FUNDAMENTALS A Complete Guide for Beginners
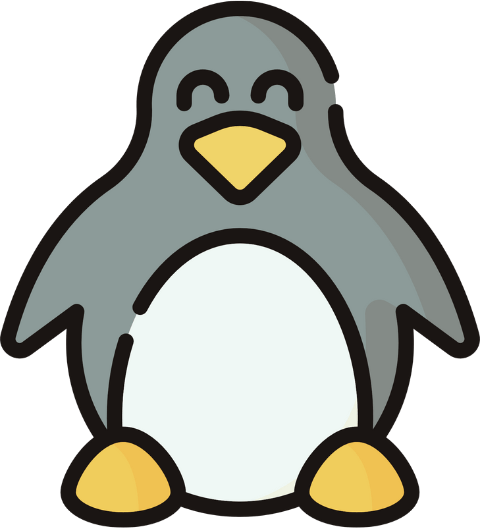
Comparing dates in Bash implies the process of converting dates into a common and standard format (usually in Unix timestamps) and then performing the conditional comparisons. When dealing with any scheduled tasks, automation, backup, or log file analysis, understanding how to compare dates becomes an essential task.
In this article, I’ll explore different practical scenarios or examples of date comparisons in Bash.
Understanding Different Date Formats
The date command in Bash is used to display and format the current date and time of the system. Bash relies on this date command to compare and manipulate dates. Some common date formats are:
- YYYY-MM-DD: Year, month, and day (Human readable format)
- YYYY-MM-DD HH:MM:SS: Year, month, and day Hours, minutes, and seconds (Human readable format)
- +%s: Epoch/Unix timestamp and so on.
Converting Dates to UNIX Timestamp Format
Bash uses the date
command to convert a provided date to UNIX timestamp format. This UNIX timestamp is used to measure time in seconds elapsed from the UNIX epoch, which is January 1, 1970, at 00:00:00 UTC (Coordinated Universal Time).
To convert a date into the UNIX timestamp in the computing system, use the syntax date -d "date_you_want_to_convert" +"%s"
, where the option -d
specifies the date and the format specifier, +"%s"
specifies the output in seconds elapsed since the UNIX epoch. For example:
#!/bin/bash
#Date in YYYY-MM-DD format
date="2024-01-29"
#Date converted to Unix timestamp
converted_date=$(date -d "$date" +"%s")
echo "Unix timestamp for $date is $converted_date"
Output:
4 Examples to Compare Dates in Bash Using If Statement
There are various aspects to compare dates in Bash, from simple comparisons to advanced scenarios including date arithmetic and UNIX timestamps. In the following section, you will see 4 different examples to compare dates in Bash.
Example 1: Checking If a Particular Date Has Already Passed
To check if a particular date has already passed, use the date in UNIX timestamp format and then compare it with the present date using the single square brackets [ ] within an if statement.
Check out the following script to compare the given date with the current one:
#!/bin/bash
#Current date in UNIX timestamp format
present_date=$(date +"%s")
#Converting the input date to UNIX timestamp format
input_date=$(date -d "2020-05-10" +"%s")
if [ "$present_date" -gt "$input_date" ]; then
echo "The input date has already passed."
fi
In the script, if [ "$present_date" -gt "$input_date" ]
checks whether the present date is greater than the given date. If the condition is satisfied, it returns a true expression with an output message. Otherwise, it returns nothing.
As you can see from the image the given date has already passed when compared to the current date.
Example 2: Checking If a File is Older than a Specific Date
During Bash scripting, while working with files, often it’s mandatory to check their creation or modification date and compare them to a specified date for several system administration or monitoring tasks. Let’s navigate to the following script to compare dates and check if a file is older than a specific date:
#!/bin/bash
file_creation_date=$(date -r "file1.txt" +"%s")
specified_date=$(date -d "2024-01-29" +"%s")
if [ "$file_creation_date" -lt "$specified_date" ]; then
echo "The file was created before and is older than the specified date."
fi
The syntax if [ "$file_creation_date" -lt "$specified_date" ]
checks if file_creation_date is less than the specified_date i.e. the file was created before the specified date. If the condition is true, it returns a zero (0) exit status i.e. a successful execution. Otherwise, it executes nothing.
This image states that the file file1.txt mentioned in the script is older than the specific date meaning that it was created before the given date.
Example 3: Calculating the Difference Between Two Dates
In Bash scripting, it’s a very common task to calculate the difference between two dates for scenarios such as schedule management, tracking duration, measuring time intervals between events, etc. In this effort, use the date command and the arithmetic calculation as a straightforward method to accomplish the task.
To calculate the difference between the two dates, go through the following script:
#!/bin/bash
date1=$(date -d "2023-05-08" +"%s")
date2=$(date -d "2024-01-29" +"%s")
#Calculating the difference in seconds
time_difference=$((date2 - date1))
days=$((time_difference / 86400)) #86400 seconds per day
echo "The difference between the given dates is $days."
Here, time_difference=$((date2 - date1))
calculates the time difference in UNIX timestamps between the two specified dates and generates the output in seconds. Then, days=$((time_difference / 86400))
divides the time difference by 86400 (the number of seconds in a day) and calculates the number of day differences and the script finally prints the result.
In the above image, you can see that the difference between the two given dates is 266 days.
Example 4: Comparing Dates in a Loop
When dealing with multiple dates and comparisons among them, it’s helpful to process them in a loop to automate the repetitive tasks efficiently.
Explore the script below to see how a loop iterates through dates and perform arithmetic comparisons within an if statement in Bash:
#!/bin/bash
#An array of dates
dates_array=("2023-09-23" "2024-04-28" "2024-01-29")
#Reference date to compare
target_date="2024-01-29"
#Iterating over each date using for loop
for date in "${dates_array[@]}"; do
#Converting dates to UNIX timestamps
array_timestamp=$(date -d "$date" +%s)
target_timestamp=$(date -d "$target_date" +%s)
if [ "$array_timestamp" -lt "$target_timestamp" ]; then
echo "$date is earlier than $target_date"
elif [ "$array_timestamp" -gt "$target_timestamp" ]; then
echo "$date comes after $target_date"
else
echo "$date and the target date $target_date are same"
fi
done
Here, the for loop iterates over each date in the dates_array. Then, the script converts each date in the array to a UNIX timestamp format, compares them with the target timestamp and shows different results according to the comparisons.
The above image dictates different results of performing date comparisons through a loop.
Conclusion
So far, you have explored several practical scenarios to compare dates in Bash. By practicing these date-handling examples, you can perform several date-based operations and streamline your workflows in Bash scripting.
People Also Ask
What is a Unix timestamp?
A Unix timestamp is a system that is used to represent time as the number of seconds elapsed since the UNIX epoch (January 1, 1970, 00:00:00 UTC (Coordinated Universal Time)). This system provides a standardized way to compute date and time calculations.
Can I compare dates directly in a specific format without converting to Unix timestamps?
Yes, you can compare dates directly in a specific format without converting to UNIX timestamps. However, you need to ensure that the date formats you are using are unambiguous and consistent. For example:
#!/bin/bash
#Defining dates in a specific format
date1="2023-01-01"
date2="2024-01-01"
#Comparing dates directly in the specified format
if [ "$date1" \< "$date2" ]; then
echo "$date1 is earlier than $date2"
elif [ "$date1" \> "$date2" ]; then
echo "$date1 comes after $date2"
else
echo "$date1 and $date2 are the same"
fi
What is the benefit of using Unix timestamps for date comparison?
Unix timestamp, a standardized and numeric representation of time provides several benefits in date comparisons such as:
- Efficient date comparisons
- Accurate date calculations
- Convenient date conversion capability
- Consistency in date handling process
- Flexible date-based calculations etc.
How do I check if a file is newer than a specific date?
To check if a file is newer than a specific date, compare the timestamp of the file’s modification time with the timestamp of the specified date. For example:
#!/bin/bash
specified_date="2024-01-29"
file_modification_date=$(date -r "$file_name" +"%s")
#Converting the specified date to the UNIX timestamp
specified_timestamp=$(date -d "$target_date" +"%s")
#Comparing the modification date of the file with the specified date
if [ "$file_modification_date" -gt "$specified_timestamp" ]; then
echo "$file_name is newer than $specified_date."
fi
Is it possible to compare dates with different formats in Bash?
Yes, it is possible to compare dates with different formats in Bash. However, you need to convert the date into a common format before the comparison. In most cases, UNIX timestamp format is used as a standard system.
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Bash Test Operations in ‘If’ Statement
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Check If Environment Variable Exists in Bash [6 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- Using Double Square Brackets “[[ ]]” in If Statement in Bash
- 6 Ways to Check If a File Exists or Not in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Negate an “If” Condition in Bash [4 Examples]
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- Different Loops with If Statements in Bash [5 Examples]
- How to Use Flags in Bash If Condition? [With Example]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial