FUNDAMENTALS A Complete Guide for Beginners
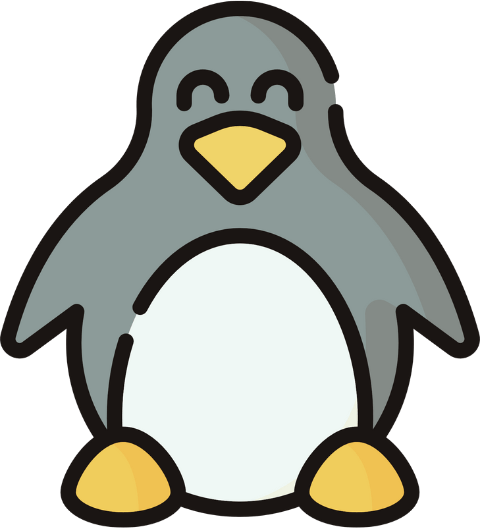
To divide two numbers in Bash use the following methods:
- Divide two integer variables x and y using expr command:
$(expr $x / $y)
- Divide two variables and capture result of division using let command:
let result=$x/$y
- Divide two integer numbers using arithmetic expansion:
$(($x / $y))
- Divide two numbers up to desired precision using printf:
printf "%.<precision>f" $((10**<precision>*$x/$y))e-<precision>
- Divide floating point numbers using bc command:
result= "scale=<precision>; $x / $y" | bc
- Divide two numbers using awk command:
$(awk "BEGIN {print $x / $y}")
- Use Python in Bash to divide numbers:
$(python3 -c "print($x / $y)")
- Perl command for division in Bash:
$(perl -e "print $x/$y")
Now, I am going to discuss each of the above methods in detail. Stay tuned.
1. Divide Two Variables Using the “expr” command
The expr
command can be used to divide two integer variables. Here’s an example:
#!/bin/bash
# Define variables
x=10
y=2
# Use expr to perform division
result=$(expr $x / $y)
# Print the result
echo "Result of division: $result"
This Bash script defines two variables x and y, with values 10 and 2 respectively. It then utilizes the expr command to perform an integer division of x by y.
2. Divide Two Integer Numbers Using “let” Command
The let
command can perform division on the shell variable as well as the variables within the context of the command itself. Let’s see how it performs division on shell variables:
#!/bin/bash
x=100
y=25
let result=$x/$y
# Print the result
echo "Result of division: $result"
Here, x=100 and y=25. The let command divides x by y and stores the result of the division in the result variable.
Additionally, variables x and y can be set in a single line using the let command like below:
3. Division of Two Numbers Using Arithmetic Expansion
Arithmetic expansion “$((expression))” can handle arithmetic operations, including division. To divide number a by b, use the expression $((a/b))
. Here’s a simple and clear example:
#!/bin/bash
x=100
y=25
echo "Result of division: $(($x / $y))"
The script initializes variables x and y with values 100 and 25, respectively. It then uses arithmetic expansion to calculate and print the result of dividing x by y.
Arithmetic expansion provides the integer portion of the result only even if the result of division contains a fraction part. For instance, when 5 is divided by 2, it returns 2, not 2.5.
4. Calculating Division Up to Fixed Decimal Points Using “printf” Command
The printf
command can be used to get around the limitations of arithmetic expansion. For example, if you divide 5 by 2, you won’t get the whole result of 2.5 using the arithmetic expansion only. However, printf with format specifier is capable of capturing the result of a division up to certain decimals.
The general syntax is:printf "%.<precision>f\n" $((10**<precision> * <numerator>/<denominator>))e-<precision>
Let me explain the syntax using the following code:
printf "%.2f\n" $((10**2*5/2))e-2
$((10**2*5/2)) performs arithmetic expansion. First, 10 raised to the power of 2, which is 100. Then 100 is multiplied by 5, yielding 500. Finally, 500 is divided by 2, resulting in 250.
In $((10**2*5/2))e-2, the result of arithmetic expansion is multiplied by e-2. e-2 represents multiplying the preceding value by 10 to the power of -2. In this case, it means dividing the preceding value by 100. So, 250e-2 is equivalent to 2.50.
The printf “%.2f\n” statement specifies a format for printing. %.2f specifies that the number should be formatted as a floating point with two decimal places.
5. Division of Floating Point Numbers Using “bc” Command
If you are trying to divide two floating point numbers don’t worry. Here comes the bc command. This command can divide integers as well as floating-point numbers. For that, you need to write the expression as a string and pass it to the bc
command.
#!/bin/bash
x=100
$ y=-8.5
result= "scale=4; $x / $y" | bc
echo "Result of division: $result"
The variables x and y contain values 100 and -8.5 respectively. “scale=4; $x / $y” indicates a division operation with a precision of 4 decimal places. The string is piped to the bc command, which evaluates the expression and outputs the result.
6. Divide Two Numbers Using “awk” Command
The awk command is popular for its text-processing utility. But it also handles arithmetic calculations. To perform division using this command, write the mathematical expression as a string within the BEGIN block, such as “BEGIN {print 5 / 2}”. Let’s see an example of that:
#!/bin/bash
# Define the numbers you want to divide
a=10
b=3
# Use awk for division
result=$(awk "BEGIN {print $a / $b}")
# Print the result
echo "Result of division: $result"
The program utilizes the awk
command to perform division on two numbers, a and b. Here, a is set to 10 and b is set to 3. The awk command divides the number a by b The BEGIN block is used to provide the expression to the awk command.
7. Using Python Programming Language
One can use Python for division if it’s already installed in the system. python2 and python3 commands allow users to use Python version 2 and version 3 functionalities in Bash. Let’s see how to employ Python 3 for division in the Bash shell:
#!/bin/bash
# Define the numbers
a=10
b=2
# Perform division using Python
result=$(python3 -c "print($a / $b)")
# Print the result
echo "Result of division: $result"
The program uses Python for the division of two Bash variables a and b. The division is performed using the python3. The -c option allows to run Python utilities. The Python command, “print($a / $b)”, calculates the result of dividing a by b.
Python supports floating point numbers. So numerator and denominator of a division can be floats. Additionally, it allows users to set the precision of the result. Look at the following line of code:
python3 -c 'x=20.5; y=2.0; print("{:.2f}".format(x/y))'
# Output: 10.25
Here, "{:.2f}"
format specifier in print("{:.2f}".format(x/y)
used to display the result of x/y with two decimal places.
Python may not be installed in your system. Run the following command to install python3:
sudo apt-get install python3
8. Using “perl” Command
The perl
one-liner with -e
option allows users to perform the division of floating point numbers and customize the precision of the result as needed. Use the following command to do that:
perl -e '$x=22.0; $y=7.0; printf("%.6f\n", $x/$y)'
In this example,
"%.6f\n"
is used to format the result of division up to six decimal places. You can adjust the precision by changing the number inside the format specifier.
How to Calculate Remainder in Bash
The remainder or modulo (%) operator is used to calculate the remainder of a division in Bash. For example, $((5%2))
returns 1 because 5 / 2 is 2 with a remainder of 1. Let’s see how it works:
#!/bin/bash
# Define the numbers
a=10
b=3
# Calculate remainder
remainder=$((a % b))
# Print the result
echo "Remainder of $a divided by $b is: $remainder"
The script calculates the remainder of dividing the number a by b using the modulus operator (%). The values of a and b are defined as 10 and 3, respectively. The expression $((a % b)) computes the remainder of the division.
How to Handle Division by Zero Error in Bash
When performing division, you might not know that the denominator is 0. But in bash, if you’re dividing by 0, you’ll get a “division by 0” error.To handle this error it is wise to check the divisor variable using an if block:
#!/bin/bash
dividend=10
divisor=0
# Check if the divisor is zero before performing the division
if [ $divisor -eq 0 ]; then
echo "Error: Division by zero is not allowed."
else
result=$((dividend / divisor))
echo "Result: $result"
fi
The script begins by setting the variables dividend and divisor to the values 10 and 0, respectively.
It uses the if statement to determine if the divisor is zero. If the divisor is indeed zero, the script outputs a warning message indicating that division by zero is not allowed.
Otherwise, within the else block, the script calculates the result of dividing the dividend by the divisor using the arithmetic expansion and prints the result.
Conclusion
In conclusion, there are multiple methods available for performing division in Bash. Once you are sufficiently familiar with these methods, you can choose the one that best suits your task. I hope this article helps you understand the different approaches to division in Bash.
People Also Ask
How to round up or floor the result of division in Bash?
By default arithmetic expansion provides the floored result of a division. For example, The exact result of 7 by 3 is 2.33333…, but the arithmetic expansion $((7/3))
gives the floored result of 2.
On the other hand, to round up the result add the remainder of the division with the result of the division like the following script:
# Example: Divide 10 by 3 and round the result
#!/bin/bash
x=10
y=3
rounded_result=$(( (x / y) + (x % y > 0) ))
echo "Rounded Result: $rounded_result"
How can I Divide a specific column of numbers in Bash?
To awk command is useful for dividing a particular column of data in a file. Suppose you have a data file named data.txt with three columns of values. If you wish to divide the numbers in the third column by 1024 while keeping the rest of the columns unchanged, you can use the awk command.
#data.txt
-450 1526.64 1205.00
-60.4 1507.84 1205.36
15.89 1535.96 2281.44
17.89 1538.96 2281.40
19.89 1542.60 2282.08
21.90 1545.96 2282.32
To do so, use the following code. It processes each line in the file, extracts all fields, divides the third field by 1024, and then prints the formatted result to the terminal:
awk '{printf "%s\t%s\t%.2f\n", $1, $2, $3/1024}' data.txt
If you want to replace the original data.txt file with the modified data, you can use the following command:
awk '{printf "%s\t%s\t%.2f\n", $1, $2, $3/1024}' data.txt > temp && mv temp data.txt
How to divide the output of a command in Bash?
To divide the output of a command first capture the output in a variable using command substitution. Then use any methods of division available in Bash. Let’s say you have a file named file.txt. Now, you want to count the total number of lines in the file and divide it by 2:
lines= $(cat file | wc -l)
div=$lines/2
echo "Result: $div"
How do you divide values in two columns from different files in Bash?
To divide the values of two columns from different files, you can use a combination of commands such as paste or awk. Here’s an example assuming two files, file1.txt and file2.txt, with values arranged in a column:
#file1.txt
10.2
20
11.5
#file2.txt
9
5
3.33
Now divide the values of file1.txt by the values of file2.txt using the following code:
result=$(paste file1.txt file2.txt | awk '{printf "%.2f\n", $1/$2}')
echo "Result: $result"
This command uses paste to merge the two files side by side and then uses awk to perform the division operation.
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Calculate in Bash [3 Practical Examples]
- How to Sum Up Numbers in Bash [Explained With Examples]
- Division of Floating Point Numbers in Bash
- How to Use “Mod” Operator in Bash [5 Basic Usage]
- How to Format Number in Bash [6 Cases]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
<< Go Back to Arithmetic Operators in Bash | Bash Operator | Bash Scripting Tutorial