FUNDAMENTALS A Complete Guide for Beginners
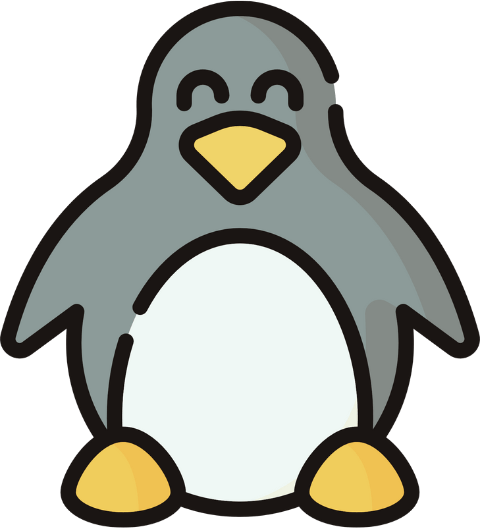
Looping through arrays is essential for processing data efficiently in Bash scripting. Arrays provide a structured way to organize and manage data. The “for” loop offers a simple yet powerful mechanism for iterating through array elements. This article will discuss how to use the “for” loop in Bash scripting to iterate over array elements.
7 Methods of Iterating Through Bash Array Using “for” Loop
Unlocking the potential of the bash array using the for loop opens up a world of possibilities in script automation. From simple iteration of array elements to more advanced techniques like accessing elements by index and traversing multiple arrays simultaneously, this article covers 7 different array manipulation strategies in Bash scripting.
1. Simple Iteration of Array Elements
Iterating over arrays is a fundamental operation for processing array elements in bash scripting. The following script demonstrates a basic iteration process applied to an array named ‘fruits’ using array expansion. Array expansion in Bash scripting refers to the process of accessing or referencing all the elements within an array. Here is the script to do so:
#!/bin/bash
fruits=("apple" "banana" "orange" "grape")
for fruit in "${fruits[@]}"; do
echo "fruit: $fruit"
done
This script uses a for loop to iterate over each element in the fruits
array. During each iteration, the loop assigns the current element to the variable fruit
, and the script echoes the value of fruit
preceded by the text “fruit:”. The loop continues until it has processed all elements in the fruits
array, printing each fruit name one by one.
Here a basic for loop echoes all elements of an array.
2. Using a User-Defined Function with Variable Arguments
Using user-defined functions with variable arguments is a versatile technique for handling array elements dynamically. This script showcases the utilization of such a function named Loop
to iterate over an array named fruits
using a straightforward for loop. As the function iterates over each argument, it echoes the value to the console. Here is the script to demonstrate this functionality:
#!/bin/bash
fruits=("apple" "banana" "orange" "grape")
Loop(){
for fruit in ${*} ;
do
echo ${fruit}
done
}
Loop ${fruits[*]}
This Bash script defines an array called fruits
containing four elements. It then defines a function named Loop
that takes arguments representing elements of an array. Within the function, a for loop iterates over each argument passed to it, assigning each one to a variable named fruit
. During each iteration, the script echoes the value of fruit
to the console. Finally, the script calls the Loop
function and passes the elements of the fruits
array to it, causing the function to iterate over each fruit and print its name.
Here the user-defined function iterates over the elements of an array and echoes them into the terminal.
3. Accessing Elements by Index Using Positional Numbers
In bash scripting, accessing array elements by their index positions is a common task for array manipulation. The provided script showcases this approach by utilizing a for loop to iterate over the indices of the fruits
array. Using the syntax ${!fruits[@]}
enables the script to access each index position dynamically and allows direct mapping between the index and the array element. Here’s how:
#!/bin/bash
fruits=("apple" "banana" "orange" "grape")
for i in ${!fruits[@]}; do
echo "element $i is ${fruits[$i]}"
done
This Bash script uses a for loop to iterate over the indices of the fruits
array using the ${!fruits[@]}
syntax. During each iteration, the index i
is assigned a value corresponding to the position of the element within the array. Within the loop, the script echoes the index i
along with the corresponding element from the fruits
array using the syntax ${fruits[$i]}
.
Here array elements are echoed with their corresponding index number.
4. Traditional C-Style “for” Loop
In bash scripting, the traditional C-style for loop offers an alternative method for iterating over array elements. This script demonstrates how to leverage this loop style to efficiently iterate through an array named fruits
:
#!/bin/bash
fruits=("apple" "banana" "orange" "grape")
for (( i=0; i<${#fruits[@]}; i++ )); do
echo ${fruits[$i]}
done
This Bash script utilizes a C-style for loop to iterate over the indices of the fruits
array. The loop initializes an index variable i
to 0 and iterates as long as i
is less than the length of the fruits
array. During each iteration, the script echoes the element of the fruits
array at the current index i
using the syntax ${fruits[$i]}
.
Here a C-style for loop iterates over all elements of an array and prints them into the terminal using the echo command.
5. Iterating Over Multiple Arrays Simultaneously
Simultaneously iterating over multiple arrays is a useful technique in bash scripting for coordinating related data stored across different arrays. The following script initializes 2 arrays fruits
and colors
each containing 4 elements. It iterates over the indices of the fruits
array and echoes the element at each index, followed by the corresponding element from the colors
array. Here is the script to do so:
#!/bin/bash
fruits=("apple" "banana" "orange" "grape")
colors=("red" "yellow" "orange" "green")
for (( i=0; i<${#fruits[@]}; i++ )); do
echo ${fruits[$i]} ${colors[$i]}
done
This script uses a C-style for loop to iterate over the indices of the fruits
array. Each iteration echoes the element of the fruits
array at the current index followed by the corresponding element from the color
array. This loop continues until the index i
reaches the length of the fruits
array. As a result, the script prints each fruit-color pair in a new line, associating each fruit with its respective color.
Here elements of 2 arrays are echoed simultaneously.
6. Looping Through Key-Value Pairs in an Associative Array
An associative array is a collection of key-value pairs where each key is unique and associated with a specific value. Looping through an associative array is a crucial task in bash scripting for processing organized data. The following script starts with defining an associative array and then utilizes a for loop to iterate over the key-value pair of the array. Here is the script to iterate over an associative array using a for loop:
#!/bin/bash
declare -A fruits
fruits["apple"]="red"
fruits["banana"]="yellow"
fruits["orange"]="orange"
fruits["grape"]="green"
for fruit in "${!fruits[@]}"
do
echo "The $fruit is ${fruits[$fruit]}"
done
This script starts with defining an associative array named fruits
and then assign key-value pairs to it, where each fruit is associated with a color. It then uses a for loop to iterate over the keys of the fruits
array. During each iteration, it prints a statement indicating the fruit name followed by its corresponding color. This loop continues until all key-value pairs in the fruits
array have been processed.
Here key-value pairs of the associative array “fruits” are echoed in a formatted way.
7. Reading Elements from a File into an Array
Reading elements from a file into an array is a common task in bash scripting for processing data from an external source. The following demonstrates how to read elements of a text file in an array and iterate over them using the mapfile command. The mapfile command in Bash is used to read lines from standard input or a file into an array variable.
The content of the fruits.txt text file is as follows:
apple red
banana yellow
orange orange
grape green
Execute the following script to read the elements of a text file and iterate through them:
#!/bin/bash
mapfile -t fruits < fruits.txt
count=1
for line in "${fruits[@]}"
do
echo "line $count $line"
((count++))
done
This bash script starts with reading the contents of a file named “fruits.txt” into an array named fruits
using the mapfile command. It reads lines sequentially and assigns each line as an element of the array variable. It then initializes a counter variable “count” to keep track of the line number.
Next, it iterates over each element in the fruits
array using a for loop. During each iteration, it prints the line number followed by the content of the line. After processing all lines in the array, the script outputs the line number and the corresponding content for each line of the “fruits.txt” file.
Here all lines of the “fruits.txt” file are echoed in a new line in the terminal.
Practice Tasks on Bash “for” Loop Array.
If you aim to be better at using the “for” loop to iterate over an array, then you can try solving the following problems using the concept discussed in this article:
- Create a script that declares an array of numbers and calculates the sum of all elements using a for loop.
- Write a script that initializes an array of numbers and uses a for loop to print only the even numbers from the array.
- Develop a script that takes an array of strings as input and prints the elements in reverse order using a for loop.
- Create a script that prompts the user to enter a number and checks if it exists in an array of numbers using a for loop.
- Write a script that sorts an array of integers in ascending order using a simple sorting algorithm implemented with a for loop.
Conclusion
Understanding the intricacies of looping through arrays using the “for” loop in Bash is paramount for effective script development. Whether you’re a novice or an experienced scripter I hope these array-looping techniques discussed in this article will enhance your bash scripting journey.
People Also Ask
What is an Array in Bash Scripting?
An array is a collection of elements stored under a single variable. To declare an array use syntax; array_name=(element1 element2 element3 ...)
. In bash scripting, the arrays are not typed, which means they can hold elements of different types such as strings, integers, or even other arrays.
Arrays in bash are indexed and the first element is started from zero. All individual elements of an array can be accessed using their index. Bash also supports associative arrays, where elements can be accessed using keys instead of numerical indices.
What is for loop in Bash?
The for loop in Bash scripting is a control flow statement that iterates over a sequence of elements. It allows you to execute a set of commands repeatedly until a specified condition is met. In Bash scripting, the for loop is often used to iterate over arrays, files, or ranges of numbers. With its simple syntax and versatility, the for loop is a fundamental tool for automating tasks and processing data in Bash scripts. It enables users to perform repetitive actions efficiently, making it a cornerstone of Bash scripting for automation and data manipulation.
Can for loop iterate over arrays?
Yes, for loop in Bash can iterate over arrays. By using the ${array[@]}
syntax within the for loop, each element of the array can be accessed and processed sequentially. This feature enables efficient handling of array elements in Bash scripts, allowing tasks to be performed on each element individually. Therefore, for loops serve as a fundamental tool for array manipulation and iteration in Bash scripting.
How do I create an array of strings in Bash?
To create an array of strings in Bash, you can use the following syntax: array_name=(element1 element2 element3 ...)
. Each element is separated by a space within the parentheses. This declaration initializes the array with the specified elements. For example, fruits=(“apple” “banana” “orange” “grape”) create an array named “fruits” containing four string elements. This method allows you to store multiple strings within a single variable, facilitating efficient data management in Bash scripting.
How to use declare in Bash?
In Bash scripting, the declare command is used to explicitly declare variables, including arrays. The basic syntax of the declare command is declare options variable_name
. It allows you to define variables with specific attributes, such as declaring an array with the -a option. For instance, declare -a fruits declares an array variable named “fruits”. Using declare helps enforce variable types and enables additional functionality like setting attributes for variables.
How to iterate over a list of strings in the Bash shell script?
To iterate over a list of strings in a shell script, you can use a for loop. The basic syntax for a for loop in shell scripting is for variable in list; do commands; done
. To iterate over a list of strings, you replace “list” with the array or list variable containing the strings you want to iterate over. Within the loop, you can perform actions or execute commands using the current string stored in the loop variable.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial