FUNDAMENTALS A Complete Guide for Beginners
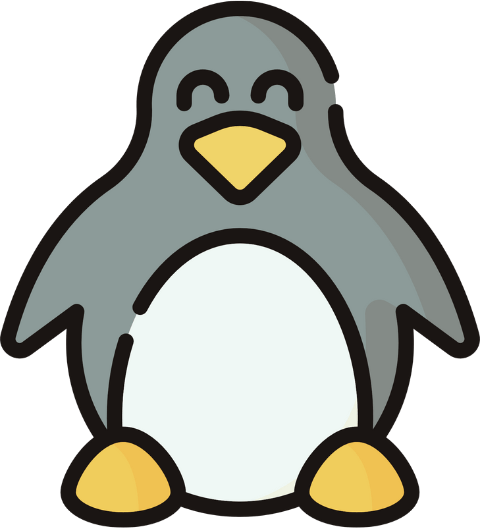
Using loops with if statements is a powerful mechanism in Bash for executing conditional logic over a series of data or as long as a condition evaluates to true. Bash provides different loop structures that can be combined with if statements to automate iterative tasks while making decisions.
In this article, I’ll cover the basics as well as different examples of using loops with if statements in Bash.
Understanding Bash Loops
Loops are fundamental constructs in Bash that can be used to iterate over a collection of data or execute a block of code repeatedly until a particular condition is met. By leveraging loops, a user can automate repetitive tasks, handle repetitive data processing, and control the flow of Bash scripts.
If Statement with Different Loops in Bash
In Bash scripting, using different loop structures with if statements can help execute different conditional logic effectively. Generally, Bash provides 3 types of loop constructs such as for loop, while loop, and until loop which can be combined with if statements.
Following are the basic structures of these loops with if statements in Bash:
-
“for” Loop with If Statement
for variable in [sequence]; do
#Code to execute for each iteration
if [ condition ]; then
#Code to execute if the condition is true
fi
done
-
“while” Loop with If Statement
while [ condition ]; do
#Code to execute while the condition is true
if [ condition ]; then
#Code to execute if the condition is true
fi
done
-
“until” Loop with If Statement
until [ condition ]; do
#Code to execute until the condition is true
if [ condition ]; then
#Code to execute if the condition is true
fi
done
Loop Control Statement
Loop control statements allow users to control the execution and flow of loops within scripts. There are mainly two loop control statements in Bash i.e. continue statement and break statement. Following is a detailed explanation of these two statements:
1. Continue Statement
The continue statement is used to jump to the following iteration, leaving the remaining code with the current iteration behind. This statement is particularly useful when you need to bypass certain conditions within a loop without ending the entire loop.
Following is a Bash script with the ‘continue’ statement:
#!/bin/bash
#'continue' statement in a loop
for n in {1..4}; do
if [ $n -eq 2 ]; then
echo "Iteration skipped for n = $n"
continue #Skipping rest of the code in this iteration
fi
echo "Iteration $n"
done
In the script, for n in {1..4}; do
initiates a for loop that iterates over the numbers from 1 to 4. Inside the loop, if [ $n -eq 2 ]
then checks if the current value of n is equal to 2. If the condition is true, the continue statement executes causing the script to skip the remaining code in the current iteration and move to the next iteration.
The above image states that the continue statement has skipped one iteration when n=2 and jumps to the next iteration.
2. Break Statement
The break statement is used to terminate the innermost loop, immediately exiting the execution of the loop.
Here is a Bash script with the ‘break’ statement:
#!/bin/bash
#'break' statement in a loop
for n in {1..6}; do
echo $n
if [ $n -eq 2 ]; then
break
fi
done
In the script, for n in {1..6}; do
initiates a for loop that iterates over the numbers from 1 to 6. Inside the loop, if [ $n -eq 2 ]
then checks if the current value of n equals 2. If the condition is true, the break statement executes causing the loop to exit immediately.
The above image states that the break statement has terminated the loop after the second iteration.
5 Examples of Loops with If Statements in Bash
Following are 5 examples demonstrating the versatility of combining loops with if statements in Bash:
1. Processing Files in a Directory
Processing files in a directory requires iteration over each file to perform particular actions. Combining loops with if statements ensures that each file is processed selectively according to the conditions or attributes of the file.
Check out the following script to iterate through files and automate the task of file processing in a directory:
#!/bin/bash
#Checking the regular files in a directory and printing their sizes
for file in /home/nadiba/new_dir/*; do
if [ -f "$file" ]; then
echo "File location: $file"
echo "File size: $(du -h "$file" | cut -f1)"
fi
done
Here, for file in /home/nadiba/new_dir/*; do
specifies the iteration through each file in a directory. Inside the loop, if [ -f "$file" ]
checks if the current item in the directory is a regular file. If the condition is satisfied, the script prints the file name with the location and its size using the $(du -h "$file" | cut -f1)
syntax where the du command calculates the disk usage of each file, the -h
flag converts the output into a human-readable format and cut -f1
extracts the size of the files from the output.
From the image, you can see all the files existing in the home directory in my system with their file size.
2. Reading Lines from a File in Bash
To read lines from a file, use a loop with an if statement in Bash. But before reading lines from a file you must ensure that the file exists in the system. Otherwise, it will cause error.
Here’s a Bash script showing how to read lines from a file using a while loop in Bash:
#!/bin/bash
file_name="file1.txt"
#Checking if the file does not exist
if [ ! -f "$file_name" ]; then
echo "$file_name does not exist."
exit 1
fi
#Reading lines from the file
while IFS= read -r line; do
#Checking for empty lines
if [ -z "$line" ]; then
continue #Skipping rest of the code for this iteration
fi
#Printing non-empty lines
echo "$line"
done < "$file_name"
Here, while IFS= read -r line; do
initiates a while loop that reads lines one by one from the file where IFS=
ensures preserving the leading and trailing whitespaces and -r
ensures preventing backslash escaping. Then, inside the loop, if [ -z "$line" ]
checks if the current line is empty, and if it is empty, the continue statement executes. If the line is not empty, the script prints the line employing the echo command.
In the above image, you can see all the lines in the file file1.txt in my system.
3. Skipping Even Numbers with “for” Loop
To skip even numbers and print only the odd numbers, first, it is necessary to figure out which numbers are odd and which are even. This can be accomplished by using a modulus operator % that calculates the remainder of a division between two integers.
Here’s how to skip even numbers using a for loop in Bash:
#!/bin/bash
#A for loop to iterate from 1 to 15
for ((n=1; n<=15; n++)); do
if ((n % 2 == 0)); then
continue
fi
echo "$n"
done
In this script, for ((n=1; n<=15; n++)); do
initializes a for loop to iterate through numbers from 1 to 15, incrementing by 1 in each iteration. Next, inside the loop, if ((n % 2 == 0))
checks if the remainder of n divided by 2 is equal to zero. If the remainder is zero, it means n is even and the continue statement executes skipping the remaining code for the current iteration. echo "$n"
prints the value of n only when it is odd.
In the image, all the odd numbers from 1 to 15 are highlighted, while the even numbers are missing.
4. Validating User Input Using “until” Loop
User input validation is such an aspect that ensures that a program gets the real data. In Bash, using the until loop is a great way to ask users for input repeatedly until the the correct information is provided.
Navigate through the following Bash script to validate user input using an until loop with an if statement:
#!/bin/bash
#Defining regular expression pattern
pattern="^[0-9]+$"
#Declaring variables
value=""
trial=0
#An until loop to repeatedly prompt the user until a valid input is entered
until [[ "$value" =~ $pattern ]]; do
read -p "Enter any number: " value
((trial++)) #Incrementing the value of 'trial'
#Checking if the entered value does not match the pattern
if ! [[ "$value" =~ $pattern ]]; then
echo "Invalid! Please enter a valid number"
fi
#Checking if maximum trial exceeds
if [ "$trial" -ge 3 ]; then
echo "Maximum trial exceeds! No more chance"
exit 1
fi
done
Here, until [[ "$value" =~ $pattern ]]; do
initiates an until loop that iterates until the entered value matches the specified pattern that is defined as any sequence of digits (0-9). When a user is prompted to enter a number, the script ensures that it becomes a valid number within three attempts. Inside the loop, if ! [[ "$value" =~ $pattern ]]
checks if the entered input does not match the pattern. If the entered value is invalid, the script displays an error message prompting the user to enter a valid input.
As you can see from the image after executing the script once, when I entered 1, it displayed as a valid number. Then, again executing the script when I entered a or $, it displayed as invalid and when I entered 5, it showed maximum trial exceeded (3 attempts).
5. Skipping Specific Options Using “select” Loop
The select loop is a specialized loop that provides an interactive way to choose an option from a list. It doesn’t directly support if statements within the loop structure. However, this loop structure can be used with an if statement to handle the behavior of the specific options.
To skip specific options using the select loop, review the Bash script below:
#!/bin/bash
linux_distro=("Arch linux" "Ubuntu" "Kali Linux" "Quit")
select distro in "${linux_distro[@]}"; do
#Checking if the selected distro is 'Ubuntu'
if [[ "$distro" == "Ubuntu" ]]; then
echo "Not available! Select another distro."
continue #Skipping the rest of the code for 'Ubuntu'
fi
#Checking if the selected option is 'Quit'
if [[ "$distro" == "Quit" ]]; then
echo "Exit!"
break #Exiting the loop
fi
#Printing the selected option for other distros
echo "Selected distro: $distro"
done
In the script, select distro in "${linux_distro[@]}"; do
initializes a select loop and displays different options for selection. Inside the select loop, if [[ "$distro" == "Ubuntu" ]]
checks if the distro Ubuntu is selected. If Ubuntu is selected, it displays a message showing that Ubuntu is not available and the continue statement executes to skip code for this iteration. Next, if [[ "$distro" == "Quit" ]]
checks if Quit is selected. If so, it prints a message and uses the break statement to exit the loop. Except for these, the script prints the selected distro for all other options.
From the image, you can see that when I selected option 2 (Ubuntu), the script skipped the option from displaying; instead printed that it was not available.
Conclusion
Throughout the article, you have learned the fundamentals of using loops with if statements in Bash. Moreover, you have explored how to iterate over files, read lines from files, manipulate data, select or skip specific options etc. By mastering the use of loops with if statements, you can create sophisticated Bash scripts capable of automating complicated tasks and handling different scenarios effectively.
People Also Ask
What are loop control statements in Bash?
Loop control statements in Bash are those that are used to alter and control the flow of execution of loops. These statements are generally useful for controlling or skipping certain iterations. There are two types of loop control statements in Bash. They are the continue statement and the break statement.
Is it possible to use an if statement with nested loops in Bash?
Yes, it is possible to use an if statement with nested loops in Bash. For example:
#!/bin/bash
#Nested loop iterations
for ((p=1; p<=5; p++)); do #Outer loop to iterate over rows
echo "Row $p:"
for ((q=1; q<=5; q++)); do #Inner loop to iterate over columns
#Checking if the row number is even
if ((p % 2 == 0)); then
#If the row number is even, print the square of the column number
echo -n "$((q*q)) "
else
#If the row number is odd, print the column number
echo -n "$q "
fi
done
echo ""
done
Can I combine multiple if statements with loops in Bash?
Yes, you can combine multiple if statements with loops in Bash to create complex conditional logic and control the flow of the Bash scripts. For example:
#!/bin/bash
#Combining multiple if statements with loops
for ((n = 1; n <= 6; n++)); do
echo "Iteration $n"
if [ $n -eq 3 ]; then
echo "First condition met in iteration $n"
fi
if [ $n -lt 9 ]; then
echo "Second condition met in iteration $n"
fi
if [ $n -gt 4 ]; then
echo "Third condition met in iteration $n"
fi
done
When should I use loops within if statements in Bash scripts?
You should use loops within if statements when you need to perform conditional execution based on specific conditions that involve iterative or repetitive tasks. It enables dynamic script behavior, allowing you to deal with different scenarios effectively.
Related Articles
- Mastering 10 Essential Options of If Statement in Bash
- How to Check a Boolean If True or False in Bash [Easy Guide]
- Bash Test Operations in ‘If’ Statement
- Check If a Variable is Empty/Null or Not in Bash [5 Methods]
- Check If a Variable is Set or Not in Bash [4 Methods]
- Check If Environment Variable Exists in Bash [6 Methods]
- Bash Modulo Operations in “If” Statement [4 Examples]
- How to Use “OR”, “AND”, “NOT” in Bash If Statement [7 Examples]
- Evaluate Multiple Conditions in Bash “If” Statement [2 Ways]
- Using Double Square Brackets “[[ ]]” in If Statement in Bash
- 6 Ways to Check If a File Exists or Not in Bash
- How to Check If a File is Empty in Bash [6 Methods]
- 7 Ways to Check If Directory Exists or Not in Bash
- Negate an “If” Condition in Bash [4 Examples]
- Check If Bash Command Fail or Succeed [Exit If Fail]
- How to Write If Statement in One Line? [2 Easy Ways]
- How to Use Flags in Bash If Condition? [With Example]
- Learn to Compare Dates in Bash [4 Examples]
<< Go Back to If Statement in Bash | Bash Conditional Statements | Bash Scripting Tutorial