FUNDAMENTALS A Complete Guide for Beginners
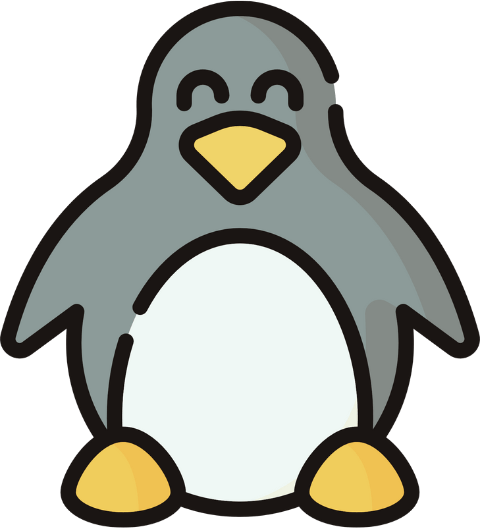
The negation of an if statement implies that the output of the satisfied condition has been altered. To understand the context of negating an ‘if’ condition in Bash, you can exercise several conditional checks such as string comparison, integer comparison and file comparison etc. Additionally, you can combine multiple conditions together and perform a negation operation on them.
In this article, I will show you 4 practical examples to negate an ‘if’ condition in Bash.
What is Negation in Bash?
Negation is the process of inverting or changing the true value of an expression. The logical NOT (!) operator is used to accomplish the task. This negation (!) operator reverses the logical state of a conditional expression. It allows one to execute a code block when the given condition evaluates to False instead of True and vice versa.
To negate an ‘if’ condition, you can append the NOT (!) operator outside [ ] or [[ ]] and alter the entire logic executed inside like the following syntax:
if ! [ condition ]; then
#code to execute if the condition is false
fi
The negate (!) operator can also be used to invert individual conditional expressions inside [ ] or [[ ]] using the syntax if [ ! condition ];
.
4 Examples of Negating an ‘if’ Condition in Bash
Bash avails different forms of negating operational tests on strings, integers, files and directories. Let’s have a clear discussion about them in the following section:
Example 1: Negating String Comparison
The negation (!) operator in string comparison involves reversing the outcome of the string comparison result. In Bash, there are various string comparison operators such as =
(equal to), !=
(not equal to), >
(greater than), <
(less than), >=
(greater than or equal to), <=
(less than or equal to), -z
(string is empty), and -n
(string is not empty).
To negate the string comparison, use the NOT (!) operator along with these string operators. For example:
#!/bin/bash
#Declaring two variables
str1="Linux"
str2="World"
#Checking if two strings are not equal
if ! [ "$str1" = "$str2" ]; then
echo "These strings are not equal."
fi
In the script, the [ "$str1" = "$str2" ]
syntax compares the values of str1
and str2
variables for equality. However, the conditional expression if ! [ "$str1" = "$str2" ];
checks if the strings stored in str1 and str2 are not equal where the negation (!) operator performs the task of inversion (i.e. inverts the False output to True). Lastly, if the condition is satisfied, the script echoes the message ‘These strings are not equal.’.
From the above image, you can see that the given strings are not equal according to the condition.
Example 2: Negating Integer Comparison
In addition to string comparison operators, Bash also provides some integer comparison operators such as -eq
(equal to), -ne
(not equal to), -gt
(greater than), -ge
(greater than or equal to), -lt
(less than), -le
(less than or equal to). The negation (!) operator can also be used to invert the result of the integer comparison expression.
Let’s see an illustration of reversing the integer comparison condition:
#!/bin/bash
#Declaring two variables
num1=2
num2=6
#Checking if two variables are not equal
if ! [ "$num1" -gt "$num2" ]; then
echo "num1 is not less than num2."
fi
Here, the expression if ! [ "$num1" -gt "$num2" ];
evaluates whether the value of num1
is not greater than the value of num2
using the negation (!) operator, where False output is altered into True. Finally, when the condition is satisfied, the script displays an output message.
The above image shows that the given num1 is not greater than num2 according to the condition.
Example 3: Negating Conditions on Files & Directories
Bash includes a number of file test operators to perform checks on files and directories. It’s very common to rewrite a conditional expression using the negation (!) operator in conjunction with different file test operators.
Below is an example explaining how to negate file-related conditions in Bash:
#!/bin/bash
#Checking if the file exists
if ! [ -f "/home/nadiba/Desktop/to/file1.txt" ]; then #Placing the actual path of the desired file in the ‘/path/to/file.txt’ format.
echo "The file does not exist."
fi
Here, if ! [ -f "/home/nadiba/Desktop/to/file1.txt" ]
checks whether the regular file does not exist in the desired directory. If the condition is satisfied, then the script displays ‘The file does not exist.’.
As you can see from the image, in my system, the
file1.txt
exists in the location ‘/home/nadiba/Desktop’. But as I used the negation (!) operator, it outputs file1.txt as a non-existent file.
Example 4: Negating Multiple Conditions
To negate multiple conditions, use the negation (!) operator with the logical operators such as logical AND (&&), logical OR (||). Negating multiple conditions can be of two types i.e. 1) negating individual expression, 2) negating entire expression simultaneously.
Negating Individual Expression in Combined Conditions
To negate individual conditions in multiple conditional expressions, use the negation (!) operator individually for each test condition. You can either use the syntax if ! [ "$VARIABLE" OPERATOR VALUE ]
or if [ ! "$VARIABLE" OPERATOR VALUE ]
to accomplish the task.
Following is an example demonstrating the negation of individual expression in multiple conditions:
#!/bin/bash
#Defining two variables
num1=35
num2=23
#Checking multiple conditions
if ! [ "$num1" -lt 17 ] || ! [ "$num2" != 9 ]; then
echo "At least one condition is true."
fi
In the condition if ! [ "$num1" -lt 17 ] || ! [ "$num2" != 9 ];
, ! [ "$num1" -lt 17 ]
checks if num1
is not less than 17 and ! [ "$num2" != 9 ]
checks if num2
is equal to 9. Consequently, the script executes an output if at least one of these conditions is True.
From the image, you can see that one of these two conditions has been transformed into a true condition because of the inversion by the negation (!) operator.
Negating Multiple Conditions Simultaneously
To negate multiple conditions simultaneously, apply the negation (!) operator only once to a group of combined conditions enclosed within curly braces { }. In this effort, you can use the syntax if ! { [ "$VARIABLE" OPERATOR VALUE ] <logical operator> [ "$VARIABLE" OPERATOR VALUE ] <logical operator> [ "$VARIABLE" OPERATOR VALUE ]; };
.
Here’s an example demonstrating how to negate multiple conditions at once:
#!/bin/bash
#Defining variables
var1="X"
var2=false
var3=8
#Checking multiple conditions
if ! { [ "$var1" = "Y" ] && [ "$var2" = "true" ] && [ "$var3" -eq 8 ]; }; then
echo "At least two conditions are true."
fi
The ‘if’ statement checks the conditions [ "$var1" = "Y" ]
, [ "$var2" = "true" ]
and [ "$var3" -eq 8 ]
combined using logical AND (&&) and encased within the curly braces { }. In this case, the negation (!) operator negates the entire group of conditions, so it evaluates to true if at least two of the conditions are true.
In the above image, at least two conditions out of three are true because of the inversion by the negation (!) operator.
if ! { [ "$var1" = "Y" ] && [ "$var2" = "true" ] && [ "$var3" -eq 8 ]; };
with if ! [[ "$var1" = "Y" && "$var2" = "true" && "$var3" -eq 8 ]];
.Conclusion
Throughout the article, you have learned several schemes of how to negate an ‘if’ condition in Bash. However, among all, select the correct one that is suitable according to your requirements and script readability.
People Also Ask
When should I use ‘negate if’ in Bash scripting?
You should use ‘negate if’ in Bash scripting when you need to run a specific block of code if a certain condition is not satisfied.
Does ‘negate if’ apply only to file and directory checks in Bash?
No, the concept ‘negate if’ is not only confined to file and directory checks in Bash but also it can be used in various schemes such as string comparison, integer comparison etc.
What happens if the condition within ‘negate if’ returns an error in Bash?
If the condition within ‘negate if’ returns an error in Bash, it can affect the performance of the script execution. Generally, the square brackets [ ] are used for performing test operations in Bash. But when the condition inside [ ] returns an error, it leads to undesired consequences. For example:
#!/bin/bash
#Checking if file exists
if ! [ -f "/path/to/nonexistent_file" ]; then
echo "File does not exist."
else
echo "File exists."
fi
Can ‘negate if’ be nested within other conditional statements for complex logic in Bash?
Yes, ‘negate if’ can be nested within other conditional statements for complex logic in Bash. For example:
#!/bin/bash
#Defining variables
num1=7
num2=40
num3=2
#Nested negated conditions
if ! [ "$num1" -eq 12 ]; then
echo "num1 is not equal to 12."
if ! [ "$num2" -lt 39 ]; then
echo "num2 is not less than 39."
if ! [ "$num3" -gt 5 ]; then
echo "num3 is not greater than 5."
fi
fi
fi
Are there any common mistakes when using ‘negate if’ in Bash?
Yes, one of the most common mistakes when using ‘negate if’ in Bash is not setting group conditions properly, as well as not knowing the correct syntax and the logical result of a negated condition.
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Bash Test Operations in ‘If’ Statement
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Check If Environment Variable Exists in Bash [6 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- Using Double Square Brackets “[[ ]]” in If Statement in Bash
- 6 Ways to Check If a File Exists or Not in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- Different Loops with If Statements in Bash [5 Examples]
- How to Use Flags in Bash If Condition? [With Example]
- Learn to Compare Dates in Bash [4 Examples]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial