FUNDAMENTALS A Complete Guide for Beginners
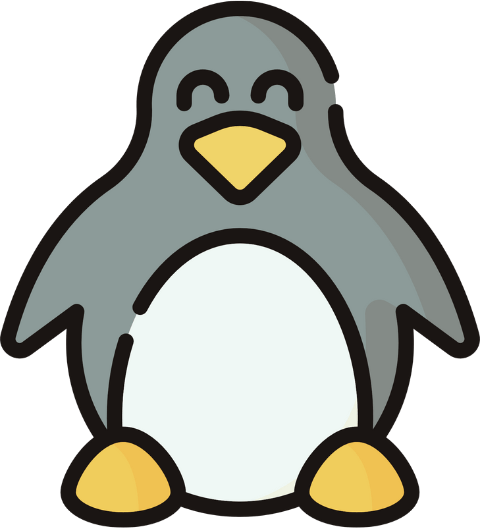
The existence of an environment variable implies that a variable with a particular name has been defined and contains a value (including an empty value) that can be utilized by any programs or processes created by the shell. Note that, Bash supports a number of predefined environment variables for various system and user application purposes. To check whether an environment variable exists, you can employ commands such as env, printenv, and compgen -v piping with grep within the if conditional statement. In addition, the declare -p, -v, -z commands and ${variable+isset} syntax can be used to validate the presence of the environment variable.
In this article, I will show you 6 methods to check if an environment variable exists in Bash with different examples.
What is an Environment Variable in Bash?
An environment variable in Bash is a variable that represents the environment of the shell and is accessible to all the current programs or subsequent child processes initiated by the shell. These variables store information that programs and scripts running in the system use to define the behavior, configuration settings, and preferences of that system. However, Bash includes some predefined environment variables. Here are some commonly used environment variables:
- HOME: Specifies the path to the user’s home directory.
- USER: Indicates the username of the current user.
- PWD: Represents the present working directory.
- PATH: Defines the directories where executable programs are located.
- LANG: Controls language settings.
- SHELL: Specifies the default shell for the user.
- TERM: Dictates the terminal type.
Using the export command in Bash, you can modify and convert a variable to an environment variable.
6 Ways to Check if Environment Variable Exists or Not in Bash
Bash avails several options to check if an environment variable exists or not. The options are -v, -z, and ${variable+isset}, env, printenv, declare -p, compgen -v, etc. Let’s have a clear discussion about them in the following section:
1. Using “-v” Option
The -v
option in Bash validates whether a variable is set i.e. exists or null. In fact, it is the most straightforward method to verify the existence of an environment variable. You can use the -v
option within [ ] or [[ ]] to check if the environment variable exists.
Following is an example to check if an environment variable exists using -v
:
#!/bin/bash
#Exporting the variable ‘env_var’ as an environment variable
export -p env_var="Linux"
#Checking if the environment variable ‘env_var’ exists
if [[ -v env_var ]]; then
echo "The environment variable 'env_var' exists."
fi
This script first exports the variable env_var
as an environment variable using export -p env_var
. Then, the if [[ -v env_var ]]
conditional checks whether ‘env_var’ exists and is set in the environment. Finally, if the condition is satisfied, the script echoes the message ‘The environment variable ‘env_var’ exists.’.
env_var
variable exists as an environment variable.
Check Existence of Multiple Environment Variables
The -v
option allows you to check the existence of multiple environment variables efficiently within a if
statement by using the logical AND (&&) operator in Bash. The following example shows how to check the existence of two environment variables at once:
#!/bin/bash
#Exporting the variables ‘var1’ and ‘var2’ as environment variables
export -p var1="Linux"
export -p var2="Windows"
#Checking if both environment variables ‘var1’and ‘var2’ exist
if [[ -v var1 && -v var2 ]]; then
echo "Both environment variables 'var1' and 'var2' exist."
fi
Here, the script exports two variables var1
and var2
as environment variables. Then, the if [[ -v var1 && -v var2 ]]
conditional checks if the two variables exist and are set in the environment. If the condition is satisfied, and both variables exist, the script echoes the message ‘Both environment variables ‘var1’ and ‘var2’ exist.’.
var1
and var2
are found with their values, the script states them as environment variables that exist like the above image.
2. Employing “compgen -v” Command With “grep”
The compgen -v
command in Bash creates possible completions according to the specified context, such as command, variable, or function. Combine this with the grep command to get a list of all the variables, and then check whether the environment variable is present. Let’s see an example:
#!/bin/bash
#Checking if the environment variable ‘USER’ exists
if compgen -v | grep -q USER; then
echo "The environment variable ‘USER’ exists & its value is $USER."
fi
Here, the grep -q USER
command looks for the string ‘USER’ within the list of variables generated by compgen -v
where the -q
flag refers to the quiet mode which suppresses output. If the condition is satisfied, the if conditional returns a True expression displaying that the ‘USER’ environment variable exists along with its value.
USER
exists and its value is ‘nadiba’ which is the username of my computer.
3. Using “${variable+isset}” Syntax
A form of parameter expansion ${variable+isset}
in Bash can be used to check whether an environment variable exists. If the variable is set and has a non-null value, it returns isset
as true.
Here’s an example how to use it in a script:
#!/bin/bash
#Exporting the variable ‘env_var’ as an environment variable
export -p env_var=41
#Checking if the environment variable ‘env_var’ exists
if [ -n "${env_var+isset}" ]; then
echo "The environment variable 'env_var' exists."
fi
The script first exports the variable env_var
as an environment variable. -n
option checks for non-empty values. So, the if [ -n "${env_var+isset}" ]
conditional checks if the expansion ${env_var+isset}
results in a non-empty string. If it meets the condition, the script displays ‘The environment variable ‘env_var’ exists.’.
env_var
really exists.
4. Using “-z” Option
When using the ‘if’ statement with the -z
option, it checks whether the length of the variable is zero (0). If the variable does not exist or is null, it returns the 0 (True) exit status.
One of the easiest ways to check if there is an environment variable in Bash is to use the “-z” option. Follow the script below to check the existence of the environment variable:
#!/bin/bash
#Checking if the environment variable ‘HOME’ exists
if ! [ -z "$HOME" ]; then
echo "The environment variable 'HOME' exists & its value $HOME."
fi
Here, the ‘if’ conditional with the negation (!) operator if ! [ -z "$HOME" ]
checks if the length of the string contained in the variable HOME
is not zero. If the condition is true, the script displays the output using the echo command.
This image illustrates that the environment variable HOME
exists and its value is ‘/home/nadiba’ in my case.
5. Using “declare -p” Command
The declare -p
command in bash is mainly used to specify variables and attribute them. You can use this command to list all variables and check if an environment variable exists in that list as well. Here’s an example:
#!/bin/bash
#Displaying the attributes and value of the environment variable ‘PWD’
declare -p PWD &>/dev/null
#Checking the exit status of the previous “grep” command
if [ $? -eq 0 ]; then
echo "The environment variable 'PWD' exists & its value is $PWD."
fi
First, declare -p
displays the attributes and value of the variable PWD
environment variable. Then, the if [ $? -eq 0 ]
checks the exit status of the previous command. If it returns zero, the script executes and displays a message confirming that ‘PWD’ exists along with its value.
PWD
and printed its value ‘/home/nadiba’ which is the path of my current working directory.
&>/dev/null
redirects both standard output and standard error to the null device, suppressing any output and error messages from being displayed on the console. 6. Employing “env” Command With “grep”
The env command in Bash lists and prints the environment variables and their values. This command is commonly used to control the environment of a given command by modifying the environment variables temporarily. However, if you want to check if there exists an environment variable, use the env
command and grep
command by piping them together. Following is an example:
#!/bin/bash
#Searching for the environment variable ‘PATH’
env | grep PATH > /dev/null
#Checking the exit status of the previous “grep” command
if [ $? -eq 0 ]; then
echo "The environment variable 'PATH' exists."
fi
In the script, the grep
command searches for the string ‘PATH’ in the output of env
. If it finds ‘PATH’, the exit status will be 0( i.e. a successful match) and will be stored in $?
. Then, the if [ $? -eq 0 ]
statement checks if the exit status is equal to 0, and shows ‘The environment variable ‘PATH’ exists.’.
PATH
and displayed it on the terminal.
- You can replace the
env
command with a distinct Bash commandprintenv
. This command works very similarly to theenv
command and displays only the values of the specified variable without modifying the environment. > /dev/null
within the script redirects the standard output to the null device, suppressing the output from being displayed on the console. It’s not mandatory to use as you can get the same variable existence check without using it.
Conclusion
To conclude, there are several ways to check if an environment variable exists in Bash. The selection of method depends on factors like personal requirements, script readability and complexity. Hence, among all the methods, choose the one that works best for you and your programming style.
People Also Ask
Can I set a default value for an environment variable if it doesn’t exist?
Yes, you can set a default value for an environment variable if it doesn’t exist by using a form of parameter expansion within conditional statements in Bash. The syntax to perform the task is variable=${variable:-default_value}
.
Is there a way to handle sensitive or secret environment variables securely?
To handle sensitive or secret environment variables securely, utilize environment variable tools like dotenv which provides secure handling and loading of sensitive data.
How can I create a function to validate the existence of an environment variable?
To validate the existence of an environment variable in Bash, you can create a function like the following:
#!/bin/bash
# Function to validate the existence of an environment variable
check_existence() {
local env_var=$1
if [ -n "${!env_var}" ]; then
echo "The environment variable '$env_var' exists."
else
echo "The environment variable '$env_var' does not exist."
fi
}
check_existence ENVIRONMENT_VARIABLE
Is there a command-line method to verify the existence of an environment variable?
Yes, as a command-line method, you can use the printenv
and grep
commands to verify the existence of an environment variable. For instance:
printenv | grep -q '^variable_name=' && echo "The variable exists." || echo "The variable does not exist."
How can I improve error handling when working with environment variables?
You can improve error handling when working with environment variables by employing techniques such as displaying error messages or setting exit codes.
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Bash Test Operations in ‘If’ Statement
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- Using Double Square Brackets “[[ ]]” in If Statement in Bash
- 6 Ways to Check If a File Exists or Not in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Negate an “If” Condition in Bash [4 Examples]
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- Different Loops with If Statements in Bash [5 Examples]
- How to Use Flags in Bash If Condition? [With Example]
- Learn to Compare Dates in Bash [4 Examples]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial