FUNDAMENTALS A Complete Guide for Beginners
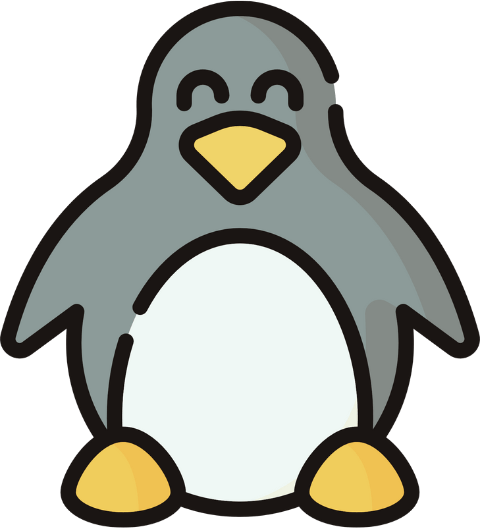
Bash scripting provides a variety of loops, and the “for” loop stands out as a versatile tool for automating tasks. It iterates over a sequence of values, executing a block of code for each iteration. One particularly useful feature in Bash scripting is the ability to leverage ranges within the “for” loop, offering a convenient way to iterate over a specific set of values. This article will dive into the various methods of utilizing range in the Bash “for” loop.
5 Methods of Using Bash “for” Loop with Range
Bash scripting offers a variety of methods for utilizing the for loop, each with its unique advantages. Whether you prefer the concise brace expansion, the versatility of the seq command, the clarity of C-style syntax, the dynamism of eval with brace expansion, or the simplicity of looping over array elements, Bash provides a solution for every scripting requirement. This guide will go through 5 different methods of using the range in the bash for loop.
1. Using Brace Expansion
Brace expansion is a powerful feature in Bash that lets you generate lists of filenames, numbers, or other text patterns within your commands. It provides a concise and readable syntax for generating sequences within loops. This feature simplifies the process of iterating through ranges of values, making scripts more efficient and easier to understand.
The basic syntax of a for loop with brace expansions is as follows:
for i in {START..END}
for i in {START..END..INCREMENT/DECREMENT}
The following example showcases various types of bash ranges using brace expansion:
#!/bin/bash
echo "Default Increment"
for i in {1..5}
do
echo "$i"
done
echo "Increment"
for i in {1..10..3}
do
echo "$i"
done
echo "Decrement"
for i in {10..1..3}
do
echo "$i"
done
This script utilizes brace expansion to create three distinct loops, each demonstrating different behaviors regarding incrementation and decrementation. It starts with the “Default Increment” loop, using the range {1..5}
for a default increment of 1, echoing values 1 to 5. The subsequent “Increment” loop uses {1..10..3}
to skip every two values, echoing “1 4 7 10”. Lastly, the “Decrement” loop echoes “10 7 4 1” using {10..1..3}
, demonstrating a reverse order with a customized decrement.
Here, using the brace expansion first, 1-5 are echoed using default increment. Then from 1 to 10, the numbers are printed with an increment of 3. After that, the same thing is done in a reverse order with a decrement of 3.
2. Using the “seq” Command
The seq command in Bash is a specialized tool for generating sequences of numbers. It simplifies the process of defining ranges with specific start, end, and increment/decrement values, providing more control over the iteration.
To use the seq command to create ranges in the for loop, execute the following example:
#!/bin/bash
echo "Default Increment"
for i in $(seq 1 5)
do
echo "$i"
done
echo "Increment"
for i in $(seq 1 3 10)
do
echo "$i"
done
echo "Decrement"
for i in $(seq 10 -3 1)
do
echo "$i"
done
This script demonstrates the versatility of the seq command in generating sequences with different increments and decrements. It starts with “Default Increment”, echoing values from 1 to 5. The “Increment” loop skips every two values, echoing “1 4 7 10”. The “Decrement” loop counts down from 10 to 1, echoing “10 7 4 1”.
Here, using the seq command 1 to 5 are echoed using default increment, 1 to 10 are echoed with an increment of 3, and 10 to 1 are echoed with a decrement of 3.
3. Using C-style “for” Loop Syntax
Bash scripts offer versatility in implementing loops, including the adoption of C-style for loop syntax. This syntax for (( initialization; condition; update ))
provides a concise and powerful way to define loops with precise control over the iteration process.
To create a range using the C style for loop, follow the script below:
#!/bin/bash
echo "Post Increment"
for (( i = 1; i <= 3; i++ ))
do
echo "$i"
done
echo "Pre Increment"
for (( i = 1; i <= 3; ++i ))
do
echo "$i"
done
echo "Increment by 3"
for (( i = 1; i <= 10; i += 3 ))
do
echo "$i"
done
echo "Decrement by 3"
for (( i = 10; i >= 1; i -= 3 ))
do
echo "$i"
done
This Bash script illustrates various C-style for loop scenarios. In the “Post Increment” section, a loop initializes i to 1 and echoes values until i reaches 5 using the post-increment operator (i++
). The “Pre Increment” section functions similarly but with the pre-increment operator (++i
). Both sections produce the output “1 2 3”.
Moving to “Increment by 3“, the script initializes i to 1 and increments it by 3 in each iteration until i is no longer less than or equal to 10, resulting in the output “1 4 7 10”. In the final “Decrement by 3” section, the script initializes i to 10 and decrements it by 3 in each iteration, echoing “10 7 4 1” until i is less than 1.
Here, using C style for loop first, 1-3 are echoed using post-increment and pre-increment. Then 1 to 10 are echoed with an increment of 3 and the reverse is echoed with a decrement of 3.
4. Using the “eval” Command with Brace Expansion
The eval command is a powerful tool that lets you dynamically execute code by treating a string as a Bash command. Bash scripting provides flexibility to integrate the eval command in conjunction with the brace expansion. This combination enables the dynamic generation of sequences and variable-based range definitions within loops.
The basic syntax of using the eval command with brace expansion to create a bash range is as shown below:
for i in $(eval echo {START..END})
for i in $(eval echo {START..END..INCREMENT/DECREMENT})
The following example showcases various types of bash ranges using the eval command in conjunction with the brace expansion:
#!/bin/bash
# Dynamic Range Generation
start_value=2
end_value=7
echo "Dynamic Range Generation:"
for i in $(eval echo "{$start_value..$end_value}")
do
echo "$i"
done
# Custom Increment/Decrement
start_value=2
end_value=20
step_size=4
echo "Looping with custom increment:"
for i in $(eval echo "{$start_value..$end_value..$step_size}")
do
echo "$i"
done
This Bash script showcases the flexibility of eval with brace expansion for dynamic range generation and custom increment/decrement loops. In the first part, it dynamically generates and echoes a sequence from 2 to 7. The second section demonstrates custom incrementing, generating a sequence from 2 to 20 with a step size of 4.
Here the eval command is used to dynamically print 2 to 7 and then print 2 to 18 with a custom increment of 4.
5. Looping Over Array Elements
An array is a powerful data structure that offers a way to group and manage multiple values under a single name. Iterating over the elements of an array is a common operation in bash scripting.
To understand how to use arrays to create ranges go through the script below:
#!/bin/bash
# Define an array
fruits=("Apple" "Banana" "Orange" "Grapes")
# Loop to iterate over array elements
echo "Looping over array elements:"
for fruit in "${fruits[@]}"
do
echo "Current fruit: $fruit"
done
This Bash script demonstrates a straightforward way to iterate over the elements of an array named “fruits”. The array is pre-defined with four elements, representing different types of fruits. The script initiates a for loop using the syntax for fruit in "${fruits[@]}"
allowing it to iterate seamlessly over each element in the array. Within the loop, the script echoes the name of the current fruit for each iteration.
Here, an array is created and a for loop is used to iterate over all elements of the array.
Practice Problems on Bash “for” Loop with Range
To sharpen your skill in bash “for” loop with range, try solving the problem using the concept discussed in this article:
- Write a Bash script to print the even numbers between 10 and 20 using a “for” loop.
- Implement a Bash script to calculate and print the square of numbers from 1 to 10 using a “for” loop.
- Write a Bash script to identify and print the prime numbers between 10 and 30 using a “for” loop.
- Create a Bash script to reverse a given string using the “for” loop and print the modified string.
- Write a Bash script to determine if a given number is a palindrome using the “for” loop.
Conclusion
In conclusion, diving into the intricacies of the Bash “for” loop range has uncovered a realm of possibilities for efficient and dynamic scripting. The range, whether achieved through brace expansion, seq command, C-style syntax, or dynamic eval with brace expansion, provides scripters with a toolkit to address a wide spectrum of scenarios. I hope you find this article helpful.
People Also Ask
How to use variables in for loop Bash?
To use variables in a Bash “for” loop, you can employ various methods, such as brace expansion, seq command, C-style syntax, and dynamic eval with brace expansion. Following is a basic syntax for using a variable in the for loop:
for variable in {start..end..increment}
do
# Your code here using $variable
done
Here, replace start, end, and increment with the appropriate values for your loop. The variable $variable
takes on each value in the specified range during each iteration of the loop.
How to iterate through numbers in Bash?
To iterate through numbers using a “for” loop in bash use the basic syntax as follows:
for num in "${numbers[@]}"; do
# Your code here, reference the current number as $num
done
Within the loop, you can perform various operations or execute commands using the current number represented by the variable $num
. This approach is particularly useful when you have a predefined list of numbers stored in an array.
What is a loop variable?
A loop variable is a variable used within the context of a programming loop, such as a “for” or “while” loop. It serves as a counter or iterator that takes on different values during each iteration of the loop. The loop variable is often utilized to control the flow of the loop, determining how many times the loop should execute or specifying the range of values it should iterate over.
How to do math in Bash script?
In Bash scripting, performing mathematical operations involves using arithmetic expressions. These expressions allow you to carry out various mathematical calculations, such as addition, subtraction, multiplication, and division, directly within your script. To initiate a mathematical operation, you can utilize the arithmetic expansion feature using the double parentheses (( ))
or the let command, the expr command, or the bc command. For example, to add two numbers, you can use result=$((num1 + num2))
command or let result=num1+num2
command or result=$(expr $num1 + $num2)
or result=$(echo "$num1 + $num2" | bc)
command.
How to calculate time in a bash script?
To calculate the execution time of a bash script, you can use the date command. To do this first encapsulate your script between start_time=$(date +"%s")
and end_time=$(date +"%s")
commands. Then using the elapsed_time=$((end_time - start_time))
command the elapsed time is calculated. Then you can echo the value of the elapsed_time
variable into the terminal.
What is for loop syntax?
In Bash, the syntax for a basic “for” loop is straightforward. Here is the basic syntax:
for variable in sequence
do
# Commands to be performed in each iteration
done
You can use different types of sequences, such as ranges, arrays, or command substitutions, providing flexibility to the “for” loop. The commands or actions inside the loop block are executed for each iteration, with the loop variable taking on the values from the specified sequence.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial