FUNDAMENTALS A Complete Guide for Beginners
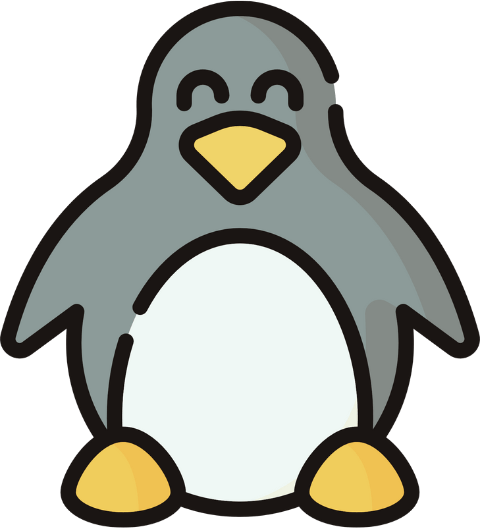
The for loop in Bash is a fundamental control structure that allows users to iterate over a list of values and perform a set of commands for each iteration. Incorporating for loop in file operation makes the file management task more fluent and effortless. In this article, I will explore for loop in Bash files. So without further delay, let’s get started!
9 Practical Examples of “for” Loop in Bash Files
For loop can do various kinds of file operations, including going through a list of files and printing the content on the terminal, checking the existence of files, printing the content of files line by line or word by word separately, recursively printing all files inside folders and subfolders of a specific directory, printing all files including hidden files of a specific directory. In this section, I will show you some practical examples of for-loop operations on the bash files.
1. “for” Loop Through All Files
For loop iterates through a predefined list. If a list of files is passed through a loop, then any kind of processing can be done on each file utilising for loop. In this section, a bash script will be developed to go through a list of files and print the content of each file. The bash script is below:
#!/bin/bash
for f in file1.txt file2.txt file3.txt
do
echo "Processing $f file..."
cat "$f"
done
The for f in file1.txt file2.txt file3.txt
command initiates the for loop, which iterates through file1.txt file2.txt, and file3.txt and prints the content of each file on the terminal.
2. Check if the Files Exist in Bash
Before starting any file operation, it is a good practice to check its existence. It will help the user avoid the execution error in case the file does not exist on the system. In this section, a bash script will be developed to check the existence of three files and then copy them to a destination directory. Here’s how:
#!/bin/bash
for f in file1.txt file2.txt file3.txt
do
#Check if the FILE exists and is a regular file, and then only copy it
if [ -f "$f" ]
then
echo "Copying $f file..."
cp -f "$f" destination
else
echo "Warning: Some problem with \"$f\""
fi
done
The for loop iterates through the file1.txt, file2.txt file3.txt and stores the file to the f variable. Then if [ -f "$f" ]
checks whether the f contains any value. If so, then the cp -f "$f"
destination command will forcefully copy the file to the destination folder. If the f variable does not contain any file, it will print an error message.
3. Processing Command Line Arguments
The for loop can handle command line arguments and do necessary processes according to the specified command. This section will develop a script for removing the files passed to the bash script as command line arguments. Here, the removal task will be achieved by using the rm command with a for loop.
The bash script is below to process command line arguments:
#!/bin/bash
for f in "$@"
do
echo "Removing $f file..."
rm "$f"
done
The for f in "$@"
command starts a for loop, which iterates over the arguments passed to the bash script. The f variable is used to represent each argument in each iteration of the loop. Then, the rm "$f"
command will remove each file, printing a message on the terminal.
4. Using a “for” Loop with “cat” Command
When dealing with files containing numerous lines, it is a good approach to read the files line by line. It will make it easier to extract the important information from the line correctly. To achieve this, one of the best practices is using the cat command to make a list of all words of file1.txt and looping through the list of words using a for loop.
Here is a bash script to print a file line by line:
#!/bin/bash
for line in $(cat file1.txt)
do
echo "$line"
done
The command for line in $(cat file1.txt)
starts a for loop that iterates over the lines of the file1.txt file. The $(cat file1.txt)
command substitution is used to read the contents of file1.txt and provide them as a list of words to the for loop. The variable line is used to represent each line in each iteration of the loop. The echo "$line"
prints the current value of the line to the terminal.
5. Looping Through Words with ‘IFS’
Important information containing files is prone to error while reading files as they store tons of data. One of the safest options to read them is to read each word separately. This can be achieved by setting the Internal Field Separator(IFS) and then looping through the file using a for loop. The IFS splits the words of the files in any context.
Run the script below for looping through each word of a file:
#!/bin/bash
filename="file1.txt"
IFS=$' \t\n'
for word in $(cat "$filename")
do
echo "$word"
done
The filename="file1.txt"
declares a variable named filename and assigns the value “file1.txt” to it. After that, the IFS=$' \t\n'
command sets the Internal Field Separator(IFS) to include space, tab, and newline characters. This “IFS” is used by the shell for word splitting after expansion and to split lines into fields.
Then the for word in $(cat "$filename")
command starts a for loop that iterates over the words in the file specified by filename. The $(cat "$filename")
command substitution reads the contents of file1.txt and provides them as a list of words to the for loop. The variable word is used to represent each word in each iteration of the loop. Then, the echo "$word"
command prints the current value of the word to the terminal.
6. Using “awk” for Advanced Looping Through File
The configuration files, system files or any kind of data files that contain valuable data should be read and checked carefully. To do this, going through each word separately is one of the reliable steps. This can be easily achieved by using the awk command with a for loop concisely.
Here is the bash script for looping through each word of a file:
#!/bin/bash
filename="file2.txt"
awk '{ for (i=1; i<=NF; i++) print $i }' "$filename"
The filename="file2.txt"
command line declares a variable named ‘filename’ and assigns the value “file2.txt” to it. Then the awk '{ for (i=1; i<=NF; i++) print $i }' "$filename"
command processes the file where for loop iterates over the fields which is word in each line. The NF is a built-in variable in the awk command that represents the number of fields in a line. This for loop prints each field on a new line.
7. Reading a File Line by Line with “for” Loop
Dealing with log files, processing data sets, or parsing configuration files is always hectic, as they contain large lines loaded with various information. Reading files line by line is always preferable to ease this kind of file reading. In such cases, the for loop helps to read files line by line.
Copy the bash script to read a file line by line:
#!/bin/bash
#Define the input file
INFILE=file3.txt
# set the Internal Field Separator to newline
IFS=$'\n'
for LINE in $(cat "$INFILE")
do
echo "$LINE"
done
The INFILE=file3.txt
defines a variable named ‘INFILE’ and assigns the value “file3.txt” to it. Then the IFS=$'\n'
sets the Internal Field Separator (‘IFS’) newline characters. This means that the script will treat each line of the file as a separate entity when processing the input file.
Afterward, the for LINE in $(cat "$INFILE")
starts a for loop that iterates over the lines in the file specified by ‘INFILE’. The $(cat "$INFILE")
command substitution reads the contents of “file3.txt” and provides them as a list of lines to the for loop.
The variable LINE is used to represent each line in each iteration of the loop. Afterward, the echo "$LINE"
line prints the current value of LINE to the terminal. In this case, it will print each line from “file3.txt” as the loop iterates.
8. Loop Through Hidden Files in the Directory in Bash
File management system contains various kinds of files. Before editing or updating any files, programmers need to list all files. In a normal manner, hidden files are out of the listing process. To list all files, including hidden files, utilizing the shopt command will be a great approach.
Here is a bash script to list all files in a directory:
#!/bin/bash
directory="/home/susmit/directory"
shopt -s dotglob
for file in "$directory"/*; do
if [ -f "$file" ]; then
echo "$file"
fi
done
The directory="/home/susmit/directory"
declares a variable named directory and assigns the value "/home/susmit/directory"
to it. Then, the shopt -s dotglob
enables the dotglob option using the shopt command. The dotglob option includes hidden files when searching.
After that, the for file in "$directory"/*;
do starts a for loop that iterates over the file in the specified directory. The wildcard (‘*’) matches all files in the directory, and the loop variable ‘file’ represents each file in each iteration.
The if [ -f "$file" ];
checks if the current value of the file is a regular file. If the file is a regular file, the echo “$file” command will print the file’s path to the terminal.
9. Looping Through Files Recursively
Looping through files recursively helps users investigate files, directories, and subdirectories of the system and do the same operation on them simultaneously, such as renaming, copying, moving, or deleting files. Otherwise, programmers would need to check the existence of files, directories, and subdirectories with each operation. Moreover, it is useful for finding files that match specific criteria or patterns within a directory structure. A straightforward solution is to use a for loop to execute operations on all these files.
Here is a practical bash script to list all files recursively:
#!/bin/bash
directory="/home"
function iterate() {
local dir="$1"
for file in "$dir"/*; do
if [ -f "$file" ]; then
echo "$file"
fi
if [ -d "$file" ]; then
iterate "$file"
fi
done
}
iterate "$directory"
The directory="/home"
sets the variable to the path "/home"
. After that, the function iterate() {
defines a Bash function named ‘iterate’. And local dir="$1"
declares a local variable ‘dir’ and assigns it the value of the first argument passed to the ‘iterate’ function.
Afterwards, the for file in "$dir"/*;
do command starts a ‘for’ loop that iterates over all files in the specified directory ('$dir')
. The '*'
is a wildcard character that matches any file or directory in that location. The if [ -f "$file" ];
then checks if the current item in the loop ('$file')
is a regular file. If the file is regular, then the echo “$file” command prints the file’s path.
After that the if [ -d "$file" ];
then command checks if the current iteration item in the loop (‘$file’) is a directory. If the item is a directory, it recursively calls the ‘iterate’ function with the directory as an argument. This allows the script to explore subdirectories.
Finally, iterate "$directory"
initiates the first call to the ‘iterate’ function with the initial directory specified in the ‘directory’ variable.
Conclusion
In conclusion, the ‘For Loop in Bash’ is a powerful tool for searching, updating, printing, deleting and copying files within the Bash scripting environment. By going through this comprehensive article, you are now equipped with the skill to enhance your scripting capabilities. As you embark on your journey of efficient file operations using for loop, It will open up a world of possibilities for increased productivity.
People Also Ask
What is the structure of for loop in Bash?
The basic syntax or structure of for loop in Bash is:
for <variable name> in <list of item>;
do
<command>
done;
Here, the variable name is the name you specify, and the command will be based on your intention.
What is the function inside the for loop in Bash?
The main function inside the for loop in Bash is doing a task repeatedly. The for loop actually repeats the execution of a command within a bash script for a specific number of times.
What are the 3 main parts of a for loop?
A for loop generally contains 3 main parts. These are:
- Initialization: It initiates the for loop.
- Condition: The condition is checked on every iteration of the for loop.
- Increment or Decrement: It is the increment or decrement of the control variable on each iteration. This control variable controls the execution of the for loop.
Can you loop through a string in Bash?
Yes, you can loop through a string in Bash using a for loop, considering the string as an array of characters and printing each character on the terminal. Here is an example of how to do it:
#!/bin/bash
string="Hello, Bash!"
for ((i=0; i<${#string}; i++)); do
echo "${string:$i:1}"
done
This script will print each character of the string on a new line.
How do I skip a for loop in Bash?
Use the continue command to skip a for loop in Bash. It will skip the subsequent part of the for loop and go to the next iteration. To stop the execution of the loop, use the break command. It will terminate the for loop and execute the commands after the for loop.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial