FUNDAMENTALS A Complete Guide for Beginners
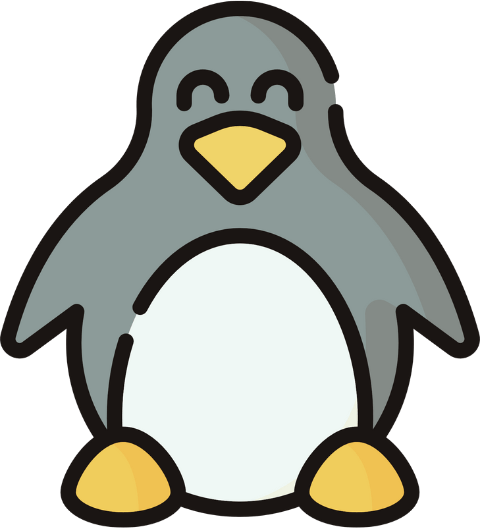
In the world of bash scripting, loops are like building blocks, helping to do things automatically and making tasks easier. One special tool in these loops is the “for” loop, and it’s great for efficient iteration through a set of values. You need to know how to use variables in a loop for precise control over the script. This article will dive into the various examples of utilizing the Bash “for” loop with variables.
12 Examples of Bash “for” Loop with Variable
Loop variables in Bash scripting play a fundamental role in executing repetitive tasks by dynamically adapting to values during each iteration. Whether iterating through arrays of numbers, strings, or processing files, the loop variable provides a flexible mechanism for accessing and handling different elements. In this article, I will go through some of the common examples of using variables in the for loop.
Example 1: Print All Array Elements
To print all array elements, use a “for” loop to go through all elements of the array. Run the following bash script to do so:
#!/bin/bash
# Define an array of numbers
numbers=(1 2 3 4 5)
for num in "${numbers[@]}"; do
echo "Number: $num"
done
This Bash script begins by defining an array named numbers containing the values 1, 2, 3, 4, and 5. Subsequently, a “for” loop is initiated, iterating through each element in the numbers array. The loop variable num dynamically takes on the value of each array element during each iteration. Within the loop, the script utilizes the echo command to print the current value of num alongside the string “Number:”.
Here, all array elements are echoed on separate lines.
Example 2: Calculate the Sum of Numbers
Calculating the sum of some predefined numbers is a very common task in Bash scripting. The script uses a “for” loop to iterate through the numbers of a list and adds them to a running total. After the loop is complete, the total sum is echoed. Execute this script to calculate the sum of numbers:
#!/bin/bash
sum=0
# Iterate over a list of numbers
for number in 1 2 3 4 5
do
# Add the current number to the sum
sum=$((sum + number))
done
# Print the sum after the loop
echo "Sum: $sum"
This Bash script initializes a variable sum to zero. It then employs a “for” loop to iterate through a list of numbers. Within the loop, the current number is added to the sum variable using the ((sum + number))
syntax. As the loop iterates through each number, the sum accumulates their values. This script effectively calculates the sum of the specified numbers and stores the result in the sum variable. After the loop is complete it echoes the total sum.
The sum of all the numbers on the list is echoed.
Example 3: Print Array of Strings
Working with arrays in Bash scripting allows for efficiently handling multiple similar values. This example introduces a bash script that iterates through an array of strings, specifically fruit names, and then prints each fruit along with a label as shown below:
#!/bin/bash
# Define an array of strings
fruits=("Apple" "Banana" "Orange" "Grapes")
for fruit in "${fruits[@]}"; do
echo "Fruit: $fruit"
done
This Bash script defines an array called fruits with strings representing different fruits. Using a “for” loop, it iterates through each element in the fruits array, assigning the current fruit to the variable fruit during each iteration. Within the loop, the script uses the echo
command to print each fruit alongside the string “Fruit:”.
Example 4: Print Words in a Sentence
Efficiently breaking down a sentence into individual words is a common need in Bash scripting. To print all words of a sentence separately in individual lines use a “for” loop to iterate over the sentence as demonstrated in the following script:
#!/bin/bash
sentence="Bash scripting is great for automation"
for word in $sentence; do
echo "Word: $word"
done
This Bash script defines a variable sentence containing the string “Bash scripting is great for automation.” It then utilizes a “for” loop to iterate through each word in the sentence, with the loop variable word representing each space-separated word. The script uses the echo
command to print each word preceded by the string “Word:”.
Here all words of the sentence are printed on separate lines.
Example 5: Check Odd/Even Numbers
Analyzing and classifying numbers as odd or even is a fundamental aspect of programming logic. This example presents a bash script that prompts the user to enter a number, validates the input, and then uses “if” conditional statements and a “for” loop to determine if each number from 1 to the user’s input is odd or even. To do so execute the script below:
#!/bin/bash
echo "Enter a number: "
read user_input
# Check if the input is a valid number
if ! [[ $user_input =~ ^[0-9]+$ ]]; then
echo "Invalid input. Please enter a valid number."
exit 1
fi
# Check if the number is greater than 0
if (( user_input <= 0 )); then
echo "Invalid input. Please enter a positive integer greater than 0."
exit 1
fi
# Use a for loop to iterate through a range
for (( i=1; i<=$user_input; i++ )); do
# Check if the current number is odd or even
if (( i % 2 == 0 )); then
echo "$i is even"
else
echo "$i is odd"
fi
done
This Bash script prompts the user to input a number, checks if the input is a valid positive integer and then uses a “for” loop to iterate through a range from 1 to the user-input number. Within the loop, it checks whether each number is odd or even using a conditional statement and prints the result. The script is a basic example of user input validation and demonstrates the determination of odd and even numbers in a specified range.
Here, all integers from zero to the given input number 5 are checked and echoed as even or odd.
Example 6: Check Variable in For Loop
To check if some variables are present in the bash script use a conditional statement within the “for” loop. This example showcases a bash script that iterates through a numerical range, creating variable names based on the iteration index. Inside the loop, it checks for the existence of these variables using the [[ -v $variable ]]
command. Run the following script to do so:
#!/bin/bash
number_3=10
for i in {1..5}; do
variable="number_$i"
# Checking if the variable exists
if [[ -v $variable ]]; then
echo "$variable exists."
else
echo "$variable does not exist."
fi
done
This bash script uses a “for” loop to iterate through a range of numerical values from 1 to 5. Inside the loop, it creates variables with numerical suffixes, such as number_1, number_2, and so on. The script then checks for the existence of each variable using the [[ -v $variable ]]
command and prints a message indicating whether the variable exists or not.
Here only the number_3 variable exists in the script and that is also shown in the output.
Example 7: Iterate with a Counter
Counter is a variable used to keep track of the number of times a particular event or operation occurs. It is often employed in loops to control the iteration process. The following script initializes a counter variable (count) to 1 and continues the C-styled “for” loop while the counter is less than or equal to 5 showcasing the efficient use of a counter variable in a loop structure:
#!/bin/bash
for ((count=1; count<=5; count++)); do
echo "Iteration $count"
done
This bash script utilizes a “for” loop to iterate through a numerical range from 1 to 5. Within the loop, it echoes a message indicating the current iteration number, denoted by the variable “count”. The loop structure initializes “count” to 1, continues while the count is less than or equal to 5, and increments the count in each iteration.
Here using a C-styled for loop 1 to 5 is counted.
Example 8: Read Variables from a File
Reading and processing data from a text file is important for task automation in Bash scripting. This example showcases a bash script that reads the user’s name and age from a text file using a for loop variable and prints them in a formatted way.
Content of the cat names_and_ages.txt text file:
Alice 25
Bob 30
Charlie 22
Diana 28
Run the following script to read data from a text file in Bash shell:
#!/bin/bash
# Read each line of the text file into an array
lines=($(cat names_and_ages.txt))
# Iterate over the array
for ((i = 0; i < ${#lines[@]}; i += 2)); do
name=${lines[i]}
age=${lines[i + 1]}
echo "Name: $name, Age: $age"
done
This bash script reads each line from a text file named “names_and_ages.txt” into an array named lines. It then uses a for loop to iterate through the array, extract values of name and age from consecutive array elements, and then store them in variables “name” and “age” respectively. Then it echoes a formatted message for each pair displaying the name and age in the specified format.
Here all data was read from the text file and then the formatted data was echoed.
Example 9: Count Lines from Files
The “for” loop can be used to count the number of lines in each file with the same extension within the current directory. The following script uses a “for” loop to iterate through the matching files, echoing a message for each file being processed. Inside the loop, the wc -l
command, coupled with redirection <
, counts and displays the number of lines in the current file. Run the following bash script to count the number of lines in each file with the “.sh” file extension within the current directory:
#!/bin/bash
for file in *.sh
do
echo "Processing file: $file"
line_count=$(wc -l < "$file") # Count lines in each file
echo "This file has $line_count lines."
done
This bash script uses a for loop to iterate through all files in the current directory with a “.sh” extension. For each file, it echoes a message indicating the name of the file being processed and then uses the wc -l
command to count and display the number of lines in that file.
This script goes through all files with the “.sh” extension from the current directory and counts the number of lines of that file.
Example 10: Process All Files in a Directory
Iterating through files within a directory is a fundamental operation often used in shell scripting. The following script utilizes a “for” loop in conjunction with parameter expansion to iterate through the list of files in the current directory. Here $file is an example of parameter expansion. During the execution of the script, the variable “file” undergoes expansion to its current value in each iteration within the “for” loop. Execute the following command to list all files in the current directory:
#!/bin/bash
echo "Files in the current directory:"
for file in *; do
echo "$file"
done
This script begins by echoing a message indicating that it will list files in the current directory. It then uses a for loop to iterate through all files in the current directory echoing each filename in a new line. This also shows all hidden files in the current directory.
This script shows all files including the hidden ones from the current directory into the terminal.
Example 11: List All Running Processes
Knowing the active processes proves essential for system monitoring and management. This example showcases a technique that leverages the ps, awk, and tail commands within a for loop to generate a comprehensive list of running processes on a Unix-like system. Execute the following script to list all processes running on the system:
#!/bin/bash
echo "Processes running on the system:"
for process in $(ps aux | awk '{print $11}' | tail -n +2); do
echo "$process"
done
This bash script starts with echoing a message indicating that it will list all the processes running on the system. Then it utilizes a for loop to iterate over the names of processes obtained by running the ps aux
command. The awk
command extracts the 11th column which corresponds to the command or process name. Then the tail -n +2
command skips the header line from the “ps” command’s output. Finally, the script echoes each process name on a new line.
The names of all processes running on my system are shown on the terminal.
Example 12: Multiplication Table (5×10)
A multiplication table is a mathematical table used to define a multiplication operation for an algebraic system. This script showcases a Bash implementation to generate a formatted multiplication table with a size of 5×10 within the shell interface, using nested “for” loops and formatting techniques for visual clarity. Run the following script to create a 5×10 multiplication table:
#!/bin/bash
echo "Multiplication Table:"
# Print header
echo -e " | \c"
for ((i=1; i<=10; i++)); do
printf "%-4s" "$i"
done
echo
# Print separator
echo "-------------------------------------------"
# Print table
for ((i=1; i<=5; i++)); do
echo -n "$i | "
for ((j=1; j<=10; j++)); do
product=$((i * j))
printf "%-4s" "$product"
done
echo
done
This Bash script generates a formatted multiplication table up to 5×10. The script starts by echoing a message indicating that it will display a multiplication table. The header row is then printed with column labels, and a separator line is added for clarity.
The main part of the script utilizes nested for loops to calculate and format the product of each multiplication. The outer loop controls the multiplicand values (i) from 1 to 5, while the inner loop handles the multiplier values (j) from 1 to 10. Each multiplication i*j
product is calculated and echoed on the same line with a space delimiter using the printf
command. The final output will look like a 5×10 multiplication table.
The output shows a 5 by 10 multiplication table with proper alignment.
Conclusion
In conclusion, getting the hang of the variable in “for loop” in Bash opens up a world of possibilities. It makes your scripting adventures more efficient and enjoyable. I hope this article helps you to get some solid idea about how to use variable in bash for loop.
People Also Ask
What is a loop in Bash?
In Bash scripting, a “loop” is a powerful construct that enables the repetitive execution of a series of commands, making it a fundamental element for automation and task iteration. Loops play a crucial role in simplifying and optimizing code, allowing developers to efficiently perform tasks without the need for redundant or excessive manual input.
How do I run a for loop in Linux?
To run a for loop in Linux, use the following simple syntax:
for item in list; do
# Commands to be executed for each item
done
Here, replace “list” with the items you want to iterate over. This loop structure is invaluable for automating repetitive tasks, making it an essential tool in Linux scripting.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial