FUNDAMENTALS A Complete Guide for Beginners
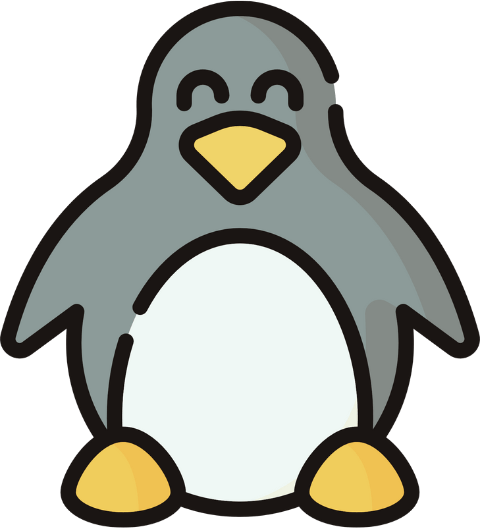
A bit is the smallest unit of data and stands for “binary digit.” It can hold either 0 or 1 as a value. These values are the building blocks of all digital data and are fundamental to computing. They form the basis for encoding information and executing operations within a computer system. Bitwise operators manipulate the binary digit, performing logical operations such as AND, OR, XOR, NOT, left shift, and right shift on the given integer value. By altering specific bits, these operators enable precise control over how data is processed and manipulated at the lowest level. In this article, you will have a comprehensive overview of bitwise operators in bash. So let’s start!
What Are Bitwise Operators?
Bash Bitwise operators are operators that directly manipulate individual bits of a binary number. They perform operations on binary representations of numbers, altering specific bits according to their defined logic. The bitwise operators include:
- AND (&): Performs a bitwise AND operation. Example: a & b results in a number where each bit is set to 1 only if the corresponding bits of both a and b are 1.
- OR (|): Performs a bitwise OR operation. Example: a | b results in a number where each bit is set to 1 if at least one of the corresponding bits of a and b is 1.
- XOR (^): Performs a bitwise XOR (exclusive OR) operation. Example: a ^ b results in a number where each bit is set to 1 if the corresponding bits of a and b are different.
- NOT (~): Performs a bitwise NOT operation. Example: ~a results in a number where each bit is inverted (0 becomes 1, and 1 becomes 0).
- Left Shift (<<): Shifts the bits of a number to the left by a specified number of positions. Example: a << b shifts the bits of a to the left by b positions.
- Right Shift (>>): Shifts the bits of a number to the right by a specified number of positions. Example: a >> b shifts the bits of a to the right by b positions.
How Bitwise Operator Works
The truth table below shows how the bitwise operator outputs according to the input:
Input | Output of Bitwise Operation | ||||
---|---|---|---|---|---|
A | B | AND | OR | NOT (A) | XOR |
0 | 0 | 0 | 0 | 1 | 0 |
0 | 1 | 0 | 1 | 1 | 1 |
1 | 0 | 0 | 1 | 0 | 1 |
1 | 1 | 1 | 1 | 0 | 0 |
Example Uses of Bitwise Operators in Bash
Here are examples of how to use different types of bitwise operators in Bash script:
1. Bitwise NOT Operator
The bitwise NOT is a unary operator, represented as ~ in Bash, flips the bits of a number. It changes each 0 to 1 and each 1 to 0 in the binary representation of the number. For instance, applying the bitwise NOT operator to a number like 5 (binary 101) would result in -6. -6 is also regarded as the two’s complement of 5.
To use the bitwise NOT operator in your bash script, follow the sample bash script:
#!/bin/bash
number=5
result=$(( ~number ))
echo "Bitwise NOT of $number is $result"
In this code, the variable number is set to 5. The bitwise NOT operator (~) is applied to this number, and the result is stored in the variable result. Finally, the script outputs the original number and the result of the bitwise NOT operation using the echo command.
From the image given above, the script changes the integer value 5 to its two’s complement -6. As a rule of thumb, you can see that NOT is always the same as subtracting 1 from the opposite of the given value. The reason for this is that Bash uses two’s complement to implement the sign change. It does this by inverting all the bits and adding 1.
2. Bitwise AND Operator
The bitwise AND operator (&) is a binary operator that performs AND operation between the individual bits of two numbers. It evaluates each bit position of the two operands and results in 1 only if both bits are 1. If either or both bits are 0, the corresponding bit in the result will also be 0.
A sample operation of bitwise AND operator is given below:
#!/bin/bash
num1=5 # Binary: 101
num2=3 # Binary: 011
result=$(( num1 & num2 ))
echo "Bitwise AND between $num1 and $num2 results: $result"
In this scenario, num1 holds the value 5, which in binary is 101, and num2 has the value 3, represented as 011 in binary. The & operator is used to perform a bitwise AND operation on these two numbers.
As the binary value of integers 5 and 3 are 101 and 011 respectively, the AND operator returns 001 which is the equivalent value of 1 in the decimal number system.
3. Bitwise OR Operator
The bitwise OR operator (|) is a binary operator that performs OR operation between the individual bits of two numbers. It results in a 1 if at least one of the bits is 1. If both bits are 0, the corresponding bit in the result will also be 0.
Here is a sample bash code illustrating the use of the bitwise OR operator:
#!/bin/bash
num1=5 # Binary: 101
num2=3 # Binary: 011
result=$(( num1 | num2 ))
echo "Bitwise OR operation between $num1 and $num2 results: $result"
In this code, num1 holds the value 5 (binary: 101) and num2 holds the value 3 (binary: 011). The | operator performs a bitwise OR operation on these numbers.
As the binary equivalency of integers 5 and 3 are 101 and 011, the bitwise OR operator returns 111 which is 7 in the decimal number system.
4. Bitwise XOR Operator
The bitwise XOR (exclusive OR) operator (^) is a binary operator that performs XOR operation between the individual bits of two numbers. Its logic dictates that if the bits being compared are different, the result is 1; if they’re the same, it yields 0. For instance, when the XOR operator is applied to 1 and 0, the result is 1 due to the difference in bits, while the XOR operator is applied to two identical bits, such as 1 and 1 or 0 and 0, resulting in 0.
To understand the operation of the bitwise XOR operator, follow the script:
#!/bin/bash
num1=5 # Binary: 101
num2=3 # Binary: 011
result=$(( num1 ^ num2 ))
echo "Bitwise XOR operation between 5 and 3 results: $result"
In this script, num1 holds the value 5 (binary: 101), and num2 holds the value 3 (binary: 011). The ^ operator performs a bitwise XOR operation on these numbers.
The bitwise XOR operator here compares 5 with 3 and returns 6 to the terminal.
5. Bitwise Left Shift
The left bitwise shift operator in bash moves the bits of a number to the left by a specified number of positions. It is represented by <<. When performing a left shift by a certain number of bits, the binary representation of the number moves to the left, which eventually adds zeros to the right.
For instance, If you have the binary number 101 (which is 5 in decimal) and you shift it left by 2 positions (num << 2), it becomes 10100, which is 20 in decimal.
In binary, shifting left effectively multiplies the number by 2 raised to the power of the shift amount. Here, shifting 5 (101 in binary) to the left by 2 positions yields 20 (10100 in binary).
#!/bin/bash
num=5
# Shifting left by 2 positions
result=$(( num << 2 ))
echo "Left Shift Result: $result"
In this script, the variable num holds the value 5. The << operator performs a left shift by 2 positions on this number and stores the result in the ‘result’ variable. The output of the ‘result’ variable is then displayed using the echo command.
Here, the left shift operator returns 20, which is the left shifting of the 5 by 2 position.
6. Bitwise Right Shift
The right bitwise shift operator (>> in Bash) moves the bits of a number to the right by a specified number of positions. It discards the rightmost bit and introduces a new bit, which is usually 0, on the leftmost position. Thus, each shift right by a single position effectively divides the number by 2
Here’s an example illustrating the right bitwise shift operator in Bash:
#!/bin/bash
num=20
# Shifting right by 2 positions
result=$(( num >> 2 ))
echo "Right Shift Result: $result"
In this script, the variable num holds the value 20. The num >> 2
performs a right shift by 2 positions on the num variable and stores the result in the ‘result’ variable. The output of the ‘result’ variable is then displayed using the echo command.
The bitwise right shift by 2 positions effectively divides the binary representation of num by 22 (which is 4), discarding the remainder. In this specific case, the result of the right shift would be 20 divided by 4, resulting in 5.
Conclusion
Bitwise operators are often used in scenarios where you need to perform operations at the bit level, such as setting or clearing specific bits, or when working with hardware-level programming. So far in this article, you have learned what the basics of bitwise operators are and how they manipulate the binary digit. However, if you have any questions or queries related to bitwise operators in bash, don’t forget to comment below. Happy Coding!
People Also Ask
What is bitwise & used for?
This bitwise AND (&) is commonly used in programming for tasks like masking specific bits, checking or setting individual bits in a number, and implementing various bitwise algorithms and optimizations. Basically, it performs AND operation between the corresponding bit pairs of the two numbers. That means, it compares each bit of two integers and produces a new integer where each bit is set to 1 only if the corresponding bits in both numbers are 1; otherwise, it sets the bit to 0.
Is bitwise operation faster?
Bitwise operations are often faster than their arithmetic and logical counterparts because they directly manipulate individual bits within data, which the CPU handles efficiently at a hardware level. However, their performance gain might vary based on the specific context and the hardware being used. In scenarios where optimization and efficiency are critical, bitwise operations can offer significant advantages in terms of speed and resource consumption compared to traditional arithmetic or logical operations.
What is XOR used for?
The XOR (exclusive OR) operation is used for toggling individual bits, error checking, and creating masks to extract specific bits from a bit sequence. It returns 1 if the bits are different and 0 if they’re the same. In addition, its properties also make it valuable in algorithms and data manipulation where alternating or selective bit manipulation is required.
How do I add bitwise?
Bitwise addition involves several steps. You start an XOR (^) operation on the bits of the two numbers to add them without considering the carry. Then, utilize the AND (&) operation and left shift (<<) to find the bits requiring a carry. Continue combining the initial XOR result with the carry (using XOR) until there are no more carries left.
What do bitwise operators return?
The bitwise AND operator (&) returns a value where each bit is 1 only if the corresponding bits of both operands are 1. The bitwise OR operator (|) returns a value with bits set to 1 if at least one corresponding bit in the operands is 1. On the other hand, The bitwise XOR operator (^) returns a value with bits set to 1 if the corresponding bits in the operands are different.
What is bitwise complement?
The bitwise complement, often denoted as ~, is a unary operation that flips each bit of its operand. In other words, it transforms 0s to 1s and 1s to 0s. For a binary number A, the bitwise complement is expressed as ~A. This operation is commonly used to invert or negate the bits of a binary value. For example, if A is 00101011, then ~A would be 11010100.
Related Articles
- How to Use Bitwise “AND” Operator in Bash Script [2 Methods]
- How to Use Bitwise “OR” Operator in Bash? [3 Different Cases]
- “XOR” Operator in Bash Scripting [3 Examples]
- Bitwise Shift Operator in Bash Script [3 Examples]
<< Go Back to Bash Operator | Bash Scripting Tutorial
The description of XOR isn’t correct. The chart showing the results of xoring 0 and 0 is correct (get a 0), but the way it is described suggests you should get a 1.
Hi Trevor,
I appreciate your concern and thanks for being with us. However, can you please specify which portion of the description seems incorrect to you? I see the description complies with the truth table and so do the given examples. Feel free to share the relevant sentence or sentences so that I can understand your concern clearly. Have a great day!