FUNDAMENTALS A Complete Guide for Beginners
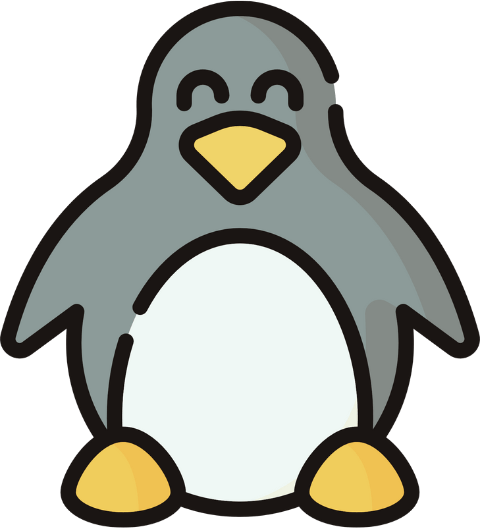
In Bash scripting, string interpolation is an important operation for dynamic string generation, command output insertion, script automation, formatting flexible output, and user interaction. So, it is essential to understand bash string interpolation and its methods how to do it. Let’s see bash string interpolation in detail.
What is String Interpolation?
String interpolation refers to inserting variables, commands, and expressions into the string. If you want to replace the value of a variable in a string, you can do this simply with string interpolation. To insert variable value to interpolate a string Bash double quotes are used. Also, remember not to use single quotes as they can’t interpolate or insert a variable value into a string.
Single quotes only contain the literal value of the characters. If you try to interpolate a string with a single quote like below it won’t show the expected result. Take a look at the code for a clearer understanding:
#!/bin/bash
name=oishi
echo 'My name is $name'
The excepted output of this script is “My name is oishi”.
But the actual output will be My name is $name. As you can see, single quotes can not interpolate strings.
Moreover, without using any quotes, you can also interpolate strings. But in this process, it can show an error when there is space between the characters in the string. So it is wise to always use the double quotes to interpolate strings in bash.
4 Methods of String Interpolation in Bash
String interpolation is the basic operation in Bash scripting. You can easily interpolate strings by following some simple ways.
Here Four simplest methods have been shown to interpolate the string which includes variable expansion, command substitution, arithmetic expansion, and curly bracket. Let’s take a look at the methods of string interpolation:
1. Using Variable Expansion
Variable expansion in string refers to the process of replacing the variables with their values. The basic syntax of the variable expansion is the $ symbol followed by the variable name. In the double quotes, the variables are interpolated by replacing the variable name with its value. For example, if you declare a variable a=20 and use $a
in the double quotes then it will show the output which is 20.
To interpolate the value of a variable into a string, using variable expansion look at the following code:
#!/bin/bash
#Declaring the variables
name = "Jacob"
age = 55
string = "My name is $name. I am $age years old."
echo "$string"
The first two lines declare two variables named name and age assigning the value Jacob and 55 accordingly. The string variable contains the literal part which is ‘My name is’ and two interpolated variables which are$name
and$age
. In the double quotes, when the shell finds the $
sign as a prefix of a variable then it inserts the value of the variable. Finally, the echo command prints the standard output.
From the output, you can see the bash script has interpolated the variables in the string.
2. Using Command Substitution
Command substitution allows you to capture the output of a command and use it as part of another command or an assignment to a variable. The$()
syntax denotes the command substitution. For string interpolation, you can store the value of the command in a variable and then use the variable with the prefix $ in the double-quoted string. It will show the result of the command inside the string.
To interpolate string using command substitution, follow the code shown below:
#!/bin/bash
current_directory=$PWD
current_date=$(date +%Y-%m-%d)
echo "My current directory is $current_directory.Today is $current_date."
Thecurrent_directory=$PWD
line stores the current directory in the variable usingPWD
. The current_date=$(date +%Y-%m-%d)
stores the output of the date command with formatting to obtain a specific format YYYY-MM-DD. The “My current directory is $current_directory. Today is $current_date.” line embeds the value of the variable current_directory and current_date.
The output shows successful string interpolation using command substitution.
3. Using Arithmetic Expansion
Arithmetic expansion refers to the process of performing the arithmetic operation in shell scripting. The basic syntax of the arithmetic expansion is$(())
and inside the double bracket, the arithmetic expression is inserted. If you store the output of the expression and insert the variable in the double-quotes it will interpolate the string.
To interpolate the value of the arithmetic expression into a string, use the following code:
#!/bin/bash
# Variables
num1=10
num2=5
# Arithmetic expansion within string interpolation
result=$((num1 + num2))
echo "The sum of $num1 and $num2 is $result."
Theresult=$((num1 + num2))
line stores the value of the arithmetic expression in the variable named result. The line following theecho
command incorporates string interpolation by interesting the value of the variables $num1
, $num2
, and $result.
The output contains the result of the arithmetic expression in string interpolation.
4. Using Curly Brackets
The curly bracket is generally used for parameter expansion and variable substitution. The basic syntax of parameter expansion is ${variable} which you can also use for string interpolation. In this string interpolation process, the script inserts the variable’s value into the string. Curly brackets provide more flexibility in handling complex string interpolation otherwise simple interpolation does not need curly brackets.
To interpolate string, you can use curly brackets by following the code:
#!/bin/bash
# Prompt user for information
read -p "Enter your first name: " first_name
read -p "Enter your last name: " last_name
read -p "Enter your age: " age
# Construct a message using string interpolation
greeting="Hello, ${first_name} ${last_name}!"
age_message="You are ${age} years old."
# Display the constructed message
echo "$greeting $age_message"
The read command with the -p
option prompts the user to enter the input of the variables. The greeting="Hello, ${first_name} ${last_name}!"
line creates a new string with the value of the first_name and last-name variable. The age_message="You are ${age} years old."
line also creates another string that contains the user’s age. At last the echo "$greeting $age_message"
concatenates the two strings and prints the output.
From the output, you can see the value taken from the user is inserted into the string.
4 Practical Examples of Bash String Interpolation
String interpolation is used for several practical applications like concatenating strings, checking undefined variables, handling special characters, printing characters without space after variables, debugging and logging, and configuring files. Let’s see some of the practical examples of interpolation below:
1. Concatenate Strings Using String Interpolation
String concatenation refers to joining a new string into an existing string. It mainly appends the string at the right of the existing one.
You can use both variable expansion and curly brackets for string interpolation to concatenate strings. Here is how:
#!/bin/bash
string1="The first line of the string."
string2="The second line of the string."
string="$string1 $string2"
echo $string
Thestring="$string1 $string2"
line inserts the value of string1
and string2
and combines it one after another. Theecho
command prints the output of the string variable.
The output shows that string1 is successfully concatenated with string2.
To concatenate using a curly bracket use the following code: concatenated_string="${string1}${string2}"
2. Check for Undefined Variables
If you check the undefined variables then you will be able to set the undefined variable and can interpolate the value of the variable into a string effectively. Here is how:
#!/bin/bash
# Check if the variable is undefined
if [ -z ${user_name+x} ]; then
user_name="Guest"
fi
echo "Welcome, $user_name! How can I assist you today?"
In the if statement the[]
operator (equivalent to the test command) employs a conditional statement. The-z
option checks for an empty variable whose length is 0. The${user_name+x}
syntax checks whether a variable is set or not. If the variable name is undefined then the user_name="Guest"
line sets the default value to the guest. In the last, theecho
command prints the string incorporating the value of user_name.
As I have not defined the user_name so, bash defines the user_name as a guest.
3. Print a Non-Space Character
To print a nonspace character after interpolation use the curly brackets or parameter expansion. Without these brackets, there will be an error. For example, if you interpolate the string using the syntax “I eat $foods” where the food=apple, it won’t show the expected result.
To print the non-space characters in string interpolation using the curly braces, please go through the below script:
#!/bin/bash
food="apple"
echo "I eat ${food}s"
The food="apple"
declares a variable named food and assigns the string “apple” to it. The ${food}s
indicates the parameter expansion with the food variable with a character “s” for string interpolation.
From the output you can see the string contains the character “s” immediately after the apple variable.
4. Handling Special Characters
To print a special character in the string, use string interpolation. Check the following code:
#!/bin/bash
price=100
echo "The cost is \$${price}."
Theprice=100
declares a variable with a value of 100. The\
prevents the variable expansion of the first$
symbol and bash treats the first $ as a literal character. And the${price}
shows the variable value in the string.
From the image, you can see that the output contains the special character as expected.
Conclusion
The article shows a few easy methods and practical examples of string interpolation. Among all the methods, the use of the curly bracket for string interpolation is a clearer and more versatile method. Hope this article is helpful for users who looking for a complete guide in string interpolation.
People Also Ask
What does ${} mean Bash?
The ${}
syntax is used for parameter expansion in bash. It is the process of manipulating the values of a variable. The basic syntax is ${variable}
and you can use this for string interpolation and concatenation.
Can I interpolate string without using quotes?
Yes, you can interpolate string without using quotes like below:
#!/bin/bash
name=John
age=25
sentence1=$name$age
#output
#John25
But if the variable sentence1 contains space it will show unexpected results. To avoid errors when there is space between characters use the following code:
sentence2=My\ name\ is\ $name\ and\ I\ am\ $age\ years\ old.
echo $sentence
#output
#My name is John and I am 25 years old.
By following this method you can interpolate strings when you are not using quotes.
Does Bash script string interpolation leave curly braces intact?
Depends on how you use them. It does not leave curly braces intact if you use the curly braces ({}) in the following way:
#!/bin/bash
version=2989
string="The version of the site is version=${version} & therest."
echo "$string"
#Output
#The version of the site is version=2989 & therest.
But if you use the braces in the following way:
#!/bin/bash
version=2989
string="The version of the site is version={$version} & therest"
echo "$string"
Then the output will be like this: The version of the site is version={2989} & therest
How can I insert a value of an array element in a string?
To insert a value of an array element in a string by using string interpolation follow the code:
#!/bin/bash
#Correct way
colors=("red" "green" "blue")
echo "Selected color: ${colors[1]}"
#output
# Selected color:green
When you use index [1]
in an array, it will show the second element of the array. Here the second element is green.
But if you don’t use the curly braces then you may face unexpected results as I have shown below:
echo "Selected color: $colors[1]"
#output
#red[1]
So try to use the curly braces{} while interpolating elements of an array.
How to interpolate a string that was sent as an argument?
To interpolate a string that was sent as an argument, use the following code:
#!/bin/bash
# Check if three arguments are provided
if [ "$#" -ne 3 ]; then
echo "Usage: $0 <arg1> <arg2> <message>"
exit 1
fi
a=$1
b=$2
message=$3
echo "$message" | awk '{gsub(/\$\{1\}/, a); gsub(/\$\{2\}/, b)} 1' a="$a" b="$b"
The awk command executes the following code and replaces any occurrence of {1} and {2} in the input with the value of the variables a and b.
Now in the terminal copy the following code:
bash question1.sh "apples" "oranges" 'There were ${1} and ${2} in the basket.'
And you will get the following expected interpolation:
#output
#There were apples and oranges in the basket.
How to preserve quotes in bash string interpolation?
To preserve quotes you can use backslash (\) while doing string interpolation. For example, if you want to expect an output like this: My name is “$name” and I am “$age” years old. Then use the following code:
#!/bin/bash
name="John"
age=25
sentence="My name is \"$name\" and I am \"$age\" years old."
echo "$sentence"
Here by using the \ character, you can preserve the double quotes (“).
Related Articles
- List of Strings in Bash [Declare, Iterate & Manipulate]
- How to Escape Special Characters in Bash String? [5 methods]
<< Go Back to Bash String Basics | Bash String | Bash Scripting Tutorial