FUNDAMENTALS A Complete Guide for Beginners
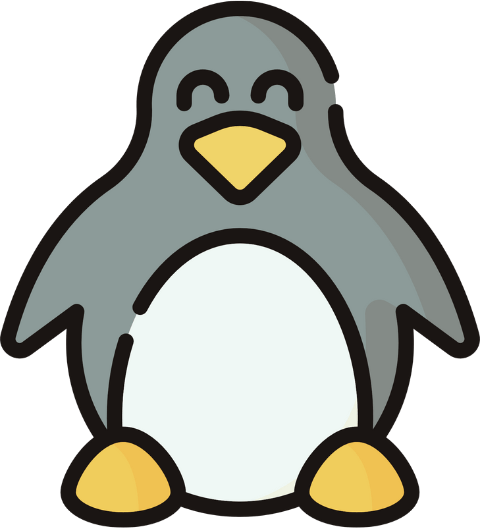
Bash string basics refer to the basic idea and operations related to string which is the sequence of characters. It is important to know about string basics for effective text manipulation and shell scripting. Let’s see some basic information about string and how to manipulate text.
What is Bash String in Linux?
A bash string in Linux is a sequence of characters that is enclosed by either a single, double, or even no quotations. A string containing space will show an unexpected behavior if it has no quotation. In single quotes, each character is treated as a literal value. For example, the characters “@” and “*” are treated in single quotes as literal values.
But double quotes allow variable expansion and command substitution, and @ and * have a special meaning in double quotes which are parameter expansion at certain constructs and word splitting. In the double quote, each character is not treated as a literal character.
Thus, the bash string is represented utilizing the general idea of variables. In addition to being a unique data type like integers and booleans, typically a bash string is used to represent text.
Create Different Types of Bash String
Creating a bash string refers to assigning a character or a sequence of characters into a variable. This variable can be read, searched, and edited as textual data. A bash string can be quickly and simply created with single quotes(”) and double quotes (” “). You can create multiline strings using here document and new line characters. Depending on creation there are different types of strings:
- Plain text
- Single-Quoted
- Double-Quoted
- Here Document
Except for these types, there are some other kinds of strings.
Below, let’s take a look at the methods for creating some different types of string:
A. Plain Text String Without Quotes
Plain text strings are the basic type of string. It consists of alphanumeric characters, symbols, and punctuation marks. The string represents text data as messages, file contents, and user input.
To create a plain text string, use a variable following the assign operator. Here is how:
#!/bin/bash
string=Hello1234!
echo $string
The string=Hello1234!
assigns the string into the string variable using the assign operator.
From the output, you can see the plain text string has been created and printed on the
B. Using Single Quotes
Single Quotes are used to create a string in which every character within the single quote is treated as a literal character. It is useful for including special characters or preventing misinterpretation.
To create a string using a string using single quotes copy the following code:
#!/bin/bash
string1='Hello World!'
string2='It’s a new string.'
echo $string1
echo $string2
Two different strings are assigned to two different variablesstring1
andstring2
. The$
is used to make the variable accessible. The echo command is used to print the strings in the terminal.
With the single quotes, a single-line string has been created.
C. Using Double Quotes
Double Quotes are used to create a string that allows variable expansion, command substitution, and interpretation of escape characters within the string. To include dynamic content and format the output, double-quoted strings are convenient.
To create a string using a string using double quotes copy the following code:
#!/bin/bash
string1="This is another string"
echo $string1
name=$(whoami)
echo "The name of the user is $name"
Using the double quotes string1 has been declared. In the$(whoami)
,command substitution$()
has been used to execute the whoami command which returns the current user name, and this value is assigned to the name variable. In the echo
command, the string with the name variable will be printed.
From the output, you can see with the double quotes one can easily create a single line string.
Note: As one knows a command substitution string allows one to execute a command and embed its output directly within a string. It uses it as part of a string or assigns it to a variable. The basic syntax of command substitution is $() and backticks (`) . In this above example, name=$(whoami)
is the command substitution string which is printed in double quotes usingecho
command.
D. Multiline String Using New Line Character
The\n
is an escape sequence representing a newline character. When the\n
is used in string it is interpreted as a command to start a new line.
To create a multiline string use the following code:
#!/bin/bash
echo "Using new line character"
multiline="Hello World!\nIt’s a new string.\nThis is another string."
echo -e "$multiline"
The\n
is the newline character which is used to separate lines. To interpret the backslash escape the -e
option is used with the echo command.
Using the newline character you can create a multiline string.
E. Multiline String Using Here Document
A here document is a feature that allows one to include a block of text without the need for explicit quoting and newline characters. The text is enclosed with<<
and the delimiter.
Using here document you can create a multiline string. Here is how:
#!/bin/bash
Multilinestring=$(cat << EOF
Hello World!
It’s a new string.
This is another string.
EOF
)
echo "$Multilinestring"
The$(cat << EOF…EOF)
is the here document that creates a multiline string. The cat command read the text between<<EOF
andEOF
. The string in the Multilinestring variable will be printed using theecho
command.
From the output, you can see with the here document, you can print multiline strings.
Read String in Bash
The read command processes the lines of the file and assigns each line to a variable. The syntax of the read command is read [option] [variable]
. The options of the read command are “-r”, “-n”, “-t”, and “-p”. This command takes the value provided by the user and stores it in the name variable.
To read a string with the read command, you can follow a few different processes just as shown below:
Reading From User Input into specific limit >
#!/bin/bash
# Prompt the user to enter a string
echo "Enter a string: "
read -n 24 userInput
# Display the entered string
echo -e "You entered: $userInput"
Theread
command takes the input from the user and stores it in the userInput
variable and the-n
option with theread
command limits the character which is denoted as 24. Then with theecho
command, it is printed on the terminal, and the-e
option is used to interpret the escape character.
In this process, if you enter a string it will print the same result and you can read it from the command line.
Reading From Prompt >
#!/bin/bash
# Read a string with a prompt
read -p "Enter a string: " userInput
# Display the entered string
echo "You entered: $userInput"
The -p
option with the read command will allow to prompt directly without any echo
command. The entered string is stored in the variable, userInput. To display the string echo command has been used.
You can also read strings like passwords silently using the-s
option with theread
command. The-s
option hides the string that one entered and provides a more secure way to read string without displaying it.
String Interpolation in Bash
String interpolation is the process of embedding variable values and expressions into the string. It is important to create dynamic strings by incorporating the values of variables or command substitutions directly into the string.
To do the string interpolation use the curly brackets to print nonspace characters immediately after the variable:
#!/bin/bash
food="apple"
echo "I eat ${food}s"
Thefood="apple"
declares a variable named food and assigns the string “apple” to it. The ${food}s
indicates the parameter expansion with the food variable with a character “s” for string interpolation.
The output shows that the character “s” has been append immediately after the apple variable.
string1=$(date)
But, you should note that it is not the right way for string interpolation within a double-quoted string.
To print specific substring from a string, use the parameter expansion. The parameter expansion has also been used in the string interpolation. Parameter expansion is a way to perform various operations on the values of variables. To extract a value from a substring, ${variable:offset:length} form of expansion is used.
To extract a specific substring use the following code:
#!/bin/bash
original_string="The extracted substring."
substring=${original_string:4:9}
echo "Original String: $original_string"
echo "Substring: $substring"
The ${original_string:4:9}
extracted a substring from the original string using the parameter expansion. The substring length is 9 and it starts after the $4th character of the original string or from the 5th character of the original string.
From the output, you can see the extracted substring from the input.
List of Strings in Array in Bash
The list of strings refers to an array that has multiple values and that values correspond to strings. One can use an array to access and manipulate the elements in it. Let’s see how to see the list of strings in bash:
#!/bin/bash
# Declare an array of strings
myList=("Apple" "Banana" "Orange" "Grape")
# Access individual elements
echo "First item: ${myList[0]}"
echo "Second item: ${myList[1]}"
# Print all elements
echo "All fruit items: ${myList[@]}"
In the variable myList array is declared with the first bracket and each string is separated with space. The${}
is the parameter expansion in whichmyList[@]
indicates all the items in the array. Replacing the@
with 0
and1
, the first and second strings can be shown.
The items in the array have been shown. You can also display each item in the list or iterate over the items using a loop.
Applications of Strings
Strings are essential in many shell scripting applications. Here are a few applications shown below:
Variable Manipulation
Data Storage: Data can be stored using strings in a variable. This enables to access the string and makes the manipulation process simple and effective.
Argument Passing: For more flexible and modular functions and scripts, strings are used as the argument source.
User Input and Output
Read User Input: By using the read command users can easily take input from the command line enabling the user to communicate with the script.
Information Display: To display information such as print output, and error messages on the terminal, the string is used with the echo command.
Output Formatting: To format the string output, concatenation, and slicing can be applied to the string. The printf command is used to format the string output.
Text and File Processing
File Reading and Writing: To read and write files strings are used widely. String plays a crucial role in storing, manipulating, and transferring textual data between the script and files.
Text Processing: To process text, you can use string to extract a specific word, split the string into substrings, and replace the word in a string.
Search for a File and Text: To search for a specific pattern of file or a text from the file, strings are used with grep and sed commands to search for the files and texts.
Extraction of Information: To retrieve specific information from a file, strings can be parsed and extracted.
Automation and Command Substitution
To automate the complex task, dynamic commands are created and executed using strings. It can be used in command substitution to capture the output of other commands into the script.
Error Handling and Debugging
To print the error message, strings can be used to inform the users. The user can also use string to provide the debugging information. With string, the user can write and store the logs and track the execution of the script of debugging.
Difference Between Single Quotes and Double Quotes in Bash String
Both single quotes and double quotes are used to declare a string. Based on how they handle special characters within it, there are some differences which have been shown below:
Parameter | Single Quote | Double Quote |
---|---|---|
History expansion | Does not allow. | Allow with the “!” character. |
Command Substitution | Does not allow. | Allow. |
Variable expansion | Does not allow. | Allow. |
Escape character | When the escape characters (\) are enclosed with a single quote, then the literal meaning of the characters is intact. | The $,(`),(‘’), and (\) characters in the double quotes can be escaped with “\”. |
Array access | Can not access it. | Can access it with double quotes. |
One quotation into another quotation | Single quotes in double quotes have no special meaning. And one can not use a single code in single quotes. | Double quotes are literally treated within the single quotes. |
Special characters | The literal meaning of special characters like @ and * in the single quotation. | The @ and * has special meaning when it is in double quotes. |
Conclusion
In this article, the fundamental concept of string has been shown. It is important for a solid understanding of string operations to be crucial for effective and efficient bash scripting. In this article, one can learn about different types of strings, string importance, and the creation of strings. Hope the user can learn the string basics properly.
People Also Ask
How to initialize a string in Bash?
To initialize a string you can directly use a variable name in which the string will be assigned followed by the assignment operator and the desired string enclosed with single or double quotes. Here is how:
#! /bin/bash
string="Here it is."
echo $string
It will print the output of the variable which is the string.
What is the difference between [[ $string == “efg*” ]] and [[ $string == efg* ]] ?
The [[ $string == "efg*" ]]
determines whether the string is equal to the variable string. "efg*"
is interpreted as a literal character since the double quotes around it stop the shell from expanding the asterisk (*) as a wildcard.
The[[ $string == efg* ]]
checks if the string starts with efg. The asterisk (*) functions as a wildcard here, matching any characters that come after"efg"
.
What is the += for strings in Bash?
The+=
operator is used to concatenate a string with another string. It will concatenate the right-hand string to the left-hand string. Here is how:
#!/bin/bash
string1="Hello"
string1+="5"
echo "$string1"
Here the string “5” will be merged to the left of the initial string Hello.
How do I declare an empty string in Bash?
To declare an empty string, use an empty quote of set in a variable. How it looks:
string1=" "
You can use{:=}
parameter expansion to declare an empty string. The parameter expansion has been used to set a default value for a variable only if the variable is unset or its value is empty. Here is how:
variable=${variable:=default_value}
If the variable is empty or unset then it will be assigned the value of default value. And if the variable is set or not empty then it will remain unchanged.
Related Articles
- String Interpolation in Bash [4 Methods]
- List of Strings in Bash [Declare, Iterate & Manipulate]
- How to Escape Special Characters in Bash String? [5 methods]
<< Go Back to Bash String | Bash Scripting Tutorial