FUNDAMENTALS A Complete Guide for Beginners
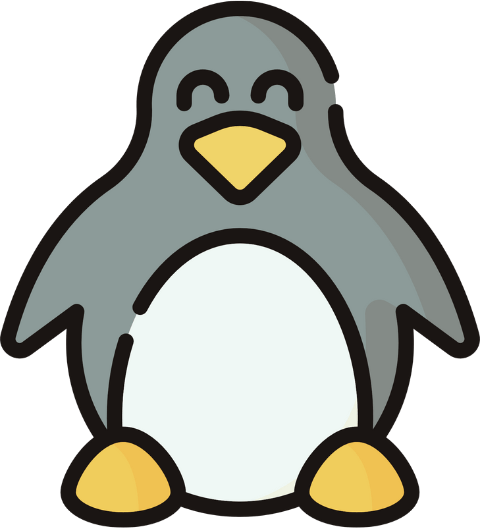
When working in Bash scripting, there exist some instances where you need to expand the traditional strings over multiple lines. Here comes the solution: multiline string which is the container of texts of multiple lines. This article will walk you through the fundamentals and advanced aspects of working with bash multiline strings, providing insights into their application and utilization in scripting.
What is a Bash Multiline String?
A multiline string is a sequence of characters that spans multiple lines. In contrast with a single-line string, which contains text within a single line, a multiline string allows text in multiple lines. In this segment, I’ll delve into the process of creating, storing, and reading multiline strings.
A. Create Multiline Strings Using Heredoc
A Heredoc, short for “here document”, is a type of redirection to define multiline strings in bash. It allows you to include a text block without escaping special characters or using concatenation. It employs a type of I/O redirection to provide a series of commands or text to an interactive program or command like cat, ftp. A delimiter is specified as a marker to indicate the beginning and end of the multiline string.
The syntax for Heredoc in Bash is:
COMMAND << DELIMITER
….
Heredoc Block
….
DELIMITER
Follow the script below to create a multiline string using a heredoc:
#!/bin/bash
cat << EOM
This is a multiline string
using the Heredoc syntax.
It's a convenient way to create
strings that span multiple lines.
EOM
<<
symbol functions as the redirection operator, and EOM serves as the delimiter. The << EOM
indicates the start of the Heredoc. The content between << EOM
and EOM is considered part of the Heredoc and it’ll be treated as a single multiline string.The output shows the content of the multiline string.
cat << 'EOM'
$var
$(date)
EOM
In this instance, the expressions $var
and $(date)
remain unexpanded, treated as literal strings.
You can also define a multiline string in one line using quotes and line continuation as below:
#!/bin/bash
#define a multiline string
multiline_string="This is a multiline string.
It is written in a single line,
but appears as multiple lines when printed."
#echo the multiline string
echo "$multiline_string"
In this method, double quotes (“”
) are used, and line breaks are introduced directly within the quotes. The string is defined in a single line but the line breaks are preserved when printed.
The output prints the multiline string defined in a single line within the quotes.
B. Store Multiline String
In Bash, multiline strings can be stored in files or variables easily.
To store multiline strings in a file, follow the below script:
#!/bin/bash
#Define a file
file=store.txt
# Use a Here Document to store a multiline string to the file
cat > $file << EOF
LinuxSimply
Bash
String
Multiline String
EOF
# Display the contents of the created file
cat store.txt
The output shows the content of the file after storing the multiline string.
To store a multiline string in a variable, follow the script below:
#!/bin/bash
#store a multiline string in a variable
multiline_string=$(cat << EOM
This is a multiline string
using Heredoc.
This string is stored
In a variable.
EOM
)
#display the content of the variable
echo "$multiline_string"
The output displays the content of the multilne_string variable.
C. Reading Multiline String from Files
You can read multiline strings from files in Bash in various methods. Follow the script below to read from a file using the cat command and a redirection <<
:
Here’s the file’s content to read:
#Contents of the file
Text of the file
This is a multiline string.
It spans multiple lines.
You can include variables, such as \$HOME.
Follow the below script to read multiline strings from files:
#!/bin/bash
multiline_string=$(cat filename.txt)
echo "$multiline_string"
The output shows the contents of the multiline string read from the file.
Manipulating Multiline String
Manipulating multiline strings in Bash is an essential skill in Bash which involves techniques such as incorporating variables, performing command substitution, excluding specific lines during string printing, inserting line breaks as specific positions, removing empty lines, clearing specific lines, and more. This segment will delve into a detailed exploration of these techniques.
1. Bash Echo Multiple Lines
When working in Bash scripting, echoing multiple lines is a common task, whether for displaying information or manipulating text. You can employ various approaches to echo multiple lines of multiline strings such as using the echo command with newlines character, using printf command, or using Heredoc.
For example, echo multiple lines of a multiline string using Heredoc as the following script:
#!/bin/bash
cat <<EOL
String
Multiline string
Bash
EOL
<< EOL
and EOL
delimiters.The output shows the echoed multiple lines.
2. Variables Within Multiline String in Bash
You can incorporate variables within a multiline string as shown in the following script:
#!/bin/bash
#define the variable
city="Paris"
cat << EOM
Greetings from $city,
Hope you enjoy this Bash example!
EOM
The displayed output exhibits a multiline string that incorporates a variable.
3. Command Substitution Within Multiline String
While working in Bash scripts, sometimes you might need to substitute commands within the multiline string. Command substitution allows you to embed the result of the command directly into the string. Follow the script below to substitute command within a multiline string:
#!/bin/bash
# Using command substitution to get the current date
current_date=$(date)
# Creating a multiline string with command substitution
multiline_string="This is a multiline string.
It includes the current date: $current_date."
echo "$multiline_string"
date
is then incorporated into a multiline string variable $multiline_string. The echo command displays the resulting multiline string, which combines static text with the dynamically retrieved current date.The output displays the multiline string which contains the result of the date command.
4. Skip the First Line of a Multiline String
In Bash, if you have a multiline string and want to skip the first line, you can use the “awk” command or parameter expansion.
#!/bin/bash
# Multiline string with several lines
multiline_string="First line
Second line
Third line
Fourth line"
# Skip the first line using awk
skipped_string=$(echo "$multiline_string" | awk 'NR > 1')
echo "$skipped_string"
awk 'NR > 1'
specifies a condition where the action (in this case, printing the line) is performed only for lines with a record number greater than 1
.The resulting output displays the content of the multiline string, excluding the first line.
5. Insert Line Break in Multiline String
To insert a line break in a multiline string, you have to specify the position and insert the line break there. You can check the following script to insert a line break in a multiline string:
#!/bin/bash
# Multiline text
multiline_string="This is a multiline string. Multiline This is the first line. Multiline This is the second line. Multiline This is the third line."
# Use a for loop to iterate through words
for word in $multiline_string; do
# Check if the word is "Multiline"
if [ "$word" == "Multiline" ]; then
# Print a newline when "Multiline" is encountered
echo
else
# Otherwise, print the word with a space
echo -n "$word "
fi
done
# Print a final newline for better formatting
echo
The output shows a multiline string after the replacement of the “multiline” word with the line break.
6. Remove Empty Lines from a Multiline String
To remove empty lines from a multiline string, use the awk command with NF. NF is a built-in variable that represents the number of fields (columns) in the current record (line). When used in a condition, such as awk NF, it checks if the number of fields in a line is greater than zero. Follow the below script to remove empty lines from a multiline string:
#!/bin/Bash
#define a multiline string with empty lines
multiline_string=$'This is a line.
This is another line.
And a third line.
Last Line'
#Remove the empty lines
new_string=$(echo -e "$multiline_string" | awk NF)
#print the string without an empty line
echo "$new_string"
$'...'
syntax which contains a few empty lines. Subsequently, the script uses the echo -e command and pipes the multiline string to the awk NF command. The awk NF command filters out lines without the empty lines. The output shows the multiline string after removing the empty lines.
7. Remove the Newline from a Multiline String
If you want to remove newline characters from a multiline string in Bash, you can use the tr command. Follow the script below to remove newlines from a multiline string:
#!/bin/bash
#define the multiline string
multiline_string=$'This is a line.
This is another line.\nAnd a third line.
Last Line'
#remove the newline
cleaned_string=$(echo -e "$multiline_string" | tr -d '\n')
#preint the string
echo "$cleaned_string"
tr -d '\n'
command to delete newline characters. The result is stored in the variable cleaned_string.The output displays the multiline string after removing all the newlines.
8. Replace a Multiline String
To replace a multiline string in Bash, you have to perform a substitution operation using the sed command. Check out the script below to replace a multiline string in Bash:
#!/bin/bash
# Original multiline string
multiline_string="This is the first line.
This is the second line.
This is the third line."
# String to replace and its replacement
string_to_replace="second line"
replacement_string="modified content"
# Perform the replacement using sed
modified_string=$(echo "$multiline_string" | sed "s/$string_to_replace/$replacement_string/g"
# Print the original and modified strings
echo "Original Multiline String:"
echo "$multiline_string"
echo
echo "Modified Multiline String:"
echo "$modified_string"
s
denotes substitution, and g
ensures that all occurrences are replaced globally. The output shows the original multiline string and the modified multiline string after the replacement.
9. Clear Line from a Multiline String
To clear a specific line from a multiline string, you can use parameter expansion. Follow the script below to remove a line from a multiline string:
#!/bin/Bash
multiline_string="First line.
Second line.
Clear this line.
Third line.
Last line."
# Specify the content to clear
Line_to_clear="Clear this line."
# Use parameter expansion to remove the line
clear_string=${multiline_string//$Line_to_clear$'\n'}
echo -e "$clear_string"
\n
) following it from the multiline_string. The output displays the multiline string after clearing a line from the string.
Conclusion
To sum up, this article provides a comprehensive guide on multiline strings in Bash. It covers fundamental concepts, and various techniques for manipulating multiline strings, including echoing multiline strings, incorporating variables, command substitution, and more. Hope this article enhances your understanding of multiline strings in Bash.
People Also Ask
What are the applications of a multiline string in Bash?
The applications of multiline strings in Bash are multiple such as handling large blocks of text. Here are common use cases of multiline strings in Bash:
- Configuration Files: Multiline strings provide a convenient way to store and manage configuration data in Bash scripts.
- Error Messages: When scripting error messages or log entries, multiline strings provide a structured way to present detailed information.
- Documentation: Multiline strings help you to document the purpose, usage, or specific instructions for the script.
Are there any limitations to using multiline strings in Bash?
Yes, there are certain limitations to be cautious of when using multiline strings in Bash. Special characters, variable expansions, and white spaces within the multiline string may behave differently compared to single-line strings. By being aware of these limitations, you can effectively navigate the use of multiline strings in Bash.
How to concatenate multiline strings in Bash?
To concatenate multiline strings in Bash, you can adopt various approaches. One common approach is to use the concatenation operator +=
. For example, follow the below script:
#!/bin/bash
# Define multiline strings
multiline_string1="This is the first line.
This is the second line."
multiline_string2="And this is the third line.
Final line."
# Concatenate multiline strings
concatenated_string="$multiline_string1"
concatenated_string+="$multiline_string2"
# Print the concatenated string
echo "$concatenated_string"
##Output
This is the first line.
This is the second line.
And this is the third line.
Final line.
This script concatenates two multiline strings using the += operator. The resulting concatenated string contains the content of both multiline strings.
How to print multiple lines using the “printf” command?
To print multiple lines in Bash, you can use a combination of newline characters and the printf command. Follow the example below to print multiple lines:
printf "This is the first line.\nThis is the second line.\nAnd this is the third line.\n"
In this example, \n
represents a newline character, and printf is used to print each line.
How to Write a Multiline Comment in Bash?
To write a multiline comment in Bash, use a combination of colon :
and here document <<
. You can establish a multiline comment by using here document which involves using the syntax << 'END_COMMENT'
to begin the comment block, followed by your comment lines, and concluding with END_COMMENT
to mark the end of the comment. Follow the script below to write multiline comments in Bash:
: <<'END_COMMENT'
This is a multiline comment in Bash.
You can write as many lines as you want here.
END_COMMENT
Related Articles
- 4 Methods to Skip First Line of Bash Multiline String
- How to Echo Newline in Bash? [Complete Guide]
- How to Remove Empty Lines in Bash? [5 Methods]
- How to Remove Newline from String in Bash? [7 Methods]
<< Go Back to Bash String | Bash Scripting Tutorial