FUNDAMENTALS A Complete Guide for Beginners
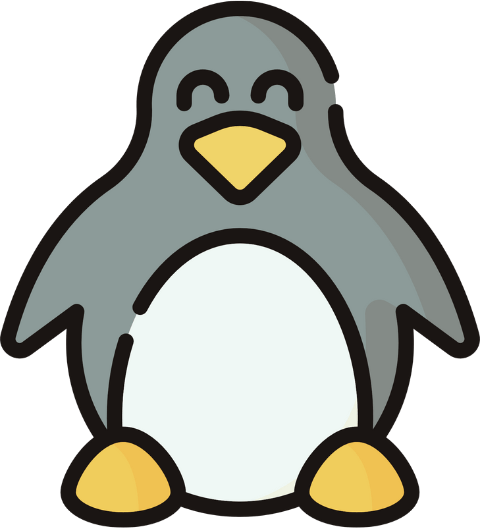
In bash scripting, removing characters is important for deleting leading spaces, tabs, and unwanted characters from an input text. Removing the first character or a specific character from a string you can extract desired output and also do input processing.
To remove the first character from a Bash string, check the following methods:
- Using parameter expansion:
${parameter:offset}
- Using the cut command:
cut [options] [action] filename
- Using the sed command:
sed 's/^.//' filename
- Using the grep command:
grep [option] 'action'
- Using the awk command:
echo "input_str" | awk 'pattern { action }'
- Using the tr command:
tr -d [character]
- Using Perl command:
echo "$input_string" | perl [Option] 'action'
Dive into the article to learn these methods of how to remove the first character from a Bash string in detail.
1. Using Parameter Expansion
Using the parameter expansion you can simply remove the first character of a bash string without any external command. Parameter expansion refers to retrieving and manipulating the value stored in the parameter or variable. Using the substring expansion ${parameter:offset}
, you can easily extract the substring by setting the starting at a specified offset that removes the first character.
To remove the first character from the input bash string, you can use parameter expansion. Check the following script below:
#!/bin/bash
# Define the input string
input_string="Remove the first character. "
# Remove the first character using parameter expansion
trimmed_string="${input_string:1}"
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
At first, the script declares the string in a variable namedinput_string
. Then the${input_string:1}
syntax will delete the first character. Mainly here the script extracts a substring that starts from the 2nd character as it sets the offsets at1
. After that, the script stores the modified string into a variable named trimmed-string
. At last, the echo command prints the output in the terminal.
The output shows the script has removed the first character R from the input string.
2. Using “cut” Command
To remove the first character of the input string, you can use thecut
command. The cut command simply extracts the substring and removes the specific portion of the input string. Take a look at the script below:
#!/bin/bash
# Define the input string
input_string="Example for removing the first character"
# Use cut to remove the first character
trimmed_string=$(echo "$input_string" | cut -c 2-)
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
With the$(echo "$input_string" | cut -c 2-)
, the pipe (|) operator redirects the output of theecho
command to the cut command. Thecut
command removes the first character and extracts a substring starting from the 2nd character to the end.
The image shows the script has removed the first character E from the input string.
To remove the leading space of an input string you can also use thecut
command to remove the space.
The image shows the cut command removes the leading space from the input string.
3. Using the “sed” Command
The sed command is a simple yet very useful command for stream editors in bash scripting. You can use it to remove characters, replace strings, match patterns, and manipulate files and texts.
To remove the first character from an input string, take a look at the following script:
#!/bin/bash
new_string="Removing the first character."
echo "Original String: $new_string"
echo "$new_string" | sed 's/^.//'
In the"$new_string" | sed 's/^.//'
syntax, thesed
command removes the first character of the echo
command’s output. Here, the^.
finds the first character and then removes it using //
.
From the image, you can see the script gave a result without the first letter “R” of the input string.
4. Using the “grep” Command
The grep command is used to search and match the pattern of characters of a string and file. But with the -o option with the grep command, you can simply remove characters from a bash string. To remove the first characters from a bash string use the following script below:
#!/bin/bash
# Define the input string
input_string='323 456 789'
# Use grep to match and extract the first character
trimmed_string=$(echo "$input_string" | grep -Po '^.{1}\K.*')
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
At first, the script declares the string in a variable namedinput_string
. Then with $(echo "$input_string" | grep -Po '^.{1}\K.*')
, it removes the first character of the input string. Here the pipe(|) operator takes the output from theecho
command to thegrep
command. In the grep command, it matches the first character using^.{1}
and after that, it resets the match using \K
and later prints the rest of the characters using.*
and the-o
option.
The image shows the script has removed the first character which is 3 from the input string.
5. Using the “awk” Command
To remove the first character from the input string, you can use the awk command. The awk is a versatile command tool used for text processing, handling multi-byte characters, and especially for pattern matching and manipulation. The awk command removes characters by using the substr()
. Check the following script below:
#!/bin/bash
# Define the input string
input_string="! The new one."
# Use awk to remove the first character
trimmed_string=$(echo "$input_string" | awk '{print substr($0, 2)}')
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
With $(echo "$input_string" | awk '{print substr($0, 2)}')
, the script removes the first characters from the input string and stores the output in a variable namedtrimmed_string
. Here, theawk
command takes the output of theecho
command as input through the pipe operator. Theprint substr($0, 2)}
extracts from the 2nd characters to the end from the input string by removing the first character.
The output shows the awk command has removed the first character which is!
from the input string.
6. Using “tr” Command
To remove the first character of a string you can use the tr command. In bash scripting, the tr command is used to translate and delete characters. With the tr -d
command, you can easily remove specific characters from a string by specifying which character you want to delete. Check the following script:
#!/bin/bash
# Define the input string
input_string="Here it is"
# Use tr to remove only the first character
trimmed_string=$(echo "$input_string" | tr -d 'H')
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
With the $(echo "$input_string" | tr -d 'H')
, thetr
command takes the output of the echo
command using the pipe operator and deletes the characterH
from the input string.
The image shows the tr command has deleted the first character of the input string.
Note: In this method, if the input_string
contains multiple occurrences of the first character then the tr command will delete all the occurrences. To specify the first character, use the following code:
trimmed_string=$(echo "$input_string" | tr -d "${input_string:0:1}")
It will remove only the first character if there are multiple occurrences of the first character in the input string.
7. Using “perl” Command
Using the regular expression, the perl command removes the first character from the input string. To remove the first character from a bash string, take a look at the following code:
#!/bin/bash
# Define the input string
input_string="example"
# Use Perl to remove the first character
trimmed_string=$(echo "$input_string" | perl -pe 's/^.//')
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
With the $(echo "$input_string" | perl -pe 's/^.//')
, the script removes the first characters from the input string. Using's/^.//
, the perl command removes the first characters with ^
. and replaces it with an empty string (//).
From the image you can see, that the script has removed the first character “e” from the input string example and returned the output “xample”.
Remove Each First Character of an Input String
To remove the first characters of all the substrings in a large string, you can use a loop with parameter expansion. Check out the following script:
#!/bin/bash
# Define the input string
input_string="All the first characters of each substring have been removed."
# Split the input string into words based on whitespace
read -ra words <<< "$input_string"
# Loop through each word and remove the first character
trimmed_words=()
for word in "${words[@]}"; do
trimmed_words+=("${word:1}")
done
# Join the trimmed words back into a single string
trimmed_string=$(IFS=" "; echo "${trimmed_words[*]}")
# Print the result
echo "Original String: $input_string"
echo "Trimmed String: $trimmed_string"
At first, the script declares the input string in a variable namedinput_string
. The read -ra words<<<"$input_string"
splits the input string based on whitespace and stores each substring in a variable named words. After that, the for loop iterates over each element of the input string. Inside the loop, the${word:1}
extracts each element removing the first character. In the trimmed_words
array, the script stores all the elements with their first character removed. At last, the$(IFS=" "; echo "${trimmed_words[*]}")
joins the modified substrings back into a string using space as a separator.
From the image you can see, that the script has removed the first character of each substring of the input string.
Remove Each First Character of an Array
To remove the first character of all the elements of an input array, use the following script:
#!/bin/bash
# Define an array
input_array=("apple" "kiwi" "orange")
# Loop through the array and remove the first character of each element
trimmed_array=()
for element in "${input_array[@]}"; do
trimmed_array+=("${element:1}")
done
# Print the original and trimmed arrays
echo "Original Array: ${input_array[*]}"
echo "Trimmed Array: ${trimmed_array[*]}"
Firstly the script declares an array in a variable namedinput_array
. Then it initializes an array in a variable namedtrimmed_array.
The for loop iterates over all the elements of the input string. After that, the${element:1}
extracts a substring starting from the 2nd character to the end.
The image shows the script has removed all the first characters of each element of the input string.
Conclusion
To sum up, the article shows different kinds of methods to remove the first character from a bash string. Using pure bash scripting (parameter expansion) and external commands like awk, sed, perl you can easily remove the first character. Hope this article helps you get better at working with strings and removing the first character correctly.
People Also Ask
Why is it important to remove the first character?
Removing characters is important to get rid of unwanted spaces and characters from an input string or a file. It is also important to clean up data, for formatting, parsing, and preprocessing. This task needs techniques like parameter expansion and external commands such as awk
, sed
, and Perl
.
How to remove the first two characters from a bash string?
To remove the first two characters from a bash string, you can use the parameter expansion. In the parameter expansion, set the offset at2
then it will extract a string starting with the 3rd character or remove the first two characters. Check out the following script:
#!/bin/bash
string="your_string_here"
trimmed_string="${string:2}"
echo "Original String: $string"
echo "Trimmed String: $trimmed_string"
#output:Original String: your_string_here
#Trimmed String: ur_string_here
How to delete the first character from each line of a file?
To delete the first character from each line of a file, you can use the sed command. After the sed command, specify the input file name and redirect the output to a new file. This will not modify the existing file rather than create a new file. Take a look at the script:
#!/bin/bash
# Define input and output file names
input_file="colors.txt"
output_file="newcolors.txt"
# Apply sed command to remove the first character from each line
sed 's/^.//' "$input_file" > "$output_file"
How to remove the last character from a bash string?
To remove the last character from a bash string, you can use the parameter expansion with %? and it will remove the last character from the input string. Check the following script below:
#!/bin/bash
# Assign a string to the variable 'string'
string="your_string_here"
trimmed_string="${string%?}"
echo "Original String: $string"
echo "Trimmed String: $trimmed_string"
#output:Original String: your_string_here
#Trimmed String: your_string_here
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial