FUNDAMENTALS A Complete Guide for Beginners
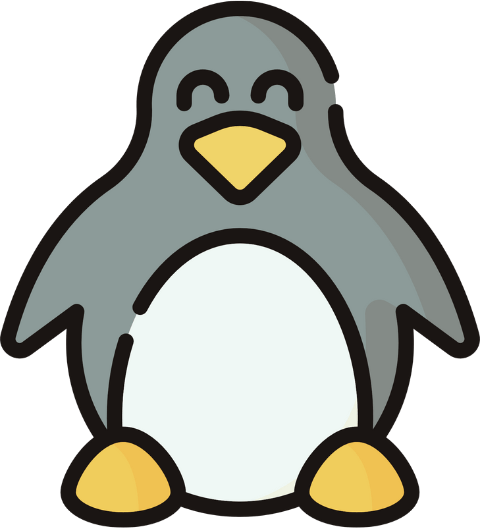
Adding numbers is one of the basic math operations one needs to perform. In the Bash shell, there are a couple of ways to add two or multiple numbers. It’s important to note that the Bash shell primarily performs integer arithmetics. For the addition of floating point numbers commands such as the bc or awk are quite useful. In this article, I will discuss different commands and techniques to sum up numbers in Bash shell.
Integer and Floating Point Numbers
Integer numbers such as 1, 5, 10, etc. are easy to handle using Bash arithmetic expansion. On the other hand, floating-point numbers for example 1.6, 10.5, 4.4, etc can not be added using simple arithmetic expansion. Fortunately, there are multiple commands for working with floating point numbers in Bash.
In the following section, I will show how you can add integer and floating-point numbers using different methods.
1. Sum Up Integer Numbers
There are several ways to sum up integer numbers. The shell built-in declare command, arithmetic expansion, and the expr command are names of a few. First, let’s talk about the use of the declare command to perform arithmetic addition:
A. Using the ‘declare’ Command
In Bash, one can declare a variable as an integer using the declare command with its option -i. This option tells bash to treat the variable as an integer. Look at the following commands:
declare -i A=2+8
echo $A
Here the declare command sums up 2 and 8, resulting in 10.
B. Using Arithmetic Expansion
To sum up two numbers using arithmetic expansion, enclose the numbers within an arithmetic expansion. For instance, to add 5 and 8, use the expression $((5 + 8)). Bash shell can successfully evaluate such expressions. For example:
#!/bin/bash
# Define the two numbers
read -p "Enter the first number: " num1
read -p "Enter the second number: " num2
# Calculate the sum
sum=$((num1 + num2))
# Display the result
echo "The sum of $num1 and $num2 is: $sum"
The read
command prompts (with option -p) the user to input the numbers for summation. Then the script uses the addition (+) operator in arithmetic expansion to add the values of num1 and num2.
C. Sum Up Integer Elements of an Array Using ‘expr’ Command
Another command is expr which is capable of adding integers. Here I will show you how to use this command to sum up integer elements of an array. To sum up the elements of an array, first, expand the array. Then iterate through each element and add them to a variable. Essentially, the variable contains the summation of all the elements of the array. See the below script to learn how it works:
#!/bin/bash
# Define an array of numbers
numbers=(2 5 8 3 6 9 4 7 10)
sum=$(expr 0)
for num in "${numbers[@]}"; do
sum=$(expr $sum + $num)
done
# Display the result
echo "The sum of the numbers is: $sum"
$(expr $sum + $num)
adds a single element with the sum
variable. The for loop accumulates the summation of all elements of the numbers
array in sum
.
(2 5 8 3 6 9 4 7 10)
. The result of the addition 54 is displayed in the terminal.
D. Utilizing the ‘let’ Command
The let command enables users to carry out arithmetic addition with minimal syntax. For instance, the expression let sum=num1+num2
captures the result of adding the variables num1
and num2
. Check the process through the following process:
#!/bin/bash
# Define integers
num1=10
num2=12
# Use let for arithmetic expansion to calculate the sum
let "sum = num1 + num2"
# Display the result
echo "The sum of $num1 and $num2 is: $sum"
In the script, let "sum = num1 + num2"
perform arithmetic addition. The let command adds the values stored in the variables num1 and num2. The result of this addition is then assigned to the variable sum.
2. Sum Up Float or Double Type Numbers Using ‘bc’ Command
To add float or double-type numbers first write the math expression using the echo command. Then, pass the expression to the bc command to evaluate. Let’s say you want to sum up 3.5 and 2.5. You can write the expression using echo "3.5 + 2.5"
. After that, you need to pass it to the bc command using a pipe. See the script below to get a clear idea:
#!/bin/bash
# Define the two floating-point numbers
read -p "Enter the first number: " num1
read -p "Enter the second number: " num2
# Use bc to calculate the sum
sum=$(echo "$num1 + $num2" | bc)
# Display the result
echo "The sum of $num1 and $num2 is: $sum"
The read
command prompts the user to input the numbers for summation. The script uses the echo command to write an expression "$num1 + $num2"
and pass it to the bc command for evaluation using a pipe (|) operator.
The awk command is also useful for adding floating-point numbers. For example, when you input echo 12.8 12.2 | awk '{print $1 + $2}'
, it calculates the sum of 12.8 and 12.2 and displays the result 25 in the terminal.
How to Sum Up Numbers From a File in Bash?
It is often necessary to sum numbers from a file. In most cases, these numbers are arranged in columns. Now, I will demonstrate how to add numbers from a file, both for integer and floating-point values:
A. Sum a Column of Integer Numbers From a File in Bash
One can add a column of numbers from a file using the awk command. This command can extract any field from each line of a file. For example, $2 and $3 extract the second and third fields of a line. Let’s consider column data like below:
# numbers.txt
4
10
15
$1 in awk command can extract the first field 4 from the first line and the first field 10 from the second line and so on. This way awk can accumulate all the numbers of the column. Let’s see a practical example:
#!/bin/bash
# Use awk to sum each column
sum_col=$(awk '{sum+=$1} END {print sum}' "numbers.txt"
# Display the results
echo "Sum of Column: $sum_col"
The program uses the awk
command to sum up the column of numbers from the numbers.txt file. It sums up the column of numbers using the '{sum+=$1} END {print sum}'
. Here, the sum variable accumulates the sum of the first column, and the END block prints the final sum.
B. Sum a Column of Floating Point Numbers From a File
Column-wise arranged numbers of a file may contain floating points. One can add these numbers in many ways. I will show you a very compact way to sum up using the perl command. Consider a file containing numbers like below:
# float.txt
4.5
10.1
15.4
To add the numbers of the float.txt file use the following script:
#!/bin/bash
perl -lne '$sum += $_; END { print $sum }' float.txt
The perl
command sums up a column of floating-point numbers from the file named float.txt. The -l
option ensures proper handling of newlines. The script increments the sum variable for each line in the input, and after processing all lines, the END { print $sum }
block prints the final sum to the terminal.
Conclusion
In conclusion, there are numerous ways to sum up numbers in Bash. However, based on the context, users should choose the proper method to complete the task quickly and efficiently. I hope this article helps you understand and implement the various techniques available for summing numbers in Bash.
People Also Ask
How to find the sum of a string of numbers in Bash?
Let’s say you have numbers in a string in the following format-
numbers="10 20 30 40 50"
To find the sum of numbers of the above string use the code below:
echo $(echo $numbers | tr ' ' '+' | bc)
How to add numbers from two files simultaneously?
Use awk to sum integers row-wise from multiple files. Let’s consider two files as follows-
#file0.txt
5
19
51
10
#file1.txt
75
11
43
845
paste file0.txt file1.txt | awk '{ sum=0; for(i=1; i<=NF; i++) sum+=$i; print sum }'
How to increment a variable by one in Bash?
To increment a variable by one use the post or pre-increment syntax depending on the situation. For example, ((var++)) and ((++var)) increase the value of the var variable by one.
# Declare a variable
var=5
# Increment the variable by one
((var++))
echo $var # output:6
How to perform a column-wise sum of numbers from a file?
To perform a column-wise sum of numbers of a multi-columned file use the awk command. Let’s say you have a file with numbers arranged as follows-
#data.txt
10 5
4 9
11 23
To add up numbers column-wise for the two columns of the data.txt file, use the following code:
awk '{sum1+=$1; sum2+=$2} END {print "Column 1 sum:", sum1, "\nColumn 2 sum:", sum2}' "data.txt"
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Calculate in Bash [3 Practical Examples]
- How to Divide Two Numbers in Bash [8 Easy Ways]
- Division of Floating Point Numbers in Bash
- How to Use “Mod” Operator in Bash [5 Basic Usage]
- How to Format Number in Bash [6 Cases]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
<< Go Back to Arithmetic Operators in Bash | Bash Operator | Bash Scripting Tutorial