FUNDAMENTALS A Complete Guide for Beginners
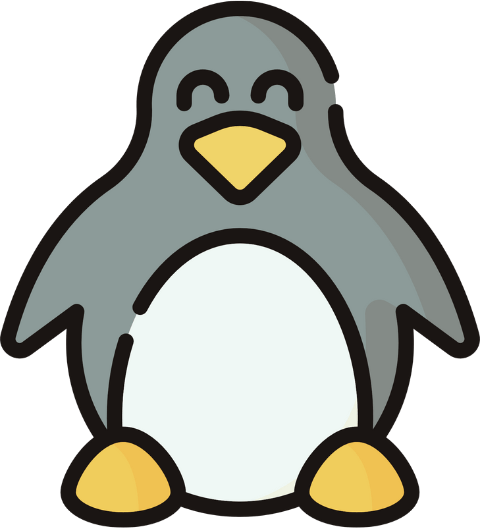
The bitwise AND operator in bash, represented by ‘&’, performs AND operation on corresponding bits of two numbers. It results in a new number where each bit is set to 1 only if the corresponding bits of both operands are also 1. The truth table of AND operation is:
Input A | Input B | Output |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
This operation is particularly useful for extracting specific bits or checking the presence of particular flags within binary representations. In this article, you will learn about bitwise AND Operator in bash and explore its applications through examples. So let’s start!
2 Different Methods to Use Bitwise “AND” Operator in Bash Script
Using the bitwise AND operator in a bash script is one the most straightforward ways to perform with the corresponding bits. It is like doing an arithmetic operation in your script. Of course, you have to do it bit by bit and that’s where the difference lies. There are two different ways to use the bitwise AND operator in a bash script:
Method 1: Bash Bitwise “AND” Operation Using “&” Syntax
You can use ampersand (&) syntax to do bitwise AND operations between two numbers.
Here’s a simple Bash script that takes two numbers and performs a bitwise AND operation:
#!/bin/bash
# Get user input
read -p "Enter the first number: " num1
read -p "Enter the second number: " num2
# Perform bitwise AND
result=$((num1 & num2))
# Display the result
echo "Bitwise AND of $num1 and $num2 is: $result"
The script takes two inputs, storing them in variables num1 and num2. Then, it executes the bitwise AND operation on these two numbers using the & operator. It then assigns the result to the variable result. The script then uses the echo command to print the values of the result.
For user inputs 2 and 3, the script performs a bitwise AND operation. The binary representation of 2 is 0010, and for 3, it is 0011. The result, obtained by evaluating each corresponding pair of bits, is 0010, equivalent to the decimal value 2. Thus, the script outputs: “Bitwise AND of 2 and 3 is: 2”.
Method 2: Bash Bitwise “AND” Operation Using “&=” Syntax
In Bash, the ‘&=’ syntax combines the bitwise AND operation (&) with an assignment (=) in a single operation. When you see ((num1 &= num2))
, it means that the script is performing a bitwise AND operation between the current values of num1 and num2, and then assigning the result back to num1. This script given below demonstrates the usage of &=
for a bitwise AND assignment:
#!/bin/bash
# Example values
read -p "Enter the value to store in num1 variable: " num1
read -p "Enter the second value: " num2
# Perform bitwise AND and assign the result to num1
((num1 &= num2))
# Display the updated value of num1
echo "Updated value of num1 after bitwise AND assignment: $num1"
The script receives two user inputs num1, and num2, and employs the &=
compound assignment operator. It also combines the bitwise AND operation (&) with assignment (=), to update the value of num1. Finally, the script displays the updated value of num1 with the message “Updated value of num1 after bitwise AND assignment.”
For user inputs 2 and 3, the script performs a bitwise AND operation on their equivalent binary representations (0010 and 0011). Using the ‘&=’ syntax, it updates the value of num1 to 2 and outputs: “Updated value of num1 after bitwise AND assignment: 2”.
Managing Access Permissions Using Bitwise “AND” Operation
In Unix-like systems, file permissions are represented using a three-bit binary sequence for each category—owner, group, and others. The binary digits correspond to Read (r): 100, Write (w): 010, and Execute (x): 001. A full set of permissions, like read, write, and execute (rwx), translates to 111 in binary, equivalent to the decimal value of 7. Conversely, the absence of permission results in a binary digit of 0, leading to a permission value of 0 in decimal.
Let’s say you have a scenario where you are managing access permissions using bitwise operations and you want to check if a user has both read and write permissions. Here is a sample file has been made to demonstrate the idea of it:
#!/bin/bash
# Permission constants
READ_PERMISSION=4 # Binary: 100
WRITE_PERMISSION=2 # Binary: 010
# User permissions
user_permissions=6 # Binary: 110 (read and write)
# Check if the user has both read and write permissions using bitwise AND
if [ $((user_permissions & READ_PERMISSION)) -ne 0 ] && [ $((user_permissions & WRITE_PERMISSION)) -ne 0 ]; then
echo "User has both read and write permissions."
else
echo "User does not have both read and write permissions."
fi
In the script, two constants are established to represent read and write permissions. READ_PERMISSION is assigned the decimal value 4, equivalent to the binary representation 100, while WRITE_PERMISSION is assigned the decimal value 2, corresponding to 010 in binary. The user’s permissions are then set to 6, representing both read and write permissions in binary as 110. Then the script employs conditional statements, using bitwise AND operations, to check if both read and write permissions are present in the user’s permissions. If both conditions are met, the script outputs “User has both read and write permissions”; otherwise, it outputs “User does not have both read and write permissions.”
Given that the user’s permissions are set to 6 (binary: 110), the script gives “User has both read and write permissions.” This is because the bitwise AND operation with the READ_PERMISSION (binary: 100) evaluates to a non-zero result. It indicates the presence of read permission. Subsequently, the bitwise AND operation with the WRITE_PERMISSION (binary: 010) also results in a non-zero value, indicating the presence of write permission. Therefore, the script concludes that the user has both read and write permissions based on the specified permission value of 6.
Conclusion
When utilizing the bitwise AND operator, ensure a clear understanding of the binary representation of the numbers involved. Also how the operator affects individual bits to achieve the desired bitwise manipulation. In this article, I have discussed 2 different ways to do bitwise AND operation in a bash script. If you have any questions or queries about this article, feel free to comment below. Thank You!
People Also Ask
What is the use of ‘bitwise &’?
In practical terms, the bitwise AND operator (&) is frequently used for operations that involve isolating, clearing, or checking specific bits in binary representations of numbers. For instance, bitwise AND is commonly employed in:
- Masking: Using a predefined mask to extract or retain specific bits within a binary number.
- Flag Checking: Verifying the status of individual flags in a set of binary flags by combining the bitwise AND operation with flag masks.
- Permission Checking: Assessing and manipulating file permissions in Unix-like systems, where each permission is represented by a bit.
- Color Manipulation: Extracting or modifying color components in graphics programming by applying bitwise AND with color masks.
What is a binary operator in Bash?
In Bash, a binary operator is an operator that operates on two operands, performing a specific operation or computation. Examples include arithmetic operators like addition (+), subtraction (-), and bitwise operators like AND (&) or OR (|).
What is the difference between ‘bitwise AND’ and ‘boolean AND’?
The main difference between bitwise AND (&) and boolean AND (&&) lies in their contexts and the types of operations they perform. Bitwise AND operates on individual bits of binary numbers, performing a bitwise AND operation. On the other hand, a boolean AND applies to conditional statements, returning true only if both operands are true.
Is ‘bitwise AND’ transitive?
Yes, the bitwise AND operation is transitive. In mathematical terms, an operation is transitive if, whenever it applies between three elements, it also applies between the first and third elements directly.
For bitwise AND: If A&B=C and B&D=E, then A&D=F.
In simpler terms, if you perform a bitwise AND operation between two numbers, and separately between the second number and a third number, you can use the result of the first operation with the third number to achieve the same result as if you directly performed the AND operation between the first and third numbers.
Related Articles
- How to Use Bitwise “OR” Operator in Bash? [3 Different Cases]
- “XOR” Operator in Bash Scripting [3 Examples]
- Bitwise Shift Operator in Bash Script [3 Examples]
<< Go Back to Bitwise Operators in Bash Scripting | Bash Operator | Bash Scripting Tutorial