FUNDAMENTALS A Complete Guide for Beginners
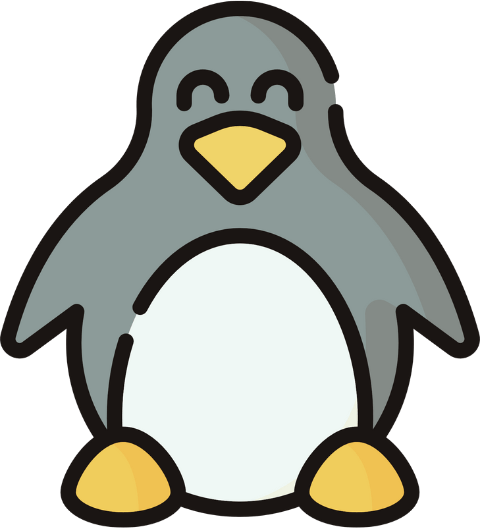
The mod or modulo operator is a binary operator that finds the remainder when a number is divided by another. The symbol of the modulus operator in Bash is “%”. When this operator is placed between two numbers within the arithmetic expansion, it finds the remainder of the first number divided by the second number. For instance, 5 % 2 returns 1 because the division of 5 by 2 has a remainder of 1. In this article, I will further explore this operator and its various practical uses.
Syntax of “Mod” Operator in Bash
Any arithmetic operation including the mod operator in Bash should follow the proper arithmetic syntax. The mod or modulo operator with arithmetic expansion to find the remainder of operand1 divided by operand2 should follow the syntax below:
echo $((operand1 % operand2))
For example, the remainder when 5 is divided by 2 is:
echo $((5%2))
Remainder when 29 is divided by 5:
echo $((29%5))
Basic Usage of “Mod” Operator in Bash
The mod operator is primarily used for finding the remainder of a division. In addition, it is used for assigning new values to variables. Now, I am going to discuss more about the practical uses of the mod operator in Bash.
1. Calculating the Remainder of a Division
The mod or modulo operator (%) is designed to calculate the remainder of a division. Arithmetic expansion in Bash can handle only integer arithmetic. Therefore, the mod operator can only find the remainder when one integer is divided by another.
To calculate the remainder of a division of two integers, use the following script:
#!/bin/bash
# Get user input for the dividend and divisor
read -p "Enter the dividend: " dividend
read -p "Enter the divisor: " divisor
# Use the modulo operator to calculate the remainder
remainder=$((dividend % divisor))
# Display the result
echo "The remainder of $dividend divided by $divisor is $remainder"
The script takes two numbers from the user and stores them in the variables “dividend” and “divisor” respectively. Using arithmetic expansion, $((dividend % divisor))
calculates the remainder of the dividend divided by the divisor and saves it in the “remainder” variable. Finally, the remainder is displayed by the echo command.
2. Checking Divisibility of a Number by Another
One can easily check the divisibility of a number using the modulo operator. For a number to be perfectly divisible, the remainder must be equal to zero. Once the modulo operator finds the remainder of a division, an if condition can check whether the remainder is zero or not.
To check the divisibility of a number by another, look at the Bash code below:
#!/bin/bash
# Get user input for the dividend and divisor
read -p "Enter the dividend: " dividend
read -p "Enter the divisor: " divisor
# Use the modulo operator to calculate the remainder
remainder=$((dividend % divisor))
# Check if the remainder is zero
if [ "$remainder" -eq 0 ]; then
echo "$dividend is divisible by $divisor"
else
echo "$dividend is not divisible by $divisor. Remainder: $remainder"
fi
$((dividend % divisor))
finds the remainder of the division using the modulo operator. Then, the program checks whether the remainder is zero using an if statement. If the remainder is zero, it prints a message indicating that the dividend is divisible by the divisor. Otherwise, it states that the dividend is not divisible by the divisor and includes the calculated remainder in the output.
In the first execution, the program checks whether 10 is divisible by 3. The result indicates that 10 is not divisible by 3, and the remainder of the division is 1. On the other hand, in the second execution, the script checks the divisibility of 100 when divided by 20. The program successfully returns that 100 is divisible by 20.
3. Checking Odd Even Number Using “Mod” Operator
If an integer including positive and negative is perfectly divisible by 2 with 0 remainder, it is considered an even integer. This condition allows for the straightforward determination of odd or even numbers in Bash. Here’s how you can do it:
#!/bin/bash
# Get user input for the number
read -p "Enter a number: " number
# Use the modulo operator to check for even or odd
remainder=$((number % 2))
# Check if the remainder is zero
if [ "$remainder" -eq 0 ]; then
echo "$number is an even number."
else
echo "$number is an odd number."
fi
The Bash script takes a number from the user and calculates the remainder using the modulo operator when the number is divided by 2. It utilizes an if statement to check if the remainder is zero. If [ "$remainder" -eq 0 ]
holds true, the program prints a message indicating that the entered number is even. Otherwise, it states the number is odd.
4. “Mod” Operator to Check Leap Year
To determine whether a year is a leap year, apply the modulo operator to check the rules for leap years. Specifically, a leap year is either divisible by 4 but not by 100, or it is divisible by 400. Implement these conditions in the code:
#!/bin/bash
# Get user input for the year
read -p "Enter a year: " year
# Use the modulo operator to check for leap year
if [ $((year % 4)) -eq 0 ] && [ $((year % 100)) -ne 0 ] || [ $((year % 400)) -eq 0 ]; then
echo "$year is a leap year."
else
echo "$year is not a leap year."
fi
The script first takes a year as input from the user. Then, it checks whether the entered year is a leap year. It uses the modulo operator (%) to find the remainder when dividing the year by 4, 100 and 400. The conditions [ $((year % 4)) -eq 0 ]
checks if the year is divisible by 4, [ $((year % 100)) -ne 0 ]
checks if it’s not divisible by 100 and [ $((year % 400)) -eq 0 ]
checks if it’s divisible by 400. The &&
and ||
operators are used to combine these conditions logically.
Here, the script checks the years 2004 and 2022. It accurately determines that 2004 is a leap year, while 2022 isn’t.
5. Generating Sequence of Numbers Utilizing the “Mod” Operator
The modulo operator in Bash can be used creatively to generate a repeating sequence of numbers. For instance, the following script utilizes the modulo operator to repeat the sequence 0 to 5 three times.
#!/bin/bash
n=0
while [ $n -le 17 ]; do
echo $((n % 6))
n=$((n + 1))
done
The script initializes a variable n with the value 0. Then, it employs a while loop that iterates as long as n is less than or equal to 17. Within the loop, the script prints the remainder when n is divided by 6 using the mod operator n % 6
. After each iteration, the value of n is incremented by 1. As a result, the script generates a sequence of numbers representing the remainder of the division by 6, creating a repeating pattern of 0 to 5.
“Mod” Operator to Find Floating Point Remainder in Bash
To find the floating-point remainder using the mod operator, one can rely on the bc (basic calculator) tool. This tool is capable of calculating the remainder of a division of floating-point numbers.
echo "5.2 % 2" | bc
The echo command is used to write the expression “5.2 % 2”
. The expression is passed to the bc command using a pipe. The bc command calculates the remainder of the division of 5.2 by 2.
Conclusion
In conclusion, the mod operator in Bash is useful for many calculations. Moreover, this operator is easy to use. After reading this article, I believe there should be no room for confusion about the use of the mod operator in Bash.
People Also Ask
How to use mod in Bash?
To use mod in Bash, utilize the direct arithmetic expression or the variable assignment format. The syntax for arithmetic expression is echo $((Dividend % Divisor))
. And the syntax for variable assignment is echo $((var1 % var2))
where var1 and var2 store the values to be calculated, i.e. the dividend and the divisor.
How to use “mod” operator with “expr” command?
You can use the expr command along with the mod operator to find the remainder of a division. Here’s an example:
expr 15 % 4 # Output : 3
What is the result of “mod” operator with negative numbers in Bash?
The mod operator with negative numbers finds the remainder of a division in the same way as positive numbers. However, if the dividend is negative, the remainder will also be negative. On the contrary, the sign of the remainder doesn’t depend on the sign of the divisor.
echo $((-12 % 10)) # Output: -2
Mod operator to get the remainder when one variable is divided by another?
To get the remainder when one variable is divided by another, use the modulo operator. For instance, if you have variables a
and b
, the command echo ((a % b))
would yield the remainder of the division of a
by b
.
How to use the modulo operator in awk?
In awk, you can use the modulo operator (%) to calculate the remainder of a division. Here’s a simple example:
echo "15 % 4" | awk '{print $1}'
The string "15 % 4"
is passed to awk command. Within the awk command, the '{print $1}'
part prints the first field. This results in the remainder of the division which is 3.
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Calculate in Bash [3 Practical Examples]
- How to Sum Up Numbers in Bash [Explained With Examples]
- How to Divide Two Numbers in Bash [8 Easy Ways]
- Division of Floating Point Numbers in Bash
- How to Format Number in Bash [6 Cases]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
<< Go Back to Arithmetic Operators in Bash | Bash Operator | Bash Scripting Tutorial