FUNDAMENTALS A Complete Guide for Beginners
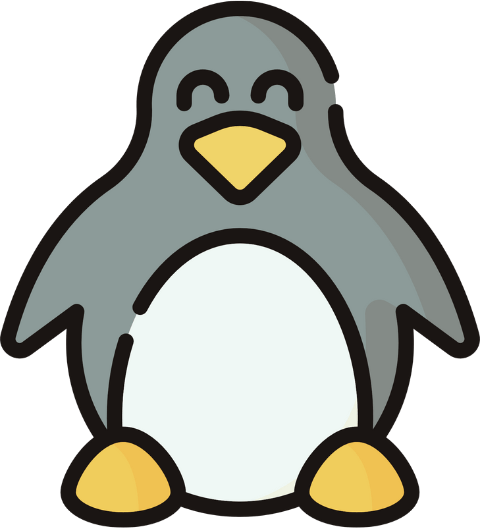
Bash logical operators are essential components of shell scripting that enable users to create conditional expressions and make decisions based on the evaluation of conditions. These operators can be combined with various conditions to control the flow of Bash scripts and perform different actions based on specific criteria.
In this article, I will discuss the role of logical operators and their functionality in Bash script. So let’s move on!
Basics of Logical Operators in Bash
In Bash scripting, logical operators can assess conditions and construct intricate expressions by merging two or more conditions. These operators enable users to determine whether a condition or a set of conditions holds true, granting the ability to manage the script’s execution flow effectively. In the following section, I will give you an overall demonstration of the types of logical operators in bash script.
Types of Logical Operators in Bash
In Bash scripting, logical operators are fundamental for making decisions and controlling the flow of commands based on conditions. They evaluate conditions to be either true or false and make a decision based on the result of the condition. The three primary logical operators are AND, OR, and NOT operators. Let’s have a syntax of each operator below.
- AND Operator: The AND operator in Bash produces a true outcome solely when both assessed conditions are true. The syntax for the AND operator in Bash scripting is
&&
. - OR Operator: The OR operator evaluates to true if at least one of the conditions it connects evaluates to true. The syntax for the OR operator in Bash scripting is
||
. - NOT Operator: The NOT operator in Bash scripting reverses the outcome of a condition. It is denoted by the
!
syntax and is used to negate the result of a given condition.
3 Cases of Using Logical Operators in Bash Scripting
In the following section of this article, I will show you the practical cases of bash logical operators to give a sense of their functionality.
Case 01: Combining Conditions With Logical Operators
Combining logical operators can help check multiple conditions simultaneously. Following is an example where two logical AND operators and an echo command are used to display the output of the command.
#!/bin/bash
grade=5
name="Bob"
[[ $grade -ge 4 && $name == "Bob" ]] && echo "Student name is $name and Grade is Greater than 4"
This Bash script begins by assigning the variables grade to 5 and name to Bob. It then uses a conditional statement within double square brackets ‘[[‘ to check two conditions simultaneously. The first condition, $grade -ge 4
evaluates whether the grade variable is greater than or equal to 4. The second condition, $name == "Bob"
checks if the name variable equals Bob.
The &&
operator ensures that both conditions must be true for the subsequent command to execute. If both conditions are met, it echoes the message “Student name is Bob and Grade is Greater than 4” to the console.
Upon execution, the code displays “Student name is Bob and Grade is Greater than 4”.
Case 02: Logical Operators in If-Else Statements
Logical operators can easily be implemented within if-else statement to perform conditional checking. Here’s such an example:
#!/bin/bash
# Check if the number of arguments is less than 3
if [ $# -lt 3 ]; then
echo "Usage: $0 <age> <name> <is_student>"
exit 1
fi
age=$1
name=$2
is_student=$3
if [[ $age -gt 18 && $name == "John" && $is_student == true ]]; then
echo "John is older than 18 and is a student."
else
echo "John does not meet all the specified conditions."
fi
This Bash script starts by checking if the number of command-line arguments provided is less than 3 using the $#
variable, which holds the number of arguments. If there are fewer than 3 arguments, it displays a usage message and exits. Then, it assigns the variables age, name, and is_student with the positional parameters $1, $2 and $3 respectively.
Next, it uses an if statement with double square brackets [[ … ]] to check multiple conditions. It verifies whether the age stored in the age variable is greater than 18, whether the name variable contains the string “John”, and whether the is_student variable is set to true. If all these conditions are met, it prints the message “John is older than 18 and is a student.” Otherwise, it displays “John does not meet all the specified conditions.”
Upon execution, the bash script returns “John is older than 18 and is a student” to the command line.
Case 03: Combining Multiple Boolean Operators in a Bash Script
Navigate through the script below to combine multiple Boolean (aka logical operators) operators &&, || and ! in Bash:
#!/bin/bash
age=25
name="Alice"
is_student=true
if [[ $age -ge 18 && $name != "Bob" || ($is_student == true && $age -le 30) ]]; then
echo "Alice is eligible: older than 18, not named Bob, and either a student or under 30 years old."
else
echo "Alice does not meet the specified conditions."
fi
This Bash script initializes variables for age, name, and student status. It then uses an if statement with logical operators to check if Alice’s age is greater than or equal to 18, her name is not Bob, and she is either a student or under 30 years old. If all these conditions are met, it prints that Alice is eligible; otherwise, it states that she does not meet the specified conditions.
As the name and age variable contain Alice and 25 respectively, the code returns “Alice is eligible: older than 18, not named Bob, and either a student or under 30 years old.”
5 Practical Examples of Using Logical Operators in Bash Scripting
Previously I showed 3 different scenarios to incorporate the logical operators in bash script. However, the following section will give 5 different examples by using the logical operator.
Example 01: Check if a Number is Within a Specific Range
Logical operators help in creating complex expressions by combining conditions. For instance, the objective of the following Bash script is to assess whether a given numerical value, provided as a command-line argument, falls within the specified range of 10 to 20.
#!/bin/bash
value=$1
if [[ $value -gt 10 && $value -lt 20 ]]; then
echo "The value is within the range of 10 to 20."
else
echo "The value is not within the range of 10 to 20."
fi
This Bash script takes a single command-line argument value and checks if it falls within the range of 10 to 20 using an if statement with the &&
logical operator. If the value is greater than 10 AND less than 20, it prints “The value is within the range of 10 to 20.” Otherwise, it prints “The value is not within the range of 10 to 20.”
Upon giving 34 for as a command line argument to number_range.sh bash file, the code returns “The value is not within the range of 10 to 20.” On the other hand, number_range.sh with given 15 as argument displays “The value is within the range of 10 to 20.”
Example 02: Check if a User is Either “root” or “admin” Using Boolean Operators
The OR operator is used to check if any of the condition is true within a conditional statement. For example, here’s a Bash script that determines whether a user, represented by the variable username, is either root or admin and ascertain if the user has administrative privileges on the system.
#!/bin/bash
# Define the username you want to check
username="$1"
# Check if the user is either the root user or has sudo privileges
if [ "$UID" -eq 0 ] || sudo -lU "$username" | grep -q "(ALL : ALL)"; then
echo "$username has sudo privileges, hence is either root or admin user"
else
echo "The username '$username' is neither root nor has sudo privileges."
fi
This Bash script begins by capturing a username provided as an argument when running the script. It proceeds to check two conditions using the if statement. First, it examines whether the effective user ID (UID) of the user executing the script is equal to 0, which signifies the root user.
Second, it employs the sudo command to evaluate whether the specified username has sudo privileges. The grep -q
part in this condition silences any output and checks if the pattern (ALL : ALL)
exists in the sudo configuration, indicating sudo privileges. If either condition is true, the script prints a message declaring that the user possesses sudo privileges and is thus either the root user or an admin user. Conversely, if neither condition is met, it communicates that the username is neither the root user nor has sudo privileges.
In the image depicted above, John is a newly created user who does not have the sudo privilege. By incorporating John as the positional argument, the command line says John does not have any sudo privilege. On the contrary, miran is the current user and has the sudo privilege. Therefore the script validates miran’s authority upon execution of the script accordingly.
Example 03: Invert a Condition Using the “NOT” Operator
The NOT (!) operator reverses the condition within an if statement in Bash. Check out the following script to see the example of condition inversion:
#!/bin/bash
# Define a new filename
file_to_check=$1
# Check if the file exists
if [[ ! -e "$file_to_check" ]]; then
echo "The file '$file_to_check' does not exist."
else
echo "The file '$file_to_check' exists."
fi
This Bash script begins by defining a variable named file_to_check and assigns it the value test_file.txt. It then uses an if statement to check if the file specified by file_to_check exists in the current directory, utilizing the -e
test operator within double square brackets. If the file does not exist, it outputs a message indicating that the file is not present. Conversely, if the file exists, it outputs a message confirming its existence.
As the test_file.txt already exists in the current directory, it is obvious the code will return “The file ‘test_file.txt’ exists” to the command line.
Example 04: Using of “NOT” and “AND” Logical Operators in a Single Line
To combine the NOT and AND operators in a single line, go through the script below:
#!/bin/bash
# Prompt the user to enter their age and whether they have a voting disqualification
echo "Welcome to the Voting Eligibility Checker!"
read -p "Please enter your age: " age
read -p "Do you have any voting disqualifications? (yes or no): " disqualifications
# Check if the user is old enough and not disqualified
if [ $age -ge 18 ] && [ "$disqualifications" != "yes" ]; then
echo "Congratulations! You are eligible to vote."
else
echo "Sorry, you are not eligible to vote at the moment."
fi
The given bash code starts by prompting the user to enter their age and whether they have any voting disqualifications. It uses the read
command to capture these inputs. Then, it uses an if statement to check if the user’s age is greater than or equal to 18 and if their response to disqualifications is not yes. If both conditions are met, it prints “Congratulations! You are eligible to vote.” Otherwise, it prints “Sorry, you are not eligible to vote at the moment.”
As you can see from the image given above, I inserted 25 as my age and gave a no response regarding my voting disqualifications. Thus the code gives the output Congratulations! You are eligible to vote, based on the conditions set.
Example 05: Using of “OR” and “NOT” Operator in a Single Line
Navigate through the following script to use the NOT and OR operators together in a single line:
#!/bin/bash
echo "Give your correct username and password to get a greeting"
# Set the correct username and password
correct_username="miran"
correct_password="1234"
# Prompt the user to enter their credentials
read -p "Enter your username: " username
read -s -p "Enter your password: " password
# Check if the entered credentials are correct
if [ "$username" != "$correct_username" ] || [ "$password" != "$correct_password" ]; then
echo -e "\nLogin failed. Invalid username or password."
else
echo -e "\nLogin successful! Welcome, $username."
fi
The code begins by printing a message asking the user to provide their correct username and password for a greeting. The correct username and password are defined as “miran” and 1234 in the script. The script uses the read
to prompt the user to enter their username and password. The -s
flag with read is used for the password input to keep it hidden as the user types.
It then uses an if statement to check if the entered username and password match the correct ones. If either the username or password is incorrect, it prints “Login failed. Invalid username or password.” Otherwise, if both are correct, it prints “Login successful! Welcome, [username].”
As the user correctly provides the username and password, the code returns “Login successful! Welcome, miran” on the command line.
Conclusion
In conclusion, Logical operators in bash scripting can play a vital role in assigning multiple conditions and making decisions based on them. In this article, I have demonstrated some different examples of logical operators in bash to convey its functionality. If you have any questions or queries related to this, feel free to comment below. I will get back to you soon. Till then, Happy Coding!
People Also Ask
What are logical operators in Bash?
Logical operators in Bash include && (AND), || (OR), and ! (NOT). They are used to perform logical comparisons and control the flow of commands based on conditions.
What is the += operator in Bash?
In Bash, the += operator is a compound assignment operator that allows you to add (concatenate) a string or value to the end of an existing variable’s value. It’s commonly used for building or modifying strings dynamically in scripts.
What is binary operator in Bash?
A binary operator in Bash is an operator that works on two operands or values. It requires two values to perform an operation, such as addition, subtraction, comparison, or logical operations. Examples of binary operators in Bash include + for addition, – for subtraction, == for equality comparison, and && for logical AND operations.
What is the use of && in Bash?
In Bash, && is a logical operator used to execute the command on its right only if the command on its left succeeds (returns a zero exit status).
Related Articles
- Boolean Variables “True False” and Logical Operators in Bash
- Usage of Logical AND (&&) Operator in Bash Scripting
- Usage of OR Operator in Bash Scripting [2 Cases]
- Usages of NOT (!) Operator in Bash Scripting [4 Examples]
<< Go Back to Bash Operator | Bash Scripting Tutorial