FUNDAMENTALS A Complete Guide for Beginners
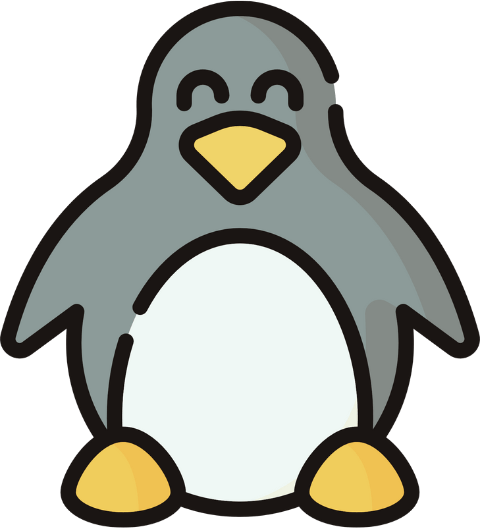
In Bash scripting, variable scope is a crucial context that determines the visibility of variables. It allows you to manage the variables’ usage, prevent unexpected conflicts, control data encapsulation, and organize codes in a modulated way. Therefore, read the following article to get a wholesome concept of variable scopes in Bash.
What is Variable Scope in Bash?
The variable scope is the regional context within a Bash script or program that represents a variable’s existence and use. This scope dictates where the variables are valid and you can easily access and modify them within the code. This essential feature actually controls the data encapsulation within functions and prevents unintended naming conflicts within Bash programs.
2 Basic Types of Variable Scopes in Bash
In the following section, you will find two primary types of variable scopes that affect a variable’s existence and accessibility in different ways within the Bash scripts. Let’s see a detailed demonstration:
1. Local Scope for Variable
Local scope for variable defines a variable that is accessible only inside a function or code block where they are declared. Even you cannot access the variable from any other function in which it is not properly defined. These local variables exist only during function execution. As they exist for a limited lifetime, once the function terminates, you can no longer access their values.
Following is an example regarding the local scope for the bash variable:
#!/bin/bash
function1() {
 local local_variable="Hello, Linux!"
 #Accessing the local variable ‘local_variable’ inside the functio
 echo "Local variable inside the function: $local_variable"
}
function1
#Accessing the local variable ‘local_variable’ outside the function
echo "Local variable outside the function as error: $local_variable"
This script indicates the access of a declared local variable from both inside and outside a function and then displays the outputs employing the echo command.
The above image depicts the local variable scope that you can access only inside the function.
2. Global Scope for Variable in Bash
Global scope for variable defines a variable that you can access from any place of the current script or process except the sub-processes. Generally, global variables are declared outside a function but can be accessible from both inside and outside the function. These variables exist permanently throughout the lifespan of the current script and any section of the program can use the values of the variables.
Here’s an example of the global scope for the bash variable:
#!/bin/bash
#Defining a global variable
global_variable="I am a global variable"
function2() {
 #Accessing the global variable ‘global_variable’ inside the function
 echo "Global variable inside the function as error: $global_variable"
}
function2
#Accessing the global variable ‘global_variable’ outside the function
echo "Global variable outside the function: $global_variable"
The above script represents the access of a defined global variable from both inside and outside a function and then uses the echo
command to display the outputs.
The above image depicts the global variable scope that you can access from inside the function as well as outside the function.
Unbound Variable – An Error!
Whenever you try to access or use any variable that is not declared or initialized yet, you will undoubtedly encounter an error called the unbound variable error. This error occurs when the variable does not exist and Bash can not refer any value to the unassigned variable.
#!/bin/bash
#Attempting to use an undefined variable
echo "The variable is: $var"
The script above demonstrates an unbound variable error due to the undefined variable var and displays the output using the echo
command.
The above image is an illustration of an unbound variable error that is the consequence of accessing an undefined variable.
2 Cases of How to Change Bash Variable Scope
In Bash, changing variable scopes is not a general and frequent task as it makes your code less obvious and leads to unintended variable conflicts. But if you have a justified reason for doing so, you can proceed with the variable scope conversion judiciously but make sure to document your scripts with clarity.
Anyway, the following section indicates two cases for changing scope from global to local and local to global. Let’s have a look!
1. Changing Scope From Global to Local
This case is about changing the variable scope from global to local. To convert a global variable to a local variable, you need to use the local keyword and declare the variable with the same name within the function. This local variable shadows the previous global variable inside the function and its scope remains limited to the function where the global variable maintains its value outside the function. This is known as the variable shadowing concept.
Here’s a bash script to change the variable scope from global to local:
#!/bin/bash
#Defining a global variable
global_var="Hello, Linux!"
function my_func() {
 #Changing the scope of 'global_var' to local
 local global_var="Hi, Ubuntu!"
 #Accessing 'global_var' inside the function
 echo "Local variable : global_var = $global_var"
}
my_func
#Accessing the global variable 'global_var'
echo "Global variable : global_var = $global_var"
This script first defines a global variable and then converts it to a local variable by using the local keyword inside a function. Finally, it echoes the results from both inside and outside the function to see the difference.
From the image, you can see the conversion of scope from global to local where the local variable within the function takes precedence over the global variable outside the function.
2. Changing Scope From Local to Global in Bash
You can convert the scope from local to global by employing the declare command with the -g
 option within a function. It allows you to declare a global variable inside the function that is accessible from anywhere in the script.
Let’s see the bash script of how to change the bash variable scope from local to global:
#!/bin/bash
function my_func() {
 local local_var="Hello, Linux!"
 #Changing the scope of 'local_var' to global
 declare -g global_var="$local_var"
}
my_func
#Accessing 'global_var' outside the function
echo "Global variable : global_var = $global_var"
Here, the script first defines a local variable inside a function using the local keyword and then converts it to a global variable by using the declare
command with the -g
 option inside the function. Finally, the script terminates by displaying the output using the echo
command.
From the image, you can see the conversion of scope from local to global where you can access the global variable even from outside the function.
Conclusion
To conclude, it’s recommended to employ the variable scopes properly to prevent unexpected conflicts within the bash scripts. Use local variables within functions to limit their scope and utilize global variables for cases when data needs to be shared across different sections of the program.
People Also Ask
Does Bash allow to make a global variable local within a function?
Yes, Bash allows making a global variable local within a function. To do so, use the local keyword and declare the variable as a local variable within the function.
For how long does a local variable exist in Bash?
A local variable is only available for a limited lifetime in Bash. It exists only during the function’s execution.
Can I access global variables from within functions in Bash?
Yes, you can access global variables from within functions in Bash as they are accessible from anywhere in the script.
Are there any ways to create limited scope variables except local variables in Bash?
No, there are no default ways to create limited scope variables except local variables within a function in Bash. But if you want to create limited scope variables outside of the function for particular cases, you can use temporary files or create a subshell using parentheses.
Related Articles
- Insights to Local Variable in Bash [4 Practical Examples]
- A Total Guide on Global Variable in Bash [3 Examples]
- How Unbound Variable Error Occurs in Bash [Cases & Solutions]
<< Go Back to Bash Variables | Bash Scripting Tutorial