "Iteration: $number"
((number++))
done
echo "Iteration completed."
The while loop is initiated by taking a variable number with the value of 1 and incrementing it by 1 in each iteration. The output of each loop is printed by the echo “Iteration: $number”. The loop is then continued until the value is equal to or less than 5. Upon completion of the loop, it echoes the message “Iteration completed”.
In this picture, you can see that the script executes 5 iterations and completes the loop when the value is 5 to meet the specified condition.
2. “while” Loop With Multiple Conditions in Bash
To run multiple conditions using a while loop, you can use logical operators to combine the conditions. I will use the OR ||
operator in this example to execute multiple conditions within a while loop. The conditions involved two comparison operators -lt
(less than) and -eq
(equal to). Here’s how:
#!/bin/bash
read -p "Enter number1: " number1
read -p "Enter number2: " number2
while [[ $number1 -lt $number2 || $number1 -eq $number2 ]];
do
echo $number1
((number1++))
done
After running the script, it prompts the users to enter number1 and number2. The while loop prints the number1 and increments it by 1 in every iteration if it is equal to or less than the number2. When the condition is met, number1 becomes greater than number2, the loop terminates.
As you can see, after each loop, number1= 1 increases by 1, and the loop continues until number1 is equal to or less than number2= 3.
3. Infinite “while” Loop in Bash
An infinite while loop is a kind of loop that runs infinitely. There is no end to the loop. If the conditions are always true, then the infinite while loop will be created and it will continue to run until you press CTRL+C in the terminal to forcefully end the loop. Let’s look at the bash script to see how the infinite loop works:
#!/bin/bash
#infinite while loop
while :
do
echo "Welcome to Linuxsimply."
done
The infinite while loop is created as the “:”
built-in command always returns true. After starting the loop, it continues to echo “Welcome to Linuxsimply.” over and over in the terminal.
Running the script with infinite.sh
, it shows an infinite loop that prints Welcome to Linuxsimply infinitely. To exit out of the infinite loop, type CTRL+C and press ENTER.
4. “while” Loop With “break” Command
Break command is essential in controlling any loop. It lets you get out of a while loop without having to give any explicit command. In this example, I will show how to exit from an infinite while loop using the break command. Let’s look at the bash script to understand how the break command works here:
#!/bin/bash
number=1
while :
do
echo $number
if [ $number -eq 5 ]; then
echo "End of the loop"
break
fi
((number++))
done
Here, while :
will create an infinite loop but when the condition $number -eq 5
within the if
statement is satisfied, the break
statement will break the infinite loop. The -eq
operator inside the condition checks the equality between numbers. When you run the script, it creates an infinite loop and then prints the value of the current number in every loop. When the number gets to 5, it prints “End of the Loop” and exits the loop with the break command.
As you can see, the loop is terminated when the number becomes 5.
5. “while” Loop With “continue” Command
The continue command is another important command to control the flow of the loop. It is the opposite of the break command. It jumps to the start of the loop block and iterates again by skipping certain commands based on certain conditions. Here’s an example of it:
#!/bin/bash
number=-3
while [ $number -lt 5 ]; do
((number++))
# Check if the number is negative or positive
if [ $number -lt 0 ]; then
echo "Skipping negative number: $number"
continue
fi
echo "Positive number: $number"
done
The script initializes by setting the number to -3. The while loop increments the number until it reaches 5. During this time, the [ $number -lt 0 ]
condition with the -lt
(less than) option checks whether the current number is less than zero (negative number). If the number is negative, it jumps to the next iteration using the continue
command. Otherwise, it prints the positive numbers.
In this image you can observe that, the script displays all positive numbers 0 to 5 skipping negative numbers -2 and -1 with the continue command.
6. Nested “while” Loop in Bash
A nested while loop is executing one while loop inside another while loop. The structure executes a set of commands within the inner loop after each iteration of the outer loop. In this example, I will show how a nested while loop works. For that, an inner while loop condition is nested inside another outer while loop condition. Let’s check the whole bash script:
#!/bin/bash
outer_loop=1
while [ $outer_loop -lt 4 ]; do
inner_loop=1
while [ $inner_loop -lt 5 ]; do
result=$((outer_loop + inner_loop))
echo "$outer_loop + $inner_loop = $result"
((inner_loop++))
done
((outer_loop++))
done
The outer loop in this script iterates until the number is less than 4, and the inner loop will iterate until the number is less than 5. Then, the result of the addition of the outer loop and inner loop will be printed in the terminal. Both the outer and inner loops are incremented at the end of their respective loop to control the iteration flow.
This output shows the combinations and outcomes of the addition operations in the nested while loop.
7. “while” Loop in One Line
Multiline while loop codes can be written in one line using semicolon (;). The semicolon separates the loop variable, loop condition, loop body, and all other parts. The use of a single-line while loop is advantageous, particularly while dealing with straightforward syntax. Nevertheless, for more complex operations, this syntax can be avoided to preserve the readability of the script.
Here’s an example of one line while code to increase the number from 0 to 5:
#!/bin/bash
number=0; while [ $number -lt 6 ]; do echo "$number"; ((number++)); done
The loop initializes the variable number with a 0 value. Then the loop starts and continues until the number becomes less than 6. During each iteration, it prints the current value of the number.
This image shows that the number increases by 1 in each iteration and ends with 5 since the condition is satisfied.
Conclusion
To sum up, here I’ve shown you how a while loop works and given you 7 cases of how to use a while loop. If you’ve read this article, you’ll know about the fundamentals of while loop. By mastering these basics, you will be able to handle the while loop more efficiently. Have a great read!
People Also Ask
What is the difference between a while loop and a do-while loop in Bash?
There is no do-while loop in bash. In contrast to other programming languages, the while loop ensures that the loop body executes at least once because the condition is evaluated after the loop is executed.
How can I use a while loop to wait for a condition?
You can use the sleep
command between the while loop iterations to wait for a condition. For example:
while [ Condition ]; do
sleep 1
done
Here sleep 1 introduces a delay of 1 second in each iteration of the loop. This command is commonly used to pause the execution of a script or a loop for a specified duration.
Can I nest while loops in bash?
Yes, definitely, you can nest while loops like other programming languages in bash. Here’s a syntax of nested while loop:
while [ outer loop condition ]; do
# Outer loop statement
while [ inner loop condition ]; do
# Inner loop statement
done
# Statement after the inner loop
done
Is it possible to exit a while loop before a condition is false?
Yes, you can use the break
command to exit a while loop before a condition is false. Here’s the syntax of the break command.
while [ condition ]; do
# Statement to be executed
if [ condition to break ]; then
break
fi
done
How to read a file line with a while loop in bash?
To read a file line with a while loop in bash, you can use the read -r
command in the while loop. Here’s the full bash script for this:
#!/bin/bash
# Check if a filename is provided as a command-line argument
if [ $# -eq 0 ]; then
echo "Usage: $0 <filename>"
exit 1
fi
filename=$1
# Use a while loop to read the file line by line
while IFS= read -r line; do
echo "Line: $line"
# Add your processing logic here
done < "$filename"
This script takes the filename as a command line argument and then reads the file directly. If the file doesn’t exist, it will show an error.
What is C-style while loop in bash?
The C-style while loop in bash is the loop that follows the syntax and structure of the while loop of the C programming language. Here’s the basic syntax:
#!/bin/bash
counter=0
while ((counter < 5))
do
echo "Counter: $counter"
((counter++))
done
How do I count the number of iterations in a while loop in bash?
You can count the number of iterations in a while loop with ((counter++))
syntax inside the while loop. It increments the iteration number in each loop by 1. Here’s the bash script for this:
#!/bin/bash
# Initialize the counter variable
counter=0
while [ "$counter" -lt 10 ]; do
# Increment the counter
((counter++))
done
# Display the number of iterations
echo "Number of iterations: $counter"
The while loop continues to increment the iteration number from 0 to 9 and when the number becomes 10, the loop will be terminated. The output of this script will be: Number of iterations: 10.
Related Articles
- “while true” Loop in Bash [4 Cases]
- 8 Examples of “while” Loop in Bash
- How to Read File Line Using Bash “while” Loop [8 Cases]
- How to Increment Number Using Bash “while” Loop [8 Methods]
- One Line Infinite “while” Loop in Bash [4 Examples]
- How to Use Bash Continue with “while” Loop [7 Examples]
- Exit “while” Loop Using “break” Statement in Bash [11 Examples]
- How to Use Bash One Line “while” Loop [8 Examples]
- How to Use “sleep” Command in Bash “while” Loop [6 Examples]
<< Go Back to Loops in Bash | Bash Scripting Tutorial