FUNDAMENTALS A Complete Guide for Beginners
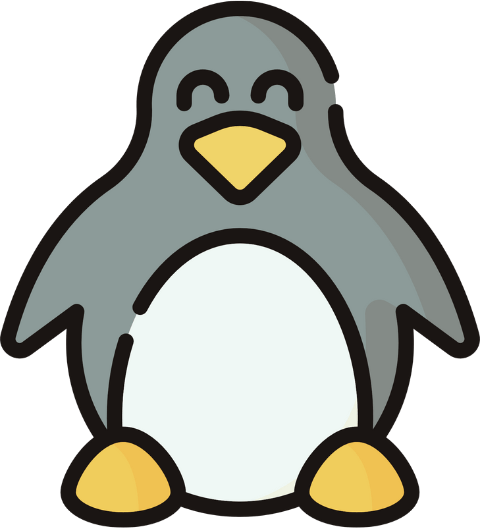
In Bash scripting, the loop iterates over a list. To skip any specific iteration entity, use the continue statement. The continue statement skips the current iteration without executing subsequent commands and jumps to the next iteration. Here, I will explore how to skip the iteration entity in the bash loop using the continue statement.
The Bash Continue Statement
The continue statement in bash skips the current iteration entity and jumps to the next iteration. The loop iterates through a list of items. Integrating the continue statement with it allows one to skip a specific iteration and move to the next iteration.
The syntax of the continue statement:
for iteration_variable in list
do
if [ condition_to_be_checked ]
then
continue
fi
done
The if [ condition_to_be_checked ]
checks for a specific condition to check. If the condition is true, it executes the continue command.
The exact syntax goes for the break statement.
9 Examples of Using Continue Statement in Bash Loop
Bash loop with the continue command can iterate through only the desired entity from the iteration list and search for a specific file type. Here, I have listed some of these.
1. Using Continue Statement in “for” Loop
The for loop iterates over a predefined list. To skip any specific item from that list, use the continue statement. Here is a bash script that will find the even numbers from a list:
#!/bin/bash
for a in {1..20}; do
if [[ $(( $a % 2 )) -ne 0 ]]; then
continue
fi
echo "Even: $a"
done
for a in {1..20};
initiates a for loop that iterates over the values of a from 1 to 20. Then if [[ $(( $a % 2 )) -ne 0 ]];
checks whether the reminder of a, divided by 2, is not zero or zero to determine whether it is odd or not. Finally, it prints a message according to comparison results.
2. Command Substitution
Command substitution means using the output of a command on another command. It eases the approach to using the output of different commands in the script cause it removes the necessity of defining extra variables. Here is a bash script to print all files:
#!/bin/bash
for f in $(ls); do
if [ ! -f $f ]; then
continue
fi
echo "Content: $f"
done
for f in $(ls);
initiates a for loop, which will iterate over the ls command output. if [ ! -f $f ];
checks whether the current iteration value is a regular file. The condition result will be true if it is not a regular file. If so, then it will skip the present iteration. Otherwise, it will print the filename on the terminal.
3. Wildcard Pattern
The wildcard pattern is used to find a match. It enables users to search for a specific pattern. Here is a bash script to search for text files inside the current directory:
#!/bin/bash
for f in *.txt; do
if [ ! -f $f ]; then
continue
fi
echo "Text File: $f"
done
for f in *.txt; do
checks for all files having .txt extension. And if [ ! -f $f ];
checks whether it is a regular file. Finally, the echo command prints a message along with the file name.
4. Using Continue Statement with an Array
The array contains a combination of characters that possess vital information or data. For loop can iterate over each array element of the array and execute as a set of commands including the continue statement for each iteration entity. Here is a bash script to print an array list on the terminal except for an element:
#!/bin/bash
a=(10 20 30 40 50)
for c in "${a[@]}"; do
if [ $c -eq 30 ]; then
continue
fi
echo "Number: $c"
done
Here, for c in "${a[@]}";
initiates a for loop where loop variable c iterates over a list 10 20 30 40 50. if [ $c -eq 30 ];
checks whether it is equal to 30 or not. If yes, it will skip the current iteration otherwise, it will print the value of c on the terminal.
5. Using Case Statement
The case statement checks various conditions and makes decisions accordingly for each condition. It is useful when working with multiple conditions at a time. Here is a bash script to search for a specific iteration entity:
#!/bin/bash
for c in 10 20 30 40 50; do
case $c in
30)
continue
;;
esac
echo "Iteration $c"
done
for c in 10 20 30 40 50;
initiates a for loop where loop variable iterates over 10 20 30 40 and 50. Then, the case statement jumps to the next iteration when the loop variable is 30. Otherwise, it will print the value of the loop variable.
6. Using Function
The function is a group name where a set of commands is grouped to perform a specific task. It helps to break down a giant script into task-specified small modules. Here is a bash script using a continue statement with a function:
#!/bin/bash
function is_even() {
if [ $(($1 % 2)) -eq 0 ]; then
return 0
else
return 1
fi
}
for a in {1..5}; do
if is_even $a; then
continue
fi
echo "Odd number: $a"
done
function is_even()
defines a function where if [ $(($1 % 2)) -eq 0 ];
checks whether the value of the remainder after dividing the passed argument by 2 is zero. If yes, it will return 0. In the main section of the code, the for a in {1..5};
initiates a for loop that will iterate over a list of 1 to 5. Then if is_even $i;
pass the loop variable to the is_even
function. If the return is zero, it will execute the continue statement, skipping the current iteration otherwise, it will print the loop variable, which is an odd number.
7. The Continue Statement in “until” Loop
The until loop executes a block of commands as long as a specified condition is false. Here is a bash script using the continue statement in the until loop:
#!/bin/bash
a=90
until [[ $a -gt 100 ]]
do
if [[ $a -eq 95 ]]
then
echo “Number $a!”
((a++))
continue
fi
echo $a
((a++))
done
until [[ $a -gt 100 ]]
initiates an until loop, which will execute as long as a is smaller than 100. After that, if [[ $a -eq 95 ]]
checks whether a is equal to 95. If so, it will print the number with a text. ((a++))
will increase the value of a by one. Otherwise, just the number will be printed.
8. The Continue Statement in “select” Loop
The select loop offers users a set of menus to choose from. Here is a bash script for using the continue statement in the select loop:
#!/bin/bash
PS3="Enter a number: "
select a in 10 20 30 40 50 60 70 80 90 100
do
if [[ $REPLY -eq 9 ]]
then
echo "Number $a!"
continue
fi
echo "Selected number: $a"
done
PS3="Enter a number: "
sets a prompt string for the select loop. select a in 10 20 30 40 50 60 70 80 90 100
presents a menu of 10 choices to the user. Then, if [[ $REPLY -eq 9 ]]
checks if the user’s reply is 9. If yes, it will print the number with a text. Otherwise, it will print the number with another text.
9. Using Continue Statement in Nested Loop
A nested loop means placing one loop inside another loop. In this approach, the loops can be of any type. Here is a bash script for using a continue statement in a nested loop:
#!/bin/bash
for i in {5..9}
do
for j in {5..9}
do
if [[ $i -eq $j ]]
then
echo "$i = $j"
continue 2
fi
echo "$i =/= $j"
done
done
echo “Done!”
for i in {5..9}
initiates a for loop which iterates over 5 to 9, assigning the current value to the variable i. Then for j in {5..9}
initiates the inner loop of the nested loop. After that, if [[ $i -eq $j ]]
checks the equality between i and j. If these are equal, it will print a message. Otherwise, it will print another message.
Using “for” Loop with a Break Statement
The break statement stops the execution of a loop and jumps to the subsequent commands after the loop. Here is a bash script that terminates the for-loop execution for a specific iteration item:
#!/bin/bash
n=("Albe" "Stane" "Ngidi" "Mark")
for a in ${n[@]}
do
echo $a
if [ $a = "Ngidi" ]
then
break
fi
done
for a in ${n[@]}
initiates a for loop, which will iterate over each element of n array and print the iteration entity. If the iteration value is “Ngidi” the break command will terminate the loop before printing.
Exiting a Loop Based on User Input
Put the break command inside an if condition to control the loop according to user input. Here is a bash script to print a series of numbers using a for loop and exit the loop according to the user input:
#!/bin/bash
echo "Enter number: "
read n
for ((a=1 ; a <= n; a++)); do
echo $a
if [ $n -eq 0 ]
then
break
fi
done
read n
reads the user’s input from the command line and stores it in the variable. After that for ((a=1 ; a <= n; a++));
initiates a loop. If the value of n is 0, then it will terminate the for loop.
Precaution of Using the Continue Statement
The purpose of the continue statement is to skip the remaining code within a loop for the current iteration and jump to the next iteration. And to avoid unnecessary processing efficiently. A wrong placement of the continue statement might skip the entire loop, so be careful before implementing it into the Bash script. To be sure about the execution of the continue statement, put a comment just before the continue statement.
Conclusion
In conclusion, the continue statement and the break statement are two loop control statements to regulate the loop execution. This statement helps users skip a specific iteration entity and terminate a loop forcefully. This article will help programmers implement the continue and break statements correctly for efficient management.
People Also Ask
How do I continue a loop in Bash?
To continue a loop in Bash, use the continue statement. It will skip the current iteration and move to the next iteration.
How do you stop a script in a loop in Bash?
To stop a script in a loop in Bash, press CTRL+C. It will immediately generate an interrupt signal and send it to the program to stop the execution of a loop inside the bash script.
How do you create an infinite loop in Bash?
To create a loop in bash, select any type of loop from for loop, while loop, until loop, then set the loop condition as accurate. It will repeatedly execute a command inside the loop until something explicitly stops it.
Can you pause a bash script?
Yes, you can pause a bash script. To pause a bash script, use the sleep command and a specified second you want to pause. For example, sleep 5 will pause the script for 5 seconds.
How to Exit from a Loop in Bash?
To exit from a loop in Bash, use the break command. The break command is a control statement that terminates a loop forcefully and jumps to the next part of the program after the loop. It is a solution when the loop can not be controlled with a loop condition.
What if break is not used?
Without the break command, the loop can not be interrupted forcefully. The loop will continue to iterate through all list elements and will not stop before any error or interruption occurs.
Is “continue” Statement Really Continuing?
The continue statement does not continue to command execution. It quickly skips the subsequent commands after the continue statement and jumps to the next iteration.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial