FUNDAMENTALS A Complete Guide for Beginners
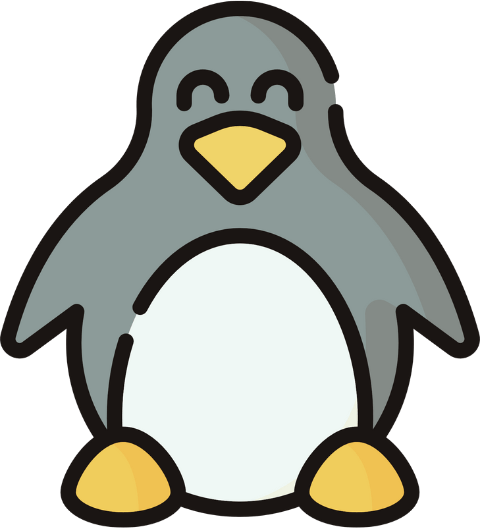
In Bash, a multiline string is an essential tool for refining the text processing workflows and enhancing script functionality. While working with multiline strings, there are scenarios where you may need to exclude the first line for various reasons, whether removing headers from data files, skipping introductory comments, or simply adjusting the output format.
To skip the first line of a multiline string, you can follow the following 4 methods:
- Using “tail” Command:
tail -n +2 <file_name>
- Using “sed” Command:
sed '1d' <file_name>
- Using “awk” Command:
awk 'NR>1' <file_name>
- Using “head” Command:
head -n +2 "$input_file"
Let’s dive into the methods to learn how to skip the first line of a multiline string.
1. Using “tail” Command
The tail command in Bash is used to display the last part of a file or input stream. and the option -n to skip the first line of a multiline string. The syntax is tail -n +2 <file_name>
tells the tail command to start displaying from the second line onwards.
To skip the first line of the multiline string using the tail command, follow the script below:
#!/bin/bash
# Define the multiline string
multiline_string='First line
Second line
Third line'
#Print the multiline
echo "The multiline string:"
echo "$multiline_string"
echo
# Skip the first line using tail
skipped_string=$(echo "$multiline_string" | tail -n +2)
# Output the result
echo "The first line skipped string:"
echo "$skipped_string"
-n
option where -n
specifies the number of lines to display and +2
means starting from the second line. The syntax skipped_string=$(echo "$multiline_string" | tail -n +2)
takes the content of multiline_string, removes the first line using tail, and assigns the result to the variable skipped_string.The output shows the multiline string before and after skipping the first line.
2. Using “sed” Command
You can use the “sed” command with the command option 1d
to skip first line in Bash of a multiline string. Follow the script below to skip the first line of the multiline string using the sed command:
#!/bin/bash
# Define the multiline string
multiline_string='First line
Second line
Third line'
#Print the multiline
echo "The multiline string:"
echo "$multiline_string"
echo
# Skip the first line using sed
skipped_string=$(echo "$multiline_string" | sed '1d')
# Output the result
echo "The first line skipped string:"
echo "$multiline_string"
echo -e "\nMultiline String after Skipping the First Line:"
echo "$skipped_string"
echo "$multiline_string" | sed '1d'
command removes the first line from the multiline string and the resulting string is stored in the skipped_string variable.The output displays the multiline string after skipping the first line using the sed command.
3. Using “awk’ Command
The awk command in bash is used to process text line by line. To skip the first line of a multiline string you can use NR which represents the record (line) number and the condition NR>1
tells awk to start the processing from the second line onwards. See the script below to skip the first line of the multiline string using the awk command in Bash:
#!/bin/bash
# Define the multiline string
multiline_string='First line
Second line
Third line'
# Skip the first line using awk
skipped_string=$(echo "$multiline_string" | awk 'NR>1')
# Output the result
echo "Original Multiline String:"
echo "$multiline_string"
echo -e "\nMultiline String after Skipping the First Line:"
echo "$skipped_string"
NR>1
ensures that awk operates only on lines numbered greater than 1
, effectively skipping the first line of the multiline string.The output presents both the multiline string and the string after skipping the first line.
4. Using the “head” Command
The head command in Bash primarily displays the first few lines of a file or input stream. However, it can be leveraged to skip lines of a multiline string from the beginning. To skip lines using the head command, you can use the -n
option followed by the number of lines you want to skip. Here’s how:
#!/bin/bash
# Define the multiline string
multiline_string='First line
Second line
Third line'
# Skip the first line using head and assign the rest to a variable
skipped_string=$(echo "$multiline_string" | head -n -1)
# Output the result
echo "Original Multiline String:"
echo "$multiline_string"
# Print the skipped string
echo "Skipped string:"
echo "$skipped_string"
head -n -1
, the -n
option is used to specify the number of lines to display from the beginning of the input. When -n
is followed by a positive number, it indicates the exact number of lines to display.The output shows the multiline string after skipping the first line using the head command.
Conclusion
To sum up, skipping the first line of a multiline string is quite straightforward to achieve following the 4 methods. You can choose any of the methods such as “awk”, “sed”, “tail”, or “head” commands to skip the lines from the beginning of the string involving different conditions. Hope this article sharpens your insight on this topic.
People Also Ask
How to skip the first line using NR and != operator?
To skip the first line using NR
and !=
operator, follow the script below:
#!/bin/bash
multiline_string='First line
Second line
Third line'
string=$(awk 'NR != 1' <<< "$multiline_string")
echo "$string"
Here, the awk reads each line of the multiline string, and if the line is not the first one (NR != 1
), it prints it to the output.
How to skip the range of lines using “sed” in Bash?
To skip a range of lines in Bash, you can use the “sed” command along with the d
parameter which specifies the range of lines to delete. Follow the script below to skip a range of lines:
#!/bin/bash
# Define the multiline string
multiline_string='First line
Second line
Third line
Fourth line
Fifth line'
# Skip a range of lines using sed
skipped_string=$(echo "$multiline_string" | sed '2,4d')
# Output the original and skipped strings
echo "Original Multiline String:"
echo "$multiline_string"
echo -e "\nMultiline String after Skipping a Range of Lines:"
echo "$skipped_string"
In the script, the sed '2,4d'
command skips lines 2 to 4 from the multiline string in the output.
How do you line break in Bash script?
In Bash scripts, you can use the newline character \n
to create line breaks. Here are a few common ways to include line breaks:
- Using echo with the
-e
option:echo -e "Line 1\nLine 2\nLine 3"
- Using printf:
printf "Line 1\nLine 2\nLine 3\n"
- Assigning a multi-line string to a variable:
multiline_string="Line 1
Line 2
Line 3"
How to skip a word in a multiline string in Bash?
To skip a word in a multiline string in Bash, you can use the “sed”, “awk”, or “grep” command. The following script replaces a specific word from the multiline string using the sed command. Check the script below:
#!/bin/bash
#Multiline string
multiline_string="This is a skipping multiline
string with several
words in it."
#specify the word to skip
word_to_skip="skipping"
# Skipping a word in the string
skipped_string=$(echo "$multiline_string" | sed "s/$word_to_skip//g")
echo "$skipped_string"
Related Articles
- How to Echo Newline in Bash? [Complete Guide]
- How to Remove Empty Lines in Bash? [5 Methods]
- How to Remove Newline from String in Bash? [7 Methods]
<< Go Back to Bash Multiline String | Bash String | Bash Scripting Tutorial