FUNDAMENTALS A Complete Guide for Beginners
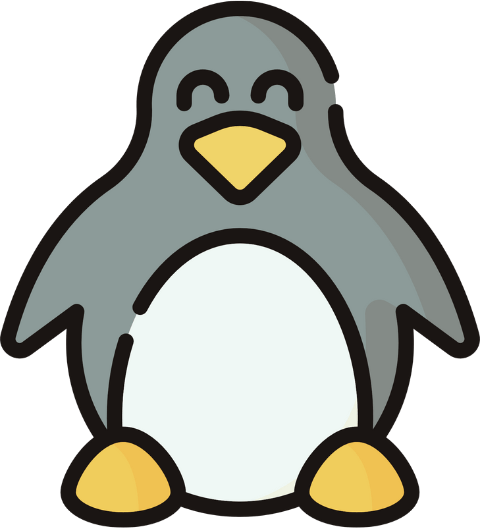
Within the realm of Bash scripting, combining the “for” loop with the “seq” command unlocks robust automation and streamlined numerical manipulation. The “for” loop serves as the iterative engine, powering the execution of tasks across a defined sequence of values. In conjunction, the “seq” command acts as a generator, efficiently creating these numerical sequences within the loop’s framework. This article will go through various Bash scripts showcasing the “for” loop in conjunction with the “seq” command.
What is the “seq” Command in Bash?
The seq command is a specialized tool designed to generate sequences of numbers in Bash scripting. It is structured to create numeric ranges based on user-defined parameters. With seq, users can specify the starting and ending points and, optionally the increment or decrement between numbers in the sequence.
The basic syntax of the “seq” command is as follows:
seq [OPTION]... LAST_VALUE
seq [OPTION]... FIRST_VALUE LAST_VALUE
seq [OPTION]... FIRST_VALUE INCREMENT/DECREMENT LAST_VALUE
Here,
seq: This command is used to generate sequences of numbers.
OPTION: Optional arguments to customize the behavior of the seq command.
FIRST_VALUE: This is the first value in the sequence.
LAST_VALUE: This is the last value in the sequence.
INCREMENT/DECREMENT: This determines how the sequence increments or decrements from the starting value. It can be a positive or negative integer or a floating-point value.
The seq [OPTION]... LAST_VALUE
syntax generates a sequence of numbers starting from 1 and incrementing by 1 until it reaches the specified LAST_VALUE. While the seq [OPTION]... FIRST_VALUE LAST_VALUE
syntax generates a sequence of numbers starting from the provided FIRST_VALUE and incrementing by 1 until it reaches the LAST_VALUE. And the last seq [OPTION]... FIRST_VALUE INCREMENT/DECREMENT LAST_VALUE
generates a sequence starting from FIRST_VALUE and applying the specified INCREMENT/DECREMENT value to generate the sequence until it reaches LAST_VALUE.
10 Examples of Using Bash “for” Loop with “seq” Command
Mastering the for loop and the “seq” command in Bash scripting enables efficient automation and numerical manipulation. This article explores their versatile applications together. This article demonstrates 10 unique practical examples showcasing the alliance between these two components.
1. Iterating Over Sequential Numbers
The combination of the Bash ‘for’ loop and the ‘seq’ command enables efficient iteration over sequential numbers. The following script showcases how to create sequential numbers starting from 1 up to the specified end value:
#!/bin/bash
# Displaying Sequential Numbers
echo "Displaying Sequential Numbers:"
for i in $(seq 10); do
echo $i
done
This script iterates through sequential numbers from 1 to 10 using a Bash “for” loop in conjunction with the “seq” command. By omitting the starting value in the “seq” command, it defaults to 1, incrementing until the specified end value is reached.
Here, 1 to 10 is echoed using just the end value 10.
2. Sequential Number Ranges
The Bash ‘for’ loop together with the ‘seq’ command efficiently iterates through sequential numbers within a specified range, facilitating various scripting tasks with ease. This script demonstrates how to iterate from 1 to 10, printing each number on a new line:
#!/bin/bash
# Iterating from 1 to 10
echo "Iterating from 1 to 10:"
for i in $(seq 1 10); do
echo $i
done
This Bash script begins by displaying a descriptive message “Iterating from 1 to 10”. It then enters a for loop that utilizes the seq command to generate a sequence of numbers from 1 to 10. During each iteration of the loop, the script echoes the current number, printing each number from 1 to 10 on a new line.
Here, a sequence of 1 to 10 is echoed using the seq command.
3. Reverse Sequential Number Ranges
With the Bash ‘for’ loop and the ‘seq’ command, iterating through sequential numbers in reverse order becomes a seamless process. The following script showcases iteration from 10 to 1 with a step size of 2:
#!/bin/bash
# Iterating from 10 to 1
echo "Iterating from 10 to 1:"
for i in $(seq 10 -2 1); do
echo $i
done
This Bash script starts by displaying the message “Iterating from 10 to 1”. It then enters a for loop using the seq command, generating a sequence of numbers starting from 10 and decrementing by 2 until it reaches 1. During each iteration, the script echoes the current number, resulting in the printing of numbers from 10 to 1 with a step size of 2.
Here, all even numbers are printed from 10 to 1 in reverse order.
4. Step-based Iteration
The ‘for’ loop coupled with the ‘seq’ command allows for precise step-based iteration through numerical ranges, enhancing the control and efficiency of Bash scripts. This script iterates from 51 to 100 in steps of 10, prints ASCII characters, and then prints floating-point numbers:
#!/bin/bash
# Iterating from 51 to 100 in steps of 10
echo "Iterating from 51 to 100 in steps of 10:"
for i in $(seq 51 10 100); do
echo $i
done
# Printing ASCII characters
echo "Printing ASCII characters:"
for i in $(seq 97 2 103); do
echo $i | awk '{ printf "%c\n", $1 }';
done
# Printing floating-point numbers
echo "Printing floating-point numbers:"
for i in $(seq .5 .1 .8); do
echo $i;
done
This Bash script performs several tasks sequentially. Firstly, it displays the message “Iterating from 51 to 100 in steps of 10”, indicating the range it will iterate through. It then enters a for loop utilizing the “seq” command to generate a sequence from 51 to 100 in increments of 10, printing each number within this range.
Following this, it prints ASCII characters by iterating from 97 to 103 with a step size of 2, converting each numerical value to its corresponding ASCII character using the “awk” command. The awk command is a powerful text processing tool primarily used for pattern scanning and text processing.
Lastly, the script prints floating-point numbers ranging from 0.5 to 0.8 with increments of 0.1.
Here, the first sequence of 51 to 100 is printed with a step size of 10. Then ASCII characters are echoed from their equivalent ASCII number. Lastly, a floating point sequence is generated.
5. Array Element
Utilizing the ‘seq’ command within the ‘for’ loop simplifies sequential array element access, streamlining array manipulation tasks in Bash scripts. The following script displays indices and elements of an array using the ‘seq’ command:
#!/bin/bash
fruits=("Apple" "Banana" "Orange" "Grapes")
for i in $(seq 0 $((${#fruits[@]} - 1))); do
echo "$i : ${fruits[$i]}"
done
This Bash script utilizes the “seq” command within a for loop to generate a sequence of numbers representing the indices of the fruits array. The “seq” command generates a sequence starting from 0 and ending at the length of the array minus 1, ensuring that the loop iterates over each index of the array. This sequence of numbers determines the indices accessed during each iteration of the loop, allowing the script to access and print the corresponding elements of the fruits array.
Here, array elements are echoed using the seq command.
6. Combined Sequences
Integrating multiple sequences within the ‘for’ loop using the ‘seq’ command empowers Bash scripts to generate complex numerical patterns efficiently. The following script combines two sequences to create a unique pattern:
#!/bin/bash
# Combined Sequences
echo "Combined Sequences:"
for i in $(seq 0 5 25; seq 50 5 75); do
echo $i
done
This Bash script first prints the message “Combined Sequences”. It then utilizes the ‘seq’ command twice within a single ‘for’ loop to generate two distinct sequences: one starting from 0 and incrementing by 5 until reaching 25, and another starting from 50 and incrementing by 5 until reaching 75. During each iteration of the loop, the script prints the current value of the combined sequence, displaying numbers from both sequences sequentially.
Here, 2 separate sequences are merged.
7. Floating-Point Sequence
With the ‘seq’ command and the ‘for’ loop, Bash scripts can generate precise floating-point sequences, facilitating accurate numerical calculations. This script demonstrates the creation of a floating-point sequence using the -f
option, which allows for precise control over the output format of the sequence:
#!/bin/bash
echo "Floating-Point Sequence:"
for i in $(seq -f '%f' 5); do
echo $i;
done
This Bash script begins by displaying the message “Floating-Point Sequence”. It utilizes the seq command with the -f
option to generate a sequence of floating-point numbers starting from 1 and ending at 5. During each iteration of the loop, the script prints the current floating-point number, allowing for precise control over the output format of the sequence.
Here, a sequence with the floating value is echoed.
8. Leading Zero Sequence
The -w
option of the ‘seq’ command simplifies the generation of sequences with leading zeros, ensuring consistent formatting and presentation of numerical data. The following script generates a sequence with leading zeros using the -w
option:
#!/bin/bash
echo "Leading Zero Sequence:"
for i in $(seq -w 5 4 15); do
echo $i;
done
This Bash script starts by printing the message “Leading Zero Sequence”. It employs the seq command with the -w
option to generate a sequence with leading zeros. The sequence begins from 05 and increments by 4 until it reaches 15. During each iteration, the script prints the current value of the sequence, ensuring that numbers with leading zeros are displayed consistently.
Here, a seq is generated with the leading zero.
9. Filenames with Sequence
Integrating the ‘seq’ command into filename generation processes enables Bash scripts to dynamically create and manage filenames with sequential numbering, enhancing file management capabilities. This script creates filenames with a sequential numbering pattern using the -s
option to specify the separator between numbers:
#!/bin/bash
echo "Filenames with Sequence:"
for i in logfile-$( seq -s '.log logfile-' 3 ).log; do
echo -n "$i; ";
done
echo
This Bash script starts by displaying the message “Filenames with Sequence”. It utilizes the seq command within a command substitution $(...)
to generate a sequence of numbers separated by ‘.log logfile-‘. The generated sequence is then used to create filenames with the format ‘logfile-n.log’, where ‘n’ is each number in the sequence. Finally, during each iteration of the loop, the script prints the generated filenames separated by semicolons.
Here, file names are generated using the seq command.
10. Hexadecimal Sequence
The ‘seq’ command, when combined with the ‘for’ loop, enables Bash scripts to process hexadecimal sequences effortlessly, facilitating tasks involving hexadecimal numerical representation. The following script generates a sequence using the hexadecimal numbers using the “seq” command:
#!/bin/bash
echo "Hexadecimal Sequence:"
for i in $( seq 0x2B 0xA 0x6C ); do
echo -n "$i; ";
done
echo
This Bash script starts by printing the message “Hexadecimal Sequence”. It utilizes the seq command to generate a sequence of hexadecimal numbers starting from 0x2B and incrementing by 0xA until it reaches 0x6C. During each iteration of the loop, the script prints the current hexadecimal number followed by a semicolon, displaying the sequence of hexadecimal values.
Here, a sequence is generated using hexadecimal numbers.
Practice Tasks on Bash “for” Loop with “seq” Command
If you aim to be better at using the “seq” command in the “for” loop then you can try solving the following problems using the concept discussed in this article:
- Write a Bash script that uses a “for” loop and the “seq” command to print sequential numbers from 1 to 10.
- Create a Bash script that prints numbers from 10 to 1 in reverse order using the “seq” command and a “for” loop.
- Create a Bash script that prints multiples of 3 from 3 to 30 using the “seq” command and a “for” loop.
- Write a Bash script to calculate the factorial of a given number using the “seq” command and a “for” loop.
- Implement a Bash script to print all prime numbers between 1 and 100 using the “seq” command and a “for” loop.
Conclusion
Exploring the Bash “for” loop and the “seq” command opens up a world of possibilities in script automation. By understanding how to leverage these tools effectively, you can streamline your workflow and tackle complex tasks with ease. I hope you find this article helpful in your Bash scripting journey.
People Also Ask
How do I create a sequence of numbers in Bash?
To create a sequence of numbers in bash you can use the seq command which is specifically designed for generating sequences. The basic syntax of the seq command is seq [OPTION]... LAST_VALUE
, where you specify the starting and ending points of the sequence. Additionally, you can include an increment or decrement between numbers by using the syntax seq [OPTION]... FIRST_VALUE INCREMENT/DECREMENT LAST_VALUE
. This command is versatile and allows you to generate sequences of numbers efficiently for various scripting tasks in the bash environment.
How can a for loop be used to iterate over a range of numbers in a bash script?
To iterate over a range of numbers in a bash script, a for loop can be utilized with the seq command. The seq command generates a number sequence for the for loop to iterate over. Users specify the starting and ending points and determine how the sequence progresses. This functionality enables the for loop to navigate through numbers and perform tasks accordingly. This approach provides a flexible and efficient method for performing repetitive tasks involving numerical sequences in bash scripting.
How do you specify a range in Linux?
To specify a range in linux, you can use various commands and syntax depending on the context. For numerical ranges, the seq command is commonly used. You can generate number sequences by setting the start and end values and optionally the step between numbers. These sequences are useful for various tasks, including data processing or file operations. Commands like grep, awk, or sed also support range specifications for filtering or manipulating data.
What is the seq command in the for loop?
The seq command in the for loop is a Bash utility used to generate sequences of numbers. It is designed to create numeric ranges based on specified parameters. The seq command helps define the start and end of a sequence, along with the step between numbers. It’s handy for looping through number ranges in bash scripts, making tasks with numerical sequences easier to automate. Using seq simplifies iterating through numbers in bash scripts.
What is a for loop in bash?
A for loop is a control structure in bash scripting that allows for repetitive execution of a block of commands based on a specified list of items. It iterates through each item in the list and executes the commands within the loop for each item. A for loop typically contains the keyword “for” and a variable that represents items in the list. It also includes the list itself and commands to execute within the loop. It can iterate through numbers, filenames, or array elements, offering a powerful automation tool in bash scripts.
How to create an array in Bash?
To create an array in Bash, you declare a variable and assign values to it within parentheses, separated by spaces. For example myArray=(“apple” “banana” “orange”)
. You can access individual elements using their index, starting from zero: echo myArray[0]
. Arrays in Bash allow you to store and manipulate collections of data efficiently within your scripts.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial