FUNDAMENTALS A Complete Guide for Beginners
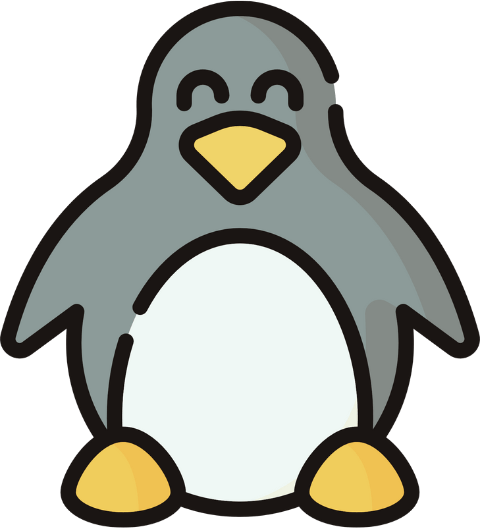
The printf command is the default choice to format number in Bash. It offers multiple format specifiers such as %d and %f to format integer and floating point numbers. Moreover, there are many external tools available in Bash to format numbers. Tools like awk are easy to use and provide greater flexibility. This article discusses various aspects of formatting numbers using printf and other utilities.
1. Zero Padding Numbers for Consistent Length in Bash
For padding numbers with zeros to achieve fixed length, make the proper use of the printf command. This command works to pad single or multiple numbers and numbers including integers or floating points.
Format Integer Number With Leading Zeros Using “printf” Command
The “%d” format specifier of printf command is useful for formatting integer numbers. It is quite easy to maintain a fixed length of integer numbers by adding leading zeros. For instance, consider a number 42. To add two leading zeros to make the number a fixed length of four digits:
num=42
printf "%04d\n" $num
“%04d” is the format specifier used for formatting the number stored in the num
variable. Here-
%: Indicates the beginning of the format specifier.
0: Specifies that padding should be done with zeros.
4: Indicates the minimum width of the field. In this case, it says that the number should be at least four characters wide.
d: Specifies that the argument is an integer.
\n: Adds a newline character after printing the formatted number, making the output appear on a new line.
The command prints 0042 by adding two leading zeros to the number 42.
Now, consider a list of integer numbers of different lengths stored in a file:
# inputfile.txt
10
4752
121
587
78
403279
To make all the integers equal in length, you need to format those by adding necessary leading zeros. Here is how to do this:
#!/bin/bash
# Specify the desired length of the formatted integers
desired_length=6
touch outputfile.txt
# Read integers from the input file and format them
while read -r number; do
formatted_number=$(printf "%0${desired_length}d\n" "$number")
echo "$formatted_number" >> outputfile.txt
done < inputfile.txt
echo "Formatted numbers written to outputfile.txt:"
cat outputfile.txt
The while loop iterates through each line in the “inputfile.txt”, where each line contains an integer. The read -r
command reads and stores each integer in a variable named number
. The printf command is then utilized to format the number by adding leading zeros, ensuring a fixed length as defined by the desired_length
variable, which is set to 6 in this example. The formatted number is subsequently appended to the “outputfile.txt” file, which was created earlier in the program using the touch command. This process repeats for each integer in the input file.
Formatting Floating Point Numbers to Maintain Equal Length
“%f” format specifier is used for formatting floating point numbers. Consider the floating point number 3.1416. The width of the number (including the decimal point) is 6. To format the number and make the width 10, try the following command:
printf '%010.5f\n' 3.1416
“%010.5f\n” is the format specifier to format the floating point number 3.1416.
%: Indicates the beginning of the format specifier.
0: Specifies that padding before the decimal point should be done with zero.
10: Specifies the minimum width of the number should be 10.
5: Specifies the length of digits after the decimal point.
f: Specifies that the number to be formatted is a floating point number.
To format multiple floating-point numbers simultaneously, list them consecutively followed by the format specifier. Place a space between each number to keep them distinct like the command below:
printf '%010.5f\n' 1.125 531.4561 31.33 1.2
As you can see all the provided numbers are returned as 10-digit numbers. For example, 1.125 becomes 0001.12500. In this case, three leading zeros and two tailing zeros are added to make it a 10-digit number.
2. Format Numbers by Thousand Separator (Commas)
To format numbers with thousand separator (commas) using the printf command in Bash, you can place a single quote (‘) after the % sign of the format specifier. Let’s say you have the number 8154321. You can represent it with thousand separators as follows:
printf "%'d\n" 8154321
Due to the single quote ('
) placed after %
and before d
, comma will be printed as a thousand separator. The whole format specifier will be applied to the number that is given after by the specifier.
To add comma as a thousand separator to multiple numbers at a time, place those numbers after the format specifier one after one.Here both the numbers 8154321 and 578757 returned with the comma inserted in the thousand positions.
You can insert comma thousand separator in a floating point number as well. But make sure you use %f format specifier to indicate the floating point number.
printf "%'.2f\n" 2405770.54
3. Convert Numbers to Currency Format in Bash
Sometimes it is necessary to represent numbers in currency format. To add currency signs with numbers using the printf command, users need to specify the signs in the format specifier. Below commands add the dollar sign and rupee sign respectively to express the numbers in currency format.
printf "$%'.2f\n" 457.50
printf "INR.%'.2f\n" 457.50
The first command adds a dollar sign to 457.50. The second command represents 457.50 in Indian Rupees with two decimal places. The prefixes $
and INR
in the format specifier ensure the addition of the currency signs.
4. Adding Scientific Notation to Numbers
Formatting numbers by adding scientific notation is the best way to represent very large or small numbers in a compact way. The printf command in Bash supports printing numbers in scientific format. Look at the following examples:
printf "%.2e\n" 0.000000123
printf "%.2e\n" 0.000000000000000000000123
printf "%.2e\n" 667154874454
In the above commands, "%.2e\n"
is the format specifier. 2
specifies the number of digits after the decimal point. e
indicates exponential. The commands adjust the number after exponential including the sign to express the number after the format specifier in scientific format.
5. Converting Numbers to Time Format in Bash
It is often required to convert numbers, such as Unix timestamps, to a human-readable time format in Bash. The -d option of the date command can convert timestamp numbers presented in seconds into a time-date format. Look at the following command to do this:
date -d @47455779
-d
option of the date command converts the Unix timestamp given after the @
sign.
6. Remove Leading Zeros From Numbers in Bash
To remove leading zeros from a number, format the numbers using parameter expansion. For instance, ${num#0} removes a single leading zero from the variable num. Iterating this using a loop essentially deletes all the leading zeros from the number. Here’a how:
#!/bin/bash
num=000475
echo “Before removing leading zeros: $num”
while [ "$num" != "${num#0}" ];
do num=${num#0};
done
echo “After removing leading zeros: $num”
The while loop iteratively removes leading zeros from a variable named num
. The loop condition checks if the original value of num
is not equal to the value of num
with leading zeros removed. Within the loop, the expression ${num#0}
removes the shortest match of 0 from the beginning of the num
variable. The loop continues until no leading zeros are left in num
.
In addition to the printf command, one can use awk command to remove leading zeros from a number. To do so, use the command below:
echo "005487" | awk '{$0=int($0)}1'
The code takes the string “005487” and converts it to an integer using awk. '{$0=int($0)}1'
ensures the integer conversion and prints the number without any leading zeros.
Conclusion
In conclusion, formatting numbers is an easy task in Bash. Different format specifier of the printf command allows the user to tailor the output of formatting. I believe this article helped you to understand the format specifiers in detail.
People Also Ask
How to zero padd a variable in ksh?
In Korn Shell (ksh), the typeset -Z
command is used to zero-fill a variable. The -Z
option is followed by a digit specifying the field width. When you use typeset -Z5 x
, it means that the variable x
will be zero-filled to a field width of 5 characters.
x=26 typeset -Z5 x
echo $x # Output: 00026
What are the pitfalls of zero padding using “printf” in Bash?
Octal representation is one of the main pitfalls of zero-padding using printf. An octal number takes a 0 prefix. Therefore, a decimal number that already has a leading zero is considered an octal. Zero-padding such numbers leads to an unintended result.
printf '%06d' 0666
# Output: 000438
How to remove leading zeros using “sed” command in Bash?
To remove leading zeros from a number using the sed command in Bash, you can use the following syntax:
echo "000123" | sed 's/^0*//'
Here, ^
anchors the pattern to the beginning of the line. 0*
matches zero or more occurrences of the digit zero. Finally, //
replaces the matched pattern with an empty string.
How to use sub() function of awk tool to remove zero padding?
To remove zero padding using sub() in awk, you can create a regular expression that matches leading zeros and replace them with an empty string. For example:
echo "000123" | awk '{sub(/^0*/,"")}1'
In this command, ^
indicates the beginning of the string. 0*
matches one or more occurrences of the digit “0”. ""
is an empty string, which effectively removes the matched portion.
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Calculate in Bash [3 Practical Examples]
- How to Sum Up Numbers in Bash [Explained With Examples]
- How to Divide Two Numbers in Bash [8 Easy Ways]
- Division of Floating Point Numbers in Bash
- How to Use “Mod” Operator in Bash [5 Basic Usage]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
<< Go Back to Arithmetic Operators in Bash | Bash Operator | Bash Scripting Tutorial