FUNDAMENTALS A Complete Guide for Beginners
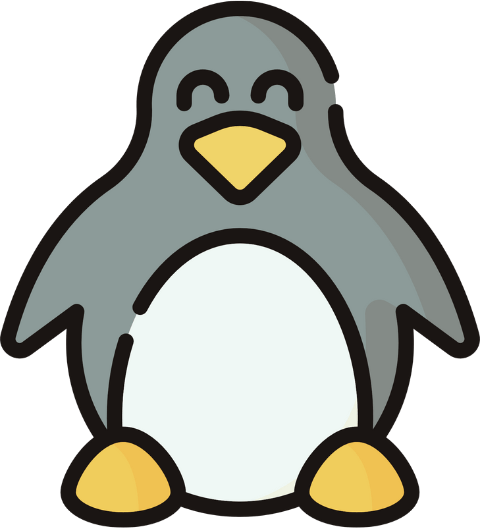
Bash users often need help with performing arithmetic calculations in Shell. This happens because of inadequate knowledge about the syntax and its applicability. In this article, I am going to talk about the syntax and limitations of Bash arithmetic. In addition, I will talk about different commands and utilities to perform various calculations in Bash.
Integer Calculations in Bash
Integers are whole numbers including positive, negative and zero. For instance, 5, 36, 0 and -9 are integers. However, fractional numbers such as 6.73 and √5 are not integers. The reason for recalling this- Bash built-in primarily performs integer arithmetic. Arithmetic expansion is the way to evaluate expressions in Bash. An expression that is written inside double parentheses after a dollar sign returns the evaluated result of the expression. Look at the following addition of 4 plus 5:
echo $((4+5))
However, arithmetic expansion is not the only way to play with integers in Bash. There are other built-ins and external utilities to perform math calculations with integer numbers. Let’s evaluate an expression with the help of the declare command:
declare -i A=(2+3)**2
echo $A
The -i
option is used to declare an integer value. First, the addition operator (+
) adds 2 and 3. After that, the exponential operator (**
) raises the sum to the power of 2. The evaluated result, 25, is then stored in the variable A
.As expected echoing A prints 25 in the console.
Floating Point Calculations in Bash
A floating-point number, also known as float is a way to represent real numbers. Unlike integers, floats have both whole and fractional parts, like 3.14159 or 0.25.
Floating point numbers are usually represented by binary or decimal bases. Computers usually store numbers in binary format (base 2). Think about a floating point decimal number 6.75. It is 110.11 in binary. It can be written as 1.1011×2^2. Here, 1.1011 part is called significand. The power of 2 (which is 2 here) is called exponent. There are two main types of floating point numbers stored in computers:
Type | Total Bits | Bits for Significand | Bits for Sign | Bits for Exponent | Range of Numbers |
---|---|---|---|---|---|
Single Precision | 32 | 23 | 1 | 8 | 1.2 x 10^-38 to 3.4 x 10^38 |
Double Precision | 64 | 52 | 1 | 11 | 2.2 x 10^-308 to 1.8 x 10^308 |
Now move on to floating point arithmetic in Bash. Unfortunately, Bash doesn’t support floating-point calculations. To handle calculations that include floating point numbers, users need to rely on external tools like bc or awk:
echo "scale=2; 5.5 * 2.3" | bc
"scale=2; 5.5 * 2.3"
to the bc command using a pipe. bc evaluates the multiplication of 5.5 and 2.3. scale=2
sets the precision of the result should be up to two decimal places.
1. How to Create a Simple Calculator in Bash
Creating a simple calculator involves taking user input and selecting the proper arithmetic operators. Here is the Bash script of a basic calculator:
#!/bin/bash
# Take user input
read -p "Enter the first number: " a
read -p "Enter the second number: " b
# Input type of operation
echo "Enter Choice :"
echo "1. Addition"
echo "2. Subtraction"
echo "3. Multiplication"
echo "4. Division"
read -p "Enter your choice (1/2/3/4): " ch
# Perform calculator operations using case statement
case $ch in
1) res=$(echo "$a + $b" | bc)
;;
2) res=$(echo "$a - $b" | bc)
;;
3) res=$(echo "$a * $b" | bc)
;;
4) res=$(echo "scale=2; $a / $b" | bc)
;;
*) echo "Invalid choice. Exiting."
exit 1
;;
esac
# Print the result
echo "Result : $res"
First of all, the read command takes two numbers from the user and stores them in a
and b
variables respectively.
Next, the script presents a menu to the user, displaying options for different arithmetic operations: addition (1), subtraction (2), multiplication (3) and division (4).
Then it utilizes the bc command to perform the chosen operation and stores the result in the res
variable inside the case statement. Moreover, if a user enters an invalid choice the program immediately terminates echoing the message, “Invalid choice. Exiting.”.
2. How to Calculate Execution Time in Bash
There are multiple ways to calculate the execution time in Bash. To calculate the execution time of a particular portion of a script first capture the start and end time using the date command. Then use subtraction to find the difference between the two times to calculate the time elapsed for executing that portion of the script. Look at the code below to implement the idea:
#!/bin/bash
start=$(date +%s)
#command block start
sleep 3
sleep 5
#command block end
end=$(date +%s)
echo "Time Elapsed : $(($end-$start)) seconds"
The script captures the current timestamp in seconds as the start time.
Then it executes two commands sleep 3
and sleep 5
. These two commands pause the script for 8 seconds.
After execution of the commands, the script captures the end time.
Finally, it calculates the time elapsed during the execution of the commands by subtracting the start time from the end time.
3. How to Calculate Logarithm in Bash
-l option of the bc command can calculate the natural logarithm of a number. To calculate e-base logarithm of a number using bc:
echo "scale=2; l(10)" | bc -l
"scale=2; l(10)"
text is passed to the bc command to evaluate the natural logarithm of 10. scale=2
defines the precision up to two decimal places.
Similarly, one can use the awk command to evaluate the natural logarithm of a number. Let’s evaluate the natural logarithm of 10 again using the awk:
echo 10 | awk '{printf "%11.9f\n",log($1)}'
The echo command passes the number to the awk command. Within the command, log($1)
calculates the natural logarithm of the provided number. The format specifier "%11.9f\n"
specifies the width as 11 and nine digits after the decimal point.
Conclusion
In conclusion, I tried to show the basic syntax for performing arithmetic calculations in Bash. Moreover, I explained the challenges users encounter due to the limitations of Bash arithmetic when dealing with floating-point numbers. Furthermore, I came up with a few external utilities that users can use to perform precise floating-point calculations. Finally, a couple of practical examples are given where performing calculations plays a crucial role. I hope you got the main theme of this article.
People Also Ask
What is the downside of using the SECONDS variable to calculate time elapsed?
The main downside of the SECONDS variable in calculating elapsed time is precision. SECONDS variables always provide the execution time of a script or portion of a script in seconds. On the other hand, when you take timestamp using the date command you can calculate elapsed time in nanoseconds as well if it’s necessary.
How to calculate total as loop output in Bash?
To calculate the total of a few numbers in Bash, use the for loop. Let’s say you have some numbers in an array. Use the following code to calculate the total:
#!/bin/bash
# Initialize total to zero
total=0
# Numbers to be added (you can modify this list)
numbers=(2 5 8 3 10)
# Loop through the numbers and calculate the total
for num in "${numbers[@]}"; do
# Add the current number to the total
total=$((total + num))
done
# Print the final total
echo "The total is: $total"
The numbers are stored in the numbers
array. The for loop iterates over the array and adds each number to the total
. Therefore, the total
becomes the summation of all the numbers of the array.
How to calculate percentage in Bash?
To calculate the certain percentage of a number in Bash, multiply the number with the required percentage then divide the result by 100. Think of an example where you want to get 80% of 249:
#!/bin/bash
# Define the number
number=249
# Define the percentage
percentage=80
# Calculate the result
result=$(( (number * percentage) / 100 ))
# Print the result
echo "80% of $number is: $result"
$(( (number * percentage) / 100 ))
calculates the percentage by multiplying 249 by 80 and diving the result by 100.
How to write a Bash script to calculate average in Bash?
To calculate the average of some numbers in Bash, first sum up numbers then divide the summation with the count of numbers. Look at the script to implement the idea in Bash:
#!/bin/bash
# Numbers to calculate the average (you can modify this list)
arr1=(15 20 25 30 35)
# Initialize sum and count variables
sum=0
count=0
# Loop through the numbers to calculate the sum and count
for num in "${arr1[@]}"; do
sum=$((sum + num))
((count++))
done
# Calculate the average
average=$((sum / count))
# Print the result
echo "The average is: $average"
The for loop counts the numbers of arr1
and accumulates them in the sum
variable. $((sum / count))
calculates the average of the numbers by dividing the summation by the count
of numbers.
Related Articles
- Performing Math Operations in Bash [12+ Commands Explained]
- How to Sum Up Numbers in Bash [Explained With Examples]
- How to Divide Two Numbers in Bash [8 Easy Ways]
- Division of Floating Point Numbers in Bash
- How to Use “Mod” Operator in Bash [5 Basic Usage]
- How to Format Number in Bash [6 Cases]
- How to Generate Random Number in Bash [7 Easy Ways]
- How to Convert Hexadecimal Number to Decimal in Bash
- [Solved] “binary operator expected” Error in Bash
- [Fixed] “integer expression expected” Error in Bash
<< Go Back to Arithmetic Operators in Bash | Bash Operator | Bash Scripting Tutorial