FUNDAMENTALS A Complete Guide for Beginners
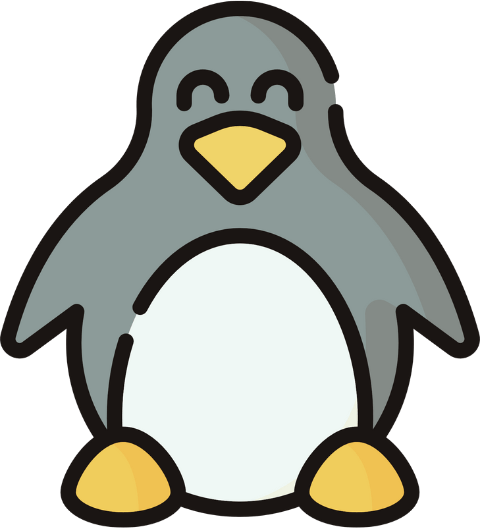
Decimal and hexadecimal are popular number systems. Decimal is base 10, using 10 symbols (0-9), while hexadecimal is base 16. Hexadecimal uses 16 symbols: 0-9 and A-F (or a-f), where A=10, B=11, C=12, D=13, E=14 and F=15. Bash users often convert hexadecimal numbers to decimal and vice-versa. Common techniques to convert hex to decimal in Bash are:
- Using arithmetic expansion with base conversion:
echo $((16#hex_number))
- Using bc command:
echo "ibase=16; hex_number" | bc
- Using printf command:
printf "%d" 0xhex_number
- Using perl code:
perl -le 'print hex("hex_number");'
- Using Python Utilities in Bash:
python3 -c 'print(int("hex_number", 16))'
- Using dc command:
dc -e '16i hex_number p'
Let’s explore the methods mentioned above for converting hexadecimal number to decimal, along with some additional approaches.
1. Using Arithmetic Expansion
Arithmetic expansion supports base conversion of numbers. The default base for Bash arithmetic is 10, but one can specify a different base using the syntax base#number
. Regardless of the original base of a number, arithmetic expansion in Bash always returns the equivalent decimal value of the number. For instance, the following command gives the decimal equivalent of the hexadecimal number “FF” :
echo $((16#FF))
The image shows that the command returns 255 which is the decimal equivalent of hexadecimal “FF”.
To take a hexadecimal number from the user and find its equivalent decimal, follow this script below:
#!/bin/bash
# Input: Hexadecimal number
read -p "Enter the hexadecimal number: " hex_number
# Conversion using Bash arithmetic
decimal_number=$((16#${hex_number}))
# Output
echo "Equivalent Decimal: $decimal_number"
The Bash script takes user input in hexadecimal format. $((16#${hex_number}))
converts the given hexadecimal number into a decimal number using arithmetic expansion and base conversion.
2. Using “bc” Command With “ibase” and “obase”
To convert hexadecimal numbers to decimal using bc (Basic calculator) command, use ibase and obase to define the input base and output base respectively. The default base in bc is 10. So it isn’t mandatory to mention obase when you convert hex to decimal. Look at the example below:
echo "ibase=16; FF" | bc
Here, the input base is 16 and the given hex number is “FF”. The output base is not defined explicitly. However, if you want to specify the output base, you can use the code below:
echo "ibase=16;obase=A;1A" | bc
obase value A denotes that the output base is 10. So, the above command will convert hexdecimal “1A” to its equivalent decimal. The first command converts hex “FF” to its decimal which is 255. The second command successfully converts hex “1A” to its equivalent decimal which is 26. The output base is set to decimal(or A) in the second command, however, the first command uses default ouput base.
3. Using “printf” Command
The printf command and its format specifiers are enough for converting a hexadecimal number to decimal. The format specifier %d
is used to print a decimal integer. To convert a hex number to decimal provide the hexadecimal literal after 0x
just like the following commands:
printf "%d\n" 0xA
printf "%d\n" 0xff
printf "%d\n" 0xFF
0x
indicates that the following characters are in hexadecimal format. For example, 0xA
represents the hexadecimal number A. \n
is a newline character, which adds a line break after printing the number.The first command converts a hexadecimal literal “A” to its decimal equivalent (10) using printf. The subsequent commands test the printf command with hexadecimal literals “ff” and “FF”, returning 255 as their decimal equivalents.
4. Using “perl” Command
One can execute Perl code in Bash using the perl command with -e option. In Perl, there is a function called hex that takes hexadecimal values and returns corresponding decimal numbers. Look at the simple command below:
perl -le 'print hex("F");'
The option -e
enables the user to execute Perl code in Bash. -l
prints the result in a new line. Within the print block, the hex function takes hexadecimal input and returns decimal output.
5. Using Python Utilities
Once a Python version is installed in the Bash shell, users can execute Python code using the python2 or python3 command. The int function in Python takes two arguments, the first one represents the number and the second one is the base of the number used in the first argument. The default base is 10. The syntax is:
int(x, base=10)
To convert a hex number to decimal with above syntax, set base value 16 and replace x
with the hex number. Following command returns the decimal equivalent of hexadecimal literal “FF”.
python -c 'print(int("FF", 16))'
Here, python -c
enables the user to execute Python code in Bash. int("FF", 16)
converts hexadecimal “FF” to its equivalent decimal integer. Finally, the print function is used to print the decimal ouput.
The command is tested for finding the decimal of hex literal “F”, “FF” and “A”. Equivalent decimals of those hex literals are displayed in the image above.
NOTE: To install python3 in your system, use the code below:
sudo apt install python3
6. Using “dc” Command
The dc (desk calculator) command can convert a hex number to decimal. The -e option of this command indicates that the string followed by the command name should be treated as an expression. To convert hex to decimal in Bash using dc, see the following command:
dc -e '16i F p'
In the above command, input base is set to hexadecimal (base 16) with 16i
. “F” is the hexadecimal number needed to convert. Finally, p
is for printing the converted value.
7. Using Ruby Code
Ruby is a programming language. Bash users can easily run ruby code in a Bash shell. To convert a hex number to decimal you can use ruby command with its option -e like the following:
ruby -e 'p "FF".to_i(16)'
ruby command with -e
option makes the ruby code executable in Bash shell. 'p "FF".to_i(16)'
indicates that the string “FF” is in hexadecimal format. p
is used to print the converted result in the console.
NOTE: If ruby is not installed in the system, install it using the following code:
sudo apt install ruby
8. Using Node.js
Javascript has a function called parseInt that can convert hex numbers to decimal. Fortunately, Bash users can run Javascript code in the shell. Here is a simple code that converts hexadecimal “FF” to its decimal value 255.
node -e "console.log(parseInt('FF', 16))"
node -e
allows the user to execute Javascript code in the Bash shell. parseInt('FF', 16)
converts the string ‘FF’ from hexadecimal (base-16) to its decimal equivalent. Then console.log(...)
prints the decimal result to the console.
NOTE: To install node.js in your system, use the code below:
sudo apt install nodejs
9. Using Rhino
One can pass a heredoc to rhino and convert a hex number to decimal. Here’s how:
rhino <<EOF
print(parseInt('FF', 16))
EOF
A heredoc is passed to rhino. The heredoc includes the parseInt function that takes hexadecimal input with base. The return of this function is the decimal equivalent of the hex literal.
NOTE: To install rhino in your system use the following code:
sudo apt install rhino
10. Using User-Defined Function
Apart from the tools and commands, one can utilize full-fledged Bash programming to define a function for hex-to-decimal conversion. The following function is written from scratch to convert a hex number to decimal:
#!/bin/bash
declare -A hex_digit_val=(
[0]=0 [1]=1 [2]=2 [3]=3
[4]=4 [5]=5 [6]=6 [7]=7
[8]=8 [9]=9 [a]=10 [b]=11
[c]=12 [d]=13 [e]=14 [f]=15
)
hex_to_decimal() {
local hex=${1,,} # Convert to lowercase to handle both upper and lower case hex
local base
local dec=0
for ((i = 0; i < ${#hex}; i++)); do
base=${hex:$i:1}
dec=$((dec * 16 + hex_digit_val[$base]))
done
echo $dec
}
# Example usage
result=$(hex_to_decimal "1A")
echo $result
The script first defines an associate array to map the hexadecimal symbols to their corresponding decimal symbols.
Then it defines a function called hex_to_decimal. It declares a local variable hex
and assigns the value ${1,,}
to it. ,,
syntax converts the variable to lowercase, ensuring that the function can handle both uppercase and lowercase hex characters. In addition, the function declares a local variable base
that will be used to store each individual hex digit during the conversion. Moreover, a local variable dec
is initially set to 0. This variable will store the decimal equivalent of the hex number.
Next, it initiates a for loop that iterates through each character in the hexadecimal string. ${hex:$i:1}
retrieves the current hex digit at position $i
and assigns it to the variable base
. $((dec * 16 + hex_digit_val[$base]))
updates the decimal equivalent by multiplying the current value by 16 and adding the decimal value of the current hex digit. The decimal values are obtained from an array defined earlier in the script. Finally, echo $dec
prints the final decimal equivalent after the conversion.
How to Convert A List of Hexadecimal Numbers to Decimal
Sometimes it’s necessary to convert multiple hex numbers or a list of hex numbers into decimals. To convert a list of hexadecimal numbers into decimals use a loop to iterate over each number of the list. Take the following list of hexadecimal numbers in hex_numbers.txt file as an example:
# hex_numbers.txt
D8E4
FF2B
1A
4C91A7
8D3F12B
I use a while loop to convert each hexadecimal number of the list into decimals. Check the following script to convert a list of hexadecimal numbers to decimal in Bash
#!/bin/bash
echo "Equivalent decimal numbers:"
# Read the file line by line
while IFS= read -r line; do
# Convert hexadecimal to decimal
decimal=$(echo "ibase=16; $line" | bc)
echo "$decimal"
echo
done < hex_numbers.txt
The script reads the list of hexadecimal numbers line by line. For each line, it utilizes the echo command to pass the hexadecimal number to the bc command with ibase=16
to specify hexadecimal input. The resulting decimal value is assigned to a variable and then printed as the equivalent decimal of the corresponding hexadecimal number.
As you can see, the program converts all the hexadecimal numbers of the list into their decimal equivalent.
Conclusion
In conclusion, hex-to-decimal conversion in Bash is fairly easy. Users can work seamlessly with different numerical bases in the Bash shell. To do the task, you can adapt any technique discussed in this article. Hope you can choose the best way to convert your hex numbers to decimal.
People Also Ask
How to convert decimal numbers to hex in Bash?
To convert decimal numbers to hex in Bash, use the printf command with %x format specifier. For example:
echo $(printf "%x" 255)
Lowercase x
returns lowercase hex literal whereas uppercase X
returns uppercase hex literal. For example ouput of the above code will be ff
. But if you use "%X"
as format specifier the output will be FF
.
What is the simpliest way to convert hex to decimal and vice-versa?
The simplest way to convert hexadecimal to decimal and vice versa in Bash is by defining a function using bc command. The following function can be useful for converting hexadecimal to decimal as well as decimal to hex:
#!/bin/bash
h2d(){
echo "ibase=16; $@" | bc
}
d2h(){
echo "obase=16; $@" | bc
}
# Convert the hex numbers to decimal
h2d "FF"
# Convert the decimal numbers to hex
d2h 10
Does hex number system use a decimal point?
No. The hexadecimal number system does not use a decimal point. The representation of values in hex is integer-based, and there is no explicit decimal point. If fractional values need to be represented, it’s typically done by using a whole number part and a separate part for fraction, rather than a decimal point within the hex number itself.
What is the limit of Bash hex to decimal conversion?
Bash hex to decimal conversion is limited to 64-bit integers, equivalent to 16 hexadecimal digits. The maximum representable hexadecimal value is 7fffffffffffffff. When a hex number exceeds 16 digits, Bash considers only the last 16 digits for conversion. For example:
echo $((16#1157e470953d0000))
echo $((16#11157e470953d0000))
The decimals of 1157e470953d0000 and 11157e470953d0000 are the same because the second hex number is 17 digits long, so Bash drops the first character. This makes both the hex number equal so the equivalent decimal.
How to convert hex to binary in Bash?
To convert hex to binary use the bc tool. For instance, following code converts the hexadecimal “FF” to its binary:
echo "obase=2; ibase=16; FF" | bc