FUNDAMENTALS A Complete Guide for Beginners
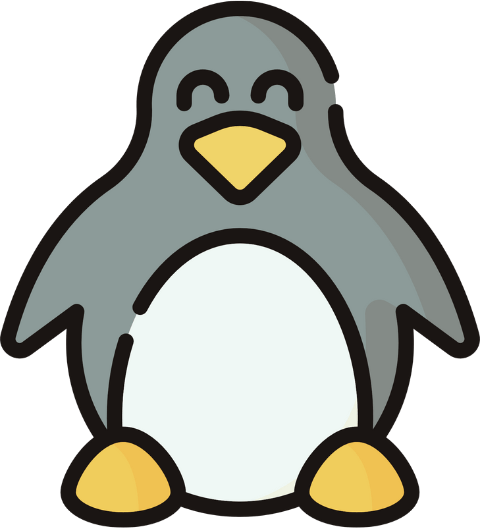
The Bash scripting language is renowned for its ability to automate tasks and manage system processes. One of its important features is the case statement, which is a powerful conditional construct that facilitates decision-making in scripts. It can be used to control multiple operations within a single code, which is not possible with if-else or else-if structures. This is particularly beneficial when a variable has a value and one wishes to perform various actions depending on its value.
This article provides an in-depth overview of the case statement and 5 examples of how to use it in a bash script.
What is Case Statement in Bash?
Case statement in bash is a conditional structure that assists in decision-making based on patterns. These patterns can be alphabets, numeric values, special characters, and more. This statement enables you to compare a variable’s value with multiple patterns and run different code blocks based on the result. It is analogous to the switch statement in other programming languages. Here’s the basic syntax of the case statement:
case $variable in
pattern1)
# Statement to execute if variable matches pattern1
;;
pattern2)
# Statement to execute if variable matches pattern2
;;
*)
# Statement to execute if no patterns match
;;
esac
- case: Indicates the start of the case statement.
- $variable: Specifies the variable or expression to be evaluated.
- in: Differentiates the expression from the patterns.
- pattern1, pattern2, …patternN: These are the patterns used to match against the expression.
- Code Blocks (;;): Each pattern has a code block between
)
and;;
which includes the statements. If the value of the expression matches the pattern, the corresponding statement will be executed. - Wildcard Pattern (*): This is a wildcard that matches any value that isn’t matched by any of the previous patterns. If none of the patterns match, the code block after
*)
will be executed. - esac: Denotes the end of the case statement.
5 Examples to Use Case Statement in Bash
The case conditional statement is a useful tool in bash scripting, allowing for handling a wide range of conditions in a structured and understandable manner. This makes the scripts more handy and readable to the users. In this part, I will explain 5 examples demonstrating the use of the case statement within bash.
1. Choose Linux Distribution Using Bash Case Statement
To choose a particular Linux distribution from multiple distros, you can use case statements. Because the case statement can execute multiple expressions by matching the pattern with the variable values. In this example, I will show you how to select a specific distro from various Linux distributions. Here, I will use shopt -s nocasematch
command in the bash script to perform case-insensitive matching. Check the following script to see the entire process:
#!/bin/bash
shopt -s nocasematch
read -p "Choose a Linux Distro: " Distro
case $Distro in
Ubuntu)
echo "You chose Ubuntu."
;;
Red-hat)
echo "You chose Red-hat."
;;
Arch-Linux)
echo "You chose Arch Linux."
;;
*)
echo "Unknown Distro."
;;
esac
The script prompts the user to enter a Linux distribution name using the read -p
command. The entered Linux distribution is then evaluated using a case statement. If you enter “Ubuntu,” the script echoes “You chose Ubuntu.” using the echo command. If you enter “Red-hat”, the script executes “You chose Red Hat.”. Similarly, the message “You chose Arch Linux.” is executed. When the entered value doesn’t match any of the specified cases, the script prints “Unknown Distro.”.
In this picture, you can see that when I entered ubuntu and red-het, it showed “You chose Ubuntu” and “You chose Red-hat”. However, if I entered fedora, it showed an “Unknown Distro” as this Linux distribution is not mentioned in any case.
2. Check File Type Using Case Statement in Bash
Use case statements to check whether a file is a bash script, text, png image, or none of these. This statement checks the type of a file based on file extensions. So, first, extract the file extension using this syntax ${Filename##*.}
. This syntax removes everything before the last dot in the filename and checks only the file extension. Let’s look at the following bash script to see how it works to find a file type:
#!/bin/bash
read -p "Enter a Filename: " Filename
File_Extension=${Filename##*.} # extract the file extension
case "$File_Extension" in
sh)
echo "It’s a Bash Script File"
;;
png)
echo "It’s a PNG Image File"
;;
txt)
echo "It’s a Text File"
;;
*)
echo "Unknown File"
;;
esac
After entering the file extension into the case statement, it will print “It’s a Bash Script File” if the extension is “sh”. If the extension is “png” it will print “It’s a PNG Image File”. Similarly, it executes “It’s a Text File” if the extension is “txt”. For any other extension, it echoes “unknown File”.
As you can see it shows “It’s a text File” after entering file.txt because the file extension is txt. When I put hello.png, it shows “It’s a PNG Image File”. However, if I put file.pdf, it displays “Unknown File” because the extension is not specified in any case.
3. String Comparison With Pipe Operator and Case Statement
Using the pipe operator |
in the case statement, multiple strings can be declared within a single line. Let’s consider an example in which the script determines the climatic conditions associated with a particular country or area. The script prompts the user to select a country and, based on the country selected, outputs the climatic conditions in that region. Here’s the bash script:
#!/bin/bash
shopt -s nocasematch
echo -n "Enter a country name: "
read Location
echo -n "The typical climate of $Location is "
case $Location in
Canada | "United States" | Russia)
echo "Temperate"
;;
Norway | Sweden | Iceland)
echo "Subarctic"
;;
India | Thailand | Vietnam)
echo "Tropical Monsoon"
;;
Japan | "South Korea" | China)
echo "Humid Continental"
;;
*)
echo "Unknown"
;;
esac
Here, the script prompts the user to enter a country name using echo -n
and read
, and the input is stored in the variable Location. If the entered country is Canada, United States, or Russia, it executes “Temperate”. When the country is Norway, Sweden, or Iceland, it prints “Subarctic”. Similarly, it prints other outputs based on the country name. Finally, the *)
case is triggered for unknown locations, and it echoes “Unknown.”
After running the script with ./climate.sh
, and entering india, the script showed “Tropical Monsoon” climate. Then I entered china, and the script said “Humid Continental” climate. And when I entered sweden, it displayed “Subarctic” climate.
4. Check Service Status With For Loop and If Else in Bash Case Statement
To check the service status of “apache2”, “mysql”, and “sshd”, you can use for loop and if-else within the case statement. For that, you can generate a bash script using a for loop that will iterate through each service in the services array, and if-else will be used to evaluate the conditions. Now, check the detailed process through the following bash script:
#!/bin/bash
services=("apache2" "mysql" "sshd") # List of system services
for service in "${services[@]}"; do
case $service in
"apache2")
if systemctl is-active --quiet $service; then
echo "Apache2 is running."
else
echo "Starting Apache2..."
sudo systemctl start $service
if [ $? -eq 0 ]; then
echo "Apache2 started successfully."
else
echo "Failed to start $service: Unit $service not found."
fi
fi
;;
"mysql")
if systemctl is-active --quiet $service; then
echo "MySQL is running."
else
echo "Starting MySQL..."
sudo systemctl start $service
if [ $? -eq 0 ]; then
echo "MySQL started successfully."
else
echo "Failed to start $service: Unit $service not found."
fi
fi
;;
"sshd")
if systemctl is-active --quiet $service; then
echo "SSH server is running."
else
echo "Starting SSH server..."
sudo systemctl start $service
if [ $? -eq 0 ]; then
echo "SSH server started successfully."
else
echo "Failed to start $service: Unit $service not found."
fi
fi
;;
*)
echo "Unknown service: $service"
;;
esac
done
In this script, the case statement handles each service individually based on its name. If the current service is “apache2,” it checks if Apache2 is running using systemctl is-active
. If not, it attempts to start the service using systemctl start
. Then it checks the exit status with [ $? -eq 0 ]
syntax. If $?
equals 0, that means the Apache2 is started. Then it echoes the if block message. If exit status 1, it prints the else block message. Similarly, it checks and starts the MySQL service and SSH server service. The *) pattern handles unknown services and prints a message indicating that the service is unknown.
In this image, you can see that while running the service.sh
script, it checks the service status of Apache2 and tries to start the service. But this service is not found in my Linux system, so, it prints “Failed to start $service: Unit apache2 not found.”. MySQL service is running successfully. The SSH server is also missing in my system, so, it displays the else block code “Failed to start $service: Unit sshd not found.”.
5. Compare Numbers Using Ternary Operator and Case Statement
To compare numbers within case statements, you can use the ternary operator ? :
to check different conditions. Here, the script uses an arithmetic expression $((...))
to evaluate a number and determine its range. Let’s see the full script of the number comparison:
#!/bin/bash
read -p "Enter a number: " number # Prompt the user to enter a number
case $(( number >= 0 && number <= 50 ? 1 :
number > 50 && number <= 100 ? 2 :
number > 100 && number <= 150 ? 3 : 0 )) in
(1)
echo "Number is >= 0 and <= 50";;
(2)
echo "Number is > 50 and <= 100";;
(3)
echo "Number is > 100 and <= 150";;
(0)
echo "Number is out of range";;
esac
Depending on the range of the entered number, the script outputs a message indicating the corresponding range. If the number is between 0 and 50 (inclusive), it falls into the range 1. When the number is between 50 and 100 (inclusive), it falls into the range 2. If the number is between 100 and 150 (inclusive), it falls into the range 3. When the number is outside these ranges, it falls into the range 0, indicating that it is out of the specified ranges.
Since the input number 100 is greater than 50, it falls between range 2. When the entered number is 25 which is less than 50, so it falls into the range 1. In the same way, number 130 falls in range 3.
Conclusion
In summary, I have provided a comprehensive overview of the case conditional statement in bash. Additionally, I have provided 5 practical examples of case statements to assist you in comprehending the concept better. Now you know how to compare numbers and strings using case statements. Moreover, you can check service status and file type with this statement. Within the case construct, you can also implement for loop and if-else statements.
People Also Ask
What is the purpose of the case statement in Bash?
The main purpose of the case statement in bash is to provide a logical structure for conditional branching based on a variable or expression value. The case statement makes it easier to handle complex conditional logic that requires checking a variable against several values.
Case Statements vs. Nested If Statements?
The case statement replaces nested if statements with more readable and organized statements. While nested if statements can achieve similar conditional branching, they often result in convoluted code, especially when dealing with multiple options. The case statement offers a more concise and readable approach, particularly for complex scenarios.
What is the use of * pattern in case statements?
The *
pattern is used as a wildcard that matches any values that are not defined by any of the proceedings patterns. It allows you to specify the code to run in case none of the other patterns match the expression’s value.
Is the case statement case-sensitive or case-insensitive?
By default, the case statement is case-sensitive. However, you can use the shopt -s nocasematch
code before starting the case statement to make it case-insensitive.
Can the case statement be nested within another case statement?
Yes, definitely. It is possible to create nested case statements by including one case statement within another case statement to address more intricate situations. Each nested statement provides a new collection of patterns and related code blocks.
How does the case statement differ from if-elif-else statements?
The case
statement is a concise and easy-to-understand alternative to the if-elif-else
statements, particularly when dealing with multiple conditions. The case statement supports pattern matching and eliminates redundant statements to provide a clear structure for handling different situations.
What is Bash case statement?
In Bash, the case statement is a conditional construct used to perform decision-making tasks by matching different patterns (alphabets, numeric values, special characters).
Related Articles
<< Go Back to Bash Conditional Statements | Bash Scripting Tutorial