FUNDAMENTALS A Complete Guide for Beginners
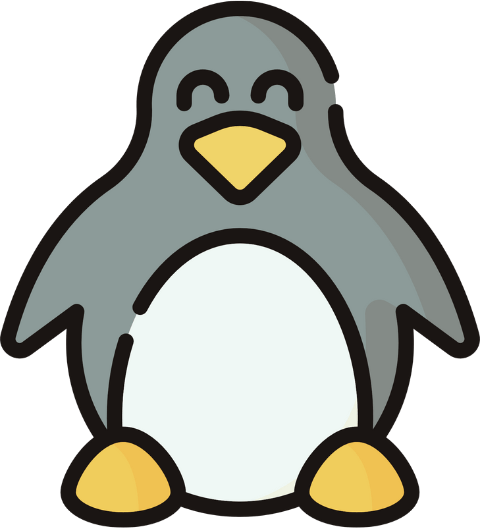
In bash scripting, converting text to lowercase or uppercase can make it easier to handle the input or output formats of files, process or compare data, and deal with case-sensitive operations, such as matching strings or file names.
To convert a string to uppercase, check the following methods:
- Using Parameter Expansion:
${String^^}
- Using the “tr” command:
tr [Option] [set1] [set2]
- Using sed command:
sed 's/pattern/replacement/' filename
- Using awk command:
echo "input_str" | awk 'pattern { action }'
- Using perl command:
echo "$input_string" | perl [Option] 'action'
- Using declare command:
declare -u variable_name
- Using ASCII value with a case statement and for loop.
Dive into the article to learn these methods of how to convert a Bash string to uppercase in detail.
1. Using Parameter Expansion
Using the parameter expansion, you can simply convert the characters of the input string into uppercase. Parameter expansion refers to getting and modifying the value stored in the parameter or variable. If you use the^
and^^
then you can convert the first character and all characters to uppercase respectively.
- To convert the first character to uppercase use the
^
operator. Check the following code:#!/bin/bash # Define a variable my_variable="the first input string" # Convert the variable to uppercase uppercase_variable="${my_variable^}" # Print the result echo "Original string: $my_variable" echo "Uppercase: $uppercase_variable"
EXPLANATIONFirstly the script declares a string into a variable named
my_variable
. Then the${my_variable^}
syntax converts the first character of the input string to uppercase. Later, the echo command prints the converted output in the terminal.The output shows the operator
^
has converted the first character “t” to uppercase “T”. -
To convert all the characters of a bash string to uppercase, use the
^^
operator. Follow the script below:#!/bin/bash # Define a variable my_variable="All the characters are converted to uppercase" # Convert the variable to uppercase uppercase_variable="${my_variable^^}" # Print the result echo "Original string: $my_variable" echo "Uppercase: $uppercase_variable"
EXPLANATIONThe script uses a bash parameter expansion syntax
${my_variable^^}
which converts all the characters of themy_variable
to uppercase.The output shows the
^^
operator in the parameter expansion has converted the input string to uppercase.
Note: Bash 4.0 and above versions support the^
and^^
operator for converting the characters to uppercase.
2. Using “tr” Command
To convert a bash string to uppercase, you can use the tr command. In bash scripting, the tr
command translates and deletes characters from a string. You can also use this command for replacing, searching, and converting the case of the string. Check out the script below:
#!/bin/bash
lowercase="All the characters have been converted to uppercase"
uppercase=$(echo "$lowercase" | tr '[:lower:]' '[:upper:]')
echo "Uppercase: $uppercase"
At first, the bash script declares a string in a variable namedlowercase
. In theuppercase=$(echo "$lowercase" | tr '[:lower:]' '[:upper:]')
, the | (pipe) symbol pipes the output of theecho
command to thetr
command. Then the tr command matches the lower-case characters and replaces them with uppercase characters.
The output shows the script has converted the input string to uppercase.
3. Using “sed” Command
To convert the input string to uppercase, you can use the sed command. The sed
command is a simple yet very useful command for stream editors in bash scripting. Using the backslash (\) with theU
inside the sed
command you can easily convert the string to uppercase. Take a look at the following script below:
#!/bin/bash
# Define a string
my_string="This is the new string."
# Convert the string to uppercase using sed
uppercase_string=$(echo "$my_string" | sed 's/.*/\U&/')
# Print the result
echo "Original input: $my_string"
echo "Uppercase: $uppercase_string"
Firstly, thesed
command takes the output through the pipe operator. In the sed command/(.*/)
captures the string and the\U&
converts the strings’ characters to uppercase. The's/.*/\U&/'
converts all the characters to the uppercase of the input string.
So, using the sed command, the script successfully converted the input to uppercase characters.
Convert Specific Character to Uppercase
To convert all the occurrences of a specific character in a string to uppercase, check the following script below:
#!/bin/bash
lowercase="hello world"
uppercase=$(echo "$lowercase" | sed 's/o/\U&/g')
echo "Uppercase: $uppercase"
The pipe operator takes the output of the echo command to thesed
command. Inside the sed command, 's/o/\U&/g'
is a command substitution that converts all occurrences of “o” to uppercase.
The image shows the script has converted a specific character “o” of the string to uppercase.
Convert Specific Element to Uppercase in a File
To convert a specific element to uppercase in a file, firstly you need to specify the file name. Once you specify the file name then perform the necessary operations using the commands. Check out the script below:
#!/bin/bash
# Check if filename is provided
if [ -z "$1" ]; then
echo "Usage: $0 <filename>"
exit 1
fi
filename="$1"
# Check if file exists
if [ ! -e "$filename" ]; then
echo "File '$filename' does not exist."
exit 1
fi
# Loop through each line in the file and convert 'o' to uppercase
while IFS= read -r line; do
uppercase=$(echo "$line" | sed 's/o/\U&/g')
echo "$uppercase"
done < "$filename"
Firstly using the if statement the script checks if a filename is provided as an argument. Then the script provided that filename as an argument using$1
in a variable namedfilename
. After that, the script checks whether the file exists or not using the if statement. To read each line of the file, the script uses a while loop. Inside the loop, thesed
command converts all the occurrences of the specified character to uppercase using global substitution-g
and\U&
.
Using the cat command, check the contents of a file.
The cat command shows all the existing elements of the file.
From the image, you can see the sed command has converted all occurrences of the specific character “o” to uppercase.
4. Using the “awk” Command with “toupper()” Function
To convert an input string to uppercase, you can use the awk command. The bash script uses the external command awk for text processing, handling multi-byte characters, and especially for pattern matching and manipulation. The awk command usestoupper()
function to convert the string to uppercase. Take a look at the following script:
#!/bin/bash
# Define a string
my_string="New converted string."
# Convert the string to uppercase using awk
uppercase_string=$(echo "$my_string" | awk '{print toupper($0)}')
# Print the result
echo "Original string: $my_string"
echo "Uppercase: $uppercase_string"
The script uses|(pipe)
operator to take the output of theecho
command to theawk
command. In theawk '{print toupper($0)}'
, the awk command converts the output of the echo command to uppercase using its functiontoupper
. In awk, the($0)
indicates the entire output from the pipe operator
.
From the image you can see, thetoupper
function has converted all the characters of the input string to uppercase.
5. Using the “perl” Command with “uc” Function
In bash scripting, the perl command provides a simple way to convert to uppercase. The perl command reads each line of the input then uses the uc function to convert the input to uppercase and later it displays the modified output. To convert a string to uppercase using perl
command, check the following script:
#!/bin/bash
# Define a string
my_string="This string will be converted to uppercase"
# Convert the string to uppercase using Perl
uppercase_string=$(perl -e "print uc('$my_string')")
# Print the result
echo "Original String: $my_string"
echo "Uppercase: $uppercase_string"
Firstly the-e
option tells the perl command to execute the code as a command line argument. Then theuc
which is a function of the perl command, converts all the characters to uppercase of the input stringmy_string
. The script stores the modified string in a variable named uppercase_string
.
The output shows the script successfully converted all the characters to uppercase.
Convert Multibyte Characters to Uppercase
To convert multibyte characters you can use theperl
command. Check the following script:
#!/bin/bash
#Converting the multibyte characters to lowercase
input_string="hello æøå"
uppercase_string=$(echo "$input_string" | perl -CS -pe '$_ = uc($_)')
echo "Original string: $input_string"
echo "Uppercase: $uppercase_string"
Firstly the script declares a multibyte string in a variable namedinput_string
. In the$(echo "$input_string" | perl -CS -pe '$_ = uc($_)')
syntax, the pipe operator
takes the output of theecho
command to the perl command. In the perl
command, the script uses-CS
option to specify theUTF-8encoded input and output. The$_
specifies the current line and the uc()
function converts the characters to uppercase.
From the image, you can see that the perl command has converted the multibyte characters æøå to uppercase.
Note: While handling multibyte characters, the tr command does not show the expected result. The awk
command can handle multibyte characters well, but there might be specific cases where it doesn’t produce the expected result. So it is recommended to use the perl command while handling multibyte characters.
6. Using the “declare” Command
While declaring a variable if you use the-u
flag, the declare command will change all the letters in the input to uppercase. To convert the input string to uppercase, use the following script:
#!/bin/bash
# Define a string
my_string="hello world"
# Convert the string to uppercase using declare
declare -u uppercase_string="$my_string"
# Print the result
echo "Uppercase: $uppercase_string"
At first, the bash script declares a string into a variable named my_string
. Moreover, the script uses the-u
option with the declare command which converts all the characters to uppercase. At last, theecho
command prints the output of theuppercase_string
variable in the terminal.
From the image you can see, the declare command has converted the “hello world” string to “HELLO WORLD”.
7. Using ASCII Values
To convert the characters of a string to uppercase, you can use the ASCII value of the characters. Using the ASCII value with the arithmetic operation in the loop, you can convert all the characters of the input string. Check the following code:
#!/bin/bash
# Define a string
my_string="The string has been converted to uppercase."
# Function to convert a character to uppercase
to_uppercase() {
local char="$1"
case "$char" in
[a-z])
char_code=$(printf "%d" "'$char") # Get the ASCII value of the character
char_code=$((char_code - 32)) # Convert ASCII value to uppercase
printf \\$(printf '%03o' "$char_code") # Print the uppercase character
;;
*)
printf "%s" "$char" # If not a lowercase letter, print as it is
;;
esac
}
# Convert the string to uppercase
uppercase_string=""
for (( i=0; i<${#my_string}; i++ )); do
uppercase_string+=$(to_uppercase "${my_string:$i:1}")
done
# Print the result
echo "Original String: $my_string"
echo "Uppercase: $uppercase_string"
At first, the script declares a function that takes single characters as input and returns the uppercase characters. In the function, the script uses a case statement that checks whether the input character is lowercase or not. If the character is lowercase then it calculates the ASCII value and returns the corresponding character. The for loop iterates over each character. For each character, the loop calls the function and appends the output to the variableuppercase_string
.
The image shows the loop and case statement function has converted all the characters of the input string to uppercase.
Conclusion
The article has highlighted the importance of converting the case of a string and shows some practical methods to convert characters to uppercase. Using the parameter expansion you can easily change the case of a character. For versatile results, you can use external commands such as tr, awk, sed, and perl. Hope this guide will give you a clear concept of converting a bash string to uppercase.
People Also Ask
How do you change a string from lowercase to uppercase?
To change a string from lowercase to uppercase you can thetr
command. The tr command transforms the characters of the first set with the second characters of the second set. Check the following script:
#!/bin/bash
# Define your string
input_string="hello world"
# Use tr to convert to uppercase
uppercase_string=$(echo "$input_string" | tr '[:lower:]' '[:upper:]')
# Print the result
echo "$uppercase_string"
#Output: HELLO WORLD
How do you change a string from uppercase to lowercase?
To change a string from uppercase to lowercase, you can use pure bash scripting parameter expansion with the,,
operator. Then the operator in the parameter expansion will convert all the characters to lowercase. Check out the following script:
#!/bin/bash
original_string="HELLO WORLD"
lowercase_string="${original_string,,}"
echo "Original String: $original_string"
echo "Lowercase String: $lowercase_string"
#Output: Original String: HELLO WORLD
#Lowercase String: hello world
Which function converts a string to lowercase?
There is no direct function to convert a string to uppercase. To convert a string to uppercase you can use {string^^}
and tr command that will directly change the case of the input string. You can usetoupper
anduc
functions but for this, you need to use external commands likeawk
and perl
.
How do I convert a list of strings in a file to uppercase?
To convert a list of strings in a file to uppercase, you can use a while loop. The while loop reads each element with each iteration and converts each character of the file to uppercase using the tr command. Take a look at the following code:
#!/bin/bash
# Check if filename is provided
if [ -z "$1" ]; then
echo "Usage: $0 <filename>"
exit 1
fi
filename="$1"
# Check if file exists
if [ ! -e "$filename" ]; then
echo "File '$filename' does not exist."
exit 1
fi
# Loop through each line in the file and convert to uppercase
while IFS= read -r line; do
uppercase_line=$(echo "$line" | tr '[:lower:]' '[:upper:]')
echo "$uppercase_line"
done < "$filename"
#Output: ORANGE,PINK,BLUE.
#BLACK
#MAGENTA
#GREEN,YELLOW.
#RED,SKY-BLUE
#PURPLE,INDIGO
How do I convert the elements of an array to uppercase?
To convert the elements of an array to uppercase, firstly you need to declare the array in a variable. Then use a loop that can iterate each element of the array. Inside the loop, utilize a tr command that converts each character from lowercase to uppercase after each iteration. At last print the modified output using theecho
command. Check the following script:
#!/bin/bash
# Define your list of strings
strings=("hello" "world" "this" "is" "a" "test")
# Loop through each string and convert to uppercase
for ((i=0; i<${#strings[@]}; i++)); do
strings[$i]=$(echo "${strings[$i]}" | tr '[:lower:]' '[:upper:]')
done
# Print the resulting list
echo "${strings[@]}"
#Output: HELLO WORLD THIS IS A TEST
Related Articles
- How to Convert a Bash String to Int? [8 Methods]
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial
The parameter expansion one at 1. doesn’t work:
my_variable=”the first input string”
uppercase_variable=”${my_variable^}”
echo “Uppercase: $uppercase_variable”
Uppercase: The first input string
This was run on Bash 5.2.26(1)-release
Here I have intended to convert the first letter to uppercase using parameter expansion. For that reason, I have used single caret (^). If you look at the output of your code, then you can see that it also worked for you and it converted the first character of the input “t” to “T”.
Disregard, That one works because it’s just the first letter.
That’s still a weird example to show, I’d get rid of the “upper case the first letter” and just go with the double carat one that does the whole string.
In the first example of parameter expansion, I used the single caret (^) to change just the first letter to uppercase. If you read the explanation before the code, it’ll make sense. If you only want to make all the letters in a string uppercase, take a look at the second example in parameter expansion, as well as other methods mentioned in the article.