FUNDAMENTALS A Complete Guide for Beginners
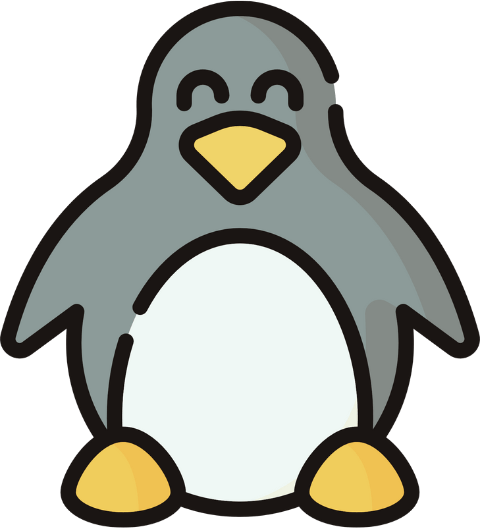
In Bash, the sleep command in combination with a while loop executes a series of statements repeatedly with a specified time interval. The sleep command is a powerful tool in Bash scripting that introduces a delay in the execution of the scripts. Therefore, if you want to have more control over your bash scripts’ execution time, then read this thoroughly. In this article, I will show you 6 examples of using the sleep statement in bash while loop. By harnessing the potentiality of the sleep command, you will be able to optimize your bash scripts’ timing and efficiency.
Basics of “sleep” Command
The sleep
command is used to pause or delay the execution of the script for a specified period. The duration can be specified as a second, minute, hour, or a combination of these. The syntax of using a sleep command in a while loop is:
while [ condition ]; do
# Code to execute in each iteration
sleep duration
done
while [ condition ];: Starts the while loop with a specified condition in square brackets “[]”. The loop continues executing as long as this condition is true.
do: Marks the beginning of the loop body.
# Code to execute in each iteration: This is where you insert the code or commands that you want to execute in each iteration of the loop. Replace this comment with your actual code.
sleep duration: It causes the loop to pause for the specified duration before moving on to the next iteration. Duration can be an integer or a fraction number.
done: Indicates the end of the loop body.
6 Examples of Using “sleep” Command in Bash “while” Loop
In a while loop, the sleep command can be used to carry out several tasks, such as downloading a file, monitoring a process, or creating a simplified counter. Here, I will explain 6 practical examples of using the sleep command in bash while loop. Let’s delve into the details.
1. Counter Loop With “sleep” Command
A counter can calculate the iteration count of a while loop. In this example, the script counts the iteration count by taking a 1-second break in each iteration due to the sleep
1 statement. Also, it uses the comparison operator -le
(less than and equal to) to set the condition inside the while loop.
Now, follow the script to see how the counter while loop with sleep
statement works:
#!/bin/bash
counter=1
while [ $counter -le 6 ]; do
echo "Iteration: $counter"
((counter++))
sleep 1
done
The script initializes the variable counter with a value of 1. while [ $counter -le 6 ]; do
starts a while loop that continues as long as the value of the counter is less than or equal to 6. It prints the current iteration number with echo "Iteration: $counter"
. Then the value of the “counter” is incremented by 1 in each iteration using ((counter++))
syntax. Finally, sleep 1
introduces a 1-second delay before the next iteration.
You can see that the while loop prints the iteration number from 1 to 6 by taking a one-second pause at each iteration.
The sleep duration can be a floating point number like this:
#!/bin/bash
counter=1
while [ $counter -le 6 ]; do
echo "Iteration $counter"
sleep 0.5
((counter++))
done
The script pauses for 0.5 seconds before going to the next iteration using the sleep 0.5
command.
The image shows that the while loop takes a 0.5-second break at each iteration to print the iteration number between 1 and 6.
2. Iterating With Custom Interval
The sleep command can be used with a custom interval. For that, a variable is defined before starting the while loop to set the execution time between each iteration according to the users. Follow the script to learn how to customize the interval using the sleep
command:
#!/bin/bash
n=0
interval=2
while [ $n -le 5 ]; do
echo "Iteration: $n"
((n++))
sleep $interval
done
First, a variable n is defined with a value of 0. Then the interval value is set to 2 which represents the number of seconds to sleep between each iteration. while [ $n -le 5 ]; do
initiates a while loop that continues as long as the value of n is less than or equal to 5. The current number of the iteration is printed by the echo command. ((n++))
increments the value of n by 1 in each iteration. sleep $interval
introduces a sleep interval of 2 seconds before the next iteration.
Upon executing the script, it displays the iteration number from 0 to 5 by taking a 2-second interval at every iteration.
3. Monitoring a Process
It is possible to monitor a process whether it is running or not with the sleep command inside the while loop. In this example, the MySQL process is continuously monitored. If the service is not running, it attempts to restart the MySQL service using systemctl
and repeats the process with a 10-second interval. Here’s how:
#!/bin/bash
while true; do
# Check if MySQL is running
if pgrep -x mysqld > /dev/null; then
echo "MySQL is running."
else
echo "MySQL is not running. Attempting to restart..."
systemctl restart mysql
echo "MySQL restarted."
fi
sleep 10
done
while true; do
initiates an infinite loop. Inside the loop, pgrep -x mysqld
checks if the MySQL process is running. If it is, it prints a message indicating that MySQL is running. If MySQL is not running, it prints a message indicating that MySQL is not running, attempts to restart MySQL using systemctl restart mysql
, and prints a message indicating that MySQL has been restarted. Before starting the service, it will ask for your system password for authentication. sleep 10
introduces a sleep interval of 10 seconds before the next iteration of the loop.
Since MySQL is not running in my system, it restarts the process to run.
4. Creating a Simple Clock Using “sleep” Command
A simple clock can be created using the sleep
command within a while loop. The while loop continuously updates the clock in the terminal and refreshes the display every second, providing an ongoing representation of the current time. Copy the script below to accomplish this task:
#!/bin/bash
while true; do
clear # Clears the terminal screen for a cleaner display
current_time=$(date +"%T") # Get the current time in HH:MM:SS format
echo "Current Time: $current_time"
sleep 1 # Refresh every 1 second
done
After starting the infinite loop, it uses clear
command to clear the terminal screen, ensuring a clean display for the current time. current_time=$(date +"%T")
uses the date command to retrieve the current time in HH:MM:SS format and stores it in the variable current_time. echo "Current Time: $current_time"
prints the current time along with a descriptive label. The sleep 1
creates a one-second refresh rate for the clock display.
As you can see, the current time is shown in the terminal.
5. Downloading a File Using Dynamic Sleep Duration
Dynamic sleep duration (duration is not fixed) can be achieved by using an exponential mechanism. In this example, a Google Chrome RPM package file will be downloaded using dynamic sleep duration that repeatedly attempts to download a file from a remote server until the download is successful. The sleep duration will increase exponentially between each attempt to avoid overwhelming the server. Now, check out the entire bash script:
#!/bin/bash
attempts=0
url="https://dl.google.com/linux/direct/google-chrome-stable_current_x86_64.rpm"
while ! wget "$url"; do
attempts=$((attempts + 1))
sleep_duration=$((2 ** attempts * 5)) # Exponentially increasing sleep duration
sleep $sleep_duration
done
echo "Download successful!"
A variable named “url” is initiated to specify the URL “https://dl.google.com/linux/direct/google-chrome-stable_current_x86_64.rpm” of the Google Chrome file to download. while ! wget "$url"; do
starts a while loop that runs as long as the wget command returns a non-zero exit status. attempts=$((attempts + 1))
increments the value of attempts by 1 in each iteration.
sleep_duration=$((2 ** attempts * 5))
calculates the sleep duration using an exponential increase (2^attempts) multiplied by a base value of 5 seconds. sleep $sleep_duration
introduces a sleep with the dynamically calculated duration. Once the download is complete, it prints a success message with the echo "Download successful!"
.
You can notice that the Google Chrome package file has been downloaded successfully.
6. Using “sleep” Command With Case Statement
In this example, a case statement is employed within a while loop to perform different tasks based on user input. While executing different actions, it uses the sleep
command to pause for a certain time, making the output more readable. Here’s the complete bash script:
#!/bin/bash
option=""
while [ "$option" != "exit" ]; do
echo "1. Display current date and time"
echo "2. Show calendar of the current month"
echo "3. Display files of current directory"
read -p "Enter your choice: " option
case $option in
1)
echo "Current date and time: $(date)"
sleep 2
;;
2)
echo "Current date with calendar:"
ncal
sleep 2
;;
3)
echo "Files of the current directory."
ls
sleep 2
;;
*)
echo "Invalid option. Try again."
;;
esac
done
The script initializes a variable option to an empty string to store the user’s input. while [ "$option" != "exit" ]; do
starts the while loop and it continues as long as a user types “exit” to quit the script. Then it uses a case
statement to perform different actions based on the value of the option. If the user enters “1”, it displays the current date and time using the date
command and sleeps for 2 seconds.
When the user enters “2”, it displays the current month’s calendar using the ncal command and sleeps for 2 seconds. If the user enters “3”, it displays the files in the current directory using the ls command and sleeps for 2 seconds. Finally, if the user enters an option other than 1, 2, or 3, it prints “Invalid option. Try again.”.
As I entered 1, it showed the current date and time using the date command. Then I typed “exit”, it printed an “Invalid option. Try again.” and ended the while loop.
Practice Tasks on Using “sleep” Command in Bash “while” Loop
To enhance your knowledge of how to use the sleep command in bash while loop, try the following:
- Create a script that prompts the user for input and continues to ask until they enter a number greater than 10 taking 2-second pause between prompts.
- Write a script that monitors a specific file and checks if it exists every 5 seconds.
- Make a script that simulates connecting to a remote server. It should attempt the connection 3 times with a 10-second delay between attempts, printing success or failure messages accordingly.
- Generate a script that monitors a specific background process (identified by PID) and reports its status (running/terminated) every 30 seconds.
Conclusion
To wrap up, I have explained the basics of the sleep command. Moreover, I have provided 6 examples of how to use the sleep command in bash while loop. Improve your understanding by completing the practice tasks. Good luck!
People Also Ask
How do I put Bash to sleep?
To put Bash to sleep, use sleep N
syntax. This syntax pauses a script for N seconds, where N is either a positive integer or a floating point number. Here’s an example bash script:
#!/bin/bash
while true; do
echo "Linuxsimply.com"
sleep 1 # Adjust the sleep duration as needed
done
This script runs the “Linuxsimply.com” indefinitely and takes a 1-second break before moving to the next iteration due to the sleep 1
command.
How to wait for 10 seconds in shell script?
To wait for 10 seconds in a shell script, you can use the sleep
command. The sleep 10 syntax will pause the script’s execution for 10 seconds.
Can I customize the sleep interval in a Bash while loop?
Yes. You can customize the sleep interval in a bash while loop. The sleep
command takes a duration as an argument, so you can specify the exact number of seconds you want the script to pause.
How to use sleep in Linux?
The sleep
command on Linux is used to stop the execution of scripts or shells for a specific time. The syntax for the sleep command in Linux is as follows:
sleep <duration>
Where <duration> is the time to sleep. It can be specified in seconds (s), minutes (m), hours (h), or a combination thereof.
Are there alternatives to using the sleep command for precise timing in a loop?
Yes. There are alternatives to using the sleep
command for precise timing in a loop. You can use usleep
command that introduces a delay in microseconds instead of seconds. Also, you can use the time.sleep()
which is a built-in time module.
Related Articles
- “while true” Loop in Bash [4 Cases]
- 8 Examples of “while” Loop in Bash
- How to Read File Line Using Bash “while” Loop [8 Cases]
- How to Increment Number Using Bash “while” Loop [8 Methods]
- One Line Infinite “while” Loop in Bash [4 Examples]
- How to Use Bash Continue with “while” Loop [7 Examples]
- Exit “while” Loop Using “break” Statement in Bash [11 Examples]
- How to Use Bash One Line “while” Loop [8 Examples]
<< Go Back to “while” Loop in Bash | Loops in Bash | Bash Scripting Tutorial