FUNDAMENTALS A Complete Guide for Beginners
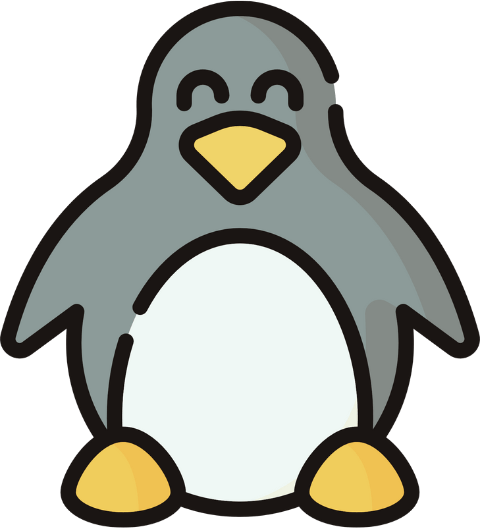
The XOR operator is a powerful bitwise operator in bash scripts that can be used to do a lot of different things. It can be used for simple encryption, dynamic bit manipulation, and more. In this article, you’ll look at what the XOR operator is and how it can be used in bash scripts. You’ll also look at how to use the XOR operator to do things like bitwise flipping. So let’s start!
What is “XOR” Operator in Bash?
XOR is a binary operator that performs the operation “exclusive OR” on the respective bits of two integer values. In binary representations, the result of XOR is “1” where the two operands have different values, and “0” where they have the same values.
The truth table for the XOR operator is as follows:
Input A | Input B | Output |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
In Bash, the XOR operator is represented by the caret symbol (^). The basic syntax for using the XOR operator in Bash is:
result=$((operand1 ^ operand2))
In this syntax, the XOR operation is performed on the bits corresponding to operands operand1
, operand2
, and the result is an integer.
It is important to note that the operands operands must be integers in decimal, octal, hexadecimal, etc., and the result will be an integer. XOR operation is used to flip the bits where only a single operand has a matching bit set.
3 Examples of Using “XOR” Operator in Bash Scripting
The XOR operator in bash is essential for performing bitwise operations in scripts. It has various use cases, such as bit toggling, simple bit encryption, flag handling, data transmission error checking, and so on. However, this article will focus on bit toggling, string encryption-decryption, and bit masking for simplicity and beginner-friendliness. Refer to the examples below to get a better understanding of how the XOR operator works in a bash script.
Example 1: Using “XOR” Operator to Flip Individual Bits
To do a bitwise manipulation for flipping a specific bit in a numerical value, use the following bash code:
#!/bin/bash
# Original value
read -p "Enter your original value: " original_value
# Bit position to flip (0-indexed)
read -p "Enter your index position: " bit_position
# Creating a bitmask with the target bit set to 1
bitmask=$((1 << bit_position))
# Flipping the target bit using XOR
result=$((original_value ^ bitmask))
echo "Original Value: $original_value"
echo "Flipping the bit at position $bit_position"
echo "Result: $result"
This Bash script takes user input for an original numerical value and a specified bit position (0-indexed). It then creates a bitmask by left–shifting the value 1 by the provided bit position, effectively setting the target bit to 1. Subsequently, it flips the target bit of the original value using the XOR bitwise operation with the bitmask, resulting in a modified value. The script prints the original value using the echo command, indicates the bit-flipping at the specified position, and displays the resulting value after the XOR operation.
As you can see from the image, integer 8 is needed to be flipped for the 3rd bit (index position is 2). Given that the original number is 8 (binary: 1000), flipping the 3rd bit (the second switch) results in the new number being 12 (binary: 1100).
Example 2: Using of “XOR” Operator to Create a Mask
In the context of computing, a mask is a pattern of bits that is used for various operations, often in combination with bitwise operations. The purpose of a mask is to select or modify specific bits within a binary representation of a value.
To create a mask with a specific bit set to 1, you can use the left shift (<<
) operator in combination with the bitwise OR (|) operator. Here’s an example in Bash:
#!/bin/bash
# Bit position to set to 1 (0-indexed)
bit_position=3
# Creating a mask with the target bit set to 1
mask=$((1 << bit_position))
echo "Bit Position: $bit_position"
echo "Mask: $mask"
The given bash script initializes a variable bit_position
with the value 3, representing the bit position to be set to 1 (0-indexed). Then, it creates a mask by left–shifting the value 1 by the specified bit position, resulting in a binary mask with only the target bit set to 1. The script prints the selected bit position and the generated mask using an echo command.
For the given bit position of 3, the binary representation of mask 8 is 00001000. This indicates that only the bit at position 3 is set to 1.
Example 3: “XOR” Hex String Encryption and Decryption in Bash Script
Hexadecimal string encryption and decryption in Bash typically involve converting characters to their hexadecimal representations. It also performs bitwise operations and then converts the results back to characters.
To encrypt or decrypt any string value, use the following bash code:
#!/usr/bin/env bash
read -p "Enter your String which you want to encrypt: " user_input
echo "Your given text is: $user_input"
encrypted_text=""
for ((i=0; i < ${#user_input}; i++))
do
char_code=$(printf '%d' "'${user_input:$i:1}")
encrypted_char=$((char_code ^ 90))
encrypted_text="${encrypted_text}$(printf \\$(printf '%03o' "$encrypted_char"))"
done
echo "Your encrypted code is: $encrypted_text"
# now XOR again and we should get the original string back
decrypted_text=""
for ((i=0; i < ${#encrypted_text}; i++))
do
char_code=$(printf '%d' "'${encrypted_text:$i:1}")
decrypted_char=$((char_code ^ 90))
decrypted_text="${decrypted_text}$(printf \\$(printf '%03o' "$decrypted_char"))"
done
echo "The given text decoded from the encrypted code is: $decrypted_text"
This Bash script begins by prompting the user to input a string, storing it in the variable user_input
. The script then initializes an empty string, encrypted_text
, to store the result of the encryption process.
Then, it iterates through each character in the input string, obtaining the ASCII code of each character and performing an XOR operation with the value 90
. The encrypted ASCII codes are then converted back to characters and appended to the encrypted_text
string.
After it initializes an empty string, decrypted_text, to store the decrypted result. Then it iterates through each character in the encrypted string. The ASCII code of each character is XORed with 90
, and the decrypted ASCII codes are converted back to characters, which are then appended to the decrypted_text
string. Finally, the script prints the decrypted string.
As you can see from the image, the provided bash code encrypts the LinuxSimply to 34/
“     37*6#. The encrypted code is further decoded by the script and returns the initial string value LinuxSimply to the command line.
Conclusion
In conclusion, the XOR is one of the most useful and powerful operators in bash scripting. The article has covered the essential role of the XOR operator in bash bitwise operations by breaking down its syntax and showing practical examples that prove its importance in scripting. However, if you have any questions or queries related to this article, feel free to comment below. Thank you!
People Also Ask
What does bitwise “XOR” do?
Bitwise XOR (exclusive OR) is a binary operation that results in a 1 where the corresponding bits of the operands are different and 0 where they are the same. In other words, if a bit is 1 in either operand but not both, the XOR operation sets that bit to 1; otherwise, it sets the bit to 0.
How to implement Bitwise “XOR”?
To implement bitwise XOR in a programming language like Bash, you can use the caret (^) symbol. For example:
result=$((a ^ b))
This XOR operation will set each bit in the result to 1 where the corresponding bits in ‘a’ and ‘b’ are different, and 0 where they are the same.
Does the bitwise “XOR” operator only return 0 or 1?
Yes, the bitwise XOR operator (^) returns 0 for bits that are the same in both operands and 1 for bits that are different.
How do you find the Bitwise “XOR” of a number?
To find the bitwise XOR of two numbers, you need to compare each corresponding pair of bits. If the bits are different, the result bit is set to 1; if they are the same, the result bit is set to 0. This process is repeated for each pair of bits, starting from the rightmost bit.
Consider the task of finding the bitwise XOR of two decimal numbers, 5 and 3. First, convert each number in binary: 5 is 101, and 3 is 011. Now starting from the leftmost bit, compare each pair of bits. When the bits differ, the corresponding result bit is set to 1; otherwise, it is set to 0.
In this case, the first bits are different (1 and 0), so the result bit is 1. Moving to the next pair, both bits are different, so the result bit is 1. Finally, the rightmost bits are the same (1 and 1). It results in a final XOR of 110 in binary, which corresponds to 6 in decimal. Therefore, the bitwise XOR of 5 and 3 is 6.
What is the string “XOR” function?
String XOR functions perform the bitwise xor operation on each of the corresponding pairs of characters in the two strings. The xor operation is often used in cryptography or computer science for the simple purpose of encryption or hashing.
In string XOR functions, each character of one string is xorred with the character of another string, resulting in a new string. The xOR operation offers a reversible transformation. This means that repeating the same xor operation on the result will return the original string. String XOR functions are often used in basic encryption or encodings where bitwise manipulations are performed on the character level.
Related Articles
- How to Use Bitwise “AND” Operator in Bash Script [2 Methods]
- How to Use Bitwise “OR” Operator in Bash? [3 Different Cases]
- Bitwise Shift Operator in Bash Script [3 Examples]
<< Go Back to Bitwise Operators in Bash Scripting | Bash Operator | Bash Scripting Tutorial