FUNDAMENTALS A Complete Guide for Beginners
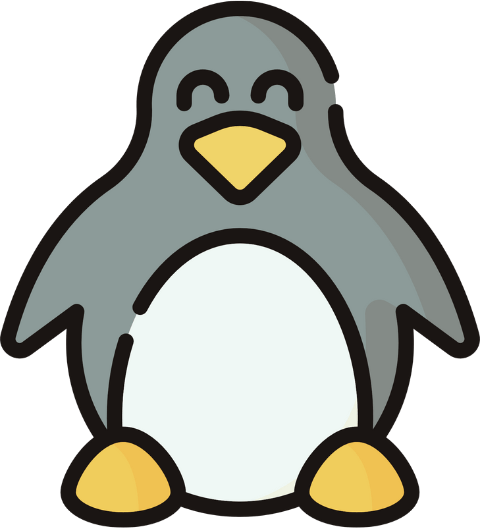
Here string is a practice to pass a string as input to a command. Here are some syntaxes to use here-string in Bash:
- Counting word numbers in a string:
wc -w <<< 'String Value'
- Searching for a pattern inside a file:
grep -q "pattern" <<< "$file_name"
- Printing contents inside a variable:
cat <<< $variable_name
- Replacing the spaces with newline character:
tr ' ' '\n' <<< ${array_name[*]}
- Using another command:
cat <<< “your string” | command
In this article, I will explore some techniques for using here string in Bash incorporating the above-mentioned approaches.
What are “Here Strings”?
Here string is an approach of passing a string to a command using “<<<” operator. This operator sends the contents of the string to the command on its left. The basic syntax for using the here-string is:
command <<< $string
The ‘<<<‘ operator will pass the string to the command that reads from standard input.
Why Use “Here Strings”?
Here string is useful to pass a short string to a command. It reduces the necessity for echo commands or temporary files. Here string makes the bash code compact, concise, and straightforward.
6 Basic Examples of Using “Here String” in Bash
In this section, I have demonstrated some simple examples of using the here-string in Bash script.
1. Using “wc” Command with “Here Strings”
Here string passes a string to a command. In this case, a string will be passed to the wc command, which will count the total word number inside the input string. The complete bash script is below:
wc -w <<< 'Welcome to LinuxSimply'
Here, the ‘Welcome to LinuxSimply’ is passed to the wc -c
command as here string that calculates the word count of the passed string and shows the count on the terminal.
The wc command has counted the total word number inside the passed string.
2. Using Parameter with “Here Strings”
Using parameters in the “here string” operation makes the script dynamic. It enables to change the output of the command based on the value stored inside the parameter. Here is a complete bash script on how to use here string with parameter:
#!/bin/bash
file='hi hello sh'
if grep -q "sh" <<< "$file"
then
echo "$file contains the substring sequence \"sh\""
fi
In the above script, if grep -q "sh" <<< "$file"
defines an if statement that searches for the substring ‘sh’ in the string stored within the parameter “file” using the grep command with the -q option. The -q option tells the grep command to suppress the output and only sets the exit status.
3. Using Variables with “Here Strings”
Using a variable in here string operation eases the execution of the command with contents inside the variable. The following is a complete bash script to print the content of a string variable on the terminal using here-string:
#!/bin/bash
variable="Welcome, Here!"
cat <<< $variable
Part of the script, cat <<< $variable
passes the variable to the cat
command that prints the content of that variable.
4. Escape Special Characters
For escaping special characters in here string, enclose the string with a single quote (‘ ‘) instead of double quote (“ “). Here is a bash script to escape the new line character ‘\n’:
#!/bin/bash
w=$'Welcome\nhere'
echo "$w"
In the script, w=$'Welcome\nhere'
declares the w variable that contains a string including the ‘\n’ character. Then, the echo "$w"
prints the content of the w variable, including the new line on the terminal.
The script escapes the “\n” characters.
5. Using “Here Strings” with Arrays
Here string can handle arrays. Here, the Bash script will replace all spaces with new line characters using the tr command:
#!/bin/bash
array=(Welcome to LinuxSimply)
tr ' ' '\n' <<< ${array[*]}
The tr ' ' '\n' <<< ${array[*]}
translate the spaces (‘ ‘) to newline characters (‘\n’) in the new input text. Thus, it splits the input text with spaces into separate lines.
6. Using “Here Strings” with Multi-line Input
Multiline input means putting more than one line one after another as input. However, to use here string with multi-line input, put the lines one after another to pass multiple lines to a command using the here-string. Here is a Bash script to do so:
#!/bin/bash
cat <<< "Hello 1
Hello 2
Hello 3"
In this code, three lines have been passed to the cat command using the here-string ‘<<<’. Then, the bash script has printed the three lines on the terminal separately.
Advance Applications of “Here String” in Bash
Let’s explore some advanced applications of here string in Bash:
1. Counting Network Interfaces
The netstat command is a tool to display network connections, routing tables, and interface statistics. To count the network interface, execute the below command on the terminal:
wc -w <<<$(netstat -i | cut -d" " -f1 | egrep -v "^Kernel|Iface|lo")
The netstat -i
command displays information about network interfaces. After that the cut -d" " -f1
commands extract the first field from each line of the output of netstat -i. egrep -v "^Kernel|Iface|lo"
command filters out lines that start with “kernel”, “Iface”, or “lo” from the output of the previous command. Then the result is passed to the wc -w command using here string “<<<”, which counts the number of words in the input text, thus providing an estimate of the number of network interfaces.
The image shows the count of the network interface.
2. Prepending a Line to a File
A simple way to prepend text to a file using a combination of user input, file handling, and here strings in Bash. The below Bash script appends a title with the content of the original file to a new file:
#!/bin/bash
E_NOSUCHFILE=85
read -p "File: " file
if [ ! -e "$file" ]
then
echo "File $file not found."
exit $E_NOSUCHFILE
fi
read -p "Title: " title
cat - $file <<<$title > $file.new
echo "Modified file is $file.new"
exit
E_NOSUCHFILE=85
defines an error code constant indicating a file was not found. read -p "File: " file
reads input from the user and stores it to the file variable, and the -p option enables it to display ‘File:’ text on the prompt. if [ ! -e "$file" ]
checks if the file does not exist. read -p "Title: " title
reads the title. cat - $file <<<$title > $file.new
concatenates the title provided by the user with the contents of the file, then redirects the concatenated content to a new file named original_file_name.new.
A title with the content of the original file has been prepended to the new file.
3. Parsing a Mailbox
Here string helps to parse important information from the mailbox. Here is a complete bash script to find out the IP address of the sender from the mailbox:
#!/bin/bash
E_MISSING_ARG=87
if [ -z "$1" ]
then
echo "Usage: $0 mailbox-file"
exit $E_MISSING_ARG
fi
mbox_grep()
{
declare -i body=0 match=0
declare -a date sender
declare mail header value
while IFS= read -r mail
do
if [[ $mail =~ ^From ]]
then
(( body = 0 ))
(( match = 0 ))
unset date
elif (( body ))
then
(( match ))
elif [[ $mail ]]; then
IFS=: read -r header value <<< "$mail"
case "$header" in
[Ff][Rr][Oo][Mm] ) [[ $value =~ "$2" ]] && (( match++ )) ;;
[Dd][Aa][Tt][Ee] ) read -r -a date <<< "$value" ;;
[Rr][Ee][Cc][Ee][Ii][Vv][Ee][Dd] ) read -r -a sender <<< "$value" ;;
esac
else
(( body++ ))
(( match )) &&
echo "MESSAGE ${date:+of: ${date[*]} }"
echo "IP address of sender: ${sender[1]}"
fi
done < "$1"
}
mbox_grep "$1"
exit $?
mbox_grep()
defines the mbox_grep function. if [ -z "$1" ]
checks whether the passed argument is empty. declare -i body=0 match=0
declares two integer variables ‘body’ and ‘match’ and initializes them to 0. declare -a date sender declares two arrays ‘date’ and ‘sender’. After that, while IFS= read -r
mail initiates a loop to read each line of the mailbox file into the variable mail.
Then, if [[ $mail =~ ^From ]]
checks if the line starts with “From” which indicates the start of a new email. Then, unset date unsets the date array. Elif (( body )) checks if the body is non-zero and (( match )) checks if match is non-zero.
Afterward, elif [[ $mail ]];
checks if the line is not empty. ‘IFS=: read -r header value <<< “$mail”
splits the line by a colon and assigns the parts to ‘header’ and ‘value’. echo "IP address of sender: ${sender[1]}"
prints the IP address of the sender. mbox_grep "$1"
calls the mbox_grep function passing the argument file.
Best Practices When Using Here Strings
Some best practices for using here-string in Bash properly are.
- Use Here Strings for Short Strings: Here string is useful to pass short strings to a command. It is not good practice to pass large amounts of data to command through here string.
- Avoid Using Here Strings for File Contents: To pass file contents to a command, choose redirection rather than here-string.
- Use Quotation Marks for Variables: Use quotation marks for variables to overcome unwanted errors due to special characters or whitespace.
- Prefer Here Strings to echo and a pipe: Here string is a preferable alternative to the echo command and the pipe command that makes Bash script concise.
- Consistent Indentation: Maintaining consistent indentation makes the Bash script more readable.
- Avoid Using Sensitive Data: Avoid using sensitive data with the here-string as it exposes the data directly on the command line.
Conclusion
This article has discussed some practical approaches to using here-string in Bash. These techniques will equip the users to make the Bash script concise and pass the variable to the necessary commands.
People Also Ask
What is the difference between heredoc and here string?
The main difference between a heredoc and a here string is here string does not need a delimiter token, but a heredoc needs one. Here string prefers delimiter when it passes some string into a command.
How do you check if a string matches in Bash?
To check if a string matches in bash, use the = or the == operator. Use the = operator for POSIX (Portable Operating System Interface) compatibility. For checking strings not equal to, use != operator.
How to initialize a string in Bash?
To initialize a string in Bash, use the syntax: string_name=’your string value’
. Moreover, to print the string on the terminal, use the syntax: echo $string_name
.
How do I find the length of a string in Bash?
To find the length of a string in Bash, use the ${#string_name}
syntax. It will return the length of the string_name variable.
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial
Thanks for sharing. I am a newbie and am getting started with Linux and shell scripting. This article is very beginner-friendly.