FUNDAMENTALS A Complete Guide for Beginners
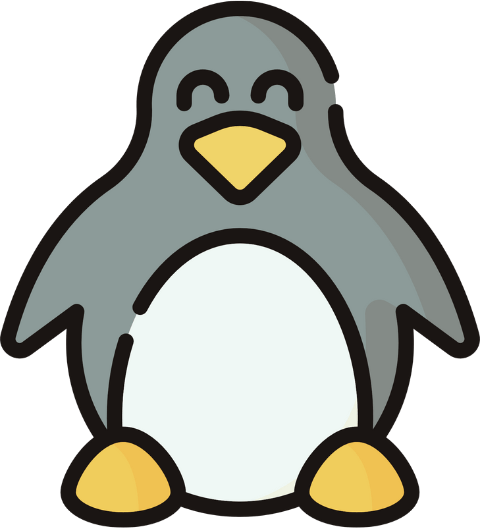
In the realm of bash scripting loop serves as a cornerstone for automating repetitive tasks and streamlining processes. Among these loops, the “for” loop stands as a versatile tool for iterating through a sequence of values. But to truly harness its power, understanding how to increment variables within “for” loops is essential. This unlocks a new dimension of control, allowing you to shape your scripts with precision and tackle complex scenarios with ease. This article dives into various methods for Bash increment and decrement variable in the “for” loop.
Basic Syntax
The basic syntax for incrementing/decrementing variables within a “for” loop involves defining a range of values using {start..end..increment/decrement}
. This concise syntax sets the stage for efficient iteration through a specified range, executing designated commands for each increment. Here is what it looks like in a bash script:
#!/bin/bash
for variable in {start..end..increment/decrement}
do
# Commands to be executed
done
Here:
#!/bin/bash: Indicates that the script should be executed using the Bash shell.
for variable in {start..end..increment/decrement}: Assigns each value of the sequence defined by {start..end..increment/decrement} to the variable for execution within the loop.
do: Indicates the start of the statements that execute in every loop iteration.
# Commands to be executed: These statements will be executed in each loop iteration.
done: Mark the end of the for loop.
10 Methods of Using Increment and Decrement Variables in “for” Loop
In Bash, a for loop is a control flow statement that allows you to iterate over a sequence of values. It is particularly useful for performing a set of commands a specific number of times or for processing elements in a list or array. From the fundamental “for” loop structure to advanced C-style syntax, sequence manipulation, and integration of external commands like “expr”, “let”, and “bc” this guide explores 10 different approaches of incrementing and decrementing variables within Bash’s “for” loop.
1. Basic “for” Loop with Increment and Decrement
Bash’s basic ‘for’ loop serves as a foundational construct for controlled iteration. Utilizing the {1..5}
and {5..1}
syntax for incrementing and decrementing, respectively, this loop demonstrates the simplicity and efficiency of Bash scripting in numerical iterations. The loop iterates through a specified range of numeric values, offering a concise and effective mechanism for executing commands in each iteration.
Here is an example of the basic for loop with increment and decrement:
#!/bin/bash
# Increment
for i in {1..5}; do
echo "Current Value (Increment): $i"
done
# Decrement
for i in {5..1}; do
echo "Current Value (Decrement): $i"
done
The script uses two “for” loops to demonstrate incrementing and decrementing values. The first loop increments the variable i from 1 to 5, echoing each value with the label “Current Value (Increment)” using the echo command. The second loop decrements i from 5 to 1, echoing each value with the label “Current Value (Decrement)”.
Here, this script echoes numbers 1-5 and 5-1 using variable increments and decrements in a basic “for” loop.
2. C-style “for” Loop with Post-increment and Decrement
In C-style programming, post-increment i++
means the current value of the variable is used and then incremented by 1. Post-decrement i--
works similarly but decrements the value. The generic syntax for the C-style “for” loop is for ((init; condition; update))
. This script utilizes post-increment (i++
) and post-decrement (i--
) operations within a C-style “for” loop, providing structured numerical iteration with precise control over the loop variable.
Copy the below script to utilize C-style for loop with post-increment and decrement:
#!/bin/bash
# Post-increment
for ((i=1; i<=5; i++)); do
echo "Current Value (Increment): $i"
done
# Decrement
for ((i=5; i>0; i--)); do
echo "Current Value (Decrement): $i"
done
This script utilizes C-style “for” loops to demonstrate post-increment and decrement operations. The first loop increments i from 1 to 5, echoing each iteration as “Current Value (Increment)”. The second loop decrements i from 5 to 1, echoing each iteration as “Current Value (Decrement)”.
3. C-style “for” Loop with Pre-increment and Decrement
In C-style programming, pre-increment (++variable
) means the variable is incremented by 1, and then its value is used. Pre-decrement (--variable
) works similarly but decrements the value first. The generic syntax for the C-style “for” loop with pre-increment is for ((init; condition; ++variable))
and for decrement, it is for ((init; condition; --variable))
. This script illustrates the use of pre-increment and pre-decrement operations in a C-style loop structure, offering fine-grained control over the loop variable.
Run the below script to utilize C-style for loop with pre-increment and decrement:
#!/bin/bash
# Pre-increment
for ((p=1; p<=5; ++p)); do
echo "Current Value (Increment): $p"
done
# Decrement
for ((p=5; p>0; --p)); do
echo "Current Value (Decrement): $p"
done
In this script, C-style “for” loops showcase pre-increment and decrement operations. The first loop pre-increments p from 1 to 5, echoing each iteration as “Current Value (Increment)”. The second loop pre-decrements p from 5 to 1, echoing each iteration as “Current Value (Decrement)”.
4. “for” Loop with Sequence
The “for” loop can iterate through sequences with specified steps. For incrementing or decrementing, the generic syntax is {start..end..increment/decrement}
. This script utilizes {0..10..2}
for incrementing and {10..0..2}
for decrementing to illustrate the flexibility of Bash in handling numerical iterations, achieving precision and control over loop variables.
The below script illustrates variable increment and decrement using sequences:
#!/bin/bash
# Increment
for i in {0..10..2}; do
echo "Current Value (Increment): $i"
done
# Decrement
for i in {10..0..2}; do
echo "Current Value (Decrement): $i"
done
This script features two “for” loops for incrementing and decrementing. The first loop increments i from 0 to 10 with a step of 2, echoing each value as “Current Value (Increment)”. The second loop decrements i from 10 to 0 with a step of 2, echoing each value as “Current Value (Decrement)”.
Here, the script echoes all even numbers from 0 to 10 by using a “for” loop with sequence-based increments and decrements.
5. C-style “for” Loop with Custom Increment and Decrement
The C-style syntax in Bash provides explicit control over loop variables. For incrementing, the generic syntax is for ((init; condition; variable+=step))
, and for decrementing, it is for ((init; condition; variable-=step))
.
This script iterates through a specified range, incrementing and decrementing values with the specified step:
#!/bin/bash
# Increment
for ((j=1; j<=10; j+=2)); do
echo "Current Value (Increment): $j"
done
# Decrement
for ((j=9; j>0; j-=2)); do
echo "Current Value (Decrement): $j"
done
Utilizing C-style syntax, this script showcases incrementing and decrementing j. The first loop increments j from 1 to 10 with a step of 2, echoing each iteration as “Current Value (Increment)”. The second loop decrements j from 9 to 0 with a step of 2, echoing each iteration as “Current Value (Decrement)”.
This script echoes all odd numbers from 1 to 9 using the “for” loop with C-styled syntax.
6. “for” Loop with Sequence of Letters
The “for” loop in Bash is versatile enough to iterate through sequences of letters. For incrementing or decrementing, the generic syntax is {start..end}
. This script demonstrates the loop’s flexibility in handling sequences of letters, both incrementing and decrementing:
#!/bin/bash
# Increment
for letter in {a..e}; do
echo "Current Letter (Increment): $letter"
done
# Decrement
for letter in {e..a}; do
echo "Current Letter (Decrement): $letter"
done
This script uses “for” loops to iterate through a sequence of letters. The first loop increments through letters ‘a’ to ‘e,’ echoing each iteration as “Current Letter (Increment)”. The second loop decrements through letters ‘e’ to ‘a,’ echoing each iteration as “Current Letter (Decrement)”.
Here, a-e and e-a are echoed using variable increment and decrement using the “for” loop.
7. Use of Changed Value and Increment-decrement
Loop variables do not always need to be modified in the loop declaration. Manual incrementing or decrementing of loop variables can occur inside the loop instead of during its initialization. The generic syntax for incrementing is((variable++))
, and for decrementing, it is((variable--)
). This script dives into the technique for modifying loop variables directly within the loop body, enabling dynamic control over iteration behavior:
#!/bin/bash
# Increment
for i in {1..5}; do
echo "Current Value (Increment): $i" # Uses the current value before incrementing
((i++)) # Increments i for the next iteration
done
# Decrement
for i in {5..1}; do
echo "Current Value (Decrement): $i" # Uses the current value before decrementing
((i--)) # Decrements i for the next iteration
done
The script combines two “for” loops, one for incrementing and one for decrementing i. The first loop increments i from 1 to 5, using the current value before incrementing, labeled as “Current Value (Increment)”. The second loop decrements i from 5 to 1, using the current value before decrementing, labeled as “Current Value (Decrement)”.
Here, the script echoes numbers 1-5 and 5-1 using variable increments and decrements in the “for” loop.
8. “for” Loop with “expr” Command
In Bash scripting, the “expr” command is a versatile tool for performing arithmetic expansions. This command finds its application within the “for” loop, offering an alternative method for numerical manipulations.
The generic syntax for incrementing is variable=$(expr $variable + value)
, and for decrementing, it is variable=$(expr $variable - value)
. The below script demonstrates an alternative method for arithmetic operations, showcasing the adaptability of Bash scripting to utilize external commands within the loop structure:
#!/bin/bash
# Increment
for i in {1..5}; do
i=$(expr $i + 3) # Increment i by 3 using expr
echo "Current Value (Increment): $i"
done
# Decrement
for i in {5..1}; do
i=$(expr $i - 3) # Decrement i by 3 using expr
echo "Current Value (Decrement): $i"
done
This script utilizes “for” loops with the “expr” command for incrementing and decrementing. The first loop increments i from 1 to 5, using the current value before incrementing. The second loop decrements i from 5 to 1, using the current value before decrementing.
9. “for” Loop with “let” Command
The “let” command is utilized for arithmetic expansions within the “for” loop. For incrementing, the generic syntax is let variable+=value
, and for decrementing, it is let variable-=value
. This script showcases another approach to numerical manipulation within the loop:
#!/bin/bash
# Increment
for i in {1..5}; do
let i+=5 # Increment i by 5 using let
echo "Current Value (Increment): $i"
done
# Decrement
for i in {5..1}; do
let i-=2 # Decrement i by 2 using let
echo "Current Value (Decrement): $i"
done
Using “for” loops with the “let” command, this script demonstrates incrementing and decrementing i. The first loop increments i from 1 to 5, using the “let” command to increment by 5. The second loop decrements i from 5 to 1, using the “let” command to decrement by 2.
10. “for” Loop with C-style Syntax with the “bc” Command
The “bc” command, standing for “basic calculator,” is a powerful and flexible command-line calculator in Linux/Unix-like operating systems. The “bc” command can seamlessly integrate into a C-style “for” loop for sophisticated arithmetic operations.
The generic syntax for incrementing involves using the “bc” command as follows variable=$(echo "$variable+value" | bc)
. For decrementing, the syntax is similarly structured variable=$(echo "$variable-value" | bc)
. This script demonstrates precise floating-point calculations within the loop:
#!/bin/bash
# Increment
for ((i=1; i<=5; )); do
i=$(echo "$i+1" | bc)
echo "Current Value (Increment): $i"
done
# Decrement
for ((i=5; i>=1; )); do
i=$(echo "$i-1" | bc)
echo "Current Value (Decrement): $i"
done
This script utilizes “for” loops with C-style syntax and the “bc” command to demonstrate incrementing and decrementing i. The first loop increments i from 1 to 5, using the “bc” command to increment by 1. The second loop decrements i from 5 to 1, using the “bc” command to decrement by 1.
Here, this code uses a “for” loop and the “bc” command to increment and decrement variables.
Increment and Decrement Variables in “while” Loop
A while loop in Bash is a control structure that executes a block of code repeatedly as long as a specified condition remains true. It continually checks the condition before each iteration. Here is the basic syntax for the “while” loop:
while [ condition ]
do
# Commands to be repeated as long as the condition is true
done
To integrate increment and decrement variables into a while loop, manipulating the loop variable within the loop body is essential.
Post Increment and Decrement
The “while” loop in Bash iterates through a set of commands as long as a specified condition holds true. In this context, i++
denotes post-increment, and i--
signifies post-decrement. The following script showcases these concepts, utilizing a “while” loop for both incrementing and decrementing and echoing the current variable value in each iteration.
#!/bin/bash
# Increment
i=1
while [ $i -le 5 ]; do
echo "Current Value (Increment): $i"
((i++))
done
# Decrement
i=5
while [ $i -ge 1 ]; do
echo "Current Value (Decrement): $i"
((i--))
done
In this script, two “while” loops are used to achieve variable incrementation and decrementation. The first loop increments i from 1 to 5, using the current value before incrementing. The second loop decrements i from 5 to 1, using the current value before decrementing.
Here, 1-5 and 5-1 are echoed using Increment and Decrement Variables in the “while” loop.
C-style “while” Loop with Increment and Decrement
The “while” loop in Bash, enriched by C-style syntax, empowers precise control over the loop variable. This Bash script utilizes a “while” loop structure to demonstrate incrementing and decrementing scenarios. The loop is controlled by conditions defined in C-style syntax, providing concise control over the loop variable. As the script iterates, it echoes the current variable value.
#!/bin/bash
# Increment
j=1
while ((j <= 10)); do
echo "Current Value (Increment): $j"
((j += 2))
done
# Decrement
j=9
while ((j >= 0)); do
echo "Current Value (Decrement): $j"
((j -= 2))
done
Featuring two “while” loops with C-style syntax, this script demonstrates variable incrementation and decrementation. The first loop increments j from 1 to 10 with a step of 2, echoing each iteration as “Current Value (Increment)”. The second loop decrements j from 9 to 0 with a step of 2, echoing each iteration as “Current Value (Decrement)”.
Here all odd numbers from 1-9 are echoed using C-styled syntax in the “while” loop.
Increment and Decrement Variables in “until” Loop
An until loop in Bash is a control structure designed to execute a block of code repeatedly until a particular condition evaluates to true. Unlike a “while” loop, the until loop continues iterating as long as the specified condition remains false. The basic syntax of an “until” loop is as follows:
until [ condition ]
do
# Commands to be repeated until the condition becomes true
done
Loop variables can be incremented and decremented within the “until” loop’s body.
Post Increment and Decrement
The “until” loop in Bash is a control structure that iterates through a set of commands as long as a specified condition remains false. In the following script, post-increment (i++
) and post-decrement (i--
) operations control the loop. The script demonstrates the “until” loop in action for both incrementing and decrementing scenarios, echoing the current variable value in each iteration.
#!/bin/bash
# Increment
i=1
until [ $i -gt 5 ]; do
echo "Current Value (Increment): $i"
((i++))
done
# Decrement
i=5
until [ $i -lt 1 ]; do
echo "Current Value (Decrement): $i"
((i--))
done
This script utilizes two “until” loops to achieve variable incrementation and decrementation. The first loop increments i from 1 to 5, using the current value before incrementing. The second loop decrements i from 5 to 1, using the current value before decrementing.
Here, 1-5 and 5-1 are echoed using Increment and Decrement Variables within the “until” loop.
C-style “until” Loop with Increment and Decrement
The “until” loop in Bash provides an effective means of iteration until a specified condition becomes true. In this context, the utilization of C-style syntax within the “until” loop allows for precise control over the loop variable. The script uses conditions like ((j > 10))
for incrementing and ((j < 0))
for decrementing, ensuring the loop continues until these conditions are met. As the script echoes the current variable value in each iteration, it highlights the seamless integration of C-style syntax within the “until” loop for controlled iteration.
#!/bin/bash
# Increment
j=1
until ((j > 10)); do
echo "Current Value (Increment): $j"
((j += 2))
done
# Decrement
j=9
until ((j < 0)); do
echo "Current Value (Decrement): $j"
((j -= 2))
done
Utilizing two “until” loops with C-style syntax, this script demonstrates variable incrementation and decrementation. The first loop increments j from 1 to 10 with a step of 2, echoing each iteration as “Current Value (Increment)”. The second loop decrements j from 9 to 0 with a step of 2, echoing each iteration as “Current Value (Decrement)”.
Here all odd numbers from 1-9 are echoed using C-styled syntax within the “until” loop.
Conclusion
The exploration of incrementing and decrementing variables within Bash scripting, particularly through the versatile “for” loop, opens doors to intricate numerical control and manipulation. From basic syntax to C-style constructs, these techniques showcase the adaptability of Bash scripting. I hope you will embrace the power of the “for loop” and use it in bash scripting.
People Also Ask
What is the += in the shell script?
The +=
in a shell script is an assignment operator that increments or appends the right-hand side value to the left-hand side variable. It is commonly used for appending, concatenating strings, or incrementing numerical values.
What does ++ mean in Bash?
In Bash, the ++
is a unary operator known as the increment operator. It is used to increase the value of a variable by 1. When applied to a variable, such as variable++
or ++variable
, it increments the variable’s value by 1. This operator is often used in loops, counting scenarios, and numeric operations, providing a concise way to increment variables in Bash scripting.
How do you increment and decrement variables in Bash?
In Bash, you can increment a variable by using the ((variable++))
syntax, where the ++
is the increment operator. This expression increases the value of the variable by 1. Similarly, you can use ((variable--))
for decrementing, where the --
is the decrement operator, decreasing the variable by 1.
How to increment a variable by 1 in Bash scripting?
To increment a variable by 1, you can use the ((variable++))
syntax within a (( ))
arithmetic expression in Bash scripting. Here, ++
is the increment operator, and it adds 1 to the current value of the variable. This expression is commonly used in scenarios where you need to iteratively increase the value of a variable, such as in loops or counting operations within Bash scripts.
how to create a variable in bash?
In Bash, you can create a variable by using the assignment operator =
. For example, to create a variable named myVar with the value Hello, you can use the following syntax myVar="Hello"
. This sets the variable myVar to the specified value, allowing you to store and manipulate data within your Bash scripts.
What are pre-increment and post-increment in bash scripting?
In Bash scripting, pre-increment and post-increment are unary operators used to increase the value of a variable.
Pre-increment (++variable
): Increases the variable’s value before its current value is used in an operation.
Post-increment (variable++
): Increases the variable’s value after its current value is used in an operation.
These operators offer flexibility in how you manipulate variable values, providing control over the order of operations in Bash scripts.
Related Articles
- 10 Common Bash “for” Loop Examples [Basic to Intermediate]
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Use Nested “for” Loop in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial