FUNDAMENTALS A Complete Guide for Beginners
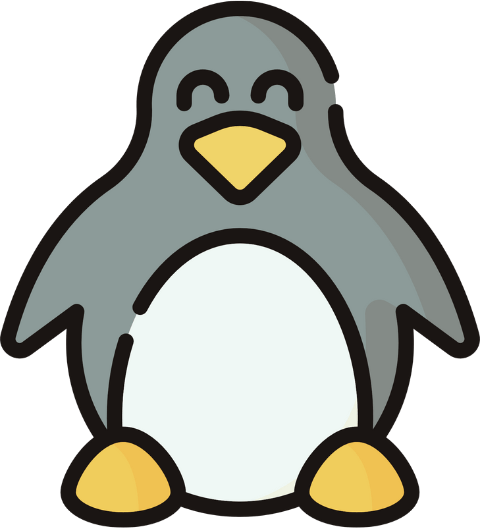
A list of strings is a collection of a group of elements that are arranged in a specific order. In bash scripting, you can consider an array as a list and the array elements as strings. A string that is separated by space and special characters is also considered a list of strings. In this article, you will learn how to declare, iterate, and modify a list of strings in bash scripting.
Declare and Print a List of Strings
Declaration of a list of strings means assigning the value of a string in a variable using space between the elements and using parentheses while declaring an array. As in Bash, an array can be used to represent a list. When you need to print a group of elements at a time and modify the input according to your preference, you can declare them in a list through an array and later print that array of elements.
To declare and print a list of strings use the following code:
#!/bin/bash
# Read a string from user input
echo "Enter a list of strings (separated by spaces):"
read input_string
# Split the input string into an array
IFS=' ' read -ra my_strings <<< "$input_string"
# Display the original list
echo "Original list: ${my_strings[@]}"
Firstly the echo command prints a prompt to the user. Then the read
command reads the user input and stores it in the input_string variable. Here the Internal field separator (IFS) is set to a space to separate the elements of a string. Theread
command option-r
prevents backslash interpretation and-a
treats the input as an array. And, the operator<<<
is a here-string that inputs the content of the input_string into the read command. At last the"Original list: ${my_strings[@]}"
prints the list of strings.
From the output, you can see the script prints the items of the declared list.
Iterate Over a List of Strings
Iterating over a list indicates accessing and processing each item of the list with each iteration. By iterating over a list, you can able to access and print each element, and split and sort the elements of the list. You can iterate each element of an array and also the elements of a string based on space and special characters. Let’s see some of the methods to iterate over the list in bash.
Iterate a List of Substrings in a String
- To iterate a list of substrings in a string use the following code:
#!/bin/bash # Read a string with spaces using for loop for value in I love jackfruit do   echo $value done
EXPLANATIONFirstly the for loop iterates over the string list separated by space. Then the loop takes each value in the variable named “value”. Lastly, the
echo
command prints each element of the string one by one.The output shows each element after iterating over the input list.
- To iterate the value of a string list, you can keep the string in a variable just like below:
#!/bin/bash # Define a string variable with a value String="This is a list of string" # Iterate the string variable using for loop for sub in $String; do echo $sub done
EXPLANATIONInitially, the bash script declares a string in a variable named String. After that, the for loop iterates over each element of the declared string and prints it using the
echo
command.Upon the script execution, the iterated elements are printed on the terminal.
- To list the multiple input strings follow the code below:
#!/bin/bash for str in "Apple" "Orange" "Jack-fruit" "Passion fruit" do echo "String: $str"; don
EXPLANATIONFirstly the loop variable
str
 takes the values in the list and then it prints the elements in each iteration inside the for loop.From the image, you can see the script shows multiple strings that are declared as an input string in the loop.
Iterate an Array List
In bash scripting, a string array is declared with parentheses () and the elements of the array are declared within quotes.
To iterate an array list you can follow the code:
#!/bin/bash
stringarray=("This" "is a" "new created" "string")
for str in "${stringarray[@]}"
do
echo "String: $str";
done
At first, the bash script declares an array in the stringarray variable. The for loop iterates over the array elements. Later the loop variablestr
takes each element in each iteration and prints that element.
The output shows each element of the array in the same declared sequence.
Note: You can use quotes just like this"${stringarray[*]}"
to avoid word splitting when there is space between the variable names.
Iterate Over Delimiter Separated String
A delimiter is a character that is used to separate multiple elements in a single string. You can use space ( ), comma (,), hash (#), colon (:), and other special characters as delimiters. To iterate over a string based on the delimiter, store the value of the delimiter in IFS (Internal Field separator). Take a look at the code below:
#!/bin/bash
lists="There are 31 mangoes,12 jackfruit, and 5 pineapples in the basket."
default_IFS="$IFS"
IFS=","
for i in $lists
do
   echo $i
done
Firstly thedefault_IFS="$IFS"
line stores the current value of the IFS into thedefault_IFS
variable. The script sets the “,” as a temporary IFS. later in the for loop, the script displays the list of elements separated by the comma.
You can also use-
," "
,@
, and .
as the delimiter in this script and it lists the elements based on these characters.
Manipulation of List of Strings
Manipulation is the process of modifying the string in the list and it includes the accession of each element, inserting and removing elements, and checking elements of the list. Let’s see some of the manipulation examples below:
Access to Specific Elements in the String List
To access specific elements in a string list, you can use array indexing. The syntax of array indexing ${my_strings[@]}
will access all the elements of the list. To access a specific element, replace the@
with the instance 0, 1, 2. The array index starts with 0
. So if you are using 0 then it accesses the first element of the list. Here is how it works:
#!/bin/bash
my_strings=("apple" "banana" "cherry" "date")
# Access specific elements
echo "First element: ${my_strings[0]}"
echo "Second element: ${my_strings[1]}"
Initially, the bash script declares an array with four elements. Here, the${my_strings[0]}
accesses the first element of the array and displays it in the terminal with anecho
command. And the${my_strings[1]}
accesses the 2nd element.
The output shows the first and second elements of the string list.
Insert a New String Into an Existing List
To insert a string into a list, use the+=
operator. The operator adds a new element to the existing list without changing the previous elements. Here look at the code below:
#!/bin/bash
my_strings=("apple" "banana" "cherry" "date")
# Add a new element
my_strings+=("grape")
# Print the updated list
echo "Updated list: ${my_strings[@]}"
Initially, the bash script declares an array named my_strings with four elements. In my_strings+=("grape")
, the bash script uses the+=
operator to append a new element to the end of the existing element. At last, the scripts show the updated array with the${my_strings[@]}
and echo
command.
The output shows the updated list in which grape the new element has been appended.
If you want to add a new element in a space-separated string then you have to add space before the element that you want to insert. Here the code looks:
#!/bin/bash
my_strings="apple banana cherry date"
# Add a new element
my_strings+=" grape"
# Print the updated list
echo "Updated list: ${my_strings[@]}"
#Output: Updated list: apple banana cherry date grape
Remove a String From an Existing String List
To remove a string from a list, use the/
operator followed by the elements that you want to delete. Take a look at the code for removing a string:
#!/bin/bash
my_strings=("apple" "banana" "cherry" "date")
# Remove an element (e.g., "banana")
my_strings=("${my_strings[@]/banana}")
# Print the updated list
echo "Updated list: ${my_strings[@]}"
In themy_strings=("${my_strings[@]/banana}")
line, the/banana
replaces the element banana with space and updates the strings of arrays. Theecho
command prints the newly updated string.
The output shows the updated list in which the element banana is not present.
Check Specific Strings in a List of Strings
To check whether a specific string is in a list or not, use the parameter expansion with the conditional operator <strong>=~</strong>
. The operator matches the left-hand side string with the right-hand side string. Check the specific string using the following code:
#!/bin/bash
my_strings=("apple" "banana" "cherry" "date")
# Check if "banana" is in the list
if [[ " ${my_strings[@]} " =~ " banana " ]]; then
echo "Banana is in the list!"
else
echo "Banana is not in the list."
fi
The conditional statement[[ " ${my_strings[@]} " =~ " banana " ]]
checks whether “banana” is present in the array string. If the condition is true, then the bash script executes the if
block; if the condition returns false, then the script executes theelse
block.
From the output, you can see the searched element is in the list.
To check specific elements you can try other methods like conditional expression with case statements and grep command with parameter expansion.
Conclusion
The article shows a basic idea of a string list and how to handle the string list efficiently. Hope this will help the beginner understand how to declare, print, and iterate a list of strings and help to learn about effective manipulation.
People Also Ask
What is the list of strings in bash?
A list of strings is a collection of multiple elements that are separated by using space or declared as an array. Here is an example of a list of strings:
mystring="element1 element2 element3"
How to create a string list in bash?
To create a string list, you can use space or special characters between elements or an array. Use the code stringlist=”str1 str2 str3″ to create a space-separate string list. To create an array list use the code stringlist=("str1" "str2" "str")
.
How to get elements from the list in bash?
To get elements from the list in bash use the following code:
#!/bin/bash
List="Its abcd 1234 something else"
set -- $List
echo $2
#output 1234
The set command sets all the words in the string into the positional parameters. With the echo command, the script prints the 2nd element of the string.
To get elements from a list you can use array index. Here it is:
List="Its abcd 1234 something else"
arr=($List)
echo ${arr[1]}
#output 1234
The list stores the items of string in the List variable. Thearr=($List)
line creates an array into a variable named arr which stores the value of the list. And with${arr[1]}
, the script prints the second element of the string.
How to iterate over a list using a while loop?
To iterate over a list using a while loop use the following code:
#!/bin/bash
# Declare an array of strings
my_strings=("apple" "banana" "cherry" "date")
# Get the length of the array
length=${#my_strings[@]}
# Initialize a loop counter
counter=0
# Iterate over the list using a while loop
while [ $counter -lt $length ]; do
current_element="${my_strings[$counter]}"
echo "Processing: $current_element
((counter++))
done
The script iterates over each string element of the array using a while loop. In each iteration, it prints each of the elements. The loop ends when the script will process all the elements.
How to expand a list in bash?
To expand a list in bash, use the following code:
echo {Orange,Apple,Pineapple,Watermelon}" is a fruit."
#output Orange is a fruit. Apple is a fruit. Pineapple is a fruit. Watermelon is a fruit.
Inside curly braces, the script stores multiple elements. The brace expands the value of the elements and with theecho
command, the bash script prints the new string one after another as you can see in the output.
How to split a string in an array?
To split a string in an array use the following code below:
#!/bin/bash
# Input String
str="Split a string into array"
#Split the string by space
readarray -d " " -t array <<< "$str"
#print the array
i=0
for element in "${array[@]}"; do
echo "Array[$i]: $element"
i=$((i+1))
done
The str variable stores the string value which has space between the elements. The readarray -d " " -t array <<< "$str"
line mainly splits the string based on space. Inside the for loop, the loop variable element prints each element using the echo command.
Related Articles
- String Interpolation in Bash [4 Methods]
- How to Escape Special Characters in Bash String? [5 methods]
<< Go Back to Bash String Basics | Bash String | Bash Scripting Tutorial