FUNDAMENTALS A Complete Guide for Beginners
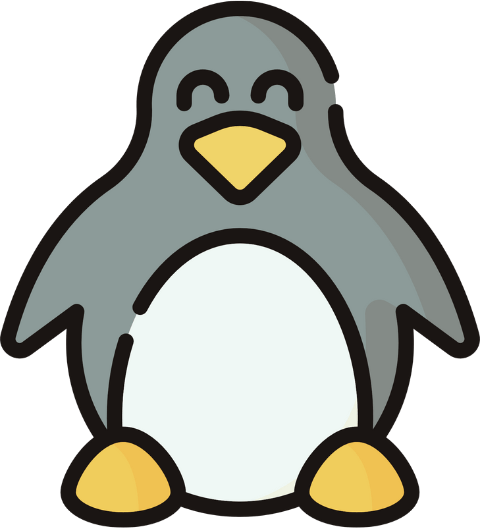
The bitwise shift operators (<< and >>) in Bash manipulate data at the binary level. They are commonly used in low-level programming, such as system-level programming or device driver development, where bitwise operations are used to interact with hardware registers and handle binary data at the bit level. This article aims to help you understand the usage of bitwise shift operators in a bash script with various examples. So, let’s get started!
What are the Bitwise Shift Operators in Bash Script?
Bitwise shift operators are programming tools that move individual binary digits to the left or right. These operators are commonly used in programming languages to manage binary data, simplify multiplication or division by powers of two, and streamline specific numerical tasks.
There are two types of shift operators in the bash script, left bitwise shift and right bitwise shift.
- Left Bitwise Shift (<<): The Left Bitwise Shift (
<<
) operator in Bash shifts the bits of a binary number to the left by a specified number of positions. Each shift to the left effectively multiplies the original number by 2 raised to the power of the shift count. Left shifts are often used for quick multiplication by powers of 2 in binary representation. A typical example of using a left bitwise shift operator in a bash code is as follows:#!/bin/bash x=5 y=2 result=$((x << y)) echo "Result of $x << $y is $result"
Here, The binary representation of 5 is 101. This means that when the number is shifted two positions to the left, it becomes 10100 in binary. In decimal, this is 20. Therefore, the result of the expression
$((x << y))
is 20. In other words, the left shift operation multiplies the original number 5 by 2 raised to the power of the shift count, which is 2. This is equivalent to 5 multiplied by 2 raised to the power of 2, which is 5*2^2 resulting in 20. - Right Bitwise Shift (>>): The Right Bitwise Shift (
>>
) operator in Bash shifts the bits of a binary number to the right by a specified number of positions. Each shift to the right effectively divides the original number by 2 raised to the power of the shift count.An example of its implementation is displayed as follows:#!/bin/bash x=16 y=2 result=$((x >> y)) echo "Result of $x >> $y is $result"
Here, The binary representation of 16 is 10000, and when the bit shifts by two positions to the right, the binary value changes to 100, which is equivalent to 4 in decimal. Therefore, the result of the expression
$((x >> y))
is 4.
3 Practical Examples of Using Bitwise Shift Operators in Bash
Bitwise shift operator is a very efficient and handy way to do binary operations like scaling up and down the number. You can also incorporate a bitwise shift operator to do regular tasks like finding even or odd numbers, converting decimal numbers into binary numbers, toggling a specific bit from the binary combination, etc. Here are three practical examples of bitwise shift operators in Bash:
Example 1: Using Bitwise Shift Operator to Multiply and Divide with the Power of Two
To do the multiplication and division using the bitwise shift operator use the following code:
#!/bin/bash
value=8
multiplied=$((value << 2))
divided=$((value >> 1))
echo "Multiplication of 8 by 2^2: $multiplied, Divided by 2: $divided"
This Bash script assigns the value 8 to a variable. The bitwise left shift operation <<
is then applied to the value by 2 positions, resulting in the variable multiplied storing the value of 8 multiplied by 2 raised to the power of 2. Subsequently, the bitwise right shift operation >>
is used to shift the bits of value to the right by 1 position, and the result is stored in the variable divided. Finally, the script prints the results using the echo command, including a message that shows the multiplication by 2^2 and the division by 2.
As shown in the image, the code outputs 32 for a left shift and 4 for a right shift on decimal 8.
Example 2: Using Bitwise Shift Operator to Convert Decimal to Binary
The conversion of a decimal number to binary involves repeatedly dividing the decimal number by 2 and keeping track of the remainder. The binary representation is obtained by reading the remainder in reverse order.
To convert a decimal number to binary using bitwise shift operators in Bash, you can use the following script as an example:
#!/bin/bash
# Decimal number to be converted to binary
decimal=10
# Initialize an empty string to store the binary representation
binary=""
while ((decimal > 0)); do
#Calculate the least significant bit
bit=$((decimal % 2))
# Prepend the bit to the binary string
binary="$bit$binary"
# Right shift the decimal number by 1
decimal=$((decimal >> 1))
done
echo "The binary value of 10 is $binary"
This Bash script converts decimal 10 to binary using a while loop. It calculates the LSB by adding the remainder of the decimal number divided by 2 to the binary string. The decimal number is right-shifted by one position in each iteration until it becomes 0. It displays the output using the echo command.
As the image shows, the code returns the binary representation of decimal 10 to 1010.
Example 3: Utilizing Bitwise Shift Operator to Toggle a Bit at a Specific Position
Toggling a bit at a specific position means changing its value from 0 to 1 or from 1 to 0 while leaving other bits unchanged. The bitwise shift operator plays a crucial role in this process by creating a bitmask that isolates the bit at the specified position, allowing it to be toggled independently of the other bits.
To toggle a bit at a specific position using bitwise shift operators in Bash, you can use the following approach:
#!/bin/bash
# Function to convert a decimal number to binary
decimal_to_binary() {
local decimal=$1
local binary=""
while ((decimal > 0)); do
bit=$((decimal % 2))
binary="$bit$binary"
decimal=$((decimal >> 1))
done
echo "$binary"
}
# Prompt the user to enter a number and position
read -p "Enter a decimal number: " user_number
read -p "Enter the position to toggle (starting from 0): " user_position
# Toggle the bit at the specified position
result=$((user_number ^ (1 << user_position)))
# Convert the original number and the toggle value to binary
original_binary=$(decimal_to_binary $user_number)
toggle_binary=$(decimal_to_binary $result)
# Output the results
echo "Original number in binary: $original_binary"
echo "Toggle value in binary: $toggle_binary (toggling bit at position $user_position)"
echo "Decimal value after toggle: $result"
In this Bash script, a function named decimal_to_binary is defined to convert a decimal number to binary. The script then prompts the user to enter a decimal number and a position to toggle. It uses the bitwise XOR operator (^) and the left shift operator (<<
) to toggle the bit at the specified position, storing the result in the variable result. The script calls the decimal_to_binary
function to convert both the original decimal number and the toggle result to their binary representations. Finally, the script outputs the binary representation of the original number.
From the image displayed above, you can observe that the binary representation of the user input 9 is
1001
. The flip operation is performed on the binary representation in the index position 2 (which is the third bit). After the flip, the binary representation returns a new value 1101
where the third bit has been flipped from 0 to 1. This new binary value results in the decimal value 13.
Conclusion
To conclude, the bitwise shift operators (<< for left shift and >> for right shift) in Bash offer an efficient way to manipulate binary data. These operators prove beneficial for tasks such as rapid multiplication or division by powers of 2. Bash scripting frequently utilizes them in bitwise operations to optimize numerical computations as well. This article provides a comprehensive demonstration of the bitwise operator in bash with various examples. If you have any questions or queries related to this article, feel free to comment below. Thank you!
People Also Ask
What is << in bitwise?
In bitwise operations, << is the left bitwise shift operator. It shifts the bits of a binary number to the left by a specified number of positions. The operation is equivalent to multiplying the original number by 2 raised to the power of the shift count.
For example, if you have the binary number 1010
and perform a left shift by 2 (1010 << 2
), the result will be 101000
, as each bit is shifted two positions to the left, effectively multiplying the original decimal value by 4.
How to do bitwise shift?
The bitwise shift in Bash is performed using the <<
(left shift) and >>
(right shift) operators. The << operator shifts the bits of a number to the left, effectively multiplying the number by 2 raised to the specified power. Similarly, the >> operator shifts the bits to the right, resulting in division by 2 raised to the specified power. For example, in the expression a << b
, the bits in the binary representation of a are shifted to the left by b positions, and in a >> b
, the bits are shifted to the right by b positions.
What is the right shift operator in bash?
The right shift operator (>>) is one type of bitwise operator in the bash script. A bitwise shift operator shifts the bits of a binary number to the right by a specified number of positions. The right shift operation effectively divides the original number by 2 raised to the power of the shift count.
For example, if you have a variable x and you want to right shift its bits by 2 positions, you can use the syntax:
#!/bin/bash
result=$((x >> 2))
This operation will shift the bits of x two positions to the right, resulting in a division of x by 2 raised to the power of 2. The variable result stores the outcome. In Bash scripting, the right shift operator is a common choice for bitwise operations and numerical optimizations.
Does the left shift multiply by 2?
Yes, the left shift operation (<<) effectively multiplies a number by 2 raised to the power of the specified shift count. When you left shift a binary number by one position, it is equivalent to multiplying the decimal value by 2. If you left shift by two positions, it is equivalent to multiplying by 2 raised to the power of 2 (2^2), and so on. Therefore, left shifting by ‘n’ positions is equivalent to multiplying the number by 2^n.
How do you divide by 4 in bit shift?
To divide a number by 4 using bitwise shift operations, you perform a right shift by 2 positions. In binary representation, a right shift by 2 is equivalent to dividing by 2^2 (which is 4 in decimal). So, if you have a variable x, the operation x >> 2 will result in x being divided by 4.
Related Articles
- How to Use Bitwise “AND” Operator in Bash Script [2 Methods]
- How to Use Bitwise “OR” Operator in Bash? [3 Different Cases]
- “XOR” Operator in Bash Scripting [3 Examples]
<< Go Back to Bitwise Operators in Bash Scripting | Bash Operator | Bash Scripting Tutorial