FUNDAMENTALS A Complete Guide for Beginners
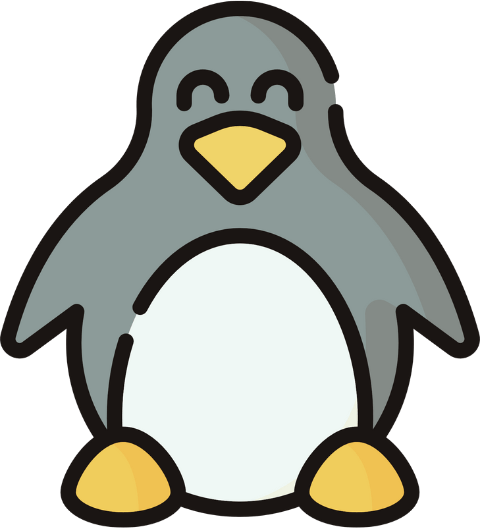
In bash scripting, the conversion of the string is important for arithmetic operations. You can not perform the arithmetic operations on the string representation numbers. Also, the string conversion is important for data validation, comparison, and formatting output. So, for effective and efficient scripting, a clear concept of how to convert a bash string to int (integer) is essential.
To convert a string into an int( integer) in bash, check the following methods:
- Using arithmetic expansion operator:
$((input_string))
- Using the expr command:
expr EXPRESSION
- Using the let command:
let EXPRESSION
- Using the bc command:
echo "expression" | bc [options]
- Using the awk command:
echo "input_str" | awk 'pattern { action }'
- Using the perl command:
perl [options] -e 'action'
- Using a loop with conditional statements
- Using the python command:
python3 [option] {action}
.
Dive into the article to learn these methods of how to convert a bash string to an integer in detail.
1. Using the “$((…))” Arithmetic Expansion Operator
To convert a string into an integer, you can use the arithmetic expansion operator $((…))
. In bash scripting, the arithmetic expansion operator is a simple but very powerful tool for performing calculations and manipulation of numerical values. This operator only works on the integer value. The $((…)) interprets the expression within the parentheses as an arithmetic expression. If the expression contains the pure numerical string, then the operator converts the string into an integer and performs the expression. Check the script below:
#!/bin/bash
# String containing a number
string_number="42"
# Convert string to an integer using arithmetic expansion
integer_result=$((string_number))
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
At first, the script declares the input string in a variable named string_number
. The integer_result=$((string_number))
syntax converts the string to its integer value. At last, the echo command prints the converted integer value in the terminal.
The image shows the script has converted the input string “42” which contains two characters “4” and “2” into integer value 42.
Mixing Another String in the Expression
If you try to add a pure numeric string with another character string inside the arithmetic operator then it will only show the integer value. Take a look at the following script:
#!/bin/bash
# String containing a number
string_number="42+Hello"
# Convert string to an integer using arithmetic expansion
integer_result=$((string_number))
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
In the variablestring_number
, the script adds a character string with a numerical string. Then with integer_result=$((string_number)) syntax
, the script will convert the numeric string into its integer value.
The output shows that the script has only converted the numerical string into the integer value.
2. Using the “expr” Command
To convert a bash string into an integer, you can use the expr command. The bash script uses the expr command to evaluate expressions and manipulate strings. But you can also use the following command for converting a string into an integer by adding a numerical value to the input string:
#!/bin/bash
# String containing a number
string_number="42"
# Convert string to integer using expr
integer_result=$(expr "$string_number" + 0)
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
Firstly the script declares a number as a string in a variable named string_number
. Then ininteger_number=$(expr "$string_number" + 0)
the expr command converts the string into a number. Adding0
with the string forces bash to interpret the string as an integer and perform the arithmetic expression. Later, the echo command prints the string as a number into the terminal.
The output shows the expr command converts the original string “123” into integer value 123.
3. Using the “let” Command
In bash scripting, the let command evaluates the arithmetic operations and assignments. The command performs the expression that the script declares after it.
To convert the bash string into an integer using the let
command, take a look at the following script:
#!/bin/bash
# String containing a number
string_number="9876"
# Use the let command to convert the string to an integer
let "integer_result=$string_number"
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
The let "integer_result=$string_number"
syntax converts the string number to its integer value by using the let command
.
The image shows that the script let.sh has successfully converted the string “9876” into its integer value 9876.
4. Using the “bc” Command
To convert a bash string into an integer you can use the bc command. The bash script uses the external command bc as a calculator that evaluates the arithmetic expression. In this method, you need to pipe the input to the bc command and then the bc
command evaluates the input. Check the following script:
#!/bin/bash
# String containing a number
string_number="789"
# Use bc to evaluate the string as an arithmetic expression
integer_result=$(echo "$string_number" | bc)
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
Firstly the script declares a string in a variable named string_number
. Then in integer_result=$(echo "$string_number" | bc)
, the pipe (|) operator redirects the output of the echo command to the bc
command. The bc command converts the string number into an integer value.
The output shows the bc command converts the original string “789” into its integer value 789.
5. Using the “awk” Command
In bash scripting, the awk is a versatile command tool used for text processing and string manipulation. Using the int function, the awk command converts the numeric string into its integer value.
To convert a string into an integer by using the awk
command, follow the script below:
#!/bin/bash
# String containing a number
string_number="456"
# Use awk to extract the numerical value
integer_result=$(echo "$string_number" | awk '{print int($1)}')
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
With the$(echo "$string_number" | awk '{print int($1)}')
the script extracts the integer value to its integer value and stores the output in a variable named integer_result
. The pipe operator
takes the output to the awk
command. The int($1)
syntax takes the first argument of the input and the awk command converts the first argument into an integer value using the int() function. The print action within the awk
command prints the converted output and later the script stores the output ininteger_result
variable.
The image shows that theawk
command has converted the original string “456” into an integer value of 456.
With the awk command, you can also convert a string that contains floating point characters into an integer. Here, the script only converts the integer part of the given string. For example, if you input a string in a variable named string_number=”456.1234″ then it will output the integer part of the string which is 456.
As you can see the script successfully converts the string into an integer value.
6. Using the “perl” Command
To convert a string into an integer value you can use the perl
command. Like theawk
command, the perl uses the int()
function to convert a string into an integer. Follow the script below:
#!/bin/bash
# String containing a number
string_number="123"
# Use perl to convert the string to an integer
integer_result=$(perl -e "print int('$string_number')")
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
Within the $(perl -e "print int('$string_number')")
, the perl -e
executes a perl expression in the script. Then the int function converts the string value to an integer value. The "print int('$string_number')"
prints the integer value of the string_number.
From the image you can see, that the original string is “123” which means three characters “1”, “2” and “3” and the converted output is an integer value of 123.
As for the perl command, it can also convert the integer part of the floating point string into an integer. Here is how it looks:
So, the script has converted the integer part which is 123 of the input string into an integer.
7. Using a Loop to Convert a String to an Integer
Without using any specific command, you can convert a string into an integer using a loop. In bash scripting, a loop allows a block of code that executes repeatedly for a set of specified values or conditions.
To convert a bash string into an int value, check the following code:
#!/bin/bash
# String containing a number
string_number="12345"
# Initialize the integer result
integer_result=0
# Iterate over each character in the string
for ((i = 0; i < ${#string_number}; i++)); do
# Get the current character
current_char="${string_number:$i:1}"
# Check if the character is a digit
if [[ "$current_char" =~ [0-9] ]]; then
# Multiply the current result by 10 and add the digit
integer_result=$((integer_result * 10 + current_char))
else
# Handle non-digit characters if needed
echo "Non-digit character found: $current_char"
fi
done
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
At first, the script declares a string in a variable named string_number
. After that, the script initializes an integer value to 0 in a variable namedinteger_result
. The for loop iterates over each character in thestring_number
variable. The iteration continues until the value ofi
is equal to or greater than the length of the string. The conditional statement checks whether the extracted character is a digit or not. If the character is a digit then the script performs multiplication and addition to convert the string to its integer value. If the string does not contain digits then the script executes the else block.
The output shows the loop has converted the original string 12345 into an integer value 12345.
8. Using “python” to Convert a String to an Integer
To convert a string into an integer you can use the python
command. While using the Python command you need to use the the action within quotes. Here is how:
#!/bin/bash
# String containing a number
string_number="789"
# Use Python to convert the string to an integer
integer_result=$(python3 -c "print(int(float ('$string_number')))")
# Print the result
echo "Original String: $string_number"
echo "Converted Integer: $integer_result"
Within the python3 command, int(float ('$string_number')))
converts the input string whether it is an integer string or floating point number into an integer. Then the echo command prints the modified output.
From the image, you can see the script has converted the input string “789” into an integer value of 789.
Bonus: Convert a Mixed String to an Integer
While converting a string that includes not only digits but also characters, it won’t show the expected result if you try to apply the pure bash scripting(arithmetic operator, loop) or external commands(awk, perl, let, bc). For this type of string, firstly you need to extract the digits from the string and then convert the string to an integer.
- To convert a string that contains both characters and digits into an integer value using bash scripting, check out the following script:
#!/bin/bash # String containing digits and characters mixed_string="123 abc 456 def" # Remove non-digit characters using tr command digits_only=$(echo "$mixed_string" | tr -cd '[:digit:]') # Convert the resulting string to an integer using arithmetic expansion integer_result=$((digits_only)) # Print the result echo "Original String: $mixed_string" echo "Converted Integer: $integer_result"
EXPLANATIONAt first, the script declares a string in a variable named
mixed_string
. After that, the pipe operator redirects the output of theecho
command to thetr
command. Using the -c option the tr command finds the complement of the matched character here the matched characters are the digits and then deletes the complementary characters using the -d option. At last, the script uses the arithmetic expansion operator to convert the extracted output which contains only digits to an integer value.The image shows the script successfully extracted the numerical value from the string and converted it to an integer.
- To convert a string that contains both characters and digits into an integer value in bash using
awk
command, take a look at the following script:#!/bin/bash # Example string containing non-numerical characters string="abc123def" # Extract numerical value using awk and print it digits_only=$(echo "$string" | awk '{ gsub(/[^0-9]/,"",$0); print $0 }') integer_result=$((digits_only)) echo "Integer value: $integer"
EXPLANATIONThe pipe operator takes the output which is the declared string to the
awk
command. Using'{ gsub(/[^0-9]/,"",$0); print $0 }'
the awk command finds and replaces all the occurrences of the non-digit characters([^0-9]) with the empty string(“”) and stores the modified string in aninteger
variable.The output shows the extracted and converted integer value of the input string.
Practice Tasks to Convert a String to an Int(integer)
- Convert the string “8889” into its integer value Using the following command:
- awk command
- bc command
- Convert the mixed string into an integer by extracting digits using the expr command: input_string=“tuyt123 4764 hgh”
- Convert the mixed string into an integer value using the perl command: input_string=“tuyt123 4764 hgh”
Conclusion
The article shows different methods to convert both the simple pure numeric string and mixed string to an integer. Among all the methods, if you want to use the pure bash script method to simply convert a string into an integer then you can use the arithmetic expansion operator and loops. Otherwise, use the external command which provides versatility. Hope this article helps you to learn how to convert a bash string.
People Also Ask
Why should you convert strings to Integer?
To perform arithmetic operations on the string which contains numbers, you need to convert the string to an integer. The other reasons for the conversion are data processing, data validation, and data comparison.
How to convert an empty string to int bash?
To convert an empty string to an integer you can use parameter expansion. Inside the parameter expansion, if the input string is empty or not set then it automatically uses the default value which is 0
. Here the script looks:
#!/bin/bash
# Define the string
empty_string=""
# Convert the empty string to an integer with a default value of 0
integer_result=${empty_string:-0}
# Print the result
echo "Original String: $empty_string"
echo "Converted Integer: $integer_result"
#Original String:
#Converted Integer: 0
How to convert int to string in a shell script?
To convert an integer to a string you can use parameter expansion and command substitution. Inside the quote, use the dollar sign$
as a prefix following the input integer string. Then it will convert the integer into a string. Follow the script below:
#!/bin/bash
# Define an integer
integer_variable=123
# Convert the integer to a string using parameter expansion
string_result="$integer_variable"
# Print the result
echo "Original Integer: $integer_variable"
echo "Converted String: $string_result"
#Original Integer: 123
#Converted String: 123
Related Articles
- How to Format a String in Bash? [Methods, Examples]
- How to Convert Bash String to Uppercase? [7 Methods]
- How to Convert Bash String to Lowercase? [7 Methods]
- How to Replace String in Bash? [5 Methods]
- How to Trim String in Bash? [6 Methods]
- How to Truncate String in Bash? [5 Methods]
- How to Remove Character from String in Bash? [7+ Methods]
- How to Remove First Character From Bash String? [7 Methods]
- How to Remove Last Character from Bash String [6 Methods]
- How to Use String Functions in Bash? [Examples Included]
- How to Generate a Random String in Bash? [8 Methods]
- How to Manipulate Bash String Array [5 Ways]
- How to Use “Here String” in Bash? [Basic to Advance]
- Encode and Decode with “base64” in Bash [6 Examples]
- How to Use “eval” Command in Bash? [8 Practical Examples]
- How to Get the First Character from Bash String? [8 Methods]
<< Go Back to String Manipulation in Bash | Bash String | Bash Scripting Tutorial