FUNDAMENTALS A Complete Guide for Beginners
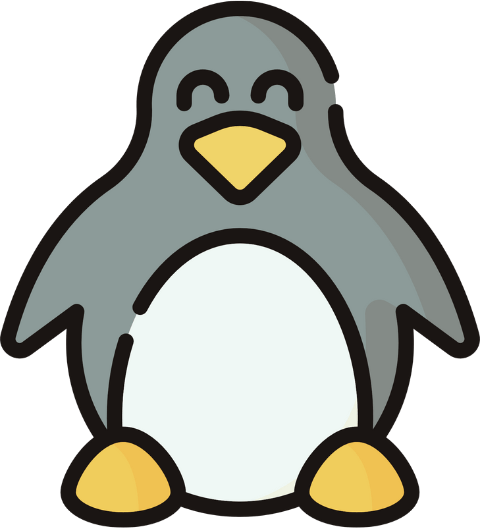
Bash test operation is a prominent way to use the built-in “test” command or the equivalent operator [ ] (square brackets) to check the validity of a conditional expression by returning exit status like 0 for (True/Success) and 1 for (False/Failure).
Performing Test Operations in ‘If’ Conditionals in Bash
The test command performs checks and makes decisions in different conditional cases such as numerical comparisons, string comparisons, file comparisons, etc. Following are 3 different schemes of Bash conditional tests.
Bash ‘if’ Conditional Test for Numerical Comparison
Numerical comparison in Bash means comparing two numbers or numeric variables. However, conditional test operators that can be used for numerical comparison within the test command are: “-eq (equal)”, “-ne (not equal)”, “-gt (greater than)”, “-lt (less than)”, ‘-ge (greater than or equal)”, “-le (less than or equal)”.
1. Equality Comparison Using “-eq”
The “-eq” is an operator that checks if two numbers are equal. The line if [ "$num1" -eq "$num2" ]
returns True if the $num1 and $num2 are equal. The script below shows the use of it:
#!/bin/bash
#Declaring two numbers
num1=6
num2=6
#Checking if the numbers are equal
if [ "$num1" -eq "$num2" ]; then
echo "Equal Numbers"
fi
This output states that the inserted two numbers make the ‘if’ condition true and print “Equal Numbers”.
2. Inequality Comparison Using “-ne”
The “-ne” is an operator that checks if two numbers are not equal. So, to check whether the inserted two numbers are not equal, use the syntax if [ "$num1" -ne "$num2" ]
. Following is an example to check if two numbers are not equal:
#!/bin/bash
#Declaring two numbers
num1=25
num2=5
#Checking if the numbers are not equal
if [ "$num1" -ne "$num2" ]; then
echo "Not-equal Numbers"
fi
The above image depicts that the script successfully satisfies the condition and displays “Not-equal Numbers”.
Bash ‘if’ Conditional Test for String Comparison
When working with Bash scripts, sometimes you may need to compare strings in cases of equality, inequality, sorting and so on. In this effort, you can use these string comparison operators “= (equal)”, “!= (not equal)”, “> (greater than)”, “< (less than)”, “>= (greater than or equal)”, “<= (less than or equal)” within the test command.
1. Checking Null String Using “-z”
The “-z” is an operator that checks if a string is empty (zero length). If the condition [ -z "$string" ]
within an “if” block is satisfied, it returns 0 (success) and executes the code of that block. Here is a detailed example:
#!/bin/bash
#Defining a string
string=""
#Checking if the string is empty
if [ -z "$string" ]; then
echo "Empty String"
fi
From the above image, you can see that the declared variable string contains zero-length which means the string is empty.
2. Checking Non-empty String Using “-n”
The “-n” is an operator that checks whether a string is non-empty (non-zero length). And this is done by using the syntax if [ -n "$string" ]
. For instance:
#!/bin/bash
#Defining a string
string="Hello, Linux!"
#Checking if the string is not empty
if [ -n "$string" ]; then
echo "Not Empty String"
fi
The above image states that the inserted variable length is non-zero meaning that it is a non-empty string.
3. Equality Checking Using “=”
The “=” is an operator that checks if two strings are equal. The syntax if [ "$String1" = "$String2" ]
does the equality checking of the strings. Here’s an example for you:
#!/bin/bash
#Defining two strings
String1="Hi, Linux!"
String2="Hi, Linux!"
#Checking if the strings are equal
if [ "$String1" = "$String2" ]; then
echo "Equal strings"
fi
The above image is a demonstration of equality checking of two strings.
4. Inequality Checking Using “!=”
The “!=” is an operator that verifies and returns true if two strings are not equal. Only using the syntax if [ "$String1" != "$String2" ]
is enough to perform the inequality check. Let’s have a look at the following example:
#!/bin/bash
#Defining two strings
String1="Hi, Linux!"
String2="Hi, Windows!"
#Checking if the strings are not equal
if [ "$String1" != "$String2" ]; then
echo "Not Equal Strings"
fi
In the above image, you will see a demonstration of inequality checking of two strings.
Bash ‘if’ Conditional Test for File & Directory Comparisons
Conditional testing is not only confined to numerical and string comparisons. Several file and directory operations can be performed using the test command in ‘if’ conditional statements in Bash.
Some common test operations can be implemented on files and directories using some Unary Operators where the output becomes true only for the existing file and directory that contains the following characteristics:
Unary Operators | Purposes |
---|---|
-d | Checks if it is a directory. |
-e | Checks if a file/directory exists. |
-f | Checks if it is a regular file. |
-h / -L | Checks if an existing file is a symbolic link. |
-r | Checks if a file is readable. |
-s | Checks if a file size is greater than zero. |
-x | Checks if a file is executable. |
-w | Checks if a file is writable. |
-N | Checks if an existing file has been modified since it was last read. |
-S | Checks if a file is a socket file. |
The following example can be a helpful demo for you to understand how to perform a simple test operation on a file:
#!/bin/bash
#Checking if the file is executable
file="file1.sh"
if [ -x "$file" ]; then
echo "$file is executable."
fi
The above image describes that the assigned file file1.sh is executable.
According to the context of the previous discussion, you can perform conditional tests on pairs of files too by using Binary Operators like “-ot (Checks if a file is older than another one)”, “-nt (Checks if a file is newer than another one)” and so on.
Conclusion
The whole article provides you a proper knowledge of how you can perform different conditional tests in Bash. So, whether it’s for checking numeric values, file permissions, string comparisons, or verifying logical conditions, the test command always empowers you to make proper decisions and create responsive scripts by automating your tasks.
People Also Ask
Is there a shorthand for combining conditions in an ‘if’ statement using the ‘test’ command?
Yes, you can use the logical operators ‘-a (AND)’ or ‘-o (OR)’ within the square brackets as a shorthand for combining conditions in an ‘if’ statement in Bash using the ‘test’ command. For example, [ condition1 -o condition2 ]
Is there any difference between the = and == operators in the ‘test’ command when comparing strings in an ‘if’ statement?
No, there is no difference between the ‘=’ and ‘==’ operators in the ‘test’ command. Both operators are equivalent and you can use any one of your choice when comparing strings in an ‘if’ statement.
How to If a string starts with a specific prefix using the ‘test’ command in an ‘if’ statement?
To check if a string starts with a specific prefix, use a string operator with ‘#’. Here’s the syntax you can follow where the conditional checks if the string starts with a prefix: if [ "${string#prefix}" = "$string" ]
Is there a way to check If a string ends with a specific suffix using the ‘test’ command in an ‘if’ statement?
Yes, you can use a string operator with ‘%’ in an ‘if’ statement in a format like if [ "${string%suffix}" = "$string" ]
to check if the string ends with a specific suffix.
Can I use wildcard patterns with the ‘test’ command in an ‘if’ statement to match filenames?
Yes, you can use wildcard patterns with the ‘test’ command in an ‘if’ statement to match filenames. For instance, if [ -f *.sh ]
checks if there exist any files with the ‘.sh’ extension in the current directory.
How to test If a command is running?
To test if a command is running, use the ps aux
command and then pipe the output to grep
. This ‘ps (process status)’ command checks the list of running processes and the ‘grep’ command then looks for the particular command/process. For example:
#!/bin/bash
#Specifying the process name
process="X"
#Checking if the process is running
if ps aux | grep -q "$process"; then
echo "Yes! $process is running."
fi
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Check If Environment Variable Exists in Bash [6 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- Using Double Square Brackets “[[ ]]” in If Statement in Bash
- 6 Ways to Check If a File Exists or Not in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Negate an “If” Condition in Bash [4 Examples]
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- Different Loops with If Statements in Bash [5 Examples]
- How to Use Flags in Bash If Condition? [With Example]
- Learn to Compare Dates in Bash [4 Examples]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial