FUNDAMENTALS A Complete Guide for Beginners
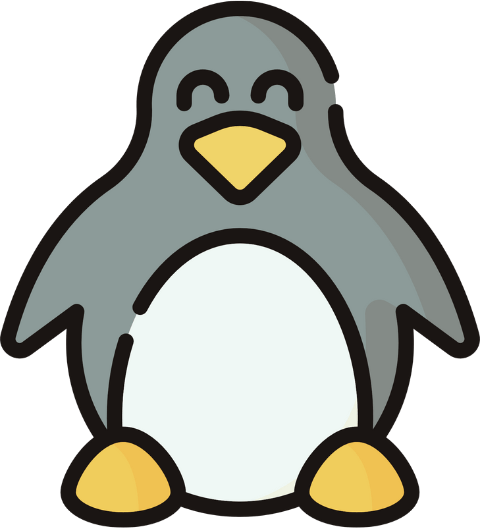
Arithmetic operators are operators that facilitate the evaluation of a desired mathematical expression. Bash shell supports a lot of arithmetic operators. One can perform simple addition, and subtraction and evaluate complex arithmetic expressions using these operators. In this article, I will talk about different arithmetic operators with their execution in Bash.
Different Arithmetic Operators in Bash
Bash offers various operators to perform arithmetic operations. These operators have different levels of precedence.
For example, the expression $((3*5+6)/7-1))
is evaluated in accordance with the rules of precedence. (3*5+6)
is computed first as it is enclosed by parenthesis. Within the parentheses, the multiplication operation 3*5
is computed first, resulting in 15. Then, the addition operation 15 + 6
is performed, yielding 21. Subsequently, division 21 / 7
is evaluated before subtraction, resulting in 3. Finally, the subtraction 3 - 1
is computed, yielding the final result of 2. Below is a list of operators sorted by increasing order of precedence:
Operators | Operation | Example | Output | Precedence |
---|---|---|---|---|
+ | Addition | $((10+5)) | 15 | Lowest |
– | Subtraction | $((10-5)) | 5 | Same as Addition |
* | Multiplication | $((10*5)) | 50 | Higher than addition lower than exponential |
/ | Division | $((10/5)) | 2 | Higher than addition lower than exponential |
% | Modulus (remainder) | $((13/5)) | 3 | Higher than addition lower than exponential |
** | Exponentiation | $((2**3)) | 8 | Highest |
Now, I am going to discuss each of the arithmetic operators in detail:
Addition
The plus sign (+) is used for addition operation in Bash. For example,
total=$((5+10))
echo "Result of summation =$total"
Subtraction
The minus sign (–) is used to perform subtraction. Such as,
diff=$((15-10))
echo "Result of subtraction=$diff"
Multiplication
The asterisk sign (*) is used to multiply two numbers in Bash. For instance,
multiply=$((5*10))
echo "Result of multiplication=$multiply"
Division
Forward slash (/) is used to divide one number by another:
division=$((10/5))
echo "Result of multiplication=$division"
Modulus (Remainder)
One can calculate the modulus or remainder using the percentage sign (%). For example,
remainder=$((17%5))
echo "Remainder of the division=$remainder"
Exponential
To calculate the exponential of a number raised to another number, use the double asterisk sign (**):
expo=$((2**3))
echo "Exponential of 2 to the power 3=$expo"
Assignment Operators for Arithmetic Calculation in Bash
Apart from arithmetic operators, there are a few assignment operators in Bash. These operators are used to assign values to variables. They take a value on the right side of the operator and change the variable on the left side based on the given value and the operator. These are also useful to perform arithmetic operations in some cases.
All the assignment operators have equal levels of precedence. The following list contains assignment operators and their functions on numerical values:
Operators | Operation | Example | Output/Value |
---|---|---|---|
+= | Increment by constant | x=20
(( x += 10 )) |
x=30 |
-= | Decrement by constant | x=20
(( x -= 10 )) |
x=10 |
*= | Multiply by constant | x=20
(( x *= 10 )) |
x=200 |
/= | Divide by Constant | x=20
(( x /= 10 )) |
x=2 |
%= | Remainder after Dividing by constant | x=19
(( x %= 6 )) |
x=1 |
Let’s discuss each of these assignment operators in short:
Increment by Constant (+=)
To increase a variable by a constant use the increment by constant +=
operator. For instance,
x=5
(( x += 15 ))
echo "After Increment, x=$x"
Decrement by Constant (-=)
To decrease a variable by a constant use the -=
operator:
x=20
(( x -= 15 ))
echo "After decrement, x=$x"
Multiply by Constant (*=)
To multiply a variable with a constant use the *=
operator:
x=10
(( x *= 2 ))
echo "After multiplying by 2, x=$x"
Divide by Constant (/=)
/=
replaces the variable with the result of division after dividing it by a constant:
x=20
(( x /= 5 ))
echo "After decrement, x=$x"
Remainder After Dividing by Constant (%=)
%=
operator replaces the variable with the remainder after dividing it by a constant. For example,
x=20
(( x %= 6 ))
echo "Remainder after division, x=$x"
Practical Application of Arithmetic Operators in Bash
Bash arithmetic operators have various real-life usages. In this tutorial, I will show two basic examples involving the calculation with multiple operators. These will help you to understand how arithmetic operators can be used in practical cases:
Calculate the Perimeter of a Rectangular
To calculate the perimeter of a rectangle, one can use the addition and multiplication operators simultaneously.
#!/bin/bash
# Get user input for rectangle dimensions
read -p "Enter the length of the rectangle: " length
read -p "Enter the width of the rectangle: " width
# Calculate perimeter
perimeter=$((2 * (length + width)))
The program adds the length and width using the plus +
arithmetic operator. Then the summation is multiplied by 2 using the multiplication operator *
to get the perimeter of the rectangle.
Here the length and width of the rectangle are 10 and 5 respectively. The program calculates the perimeter of the rectangle 30 using the addition and multiplication operator.
Check Odd or Even Number
Divide a number by 2 and compare the remainder with zero to determine whether a number is odd or even. To check it in Bash, the modulo (%) operator is quite useful.
#!/bin/bash
# Check if a number is odd or even
read -p "Enter the number: " number
if ((number % 2 == 0)); then
echo "$number is even."
else
echo "$number is odd."
fi
The %
operator is used to find the remainder when the variable number is divided by 2.
The number provided by the user is 9. The program determines it as an odd number by successfully using the modulo (%) operator in Bash.
Conclusion
In conclusion, arithmetic operators are for performing mathematical calculations. Bash users should familiarize themselves with arithmetic operators to become more efficient scriptwriters.
People Also Ask
What are the ways to perform arithmetic calculations in Bash?
There are multiple ways to perform arithmetic calculations in Bash.
- Arithmetic expansion:
$(( ))
allows users to evaluate arithmetic expressions like the
following.
a=5
b=3
echo $((a + b))
# Output: Result: 8
- expr command: The expr command evaluates expressions and performs arithmetic
operations.
echo $(expr 5 + 3)
Output: Result: 8
- let command: The let command is also useful for evaluating a mathematical
expression in Bash. To be specific let '5 + 3'
perform the addition of 5 and 3 and result in 8.
How to evaluate arithmetic expressions in the if statement?
To evaluate arithmetic expression within an if statement one can use double parenthesis. For instance, ((13 % 2 == 0))
calculates the remainder using the modulo (%) operator when 13 is divided by 2 and compares it with zero.
How to perform floating point calculations in Bash?
To perform floating point calculations pass the expression to the bc command. For instance, echo 2.2*3.5 | bc
performs the multiplication of two floating point numbers 2.2 and 3.5.
How to fix “invalid arithmetic operator” in bash?
In Bash,‘invalid arithmetic operator’ error occurs due to many reasons. To fix the error first identify the reason behind it. In most cases, it occurs due to the intention of calculating floating point using arithmetic expansion. Arithmetic expansion can’t handle floating point arithmetics. For instance, echo $((2.5+5))
will show ‘invalid arithmetic operator’ because of the floating point number 2.5. Use the bc command to perform this type of calculation.
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Calculate in Bash [3 Practical Examples]
- How to Sum Up Numbers in Bash [Explained With Examples]
- How to Divide Two Numbers in Bash [8 Easy Ways]
- Division of Floating Point Numbers in Bash
- How to Use “Mod” Operator in Bash [5 Basic Usage]
- How to Format Number in Bash [6 Cases]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
<< Go Back to Bash Operator | Bash Scripting Tutorial