FUNDAMENTALS A Complete Guide for Beginners
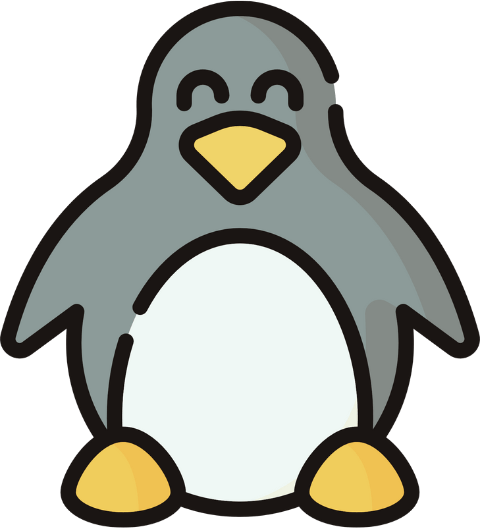
Incrementing and decrementing numbers is one of the most common arithmetic operations in bash scripting, crucial for creating dynamic and responsive scripts. So to increment a number in bash, you can use arithmetic operators like ++, +=, and +. Moreover, some built-in commands such as let, bc, expr, and awk can be used to do so. In this article, I will provide you with the 8 simplest methods of how to increment a number in bash using a while loop. Let’s delve into the methods.
8 Methods to Increment Number Using Bash “while” Loop
A number can be incremented within a while loop in 8 ways. Let’s see each method thoroughly.
1. Using “+=” Operator
A “+=” operator within a while loop can be applied to increment a number in bash. With the syntax ((number+=1))
, the assignment operator (+=) is used to increment the number by adding the value on the right (1 in this case) to the variable on the left (number). Moreover, this script makes use of the -lt
(less than) operator which is a comparison operator in Bash.
Follow the script to see how the while loop works with the +=
operator to increment number:
#!/bin/bash
number=0
while [ $number -lt 6 ]
do
echo Number: $number
((number+=1))
done
The script initializes with a variable number with a value of 0. The while [ $number -lt 6 ]
starts a while loop and continues as long as the number becomes less than 6. echo Number: $number
prints the current value of the variable “number” inside the loop and increments the value of “number” by 1 in each iteration with ((number+=1))
syntax.
As you can see the while loop prints the number from 0 to 5, incrementing by 1 in each iteration till it reaches 5.
2. Using “++” Operator
The simplest way to increase a number in Bash is using the ++
operator. It increments a variable’s current value by 1. There are two ways to use this operator: postfix and prefix. Now, to know how postfix and prefix methods work with a while loop, follow the cases:
Case 01: Postfix Increment
In the postfix increment ((number++))
within a while loop, the current value of a number is used in the expression, and then it is incremented. So, in the first iteration of the loop, the number is used as 0, and after that, it is incremented by 1. Here’s how:
#!/bin/bash
number=0
while [ $number -lt 6 ]
do
echo Number: $number
((number++))
done
while [ $number -lt 6 ]
starts a while loop that continues executing the code inside the loop as long as the condition within the square brackets [ ] is true. In this case, the condition is that the value of the number is less than 6. The echo command prints the current value of the number and ((number++))
increments the number value by 1 in each iteration.
You can see that the script increments by 1 for each iteration, printing numbers from 0 to 5.
Case 02: Prefix Increment
In the prefix increment ((++number))
within a while loop, the variable is incremented first, and then its new value is used in the expression. So, in the first iteration, the number is incremented to 1 before being used in the loop. Check the script below:
#!/bin/bash
number=0
while [ $number -lt 6 ]
do
echo Number: $number
((++number))
done
After assigning the value 0 to the number variable, while [ $number -lt 6 ]
starts the loop to iterate and breaks the loop when the value of the number is no longer less than 6. ((++number))
increases the value of the number by 1 in every iteration and prints the current iteration number by the echo
command.
Upon executing the script, it prints the numbers from 0 to 5 using the prefix operator.
3. Using “+” Operator
Another easiest approach to increase a number in Bash is to use the +
operator in the while loop. Here, ((number=number+1))
syntax is used to increment a number by 1. The bash script to complete the task is as follows:
#!/bin/bash
number=0
while [ $number -lt 6 ]
do
echo Number: $number
((number=number+1))
done
The while loop
starts and keeps incrementing the value of the number variable by 1 in every iteration using the ((number=number+1))
syntax. When the number becomes greater than 5, the loop terminates.
The picture shows that the number is increasing by 1 and prints from 0 to 5.
4. Using “let” Command
For more flexibility, a built-in “let” command can be used to increase a number variable in place of double parentheses ((...))
. Now, have a look at the following bash script to increase the number using the let
command in a while
loop:
#!/bin/bash
number=0
while [ $number -lt 6 ]
do
echo Number: $number
let number++
done
Within the while loop, the let number++
syntax is used to increment the number variable by 1 in each iteration. The while loop
ends if the number becomes greater than 5.
The image indicates that the number is printing from 0 to 5 and is increasing by 1.
5. Using “expr” Command
The “expr” command in Bash can be used for arithmetic operations like incrementing or decrementing a number. In this example, $(expr $number + 1)
performs the addition operation. This expression takes the current value of the number, adds 1 to it, and assigns the result back to the number. Here’s the bash script to increment numbers using the expr
command:
#!/bin/bash
number=0
while [ $number -lt 6 ]
do
echo Number: $number
number=$(expr $number + 1)
done
A variable number with the value 0 is used at the beginning of the script. The while loop
then begins, printing numbers till they are less than 6. In this case, number=$(expr $number + 1)
executes the addition operation using the expr
command. It takes the number’s current value and returns the result to the number variable by adding 1.
As you can see, the number is increasing from 0 to 5 using the “expr” command.
6. Using C-Style “while” Loop
A loop construct in Bash that closely mimics the syntax of the C programming language is called a C-style while loop. There are two methods to employ this loop in an incrementation operation: postfix and prefix. Let’s explore each strategy in more detail.
Case 01: Postfix Increment
In the C-style while loop in bash, first, the expression uses the current value of the number and then, the number is incremented using the ((n++))
. Here, the following bash script performs the post-increment operation:
#!/bin/bash
n=0
while ((n < 6)); do
echo "Number: $n"
((n++))
done
The variable n assigns a value 0. while ((n < 6)); do
starts a while loop that continues as long as the value of the n is greater than or equal to 6. The echo
command prints the current value of the n and ((n++))
increments the value of the n variable by 1 in each iteration.
After running the script, you will get the following output:
Case 02: Prefix Increment
Within a C-style while loop, in the prefix increment format ((++n))
, the variable n is incremented first, and then its updated value is used in the expression: Here’s how:
#!/bin/bash
n=0
while ((n < 6)); do
echo "Number: $n"
((++n))
done
while ((n < 6)); do
starts the loop to iterate and breaks the loop when the value of the number is greater than or equal to 6. ((++n))
increases the value of the n by 1 in every iteration and the echo
command prints the current iteration number.
The following will be produced when the script is run:
7. Using “bc” Command
In this example, the bc command (basic calculator) will be incorporated with a while loop to increment the number in bash. This command allows more complex arithmetic operations like calculating floating point numbers. To know how to use the bc
command for incrementing non-integer numbers, check the script below:
#!/bin/bash
number=1.5
while [ $(echo "$number < 6" | bc -l) -eq 1 ]
do
echo "Number: $number"
number=$(echo "$number + 1" | bc)
done
number=1.5
initializes a variable named number with the value 1.5. while [ $(echo "$number < 6" | bc -l) -eq 1 ]
starts a while loop that continues as long as the result of the comparison $number < 6
is equal to 1. The bc -l
option is used for performing floating-point arithmetic in bc. echo "Number: $number"
prints the current value of the number. number=$(echo "$number + 1" | bc)
increments the value of the number by 1 in each iteration of the loop. The bc
command is used for floating-point addition.
As you can see, the non-integer number is incremented from 1.5 to 5.5.
8. Using “awk” Command
Another way to increment non-integer numbers is the use of the awk command within the while loop. To learn how to use the awk
command to increment non-integer numbers, follow the script:
#!/bin/bash
number=1.5
while [ $(awk -v n="$number" 'BEGIN{print(n < 6)}') -eq 1 ]
do
echo "Number: $number"
number=$(awk -v n="$number" 'BEGIN{print(n + 1)}')
done
while [ $(awk -v n="$number" 'BEGIN{print(n < 6)}') -eq 1 ]
starts a while loop and continues to execute the code within the loop as long as the result of the comparison $number < 6
is equal to 1. The awk -v
option is used to pass the value of the number to the awk command. echo "Number: $number"
prints the current value of the number. number=$(awk -v n="$number" 'BEGIN{print(n + 1)}')
increments the value of the number by 1 in each iteration of the loop. The “awk -v” option is used to pass the value of the number to the awk command to perform a floating-point addition.
The non-integer number is incremented from 1.5 to 5.5.
Common Problems in Incrementing Numbers in Bash
You could come across some issues while attempting to increment non-integer values with Bash. It may also run into certain errors if a variable is not defined. Let’s see how you can get past these obstacles.
Incrementing Non-Integer Number
All the arithmetic operators and commands in Bash except the bc
and awk
commands work with integer values. So if you want to increment a floating point number like 1.5 using those methods, you will get an error like this:
#!/bin/bash
number=1.5
while [ $number -lt 6 ]
do
echo Number: $number
((number+=1))
done
To avoid this problem, follow the methods discussed in method 7 and method 8.
Variable Not Defined Error
Sometimes you may forget to define a variable before starting to increment the number within a while loop. When that happens, the bash script shows an error like this:
#!/bin/bash
while [ $number -lt 6 ]
do
echo Number: $number
((number+=1))
done
As the variable number is not defined in the script, it shows an error. So, always initialize a variable beforehand to prevent this issue from occurring.
How to Decrement Number Using Bash “while” Loop
Decrementation is the opposite of incrementing numbers. In Bash, decrementing can be achieved using the arithmetic operators within a while loop. Additionally, a C-style while loop can be used to accomplish this task.
1. Using “- -” Operator
To decrement a number in Bash, use the --
operator in a while loop. With the ((number--))
syntax, the number will be decreased by 1 in each iteration. Copy the script below to achieve this:
#!/bin/bash
number=6
while [ $number -gt 0 ]
do
echo "Number: $number"
((number--))
done
number=6
initializes a variable called number with the value 6. while [ $number -gt 0 ]
starts the while loop and continues to decrease the number until it is no longer greater than 0. echo "Number: $number"
prints the current value of the number and ((number--))
decrements the value of the number by 1 in each iteration of the loop.
As you can observe, the number 6 is decrementing by 1 in each iteration till it reaches 1.
--
to decrease the number variable, you can use -=
or -
. Additionally, the commands let, expr, bc, and awk can be used for this.2. Using C-Style “while” Loop
Like incrementing a number using a C-style while loop, the decrementation can be obtained. ((n--))
executes post-decrement in this example. Pre-decrement can also be accomplished using the ((--n))
syntax. Here’s how:
#!/bin/bash
n=6
while ((n >= 0)); do
echo "Number: $n"
((n--))
done
n=6
assigns a value of 6 to the variable n. while ((n >= 0)); do
starts a while loop that runs as long as the value of the n is greater than and equal to 0. The echo "Number: $n"
command prints the current value of the n and ((n--))
decreases the value of the n by 1 in each iteration.
After executing the script, you will get the output as follows:
Practice Tasks on How to Increment Number Using Bash “while” Loop
To elevate your skills on how to increment number in Bash using a while loop, do the following practices:
- Make a bash script that increases a number from 1 to 10 by incrementing 1 in each iteration.
- Create a bash script using a while loop that prints only even numbers.
- Generate a bash script that increments a number by 5 in each iteration.
- Write a bash script that increments a floating point number from 0.5 to 5.5 by increasing 0.5 in each iteration.
Conclusion
To conclude, I have covered 8 methods of how to increment number in Bash using a while loop. In addition, I have explained some common issues encountered during incrementing numbers. You will also get to know how to decrease the number in bash. Have a great day!
People Also Ask
How to increase number in shell script?
To increase the number in the shell script, you can use ((number++))
syntax within a while loop. It adds 1 in each iteration of the loop. Here’s an example bash script that increments the number=1
by 1 in every iteration till it reaches 6:
#!/bin/bash
number=1
while [ $number -lt 7 ]
do
echo Number: $number
((number++))
done
What are increment and decrement operators in Bash?
In Bash, the increment and decrement operators are used to increase or decrease the value of a variable by a specified amount. The two common operators for incrementing and decrementing in Bash are ++
and --
. These operators usually increase and decrease by 1 in every iteration.
What is $$ in shell?
In a shell script, $$
is a special variable that represents the process ID (PID) of the currently running script or shell.
What is the += in shell script?
The +=
is an assignment operator used to concatenate or append values to a variable. It can be used to increment the number in bash.
Is ++ an increment operator?
Yes, ++
is the increment operator in many programming languages, including Bash. It is used to increase the value of a variable by 1. There are two forms of the increment operator: pre-increment ((++variable))
and post-increment ((variable++))
.
What syntax will increment $I by 1 at every iteration Bash?
((I++))
will increment $I by 1 at every iteration in Bash.
Is increment a binary operator?
No, the increment operator is not a binary operator. It is a unary operator that works on a single operand.
Related Articles
- “while true” Loop in Bash [4 Cases]
- 8 Examples of “while” Loop in Bash
- How to Read File Line Using Bash “while” Loop [8 Cases]
- One Line Infinite “while” Loop in Bash [4 Examples]
- How to Use Bash Continue with “while” Loop [7 Examples]
- Exit “while” Loop Using “break” Statement in Bash [11 Examples]
- How to Use Bash One Line “while” Loop [8 Examples]
- How to Use “sleep” Command in Bash “while” Loop [6 Examples]
<< Go Back to “while” Loop in Bash | Loops in Bash | Bash Scripting Tutorial