FUNDAMENTALS A Complete Guide for Beginners
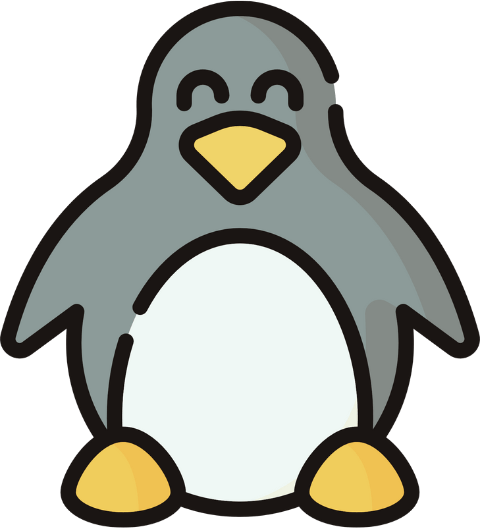
In Bash, newline characters (\n) are often inserted in strings from various sources, such as file inputs or command outputs. This character represents the end of a line and can disrupt string processing, especially when you need to treat the text as a single, continuous entity. You can adopt any one of the following 6 methods to remove a newline from a string in Bash:
- Using parameter expansion:
${string//$'\n'/}
- Using “tr” Command:
tr -d '\n'
- Using the “sed” command:
sed ':a;N;$!ba;s/\n//g'
- Using the “awk” command:
awk '{ printf "%s", $0 }' <<< "$string"
- Using “perl”:
perl -pe 's/\n//g' <<< "$string"
- Using “paste” command:
paste -sd ""
- Using “extglob” option:
${string//+($'\n')/}
Let’s dive into the 7 methods to remove newline (‘\n’) from a string:
1. Using Parameter Expansion
Parameter expansion is a powerful tool in bash that allows you to perform various operations such as substitution, trimming, and pattern matching on strings. To remove newline characters from a string using parameter expansion in bash, you can use the ${parameter//pattern/replacement}
syntax.
Follow the script below to remove newline (‘\n’) from a multiline string:
#!/bin/bash
# Original string with newline characters
string="First
Second
Third"
#print the multiline string
echo "The multiline string:"
echo "$string"
echo
# Remove newline characters
string="${string//$'\n'/}"
# Print the updated string
echo "After removing the newlines"
echo "$string"
"${string//$'\n'/}"
. The expression replaces all occurrences of the pattern $'\n'
(newline character) with nothing in the value of the variable string
. The output shows that all the newlines are removed from the input string.
2. Using “tr” Command
The tr command in Bash is a powerful utility used for translating or deleting characters. It is well-suited for various text processing tasks in shell scripts, including data cleaning, formatting, and transformation. Here’s how you can remove newline characters from a string using the tr
command in Bash:
#!/bin/bash
# Original string with newline characters
string="This is a string
with newline characters
included."
#print the multiline string
echo "The multiline string:"
echo "$string"
echo
# Remove newline characters using tr command
string=$(echo -n "$string" | tr -d '\n')
# Print the updated string
echo "After removing the newlines"
echo "$string"
tr -d
deletes newline characters from the input stream generated by echo -n "$string"
. The -n
option for echo ensures that no trailing newline is added to the output. The output shows that all the newlines are removed from the input string using the tr command.
3. Using the “sed” Command
In Bash, the sed command stands for “stream editor”, which allows text transformation on an input stream (a file or data supplied via a pipeline) and generates an output stream to STDOUT (standard output) or a file.
To remove newlines from a string using the sed command, you can use the following script:
#!/bin/bash
# Original string with newline characters
string="This is a string
with newline characters
included."
#print the multiline string
echo "The multiline string:"
echo "$string"
echo
# Remove newline characters using the sed command
string=$(echo "$string" | sed ':a;N;$!ba;s/\n//g')
# Print the updated string
echo "After removing the newlines"
echo "$string"
':a;N;$!ba;s/\n//g'
. In the expression, the label :a
marks the beginning of a loop, while N
appends the next line of input to the current pattern space. The conditional $!ba
checks if the current line is not the last one; if not, it branches back to label a
, effectively creating a loop that reads in all lines. Lastly, s/\n//g
substitutes all occurrences of newline characters with nothing, effectively joining all lines into a single line.The output displays the string after removing the newline characters using the sed command.
4. Using the “awk” Command
In Bash, awk is a scripting command that handles text processing, and data manipulation. You can use the awk command to remove the newlines from a string.
To remove newline characters from a string using the awk command, follow the script below:
#!/bin/bash
# Define a string
string="This is a string
with newline characters."
# Print the multiline string
echo "The multiline string:"
echo "$string"
echo
# Remove newlines using awk
string=$(awk '{ printf "%s", $0 }' <<< "$string")
# Print the updated string
echo "After removing the newlines:"
echo "$string"
{ printf "%s", $0 }
prints each line of input without appending newline characters, effectively joining all lines into a single line. %s
is used to print a string without any additional formatting, and $0
refers to the entire line. So, when printf "%s", $0
is executed, it prints the content of the current line ($0
) without any additional formatting, effectively printing the line as it is.The output shows the result of newline characters removal from the string using the awk command.
Using Output Record Separator (ORS) with “awk”
Alternatively, You can make the “awk” command remove the newlines characters from the string with the ORS (Output Record Separator) variable. Here’s how to do it:
#!/bin/bash
# Define the string
string="This is a string
with
Newlines."
# Print the multiline string
echo "The multiline string:"
echo "$string"
echo
# Use awk to remove newline characters
new_string=$(awk 1 ORS='' <<< "$string")
# Print the modified string
echo "After removing the newlines:"
echo "$new_string"
awk 1 ORS=''
, where 1 serves as a pattern that always evaluates to true, ensuring that awk executes the default action for every input record, which is to print it. The ORS=' '
option sets the Output Record Separator to an empty string, effectively instructing awk to output all lines without any newline characters. The outpost presents the result after the removal of the newline characters from the string using the awk command and ORS variable.
5. Using “perl” Command
Perl offers powerful text processing capabilities, making it well-suited for tasks like removing newline characters from strings. To remove newline characters from a string using Perl within a Bash script, use the following code:
#!/bin/bash
# Original string with newline characters
string="This is a string
with newline characters
included."
# Print the original string
echo "Original string:"
echo "$string"
# Remove newline characters using Perl
result=$(perl -pe 's/\n//g' <<< "$string")
# Print the updated string
echo -e "\nString after removing newline characters:"
echo "$result"
perl
command with the -pe
options to process the string. The -e
option is used to specify the Perl one-liner script 's/\n//g'
.The code s/\n//g
matches newline characters (\n) and replaces them with nothing. Here, the s///
operator is the substitution operator in Perl, used for search and replace operations. This g flag
ensures that all occurrences of newline characters within the string are replaced, not just the first one.The output displays the string before and after removing the newline characters from the string using Perl.
6. Using “paste” Command
The paste command is a versatile utility available in Linux whose primary function is to merge lines of files, either side by side or sequentially. It’s often used to concatenate lines from one or more files or streams, with a specified delimiter between them. In Bash scripting, the paste command can be utilized to remove newline characters from a string.
To remove newline characters from the string using the paste command, follow the script below:
#!/bin/bash
# Define the string
string="This is a string
with
newlines."
# Print the original string
echo "Original string:"
echo "$string"
echo
# Use paste to remove newline characters
new_string=$(paste -sd "" <<< "$string")
# Print the modified string
echo "After removing the newlines:"
echo "$new_string"
paste
command with -sd
option to remove newline characters from the string variable. Here, -s
option tells the paste to concatenate all input lines into a single line. Whereas, -d ""
specifies an empty string as the delimiter, effectively removing newline characters.The output represents the string before and after removing the newline characters (\n) from the string using the paste command.
7. Using “extglob” Option
The extglob option in Bash enables extended pattern-matching features, expanding pattern-matching capabilities beyond the standard wildcard characters (*, ?, [ ]). It allows for more complex pattern-matching expressions, providing greater flexibility in string manipulation within Bash scripts. To remove newline characters from a string using the “extglob” option, follow the script below:
#!/bin/bash
# Enable extglob option
shopt -s extglob
# Original string with newline characters
string="This is a string
with newline characters
included."
#print the multiline string
echo "The multiline string:"
echo "$string"
echo
# Remove newline characters using parameter expansion with extglob
string="${string//+($'\n')/}"
# Print the updated string
echo "After removing the newlines"
echo "$string"
shopt -s extglob
enables the extglob option, which enables the extended pattern matching features. This script removes the newlines from the string variable, through the ${string//pattern/replacement}
syntax, where the pattern matches one or more newline characters represented by +($'\n')
and the replacement is empty. The output displays that all the newlines are removed from the input string using the extglob option.
Conclusion
To sum up, removing the newlines from a string in Bash is an essential and straightforward task for scriptwriters. This article illustrates 6 methods to remove the newline from a string using parameter expansion, “tr” command, “extglob” option, “sed” command, “awk” command, and “perl”. Hope this article clears your understanding of the concept “Bash remove newline string from string”.
People Also Ask
How to remove newline from a file in Bash?
To remove newline characters from a file in bash, you can use tr -d '\n' < [filename]
. For example, a file named input.txt whose contents are:
This is a text file
which contains newline characters.
These are to be removed.
Run the below code to remove the newline from the file:
tr -d '\n' < input.txt
Here, the tr command uses the -d
option to delete the newline characters (‘\n’) from the input file (input.txt).
How to replace newline with space from a multiline string in Bash?
You can replace newline characters with spaces from a multiline string in Bash using parameter expansion. Here’s how you can do it:
#!/bin/bash
# Multiline string
multiline_string="This is a
multiline
string."
# Replace newline characters with spaces
formatted_string="${multiline_string//$'\n'/ }"
echo "$formatted_string"
The script will output the multiline string with newline characters replaced by spaces.
How to remove only the last newline character from the multiline input?
Use Perl to remove only the last newline character from a multiline input. For example, use the following Perl one-liner:
#!/bin/bash
#define string
multiline-string="This is a
Multiline string
With newline characters."
perl -pe 'chomp if eof' <<< multiline_string
The script removes the newline character from the end of the last line of the multiline string.
How to remove the newline characters from a file in Vim?
To remove the newline characters from a file in Vim, follow the steps below:
- First, open the file using the vim filename command.
- Press Esc to ensure you’re in normal mode and then type
:
to enter the command mode. - Type this
:%s/\n/ /g
substitution command to replace newline characters (\n) with white space and press ENTER. - Finally, save the changes and exit vim by typing
:wq
and press ENTER.
Related Articles
- 4 Methods to Skip First Line of Bash Multiline String
- How to Echo Newline in Bash? [Complete Guide]
- How to Remove Empty Lines in Bash? [5 Methods]
<< Go Back to Bash Multiline String | Bash String | Bash Scripting Tutorial