FUNDAMENTALS A Complete Guide for Beginners
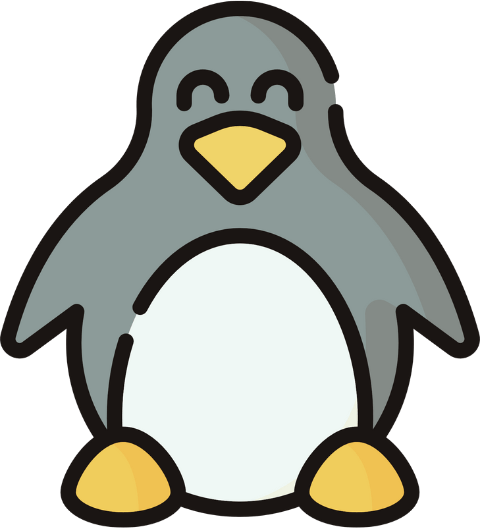
In Bash, the for loop is a well-known and versatile feature that helps you automate repetitive tasks efficiently, making your scripts more concise and powerful. If you are a Linux enthusiast, understanding how to use the for loop in the command line can significantly improve your productivity. This guide will provide an overview of the for loop in bash and how to use it properly within a script.
What is “For Loop” in Bash?
The “For Loop” in Bash is a control flow statement that allows you to iterate over a sequence of values and perform a set of commands for each value. It is useful for iterating over a sequence of items, such as a list of files, a set of numbers, or the characters in a string.
The basic syntax of a “for loop” in Bash looks like this:
for item in list
do
# Commands to be executed
done
- for: Initiates the for loop and iterates over each item in the list.
- item: Represents a placeholder that takes each value from the list individually in each iteration.
- list: Specifies the set of items over which the loop will iterate.
- do: Indicates the start of the statements that execute in every loop iteration.
- # Commands to be executed: These statements will be executed in each loop iteration.
- done: Mark the end of the for loop.
6 Cases of For Loop in Bash
For loop is one of the most fundamental programming constructs in Bash scripting. They allow a block of code to be executed repeatedly for a set of specified values or conditions. Loops are necessary for automating repetitive operations and for iterating over data efficiently. Now check the following 6 ways you can utilize the for loop in bash scripting:
1. List Based For Loop Example
Bash’s simple for loop provides a simple syntax for iterating over a set of elements in Bash. The following example illustrates the use of the for loops by using a simple counting mechanism. Here, for variable in element1 element2 ... elementN
structure is used to count from 1 to 5. The loop iterates over a specified set of elements, in this case, numbers, executing commands for each iteration.
#!/bin/bash
# Simple For Loop Example
for number in 1 2 3 4 5
do
echo "Count: $number"
done
echo "Done."
This Bash script uses a simple “for loop” to loop through the numbers 1 through 5, echoing each count. The loop begins by initializing the variable “number”, and then prints “Count: 1”, “Count: 2”, and so on. Once all the numbers have been processed, the script concludes by echoing “Done.” The script demonstrates the basic functionality and versatility of a simple “for loop” in Bash.
In this picture, you can see that the script loops through 5 iterations and prints each iteration number, and after finishing that prints “Done”.
2. Range-Based For Loop
In Bash scripting, the range-based for loop provides a concise way to iterate over a sequence of values. Numerical ranges can be processed efficiently through the use of the for value in {initial value..final value
} structure. In the following example, the range is used in bash scripting using a for loop to print values from 1 to 5. Let’s see how:
#!/bin/bash
# Range-based For Loop Example
for value in {1..5}
do
echo "Value: $value"
done
echo "Done."
This script demonstrates a range-based “for loop” that iterates over values between 1 and 5. The loop begins by initializing the variable “value” and echoing each value. After processing the range, it concludes by echoing “Done.”
In this picture, for loop runs for 5 iterations, print the iteration value and then prints “Done”.
3. Array Iteration Using For Loop
Array iteration is the process of going through each element one by one. In Bash, iteration is commonly achieved using loops. For example, to iterate through an array, first you will create an array like Array=("item1" "item2" "item3" ... "itemN")
. Then you will iterate over each element of the array using the for loop.
See the below Bash script where array iteration is used to iterate over some fruits’ names and print their name using bash for loop:
#!/bin/bash
# Array Iteration For Loop Example
fruits=("apple" "banana" "orange")
for fruit in "${fruits[@]}"
do
echo "Fruit: $fruit"
done
echo "Done."
This script iterates through an array named fruits using a for loop. The loop initializes the variable “fruit,” echoing each fruit name. The loop continues until all fruits in the array have been processed.
In this image for loop iterate through 3 fruits’ names and print their name and finally after completing it print “Done”.
4. C-Styled For Loop
In bash scripting, a C programming styled for loop is used to iterate over a sequence of numbers. Similar to C programming this for (( initialization; condition; increment/decrement ))
structure is used. In the following example, all integer number from 1 to 5 is iterated using a C-styled for loop:
#!/bin/bash
# C-Styled For Loop Example
for ((i=1; i<=5; i++))
do
echo "Index: $i"
done
echo "Done."
This script uses a C-style for loop to iterate from 1 to 5. In each iteration, the loop variable i
is incremented by 1, and its value is echoed. The loop increments “i” until it reaches 5, and then the script concludes by echoing “Done.”
The output shows a C-styled for loop that runs & prints Index: (1…5) for 5 iterations and then breaks free from the loop.
5. Infinite For Loop
An infinite loop in bash loop is similar to a C-styled for loop in structure but inner conditions are kept blank like for (( ; ; ))
. Here is an example of using for loop in bash scripting to create an infinite loop:
#!/bin/bash
# Infinite For Loop Example
for (( ; ; ))
do
echo "This loop is infinite. Press Ctrl+C to exit."
# Additional commands can be placed here
sleep 1 # Adding a sleep command to prevent excessive output
done
# This won't be reached in an infinite loop
echo "Done."
This script utilizes an infinite for loop, in which the loop condition is deliberately left blank, resulting in the loop running continuously. During the loop, a message is printed indicating that the loop is infinite, and the user is then prompted to press Ctrl+C to terminate the loop. This is followed by a delay of one second using the sleep command, which prevents excessive output. Generally, an infinite loop will terminate when the user manually interferes with it by pressing Ctrl+C.
From the image, you can see that the infinite for loop was running and only stopped after interrupting it by pressing CTRL+C.
6. For Loop with Break & Continue
In Bash scripting break
and continue
are very important control statements. The continue
statement is used to skip the rest of the commands inside the loop for the current iteration and move on to the next iteration. While the break
statement terminates the current iteration of the loop.
In the following example for loop is used to iterate over some number and then continue
is used to skip loop content for the even numbers and break
is used to terminate the loop after reaching number 5:
#!/bin/bash
echo "Example with Break and Continue:"
# Using a for loop to iterate from 1 to 7
for number in {1..7}
do
# Using a conditional statement to skip the loop for even numbers
if [ $((number % 2)) -eq 0 ]; then
echo "Skipping the loop for even number: $number"
continue
fi
echo "Processing odd number: $number"
# Using a conditional statement to break out of the loop at number 5
if [ $number -eq 5 ]; then
echo "Breaking out of the loop at number 5."
break
fi
done
echo "Loop complete."
This script demonstrates a for loop that iterates from 1 to 7. It skips even numbers using continue, processes and echoes odd numbers, and breaks out of the loop at number 5 using break. The script concludes by echoing “Loop complete”. This demonstrates the strategic utilization of break and continue when controlling a for loop.
See from the image, odd numbers are processed and the loop task is skipped for even numbers using the continue
statement, and after reaching 5 the whole loop ended from the break
statement.
Conclusion
In summary, becoming proficient with the “for loop” unlocks a realm of opportunities, enhancing the efficiency and enjoyment of your Linux experience. Whether working with files, examining data, or performing multi-step operations, the for loop is your reliable companion. Its straightforward structure and adaptability make it a must-have tool for Linux enthusiasts. So, dive in, explore, and witness the magic of automation unfold with this powerful command!
People Also Ask
What is a loop?
Loop in Bash Scripting is a powerful tool that allows you to execute a block of code repeatedly. The for loop iterates over a sequence, the while loop repeats as long as a condition is true, and the until loop continues until a condition becomes true. Loops are essential for automating repetitive tasks and processing data in a script.
What is a Bash for loop used for?
A Bash for loop is used for iterating over a sequence of elements, such as numbers, strings, or file names. It allows you to perform a set of commands repeatedly for each element in the specified sequence. The loop structure is versatile, making it useful for various tasks, including processing files, generating sequences, or executing commands with different parameters.
What is the purpose of “continue” in a for loop?
In a Bash for loop, the continue
statement is used to skip the rest of the commands inside the loop for the current iteration and move on to the next iteration. When a continue
statement is encountered, the loop skips any remaining commands in its body and proceeds with the next iteration, ignoring the remaining code for the current loop cycle. This enables the execution of loop iterations according to certain conditions.
How does “break” work in a Bash for loop?
In a bash for loop, the break
statement terminates the current iteration of the loop at the same time it exits the loop. This statement terminates the loop execution at the same time as it exits the loop, skipping any other statements in the body of the loop. Upon the break statement’s occurrence, the loop’s control variable is not incremented and the loop’s condition is not evaluated. The loop terminates at the same time, and execution continues to the code that follows the loop. Here’s a simple example to illustrate the use of “break” in a for loop:
#!/bin/bash
for i in {1..5}; do
echo "Iteration $i"
if [ $i -eq 3 ]; then
break # Exit the loop when i equals 3
fi
done
echo "Loop complete"
In this example, the loop will print “Iteration 1,” “Iteration 2,” and “Iteration 3.” Once i equals 3, the “break” statement is executed, and the loop terminates. The final “Loop complete” message is then printed.
Can you nest for loops in Bash?
Yes, you can nest for loops in Bash. Nesting means placing one or more loops inside another loop. This allows you to create more complex loop structures for iterating through multiple data sets. Here’s a simple example of nested for loops:
#!/bin/bash
for i in {1..3}; do
echo "Outer loop iteration $i"
for j in {A..C}; do
echo " Inner loop iteration $j"
done
done
In this example, there’s an outer loop that iterates three times, and for each iteration of the outer loop, there’s an inner loop that iterates through the letters A to C. The output will show the iterations of both the outer and inner loops.
What is the significance of “done” in a for loop?
The keyword done
in a Bash for loop serves as a marker that signals the end of the loop body. It indicates that the code block enclosed between the do
and done
keywords must be executed for each successive iteration. After the loop has completed processing all elements or conditions in the loop, the ‘done’ keyword indicates the point at which the ‘loop control variable‘ is updated and the ‘loop condition‘ is re-evaluated.
How do you break out of nested for loops?
In Bash scripting, you can break out of nested for loops using the break
statement along with an integer. Here’s an example to illustrate how this can be done:
#!/bin/bash
# Outer loop
for ((i=1; i<=3; i++)); do
# Inner loop
for ((j=1; j<=3; j++)); do
echo "Outer loop: $i, Inner loop: $j"
# Check a condition to break out of both loops
if [ "$i" -eq 2 ] && [ "$j" -eq 2 ]; then
break 2 # Breaks out of both loops
fi
done
done
In this example, the script uses a labeled break statement (break 2) to break out of both the outer and inner loops when a specific condition is met.
If you use the break
command without specifying a number, it breaks out of the innermost loop. The break
statement is used to exit from a loop prematurely based on a specified condition. Here’s another example:
#!/bin/bash
for ((i=1; i<=3; i++)); do
echo "Outer loop: $i"
for ((j=1; j<=3; j++)); do
echo " Inner loop: $j"
if [ $i -eq 2 ] && [ $j -eq 2 ]; then
break # Breaks out of the innermost loop
fi
done
done
In this example, when the inner loop reaches the specified condition, the break
statement is triggered without a number argument, causing it to exit the innermost loop (the “j” loop). The outer loop (the “i” loop) continues until completion.
What happens if “continue” or “break” is used outside of a loop?
In Bash scripting, if the continue
or break
statement is used outside of a loop, it will result in a syntax error. These statements are designed to be used within loops to control the execution flow.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to Loops in Bash | Bash Scripting Tutorial