FUNDAMENTALS A Complete Guide for Beginners
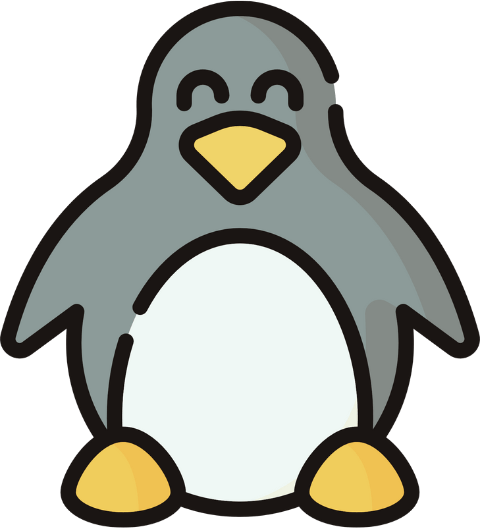
The loop is a powerful tool in programming languages that elevates programmers’ ability to automate repetitive tasks efficiently. From initiating the execution flow to strategically influencing iterations, loop control empowers programmers to overcome complex scenarios and optimize their code. In this article, I will explore loop control in Bash scripting.
What is Loop Control Statement?
The statements that control the execution of a loop from its normal sequence are known as loop control statements. Mainly, 2 types of loop control statements are well known to programmers. These are the continue statement and the break statement. In this section, I will briefly explain these two statements:
1. Continue Statement
The continue statement skips the current iteration of a loop execution and jumps to the next iteration. When it is encountered, the remaining commands in the loop block are skipped, and the loop proceeds with the next iteration.
Here is a bash script with having continue statement:
#!/bin/bash
for i in {1..5}; do
if [ $i -eq 3 ]; then
echo "Skipping iteration for i = $i"
continue
fi
echo "Processing iteration for i = $i"
done
The for i in {1..5}; do
command initiates the for loop and iterates through 1 2 3 4 5
list. After that, the if [ $i -eq 3 ]; then
command checks whether the iteration value equals 3. If the condition is true, the continue command
executes, and it skips that specific iteration and jumps to the next iteration.
The image shows that the continue command has skipped a specific iteration of the loop and jumps to the next iteration.
2. Break Statement
The break statement is used to terminate a loop forcefully. It can be employed within any type of loop. This statement immediately exits the execution of a loop and jumps to the subsequent statement after the loop.
Here is a bash script with a break statement:
#!/bin/bash
for i in {1..5}; do
echo "Iteration: $i"
echo "Processing iteration for i = $i"
if [ $i -eq 3 ]; then
echo "Breaking out of the loop at iteration 3."
break
fi
done
The for i in {1..5}; do
command initiates the for loop and iterates through the 1 2 3 4 5
list. After that, the if [ $i -eq 3 ]; then
command checks whether the iteration value equals 3. If the condition is true, the break command
executes, which terminates the execution of the for loop and jumps to the subsequent code after the loop.
Types of Loop Control in Bash
There are mainly 3 types of loops in Bash. These are for loop, while loop, and until loop. Each loop type has some special purpose, making using them in our script convenient. Here, I am giving you some insight into these loops with some sample bash scripts on how to use them to achieve your task:
1. For Loop
For loop iterates over a sequence of values. It is employed when the number of iterations is predefined before. The basic syntax of for loop iterations is for i in [predefined list]
. Here, the for loop will iterate through all elements of the predefined list.
Follow the script to know about how for loop works:
#!/bin/bash
for i in 1 2 3 4 5
do
echo "Hello! $i"
done
At first, the for loop will iterate through the list 1 2 3 4 5 and $i prints Hello! 1 Hello! 2 … Hello! 5 for each element of the iteration list.
The image shows the execution of a simple for loop.
For loop with “seq” Command for Number Generation
The seq command can generate numbers at a regular interval, which generates a list of numbers according to the necessity and iterates through that list. The basic syntax for generating a controlled list is: seq start_number interval end_number
.
Here is a for loop using a list made from the seq command:
#!/bin/bash
for i in $(seq 1 2 9)
do
echo "Count $i"
done
The seq 1 2 9
command will generate a list of numbers from 1 to 9 with an interval of 2. It will generate a list of some odd numbers. Then, the echo command will print each element of the list.
For Loop Iterating Through Array
The array can contain a list of important elements, and the for loop can iterate through the array elements one by one. For this, define an array before the start of the for loop. Then, iterate through each element of the array by proper indexing.
Here is a bash script to iterate through the array element:
#!/bin/bash
color=(red green blue pink yellow)
for i in ${color[@]}
do
echo "$i"
done
The color=(red green blue pink yellow)
command generates an array named color. Then, the for i in the ${color[@]}
command iterates through each array element and prints them on the terminal.
For Loop Iterates Through String
The for loop can iterate through each separate word of a string. For this, define a string first. Then follow the syntax: for i in ${string_name}
to iterate through each separate string element.
Here is a sample bash script of iterating through string:
#!/bin/bash
strings="Hello from a string"
for i in ${strings}
do
echo "String $i"
done
The strings="Hello from a string"
command defined a string. Then, the for i in ${strings}
command iterated through each word of the strings one by one and printed it on the terminal.
2. While Loop
The while loop generally executes a code block as long as the specified condition remains true. This loop continues the execution before any interruption is made or any operation makes the condition false.
Here is a simple bash script of the while loop:
#!/bin/bash
c=5
while [ $c -gt 0 ];
do
echo $c
((c--))
done
echo "Loop Terminates"
At first, the value of c is defined as 5. Then, the condition checks if the value of c is greater than 0. If the condition is true, then the echo command prints the value of variable c, and the ((c--))
command decreases the value of c by one. Afterwards, the condition is checked again. If the condition remains true, it will execute the echo command
again; otherwise terminates the loop.
The image shows that the while loop iterated from 5 to 1 and then terminates.
3. Until Loop
The until loop in Bash is a control flow construct that is similar to the while loop. The different feature lies in the until loop is that the block of code is executed repeatedly until a specified condition becomes true.
Here is a simple bash script of the until loop:
#!/bin/bash
c=0
until [ $c -gt 5 ]
do
echo Count: $c
((c++))
done
At first, c is defined as zero. Then, the until [ $c -gt 5 ]
command checks if c is greater than 5. If the condition is false, the echo command will print the value of c, and the ((c++))
command will increase the value of c by one. After that, the condition will check again whether c is greater than 5. If not, the loop will be executed again.
Infinite Loop
Some system monitoring scripts are bound to run continuously in the background for an infinite time. In such scenarios, employing an infinite loop helps programmers to execute a specific script repeatedly in a regular manner.
Here is a bash script of an infinite loop:
#!/bin/bash
while true; do
echo "This is an infinite loop. Press Ctrl+C to exit."
sleep 1
done
The while true; do command initiates the while loop where the condition is true means the code block inside the loop will be executed. The sleep 1
command marks a 1-second delay for the next iteration. This infinite execution of the while loop can be stopped only if CTRL+C
is pressed.
Nested Loop
Nested loops in Bash script means placing one loop inside another loop. This nested loop allows complicated iterations on multilayers, especially with multidimensional data or nested conditions.
Here is a bash script on nested loop:
#!/bin/bash
# Set the range for the multiplication table
rows=5
cols=5
# Outer loop for rows
for ((i=1; i<=$rows; i++))
do
# Inner loop for columns
for ((j=1; j<=$cols; j++))
do
# Calculate and print the product
product=$((i * j))
echo -n -e "$product\t"
done
# Move to the next line after each row is printed
echo ""
done
At first, the rows=5 cols=5
command defines the rows and cols. After that, the for ((i=1; i<=$rows; i++))
initiates the first for loop that will iterate through each element of rows. Then, the for ((j=1; j<=$cols; j++))
initiates the second for loop that will iterate through all elements of cols for a single element of rows. Here, the product=$((i * j))
calculates the multiplication of the cols number with the rows number, and the echo -n -e "$product\t"
prints the result afterward in the same line.
The nested loop has printed a multiplication table on the terminal.
Practical Examples of Loop Control in Bash
Loop eases our way to solving many practical life problems and makes our bash scripting journey effortless. Here I will show you some practical examples of loop control in Bash:
Finding Specific Files in Bash
Loops can search for specific files and list them on the terminal. To do this, specify the file type in the for loop you want to search
Here is the complete bash script to find specific files:
#!/bin/bash
#searching shell script
for s in *.sh
do
echo $s
The for s in *.sh
marks the beginning of a for loop. It iterates over all files in the current directory with a .sh
extension. The ‘*.sh’
is a wildcard that matches all files ending with ‘.sh’. The variable s is used to represent each file in each iteration. Finally, the echo $s
command prints each match on the terminal.
The bash script has displayed all shell scripts of the current directory.
Command Substitution
Command substitution means substituting a command with a small user-defined keyword. It helps avoid writing long commands again and again.
Here” is a script using command substitution:
#!/bin/bash
#command substitution
command=`cat list.txt`
for i in $command
do
echo $i
done
The command=`cat list.txt`
assigns the file ‘list.txt’ contents to the variable command. Here the cat command concatenates and displays the content of ‘list.txt’, and the backticks ‘’ are used for command substitution. After that, the for i in $command
initiates the for loop that prints each comma or newline-separated element inside the command variable.
The script printed all the contents of the list.txt file.
Command Line Arguments
The Command line is a powerful interface for giving input and getting output from a computer, providing a direct way to communicate instructions to the operating system. Bash script takes arguments to a script or program at the time of execution and makes necessary changes or operations.
Here is an example of a bash script taking command line arguments:
#!/bin/bash
#command line arguments
for i in $@
do
echo "$i"
done
The for i in $@
iterates over the command line arguments, and $@
represents all the command line arguments passed to the script. Then, the echo "$i"
prints all the arguments passed to the bash script.
Conclusion
Learning about loop control is not just about efficient programming; it’s a strategic approach to enhancing code flexibility, readability, and performance. As I have explored the versatility of loop control statements such as break and continue in this article, the readers can now precisely shape the flow of their bash scripts. Whether optimizing processes, handling user interactions, or delving into complex simulations, loop control is invaluable.
People Also Ask
What is the use of loop control?
Basic loop control structures are the break command and the continue command provide a way to influence the flow of execution within loops. These commands are used to enhance the flexibility and control the loop’s execution. The continue statement skips the rest of the loop body and moves to the next iteration, and the break statement terminates a loop when a specific condition is met.
Can you loop through a string in Bash?
Yes, you can loop through a string in Bash. For this, follow the below script:
#!/bin/bash
strings="This is a string"
for i in ${strings}
do
echo "String $i"
done
This script will iterate through each separate element of the main string and print them on the terminal.
What is the main use of a loop?
Loops are fundamental constructs in programming languages. It serves the main purpose of repeating a block of code multiple times. The primary uses of loops are as follows:
- Efficiently process each element within a data structure without manually repeating code.
- Automate repetitive processes, making scripts and programs more efficient and less error-prone.
- Implement conditional logic that depends on dynamic factors such as user input or changing system states.
- Enhance user interaction and input validation by allowing users multiple attempts to provide correct information.
What is the difference between a for loop and a while loop in Bash?
The for loop iterates over a predefined list on the other hand, the while loop continues iterating as long as a specified condition is true. Generally, the for loop is well-fitted for a known number of iterations or iterating over a specific range or list. On the other hand, the while loop is well suited for scenarios where the loop continuation depends on dynamic conditions or is not known beforehand.
How does loop function work?
A loop is a control flow structure that allows a set of instructions to be repeatedly executed based on a certain condition or a predefined number of iterations. There are three types of loop: for loop, while loop, and until loop. They execute a set of code repeatedly depending on the conditions. The for loop iterates through a predefined list and executes the code block. The while loop executes the code block if the condition is true. The until loop executes the code block if the condition is false.
Related Articles
- For Loop in Bash
- While Loop in Bash
- Until Loop in Bash
- Select Loop in Bash [5 Practical Examples]
- How to Use Loop Control in Bash [Break & Continue Statement]
<< Go Back to Loops in Bash | Bash Scripting Tutorial